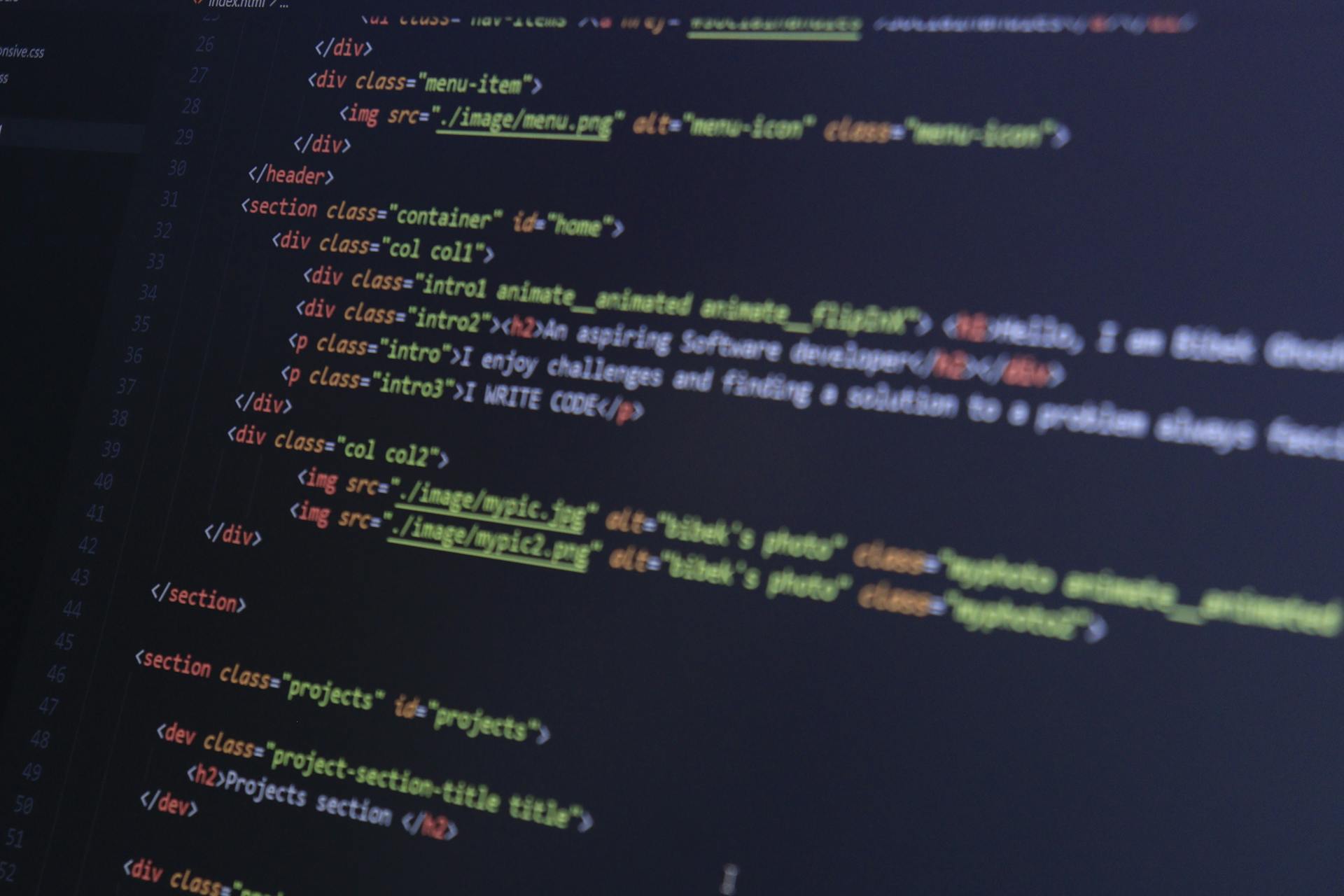
Next.js and MySQL integration is a powerful combination for building fast, scalable, and secure web applications.
With Next.js, you can create server-side rendered (SSR) pages, which improves SEO and user experience.
To integrate Next.js with MySQL, you need to install the mysql2 package using npm or yarn. This package allows you to connect to your MySQL database from your Next.js application.
In a real-world example, we can use the mysql2 package to connect to a MySQL database and fetch data for our Next.js application. This can be achieved by creating a separate file for database connection and importing it in our pages.
