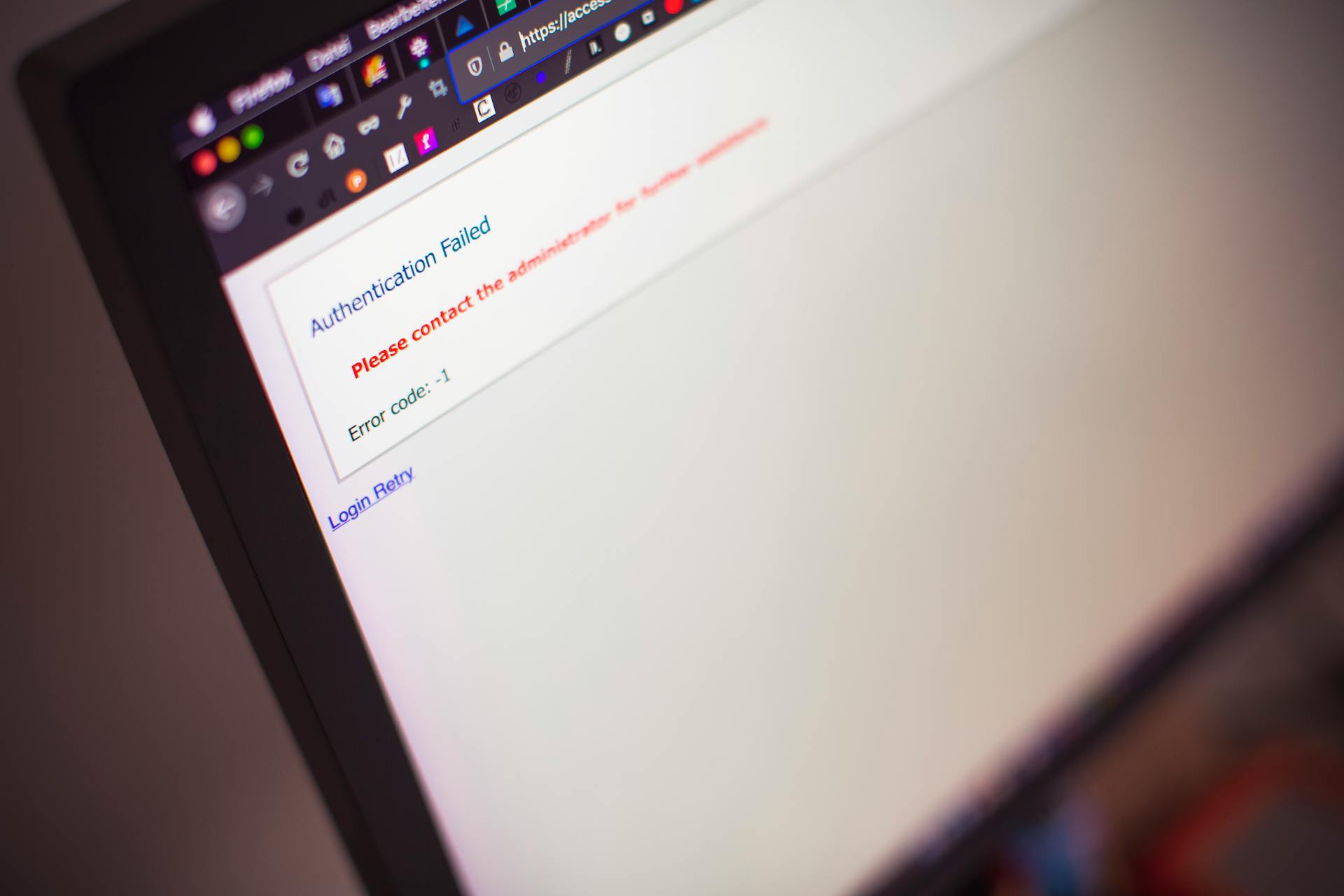
Next.js auth providers with AWS Amplify and Cognito offer a seamless authentication experience for users.
You can use AWS Amplify to integrate AWS Cognito into your Next.js app.
AWS Amplify provides a simple and secure way to add authentication to your Next.js app.
With AWS Amplify and Cognito, you can easily manage user authentication and authorization.
You can use AWS Amplify's built-in support for AWS Cognito User Pools to create a user pool and manage user authentication.
