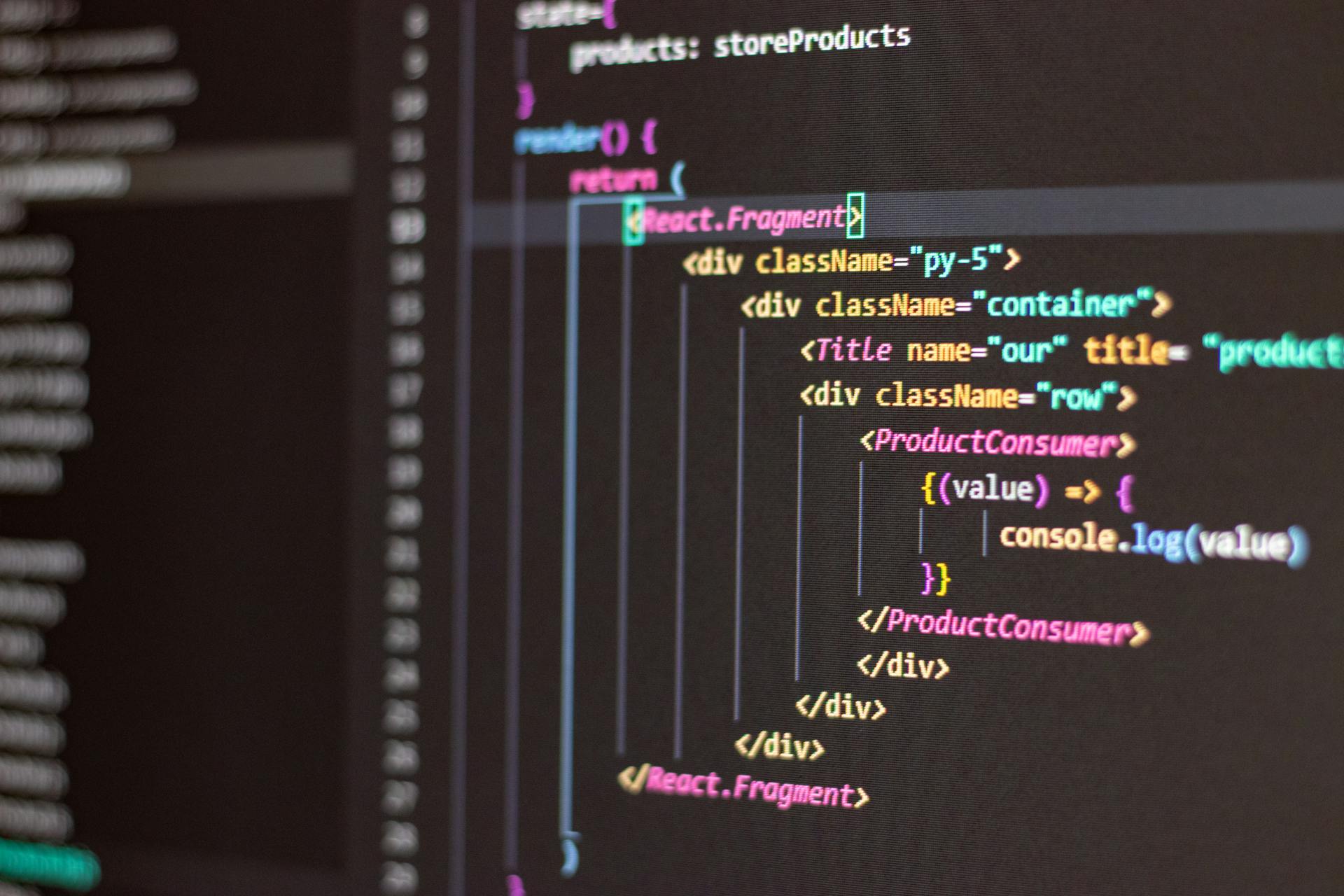
Optimizing the Next.js build process is crucial for achieving optimal performance and speed. This can be done by using the `next build` command with the `--target` flag, which allows you to specify the target browser versions to optimize for.
By default, Next.js uses the `serverless` target, which is ideal for serverless environments. However, if you're building a production application, you may want to use the `static` target to generate static HTML files.
Using the `next build` command with the `--target` flag can significantly improve build times and reduce the size of your application. This is because it allows you to take advantage of browser-specific optimizations, such as tree shaking and code splitting.
On a similar theme: Nextjs Spa
Project Setup
Before you start building, make sure your project folder is organized. This includes having all necessary files in place.
Your 'pages' folder should contain all your app's page components, which Next.js uses to create the app router.
Next.js needs a clear structure to function properly, so take the time to set up your project correctly.
Explore further: What Is .next Folder in Next Js
Development Process
The development process is where the magic happens. You'll start by setting up your development server, which is where you'll write code, test features, and preview your app in a browser.
Hot reloading is a game-changer, allowing any changes you make to your code to automatically update in the browser, streamlining your dev process.
On a similar theme: Next Js Development Company
Step-by-Step: The Process
To start the development process, you'll need to set up your development server. This is where you'll write code, test features, and preview your app in a browser.
