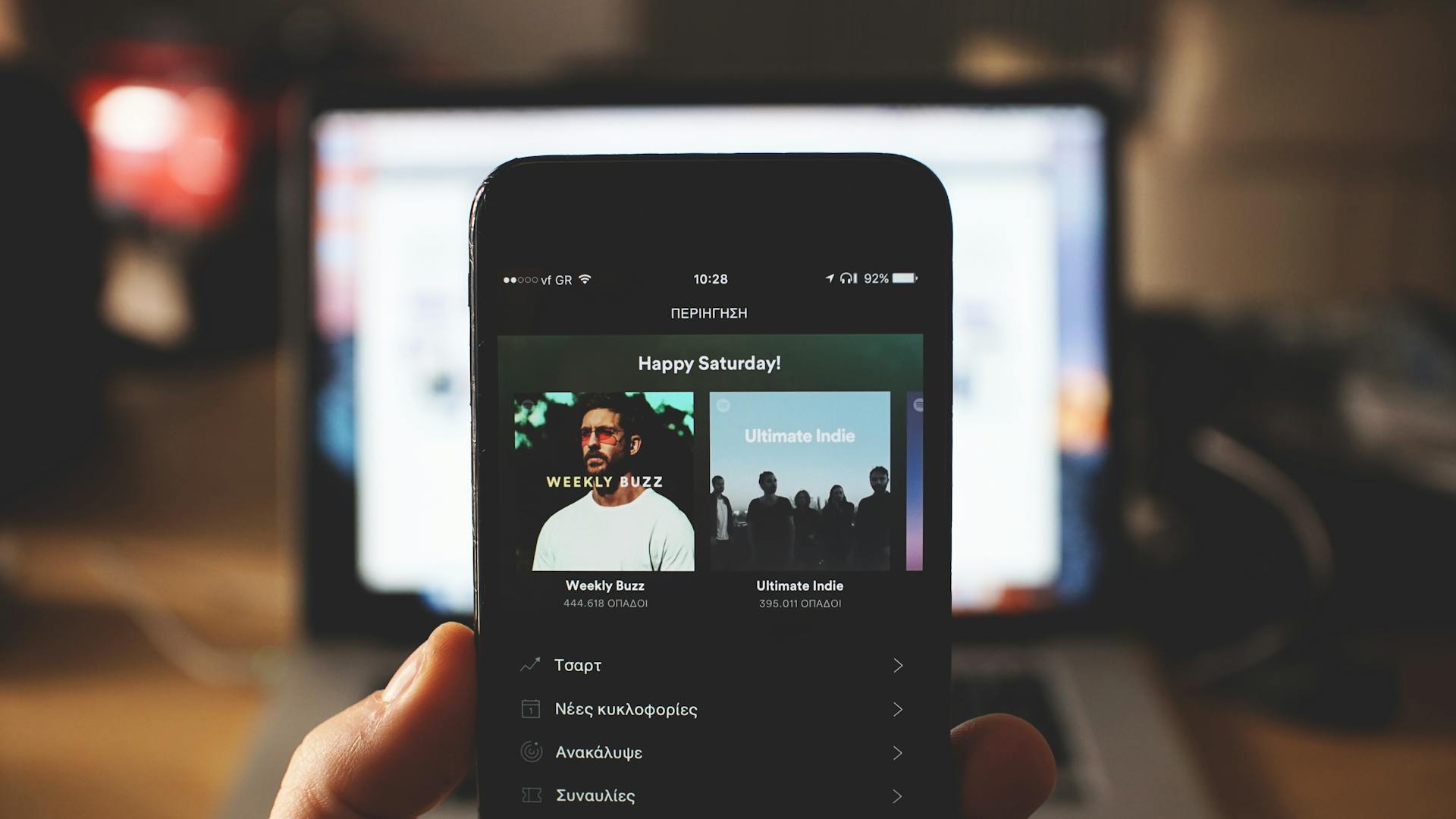
To implement Spotify Auth in your Next.js app, you'll need to create a new project using the `create-next-app` command with the `--experimental-app` flag. This flag enables experimental features, including support for Spotify Auth.