See what others are reading: Nextjs Pages
Database Setup
To set up the database in a Next.js API, you'll be using MySQL and Sequelize ORM. MySQL is the database used for storing user data, and Sequelize ORM connects, queries, and manages data in the MySQL database.
Sequelize also supports model synchronization, which automatically generates database tables and columns based on models defined in code. This makes it easier to work with the database.
A fresh viewpoint: Next Js Fetch Data save in Context and Next Route
The MySQL database is initialized on the first API request by calling db.initialize();, which sets the db.initialized property to true after initialization. This ensures that the database is properly set up before any data is accessed.
In development, db.initialize() may be triggered multiple times due to hot reloading, but this does not occur in production. This is because hot reloading is enabled when you start the app with npm run dev, but not when you build the app with npm run build then start it with npm start.
The initialize() function creates the MySQL database if it doesn't exist, initializes db models, and adds them to the exported db object. It is called from the API handler when the first request is sent to the Next.js API.
Additional reading: Nextjs Api Call Another Api
Data Access
The MySQL data context is used to connect to MySQL using Sequelize and exports an object containing all of the database model objects in the application.
It provides an easy way to access any part of the database from a single point, making it a convenient solution for managing data access.
The data context class is used to connect to MySQL with Sequelize and exports an object containing all of the database model objects in the application.
It is used to create a MySQL database if it doesn't exist, initializes db models and adds them to the exported db object.
The initialize() function is called from the API handler when the first request is sent to the Next.js API.
You can access any part of the database from a single point using the data context class.
Here's a breakdown of the steps to connect to MySQL in Next.js with Sequelize and MySQL2:
- Populate the connection parameters object using the GetDBSettings() function.
- Connect to database using mysql.createConnection() method.
- Create the SQL query to fetch data.
- Execute the query and retrieve the results using mysql.execute() method.
- Close the connection when done using mysql.end() method.
You can also use mysql2.query() the same way to run a SQL query.
For another approach, see: Can I Use React Query with Nextjs
The Sequelize User Model is used to define the schema for the users table in the MySQL database.
The exported Sequelize model object gives full access to perform CRUD (create, read, update, delete) operations on users in MySQL.
The defaultScope configures the model to exclude the password hash from query results by default.
The withHash scope can be used to query users and include the password hash in results.
Here's a summary of the available scopes for the Sequelize User Model:
Data Retrieval
Data Retrieval is a crucial step in building a Next.js app that interacts with a MySQL database.
To get data from the database, you'll need to import the db function from the database file.
Inside a Next.js page like pages/index.tsx, you can create a getServerSideProps() function to fetch data server-side. Next.js will use this function to get data from the database and pass it as props to the page.
This function assumes you have a table named Message with columns for id and text.
For more insights, see: Nextjs save Data Pulled from Api Using State Context
API and Integration
In a Next.js application, the API plays a crucial role in handling requests and interacting with the database. The Next.js API contains routes/endpoints, including the users index handler, which receives HTTP GET requests and returns all users from MySQL by calling the usersRepo.getAll() function.
The users index handler is secured by the global JWT middleware, ensuring that only authorized requests are processed. This is a best practice for protecting sensitive data and preventing unauthorized access.
Next.js blurs the lines between client and server, supporting pre-rendering pages at build time or request time. This flexibility makes it an ideal choice for working with a database in a Next.js app, where Prisma can be used to access the database at build time, request time, or by separating the backend into a standalone server.
Users API Handler
The users API handler is a crucial part of the Next.js application, responsible for managing user data stored in MySQL.
It receives HTTP GET requests sent to the base users route /api/users and passes an object with a get() method to the apiHandler() which is mapped to the getAll() function.
The getAll() function returns all users from MySQL by calling usersRepo.getAll().
Security for this route is handled by the global JWT middleware.
The users repo encapsulates all access to user data stored in MySQL and exposes methods for authentication and standard CRUD operations for reading and managing user data.
On successful authentication, a JWT (JSON Web Token) is generated with the jsonwebtoken npm package, digitally signed using the secret key stored in next.config.js, and returned to the client application.
Worth a look: Next Js Jwt
Prisma and Next Integration
Next.js blurs the lines between client and server, making it a great fit for Prisma. Prisma is the perfect companion for working with a database in a Next.js app.
You can access your database with Prisma at build time using getStaticProps. This allows for pre-rendering pages at build time.