AWS Cognito User Pools provide a scalable and secure way to manage user authentication for your Next.js app.
A unique perspective: Next Js Aws Amplify Gen2 Architecture Diagram
Authentication Providers
Authentication Providers are a crucial aspect of Next.js applications, allowing users to log in and interact with your app securely. NextAuth.js supports a variety of authentication providers, making it easy to implement different login methods for your users.
You can choose from popular options like Email and Password (Credentials), Magic Links via Email, and Social Media Logins (e.g., Twitter, Facebook, Google). Let's explore these options in more detail.
Here are some popular authentication providers supported by NextAuth.js:
- Email and Password (Credentials)
- Magic Links via Email
- Social Media Logins (e.g., Twitter, Facebook, Google)
These providers can be easily implemented in your Next.js application, providing a seamless and secure user experience.
Next
Next, let's talk about Next.js and authentication providers. Next.js is a popular framework for building server-rendered and statically generated React applications. One of its key features is integration with authentication providers, which allows you to add authentication to your app with ease.
Next.js has a built-in integration with NextAuth.js, a popular authentication library for Next.js. NextAuth.js supports a wide range of sign-in providers, including OAuth 1.0, 2.0, OIDC, email/passwordless authentication, and more.
To get started with NextAuth.js, you'll need to install the dependency and set up the configuration file. This can be done by creating a file called [...nextauth].js in the pages/api/auth directory. This file will handle all authentication-related requests, such as sign in and sign out.
NextAuth.js also provides a SessionProvider component that makes the authentication session available throughout your Next.js app. This can be achieved by wrapping your entire application with the SessionProvider component.
Here are some popular authentication providers supported by NextAuth.js:
- Email and Password (Credentials)
- Magic Links via Email
- Social Media Logins (e.g., Twitter, Facebook, Google)
By using NextAuth.js and one of these authentication providers, you can easily add authentication to your Next.js app and protect your routes and components.
Auth by AWS Amplify
Auth by AWS Amplify is a powerful solution built on AWS. It's powered by Amazon Cognito, which handles user registration, authentication, account recovery and other operations.
Auth by AWS Amplify requires a bit more work to integrate into Next.js. However, it's worth the effort for the robust features it offers.
Amazon Cognito handles user registration, which is a crucial aspect of authentication. This includes creating new user accounts and managing existing ones.
Here are some key benefits of using Auth by AWS Amplify:
- https://docs.amplify.aws/nextjs
Configuring Providers
To set up the SessionProvider, you need to wrap your entire application with it. This component makes the authentication session available throughout your Next.js app.
You can configure the SessionProvider by creating an AuthProvider.tsx file and inputting the code. This will flag the SessionProvider as a Client Component and make it available for use.
The NextAuth SessionProvider component is a client component that uses the Context API. It's exported from next-auth/react because it only works on the client.
Here's an interesting read: Nextjs Pathname Server Component
You can solve the error of Context API being unavailable in Next.js 14 by flagging the SessionProvider as a Client Component and then exporting it.
Here's a list of the steps to configure the SessionProvider:
- Create an AuthProvider.tsx file and input the code.
- Flag the SessionProvider as a Client Component.
- Export the SessionProvider.
Supported database providers include Prisma, PostgreSQL, SQLite, and MongoDB. You can choose the one that aligns with your tech stack and requirements.
You can edit the PostgreSQL configuration file to set up the database adapter.
Take a look at this: Next Js Database
Callbacks and Routes
Callbacks in NextAuth.js allow you to control the authentication flow with asynchronous functions.
You can use callbacks to implement access controls, integrate with external databases or APIs, and customize the behavior of the authentication flow.
The signIn callback is used to control whether a user is allowed to sign in or not, and it receives parameters such as user, account, profile, email, and credentials.
You can use the signIn callback to implement custom logic for allowing or denying user sign-in based on specific conditions, such as checking if the email address is already in the database.
You might like: Next Js Useeffect
The redirect callback is called anytime the user is redirected to a callback URL, and by default, Auth.js only allows URLs on the same origin as the site.
You can use the redirect callback to customize this behavior and allow or deny specific redirect URLs.
Next.js provides two primary methods for protecting routes in your application: protecting routes with middleware and using the useSession hook.
