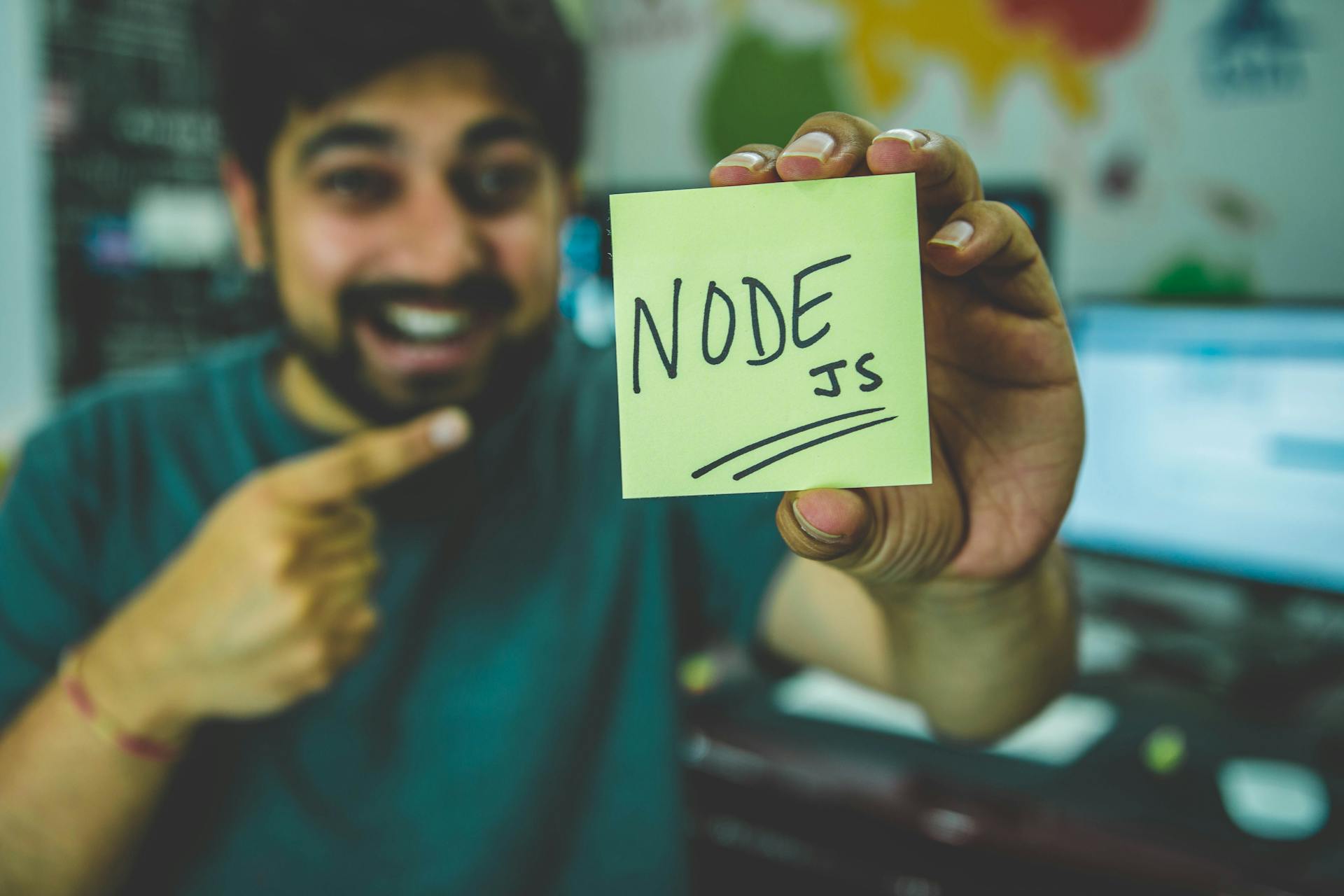
Developing a Next.js application with Drizzle can be a game-changer for efficient development. Drizzle is a powerful library that makes it easy to manage state and interactions with your database.
One of the key benefits of using Drizzle with Next.js is that it allows for easy integration with various databases, including PostgreSQL, MySQL, and MongoDB. This flexibility is a major plus for developers who need to work with different data sources.
Drizzle's data fetching capabilities can significantly speed up development time by automatically handling API calls and caching data. This means you can focus on building your application's features rather than worrying about the underlying database interactions.
By leveraging Drizzle's features, you can create a seamless user experience and improve the overall performance of your Next.js application.
For another approach, see: Next Js Drizzle Supabase
Database Setup
To set up a database with Drizzle, you'll need to create a file called drizzle.config in the root of your project. This file requires a schema field to identify where your project's database schema is defined, which can be a single file or split into multiple files using glob patterns.
Consider reading: Can Amazon S3 Take in Nextjs File
The out field in the config determines where your migration outputs will be stored, and it's recommended to put them in a folder in the same directory as your schema. You'll also need to specify a driver and dbCredentials.connectionString to tell Drizzle what APIs to use and where your database lives.
