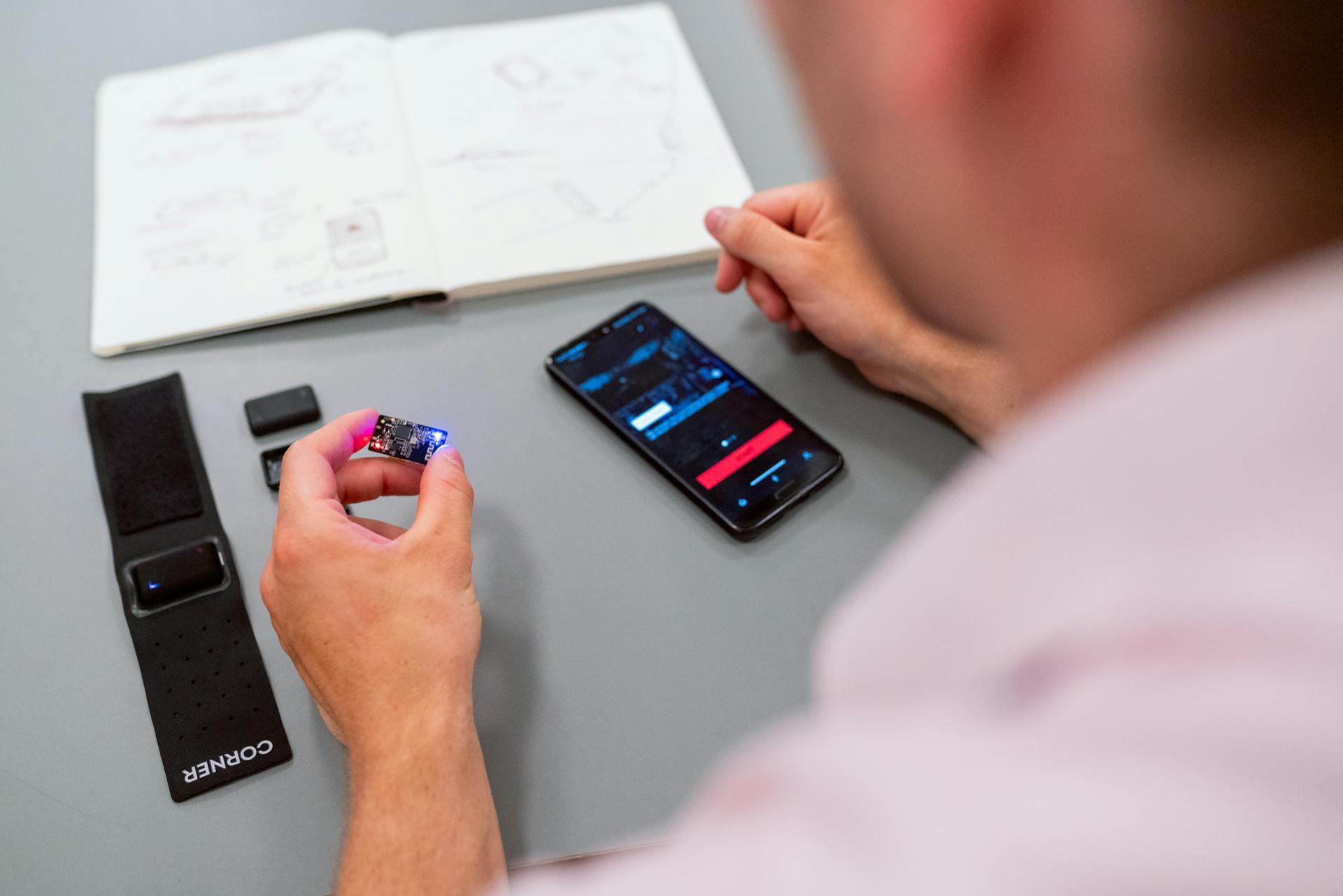
Setting up file upload in Next.js can be a bit tricky, but don't worry, I've got you covered.
To start, you'll need to create a new API route to handle file uploads. This can be done by running `npx next generate api` in your terminal.
File uploads in Next.js require a server-side approach, which is why API routes are a crucial part of the setup.
For security reasons, it's essential to validate and sanitize user-uploaded files to prevent potential security breaches. This can be achieved by using a library like `multer` to handle multipart/form-data requests.
Next.js Setup
To set up Next.js for file upload, you'll need to initialize the Next.js SDK. This requires obtaining the necessary initialization parameters, such as the urlEndpoint, which can be found in the URL-endpoint section or the developer section on your ImageKit dashboard.
The urlEndpoint is a required parameter, and you can also obtain the publicKey from the Developer section on your ImageKit dashboard if you want to use the SDK for client-side file upload. Be aware that passing the privateKey parameter while initializing the SDK will throw an error.