Protecting routes with middleware allows you to run some logic before processing a request, similar to middleware in backend frameworks.
To use middleware in Next.js, create a middleware.ts file and update it with the following code, where you define the routes you want Next.js middleware functionality to apply to.
The matcher property dictates the paths — and subpaths — to which the middleware function should be applied.
To protect a route, create an auth instance from your middleware file that doesn’t have a database adapter attached to it, and then initialize it in your middleware.
The middleware code works similarly to the strategy we used in the auth() call in layout.tsx, and the key advantage is that the logic for protecting routes is now centralized and not scattered across several layout.tsx files.
You might enjoy: Can Amazon S3 Take in Nextjs File
The useSession hook is the simplest way to check the logged-in state, and if the user isn't logged in, show a Sign in button with a click handler.
By using the useSession hook, you can easily protect routes and pages based on user authentication status.
To use the useSession hook, simply import it and call it in your component, passing the authentication provider as an argument.
The useSession hook returns the logged-in session, or null if the user isn't logged in.
Magic Links and Emails
Magic links with Auth.js can be set up quickly using the Resend provider, which requires creating a Resend account and registering a new API key for your app.
To use the Resend provider, add your API key to your .env.local file under AUTH_RESEND_KEY, and update your auth.ts file with the provided code.
With the Resend provider, you can send pre-made test emails exclusively to your email address using the free [email protected] domain.
Recommended read: Next Js Provider
Customizing magic link emails in Auth.js is also possible by using the sendVerificationRequest function present in the EmailProvider options.
For full customizability, you can use the sendVerificationRequest function to send out the magic link emails as needed.
To set up an authentication provider for magic links, add the Email provider to the providers array in your authOptions configuration, specifying the sender's email address and maxAge for the magic link expiration time.
The from option in the Email provider specifies the sender's email address, and maxAge sets the expiration time for the magic link, which is 2 hours in this case.
A custom function, sendLoginEmail, can be used to send the login email, which extracts necessary information from the params object and constructs a simple HTML email template with the login link.
To complete the magic link authentication flow, create a login page where users can enter their email address to receive the magic link, and use the signIn function from next-auth/react to initiate the authentication flow.
The callbackUrl parameter specifies the page to redirect to after successful authentication, which in this case is the /protected route that we'll create soon.
Curious to learn more? Check out: Next Js 14 Redirect to Another Page Loading Indicator
Database Providers
NextAuth.js offers a range of database providers to choose from, including Prisma, PostgreSQL, SQLite, and MongoDB.
Prisma is a modern database toolkit that simplifies database management and provides a type-safe API for querying and mutating data. It supports multiple databases, including PostgreSQL, MySQL, and SQLite.
The database adapters for NextAuth.js are flexible and can be easily switched between different databases, giving you the freedom to choose the one that best fits your application's requirements.
Here are some of the popular database providers supported by NextAuth.js:
- Prisma: Supports multiple databases, including PostgreSQL, MySQL, and SQLite.
- PostgreSQL: A powerful, open-source relational database known for its reliability, scalability, and extensive feature set.
- SQLite: A lightweight, file-based relational database suitable for development and testing environments.
- MongoDB: A popular NoSQL database that offers flexibility and scalability.
Amazon Cognito
Amazon Cognito is a crucial component in our authentication flow. To set it up, log into the AWS console and click Manage User Pools.
You'll need to create a new user pool if you don't already have one. Click 'Create a user pool' and enter the pool name, then select Review Defaults. Make sure to select Add app client, which will contain the details required to hook the client up to the authentication service.
Expand your knowledge: Next Js Client Portal
Ensure you enter an App client name and generate a client secret. Select Return to pool details and click 'Create pool'. Next, set up a domain by selecting 'Domain name' from the left-hand menu and entering a domain name.
Check the availability of the domain name and save it for later use. Finally, click 'Save changes' and select App client settings to retrieve the App client id and secret.
Here's a summary of the steps:
- Log into the AWS console and click Manage User Pools
- Create a new user pool and select Add app client
- Enter an App client name and generate a client secret
- Set up a domain and save it for later use
- Retrieve the App client id and secret from App client settings
These steps will get you set up with Amazon Cognito.