First, install the required packages, including `@spotify/auth` and `@spotify/web-api-auth-js`. These packages provide the necessary functionality for authenticating users with Spotify.
Next, create a new file called `spotify-auth.js` to handle the authentication flow. This file will be responsible for redirecting the user to the Spotify authorization page and handling the resulting code.
In this file, you'll need to set up an instance of the `SpotifyAuth` class, passing in your client ID and redirect URI. This will allow you to authenticate users and obtain an access token.
Readers also liked: Next Js Send Email
Getting Started
To get started with Spotify Auth in Next.js, you'll need to install the required packages, including `@spotify/auth-api-js` and `next-auth`.
First, create a new Next.js project using the `create-next-app` command. This will give you a basic setup to work with.
Consider reading: Next.js
Next, install the required packages by running `npm install @spotify/auth-api-js next-auth` in your project directory.
Make sure to configure NextAuth with Spotify as a provider by adding the `Spotify` provider to your `pages/api/auth/[...nextauth].js` file.
Then, create a new file called `spotify.js` to handle the authentication flow. This file will contain the logic for redirecting the user to the Spotify authorization page and handling the redirect back to your app.
If this caught your attention, see: Next Js Mysql
Setup Authentication
To set up authentication for your Spotify app in Next.js, you'll need to create a .env.local file in the root of your project. This file will securely store your Spotify API credentials.
Replace your-client-id and your-client-secret with the actual credentials you obtained from the Spotify Developer Dashboard.
Next, you'll need to create a pages/api/auth/[...nextauth].js file in your project directory. This file will configure the Spotify provider for authentication and set up the Spotify API access token when a user signs in.
Take a look at this: Figma Next Js
Make sure to add the following code to this file:
You can also use a command on your terminal to generate a hash_key, which is very important if you deploy your app.
Here are the two extra env variables you'll need to provide when deploying your app:
If you don't provide these variables, production will throw an error.
To create the authentication routes, create a file named route.ts in the src/app/api/auth/[...nextauth]/ directory.
Remember to store your Spotify credentials in the .env.local file, and don't forget to provide the extra env variables when deploying your app.
Creating API Functions
Creating API functions is a crucial step in integrating Spotify authentication into your Next.js app. To do this, you'll need to create a new file called `spotify.js` inside the `lib` directory.
This file will contain the functions that interact with the Spotify API using the `spotify-web-api-node` package. You'll set up your Spotify API client with the credentials from your environment variables.
You'll need to obtain API credentials from Spotify by creating an app in the Spotify Developer Dashboard, which requires details about your app such as its name and description.
Creating API Functions
Creating API functions is a crucial step in integrating with external services like Spotify. You'll need to create a new file to hold your API functions, which in this case is called `spotify.js`.
To set up your Spotify API client, you'll need to add some code to this file. This code will use the `spotify-web-api-node` package and your environment variables to authenticate with the Spotify API.
To interact with the Spotify API, you'll need to obtain API credentials from Spotify. This involves creating an app in the Spotify Developer Dashboard and providing details about your app.
Here are the steps to obtain API credentials:
- Create an app in the Spotify Developer Dashboard.
- Provide details about your app, such as its name and description.
- Obtain the necessary credentials to interact with the Spotify API.
Once you have your API credentials, you can create functions to interact with the Spotify API. This will involve using the `spotify-web-api-node` package and your credentials to make API calls.
As you create your API functions, you'll also need to set up routes to handle API requests. One example of this is creating a route for `/get-top-tracks`.
Fetching Saved Tracks
Fetching Saved Tracks is a crucial step in creating a seamless music player experience.
To fetch a user's saved tracks, you need to interact with the Spotify API.
You can do this by adding a function to your spotify.js file, as shown in Example 1.
This function will fetch the user's saved tracks when they are signed in.
The player.js file then needs to be modified to fetch and display the user's saved tracks.
This can be achieved by using the function added to the spotify.js file.