Here are the components you can import individually for Next.js setup:
- IKImage for image rendering
- IKVideo for video resizing
- IKUpload for file uploading
- ImageKitProvider to define options such as urlEndpoint, publicKey, or authenticator
- ImageKitClient to expose methods from ImageKit javascript SDK like url and upload
- ImageKitContext to provide access to options such as urlEndpoint, publicKey, ikClient or authenticator to child components within ImageKitProvider
File Handling
To handle file uploads in Next.js, you can use Server Actions. This approach allows you to store files locally on the server in the uploads directory, or even save them to a CDN location.
To get started, create a new directory called public/uploads to store the uploaded files. This is where the uploaded files will be saved.
When you upload a file, Next.js takes care of submitting the form data to your server action, which receives the form data, extracts the uploaded file, and saves it to the public/uploads directory.
File
Uploading files in Next.js can be done using the IKUpload component, which renders an input type="file" tag that you can use to upload files to the ImageKit media library directly from the client side.
To implement this functionality, you need a backend server to authenticate the request using your API private key. This can be done using the IKUpload component, which you can import from the SDK into your Page.js file.
You can validate files before upload using the validateFile prop, which allows you to add arbitrary validation such as file type, file size, and file name. For example, you can prevent upload if the file size is bigger than 2 MB.
File upload can be handled by letting users select a photo and upload it, and then storing the photo locally on the server in the uploads directory. This can be achieved by modifying your page.tsx file to include a Server Action to receive user submitted form data and save the file to the uploads directory.
To use Server Actions, you need to modify your next.config.js file accordingly. This will allow you to trigger server actions when users submit forms, and create files in the uploads directory.
Handling
You can validate a file before uploading it by using the validateFile prop, which allows you to check for arbitrary properties such as file type, size, and name.
To prevent a file from being uploaded if it's too large, you can set the validateFile prop to check for a maximum file size, like 2 MB.
To handle file uploads with server actions, you need to create a server action that receives form data and extracts the uploaded file.
This server action can then convert the file to a byte array and save it to a designated directory, such as public/uploads.
You can wire up the action to your form by setting the action attribute of the form, which will submit the form data to your server action.
By doing this, you can save the uploaded file to the public/uploads directory.
Frontend Configuration
To configure the frontend for file upload in a Next.js app, you'll need to initialize the SDK with authentication parameters. This involves adding specific code to the app/page.js file.
Add the publicKey and authenticator values as props to a new ImageKitProvider instance, which will hold the upload component. This instance will be used to manage file uploads.
In the app/page.js file, you'll see how these values are passed into the ImageKitProvider instance, making it ready for file uploads.
Displaying Images
To display uploaded images, you need to iterate through the public/uploads directory and create a list of image URLs.
First, map over the image URLs and render them below the upload form on your homepage. This will show the existing images.
Uploading a new file won't make it show automatically, so you'll need to revalidate the homepage route after each upload.
To fix this, add a call to revalidatePath to the uploadFile function in app/upload-action.ts.
Configure Frontend App Authentication
To configure authentication in the frontend app, you need to add the publicKey and authenticator to the SDK. This is done by initializing the SDK with auth params in the app/page.js file.
Add the following code to initialize the SDK with auth params:
Now, pass these values as props into a new ImageKitProvider instance, which will hold our upload component.
The ImageKitProvider instance should be configured like this.
Custom Button
To create a custom upload button, you can use the IKUpload component and assign a reference to the input element, such as ikUploadRefTest. This allows you to style the default file selector using className or style, and then use the custom upload button as needed.
You can hide the default file selector by adding a style attribute with display set to 'none'. This will remove the default file selector from view, making way for your custom upload button.
Show Progress Bar
Showing progress to the end user can be as simple as passing the onUploadProgress prop, which includes a ProgressEvent. This event can be used to calculate the percentage of upload progress.
The onUploadProgress prop can be used to display a progress bar to the user, giving them a sense of how much longer the upload will take.
Additional Options
When passing parameters to the ImageKit Upload API, you can also include additional options to customize the upload function.
You can pass parameters like extensions, webhookUrl, and customMetadata to the upload function.
Extensions allow you to specify file types that are allowed or blocked during the upload process.
Custom metadata can be used to add extra information to your uploaded files, such as tags or descriptions.
This can be particularly useful when you need to filter or categorize your uploaded files later on.
Sources
- https://jaluwibowo.id/blog/en/upload-next-shadcn-to-aws-s3/
- https://imagekit.io/docs/integration/nextjs
- https://stackoverflow.com/questions/77260715/how-to-upload-a-fileimage-with-nextjs
- https://createappai.com/blog/file-upload
- https://www.pronextjs.dev/workshops/pro-next-js-workshop-hl06z/file-uploads-in-next-js-app-router-apps-vqozo
Featured Images: pexels.com