Supported Database Providers
NextAuth.js offers adapters for various databases, giving you the flexibility to choose the one that aligns with your tech stack and requirements. Some popular database providers supported by NextAuth.js include Prisma, PostgreSQL, SQLite, and MongoDB.
Prisma is a modern database toolkit that simplifies database management and provides a type-safe API for querying and mutating data. It supports multiple databases, including PostgreSQL, MySQL, and SQLite.
Suggestion: Next Js Sqlite
PostgreSQL is a powerful, open-source relational database known for its reliability, scalability, and extensive feature set. NextAuth.js provides adapters for using PostgreSQL directly or through ORMs like Prisma.
SQLite is a lightweight, file-based relational database that is easy to set up and suitable for development and testing environments. NextAuth.js offers adapters for SQLite, making it convenient for local development.
MongoDB is a popular NoSQL database that offers flexibility and scalability. NextAuth.js provides adapters for MongoDB, allowing you to store user and session data in a document-oriented format.
Here's a brief rundown of the supported database providers:
Route Protection and Access
Route protection is a crucial aspect of any Next.js application, especially when using authentication providers like NextAuth.js. You can protect routes using middleware in Next.js, which runs some logic before processing a request.
To use middleware in Next.js, create a middleware.ts file at the root of your application or in the src directory. This file will hold the logic for protecting routes, making it centralized and easier to manage.
On a similar theme: Can Nextjs Be Used on Traditional Web Application
NextAuth.js provides a middleware feature that allows you to protect routes based on user authentication status. This middleware can be used to check if a valid token exists, indicating that the user is authenticated.
To protect routes with middleware, you'll need to define the routes you want to apply the middleware to in the config object. The matcher property dictates the paths and subpaths to which the middleware function should be applied.
You can also use the useSession hook to check the logged-in state, which is the simplest way to determine if a user is authenticated. If the user isn't logged in, you can display a Sign in button with a click handler that redirects to a protected page.
To test the sign-up or sign-in process, run the development server and go to http://localhost:3000/. If you select Sign in, you should be able to log in with an existing account or sign up for a new account using a provider like Cognito.
Token Management
Token Management is a crucial aspect of Next.js authentication. By default, NextAuth.js sessions expire after 30 days.
To handle session expiration and refresh tokens, you can configure the session and jwt options in your NextAuth.js configuration. This ensures users are logged out when their session expires and prompted to re-authenticate when necessary.
Remember to choose appropriate session and token expiration durations based on your application's security needs and user experience considerations. This will help you strike a balance between security and user convenience.
NextAuth.js will handle session expiration and refresh tokens automatically, so you don't have to worry about manually updating tokens or sessions. This saves you time and effort in the long run.
Here's an interesting read: Nextjs Session
Social Login
You can add social login options to your Next.js app using the respective providers from next-auth/providers.
To configure Google, Twitter, and Facebook providers, you'll need to install the necessary provider packages.
You'll also need to update your pages/api/auth/[...nextauth].js file to include the desired providers.
Obtaining the necessary API keys and secrets from the respective developer platforms is a crucial step.
For Google, you'll need to create a new project in the Google Developer Console and set up OAuth 2.0 credentials.
You'll obtain the client ID and client secret, which you'll then add to your .env.local file.
Similarly, for Twitter, you'll need to create a new app in the Twitter Developer Portal and obtain the consumer key and consumer secret.
For Facebook, you'll create a new app in the Facebook Developer Portal and obtain the app ID and app secret.
Creating login buttons for each provider in your login page component is the next step.
When a user clicks on a provider's login button, the signIn function from next-auth/react is called with the provider's name.
Here's a quick rundown of the login flow:
- The user is redirected to the provider's login page.
- After successful authentication, the user is redirected back to your app's callback URL.
- NextAuth.js creates a session for the authenticated user and redirects them to the specified callbackUrl (default is /).
By following these steps, you've successfully added social login options to your Next.js app using NextAuth.js.
Sources
- https://authjs.dev/getting-started/migrating-to-v5
- https://blog.logrocket.com/auth-js-client-side-authentication-next-js/
- https://ilyagru.com/blog/12-great-auth-libraries-to-use-with-next-js
- https://www.pedroalonso.net/blog/authentication-nextjs-with-nextauth/
- https://darrenwhite.dev/blog/nextjs-authentication-with-next-auth-and-aws-cognito
Featured Images: pexels.com