The code for this is straightforward and can be easily implemented.
Check this out: Next Js Fetch Data
Response
After you've successfully authenticated a user with Spotify, you'll receive a response that will guide you through the next steps. The response will contain two query parameters: code and state.
The code parameter is an authorization code that can be exchanged for an access token. This is what you'll use to authenticate the user in your application. For example, the code might look something like this: "If the user accepts your request, then the user is redirected back to the application using the redirect_uri passed on the authorized request described above."
The state parameter is used to prevent cross-site request forgery (CSRF) attacks. It's a value that you supplied in the request to Spotify, and you should compare it with the value received in the response. If there's a mismatch, you should reject the request and stop the authentication flow.
Here's a summary of the query parameters you can expect to receive in the response:
If the user doesn't accept your request or an error has occurred, the response will contain different parameters. You should check for the error parameter, which will indicate the reason authorization failed. For example, the error might be "access_denied".
The request must include the following HTTP headers, but this is not explicitly mentioned in the article section facts.
User Authentication
User Authentication is a crucial part of the Spotify authentication process in Next.js. To set up user authentication, you'll need to create a pages/api/auth/[...nextauth].js file and add the necessary code to configure the Spotify provider.
You can either use the next-auth package to handle user authentication or create your own authentication routes. To create authentication routes, you'll need to create a file named route.ts in the src/app/api/auth/[...nextauth]/ directory.
To request an access token, you'll need to send a POST request to the /api/token endpoint with the authorization code and client ID and secret key. The response will contain an access token, token type, scope, expires in, and refresh token.
Here's a summary of the required parameters for the POST request:
By following these steps, you'll be able to set up user authentication for your Spotify authentication flow in Next.js.
Userinfo Option
The userinfo option is a convenient feature in next-auth that allows you to retrieve information about the logged-in user.
You can configure the userinfo option in three ways: by providing a full URL, an object with a URL and parameters, or by taking complete control of the request.
Here are the three ways to configure the userinfo option:
- You can set userinfo to be a full URL, like "https://example.com/oauth/userinfo?some=param".
- Use an object with url and params like userinfo: {url: "https://example.com/oauth/userinfo", params: {some: "param"}}.
- Completely take control of the request: userinfo: {url: "https://example.com/oauth/userinfo", async request(context){ return await makeUserinfoRequest(context) }}.
If your provider does not have a userinfo endpoint, you can create a noop function.
User Authentication
User Authentication is a crucial step in building a music streaming app that integrates with the Spotify API. You'll need to use a library like next-auth to handle user authentication.
To get started, create a file called `[...nextauth].js` in your project directory and add the following code to configure the Spotify provider for authentication. This code sets up the Spotify API access token when a user signs in.
When setting up authentication, you'll need to configure the Spotify Provider with NextAuth technology. This involves utilizing the Spotify API credentials stored in your `.env.local` file.
Here are the steps to create authentication routes:
1. Inside your project directory, create a path `src/app/api/auth/[...nextauth]/`.
2. Create a file named `route.ts` in this directory.
If you want to retrieve information about the logged-in user, you can use the userinfo endpoint. This endpoint returns information about the user, but it's not part of the OAuth specification. You can either set userinfo to a full URL or use an object with a url and params.
Related reading: Nextjs Middleware Firebase Auth
Here are the different ways to configure the userinfo endpoint:
In the rare case you don't care about what this endpoint returns, or your provider doesn't have one, you can create a noop function. If your provider is OpenID Connect (OIDC) compliant, we recommend using the wellKnown option instead.
Sources
- https://next-auth.js.org/configuration/providers/oauth
- https://clouddevs.com/next/music-streaming-app-with-spotify-api/
- https://dev.to/matdweb/how-to-authenticate-a-spotify-user-in-nextjs-14-using-nextauth-5f6i
- https://www.tarungowda.com/blog/how-to-integrate-spotify-api-with-next-js
- https://developer.spotify.com/documentation/web-api/tutorials/code-flow
Featured Images: pexels.com