To get the connection string, you can use a service like Vercel's Postgres offering, which sets environment variables that you can pull into your local development environment. Alternatively, you can use a different database hosting solution and follow their instructions for fetching the connection string.
For a local database, you can use Docker Compose to set up a database that can be easily replicated across different machines. You'll need to create a docker-compose.yaml file to define the database.
To define your database schema, create a file called db/schema.ts in the root directory. This file can contain tables, such as a users table, which you can populate later. You can use text for the id field if your database provider, like Kind, provides an id string rather than an integer.
To set up the database, create a file called db/index.ts in the root directory. This file will set up an sqlite3 database and connect it to Drizzle. To explore the data in your database, you can run npx drizzle-kit studio.
You might enjoy: Next Js Upload File
Database Connection
In a Next.js app, creating a database connection is crucial for operations. This connection instance is typically stored in a file that keeps related database files together.
To make operations work, we need a database connection instance. This instance is often stored in a file like db/drizzle.ts.
We use 2 different Drizzle adapters depending on the environment - the generic PostgreSQL adapter for local development and the Vercel adapter for production. These adapters have different initializers but the same output interface.
The same adapter can be used for both local and production environments if we choose a RDS or similar PostgreSQL solution, just by changing the connection string.
A different take: Save Api Data on Local Storage Next Js
Database Management
To create database tables with Drizzle, you'll want to leverage its Migrations feature, which allows for atomic changes to the database as the schema evolves.
This involves defining schema changes in your schema files, which are then used to generate a migration SQL file. You should check this file into source control.
You might enjoy: Next Js Db
Drizzle doesn't currently allow overriding migration names, so if you want a more descriptive name, you'll need to rename the migration file and update the _journal.json file in the migration folder.
To connect to the correct database, you'll need to specify the migrations folder location, which can be done using the drizzle migrate command.
Here's a quick rundown of the steps involved:
- Rename the migration file and take note of the old name.
- Update the _journal.json file in the migration folder to reflect the new file name.
In local development, it's recommended to set the connection pool to max at 1, and to close the connection to the db when you're done.
Using a Database with Next.js
You can use a PostgreSQL-compatible database like Neon to store and manage your application's data. Neon offers scalability and ease of management, making it a great choice for building scalable web applications.
To get started, create an account on Neon and create a new database. You'll receive a connection string that you can add to your .env.local file.
Drizzle ORM is another great option for working with databases in Next.js. It provides a smooth database interaction experience and allows you to write more intuitive and maintainable database queries. To set up Drizzle ORM, create a new folder named database in your root directory and create a new file drizzle.ts.
Recommended read: Nextjs File Upload Api
Here are the steps to set up Drizzle ORM with Neon:
- Install Drizzle ORM using npm or yarn.
- Create a new file drizzle.ts in the database folder.
- Create the schema within the database folder.
- Create a drizzle.config.ts file in the root of your app.
- Add the following script to your package.json file.
- Run the following command to create the database schema.
Using
To use a database with Next.js, you'll need to make some changes to your project settings. Update your tsconfig.json file to change the "target" from es5 to es6, as Drizzle does not yet support es5.
Drizzle is a great tool for saving user data to a local Sqlite3 database, and it's easy to get started. You can find more information on how to use it in the DrizzleORM docs.
After updating your environment variables, be sure to restart the dev server to see the changes take effect.
Consider reading: How to Use Reducer Api in Next Js 14
Using ORM with PlanetScale and NextAuth
Using an Object-Relational Mapping (ORM) tool like Drizzle with PlanetScale and NextAuth can be a bit tricky, but it's definitely doable. To get started, you'll need to install the necessary dependencies, including Drizzle and NextAuth.
Drizzle Kit allows you to alter your database schema with a simple command, so you'll need to add `db:push` to your `package.json` file. This will enable you to push your database schema changes to PlanetScale.
To create a Drizzle Adapter for NextAuth, you'll need to install another dependency for generating unique IDs. This will allow you to create a connection between Drizzle and NextAuth.
Here are the steps you'll need to follow:
- Install `dotenv` and import it in your Drizzle configuration
- Add your database URL to an environment variable file
- Create a `db` folder and add `index.ts` and `schema.ts` files
- Create a `lib` folder and add an `auth` folder with `drizzle-adapter.ts` and `index.ts` files
- Declare the `next-auth` and `next-auth/jwt` modules in a `types` folder
By following these steps, you'll be able to use Drizzle with PlanetScale and NextAuth.
Frequently Asked Questions
Is drizzle orm fast?
Drizzle ORM is designed to be fast, with minimal runtime overhead and no n+1 problem for relational queries
Sources
- https://www.thisdot.co/blog/configure-your-project-with-drizzle-for-local-and-deployed-databases
- https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth/
- https://medium.com/@pether.maciejewski/clerk-next-js-14-and-drizzle-orm-boilerplate-3fdeb90b0de0
- https://www.fullstackbook.com/blog/next-auth-drizzle-sqlite-tutorial
- https://dev.to/miljancode/drizzle-orm-next-auth-and-planetscale-2jbl
Featured Images: pexels.com