The development server offers hot reloading, which means any changes you make to your code will automatically update in the browser, streamlining your dev process. This is a game-changer for developers, saving time and effort in the long run.
The command to start the development server is straightforward, typically on port 3000, allowing you to access your app at http://localhost:3000.
Discover more: How to Start Next Js Project
Custom Babel Config Setup
Custom Babel Config Setup is a crucial step in tailoring your Next.js app to your needs. Next.js provides a convention-over-configuration approach, but you can customize Babel beyond the defaults by creating a .babelrc file or babel.config.js in your project root. This file is automatically used by Next.js to apply your custom Babel presets and plugins.
To set up a custom Babel config, you'll want to create a .babelrc file or babel.config.js in your project root. Next.js will automatically use this file to apply your custom Babel presets and plugins. This allows you to fine-tune your Babel configuration to suit your specific project requirements.
Related reading: Apply Css Nextjs
Static Generation and Server-Side Rendering
In Next.js, pages are pre-rendered to static HTML wherever possible. This means that for most pages, the content is generated ahead of time and served directly to users.
Next.js generates the necessary server code to render HTML on the fly when a request is made for pages that require server-side rendering.
Readers also liked: App Routing vs Page Routing Nextjs
Code Splitting
Code Splitting is a crucial step in optimizing load times. It's a technique used by Next.js to split your JS code into smaller chunks.
This ensures that the browser only loads the code necessary for the current view, which can significantly improve performance. By doing so, you can reduce the amount of code that needs to be loaded upfront, making your application feel snappier and more responsive.
For another approach, see: Nextjs Code Block
App Configuration
Next.js provides a convention-over-configuration approach, but you can still customize the build process to suit your needs. This is done through its next.config.js file.
You can extend Next.js's webpack configuration by providing a function in next.config.js that modifies the existing config. This allows you to add custom webpack plugins.
Next.js also gives you the flexibility to define environment variables in next.config.js using the env key, making them available in your app at build time.
If this caught your attention, see: Next Config Js
Ecommerce with Shopify
Building an ecommerce site with Shopify is a popular choice among developers, and for good reason. Next.js ecommerce with Shopify is a powerful combination that allows for seamless integration and customization.
Shopify provides a robust ecommerce platform that can be easily integrated with Next.js, a popular React-based framework for building server-rendered applications. This combination enables developers to create high-performance ecommerce sites with ease.
To get started, you can follow tutorials like "Next.js ecommerce with Shopify" that walk you through the process of building an ecommerce site from scratch. These tutorials often include step-by-step instructions and code examples to help you learn as you go.
Consider reading: Next Js 14 Ecommerce
Configuration and Customization
Next.js provides a convention-over-configuration approach, but it also offers ample room for customization through its next.config.js file.
You can extend Next.js's webpack configuration by providing a function in next.config.js that modifies the existing config, allowing you to add custom webpack plugins.
Customizing Babel beyond Next.js defaults is possible by creating a .babelrc file or babel.config.js in your project root, which Next.js will automatically use to apply your custom Babel presets and plugins.
To define environment variables, use the env key in next.config.js, and these variables will be available in your app at build time.
Intriguing read: File Upload Next Js Supabase
Environment Variables and Runtime Config
You can define environment variables in next.config.js using the env key. These variables will be available in your app at build time.
Environment variables can be used for runtime configuration, which means they can be set at runtime rather than at build time. You can add a publicRuntimeConfig or serverRuntimeConfig object in next.config.js to achieve this.
Variables prefixed with NEXT_PUBLIC_ are exposed to the browser. This is useful for variables that need to be accessible to the client-side of your app.
Environment variables can be set using .env files or your hosting provider's dashboard. This is especially important during deployment, when you may need to set environment variables to configure your app for the production domain.
Remember that server-side variables need to be loaded correctly in your next.config.js or through your hosting provider.
For another approach, see: Next Js Build Time Slow
Why Do We Need Pre-Scripts?
Pre-scripts are a game-changer when it comes to handling complex tasks in app configuration. They simplify implementation and make it easier to handle tasks that would be otherwise tricky.