Prisma Accelerate is a great tool for deploying your app to a Serverless or Edge environment. It speeds up database queries with a scalable connection pool.
This connection pool ensures your database doesn't run out of connections, even during traffic spikes.
Related reading: Next Js Build
Code and Configuration
To set up a Next.js app with MySQL, you'll need to install the necessary packages, including `mysql2` and `express`. These packages will help you connect to your MySQL database and create a RESTful API.
The `mysql2` package is a popular MySQL driver for Node.js, and it's the one we'll be using in our example. To install it, simply run `npm install mysql2` or `yarn add mysql2` in your terminal.
With the packages installed, you can then create a database connection using the `mysql2` package, as we did in our example. This involves creating a new instance of the `Pool` class, passing in your database credentials, and then using the `query` method to execute SQL queries against your database.
Install Dependencies
To get started, you'll need to install the necessary dependencies for this project. The solution uses the knex and mysql2 packages, so make sure to include them in your dependencies.
These packages are the foundation of the project, and they'll help you connect to your database and perform various operations. You can install them using npm or yarn, whichever package manager you prefer.
You might like: Nextjs Install
The knex package is a query builder that allows you to write SQL queries in a more readable and maintainable way. It's a great tool for simplifying your database interactions and reducing errors.
mysql2 is a MySQL driver that enables you to connect to your MySQL database. It's a reliable and efficient way to interact with your database, and it's widely used in the industry.
By installing these packages, you'll be able to move on to the next step and start configuring your project. Don't forget to include them in your project's dependencies to ensure everything runs smoothly.
Code Documentation
Code documentation is crucial for any project's success, and Next.js provides a clear structure for organizing code.
In the provided example, the code is organized into five main folders: components, helpers, pages, services, and styles. This structure helps keep related code together and makes it easier to find what you need.
The components folder contains React components used by pages or other React components, with global components in the root /components folder and feature-specific components in subfolders.
Here's an interesting read: Next Js Components
The helpers folder is for anything that doesn't fit into the other folders and doesn't warrant its own folder, with front-end React helpers in the root /helpers folder and back-end API helpers in the /helpers/api subfolder.
Next.js pages and API route handlers are in the pages folder, with each page's route defined by its file name, and API route handlers in the /pages/api folder.
API route handlers can be found in the /pages/api folder, and more information on Next.js Page Routing and file name patterns can be found at https://nextjs.org/docs/routing/introduction.
The services folder handles all HTTP communication from the React front-end app to the Next.js back-end API, with each service encapsulating the API calls for a content type (e.g. users) and exposing methods for performing various operations (e.g. CRUD operations).
Here's a breakdown of the main folders and their contents:
Environment Variables
You can define environment variables in Next.js by adding a property called `env` to the `nextConfig` object in the `next.config.js` file.
This allows you to have two sets of parameters, one for development and one for production.
To retrieve these variables, you can create a function, like `GetDBSettings`, that uses the `process.env` object to get the parameters based on the environment.
Create a folder under `src` called `sharedCode` to put common codes and functions, and inside it, create a file called `common.ts` to store the function.
You can use TypeScript's `Non-null assertion operator`, the `!`, to tell the compiler that variables won't be null, avoiding errors like "Type 'undefined' is not assignable to type 'string'".
This operator is particularly useful when accessing environment variables, as they might be undefined in certain situations.
By defining environment variables in the `next.config.js` file, you can keep your code organized and make it easier to switch between different environments.
Frequently Asked Questions
What is the best database for next JS?
For Next.js applications, a PostgreSQL-based database like Supabase is a popular choice due to its scalability and ease of integration, while MongoDB's flexibility also makes it a suitable option for complex data models.
Is NextJS good for backend?
Yes, NextJS offers robust server-side capabilities, making it a suitable choice for building backend logic. Its versatility allows for seamless integration of frontend and backend development.
Sources
- https://jasonwatmore.com/next-js-13-mysql-user-registration-and-login-tutorial-with-example-app
- https://stackademic.com/blog/next-js-api-connect-to-mysql-database
- https://jasonwatmore.com/next-js-13-mysql-connect-to-mysql-database-with-sequelize-in-nextjs
- https://travishorn.com/integrating-sql-data-into-your-nextjs-app
- https://www.prisma.io/nextjs
Featured Images: pexels.com