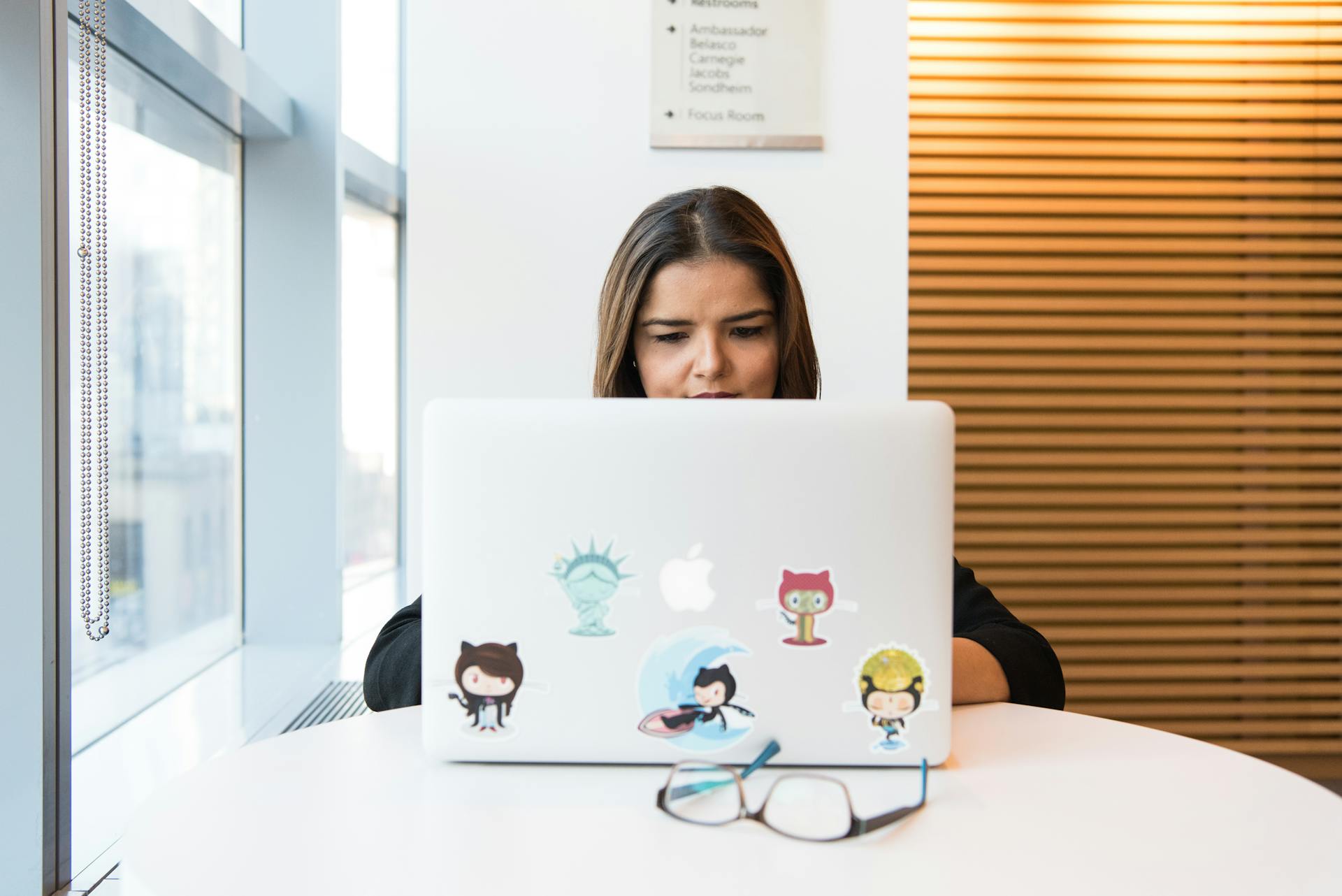
One of the most common use cases for pre-scripts is handling old URL slugs. This involves providing the old and new URLs before running a build, which can be a pain to manage manually.
We've found that pre-scripts are particularly useful when dealing with site-wide content. This includes basic product info, logos, links, and other elements that are used across the entire project.
Pre-scripts can be used to generate a code file that's available globally in the project, making it easy to access and update this content.
Here are some common use cases for pre-scripts:
- Handling old URL slugs
- Site-wide content
Upgrade to V5.x
Upgrading to V5.x is a straightforward process, and you can rely on Netlify to automatically update the adapter for you. Unless you've manually installed a specific version of the adapter, you don't need to worry about updating it.
You can simply remove the package from your package.json and netlify.toml files, and Netlify will take care of the rest. This way, you'll always have the latest features and fixes without any extra work on your part.
However, if your site is pinned to an earlier runtime version, you'll need to upgrade Next.js to 13.5 or later, and Node.js to 18 or later. This will ensure a smooth transition to V5.x.
To upgrade from v4.x to v5.x, follow these steps:
- Upgrade Next.js to 13.5 or later, and Node.js to 18 or later.
- Address the breaking changes noted below.
- Change the package name in your package.json and netlify.toml from @netlify/plugin-nextjs to @opennextjs/netlify, and ensure the version is at least v5.9.
Pin a Specific Adapter Version
If you need to return to a specific version of the Next.js adapter or runtime, you can pin that version to your site.
We recommend not pinning the adapter version, as the v5 adapter is actively maintained to support all Next.js versions starting from 13.5, and Netlify will automatically update the adapter to the latest version on each site build for you.
To pin a specific adapter version, you need to install the desired version of the @netlify/plugin-nextjs package.
You can do this by running the command `npm i @netlify/[email protected]` in your terminal.
Once you've installed the package, you need to add the package version to your netlify.toml file.
This involves adding the following code to your netlify.toml file:
```html
[[plugins]]
package = "@netlify/plugin-nextjs"
```
This will pin the adapter version to the specified version, allowing you to maintain control over the version used in your site.
Recommended read: Next Js Static Site
Featured Images: pexels.com