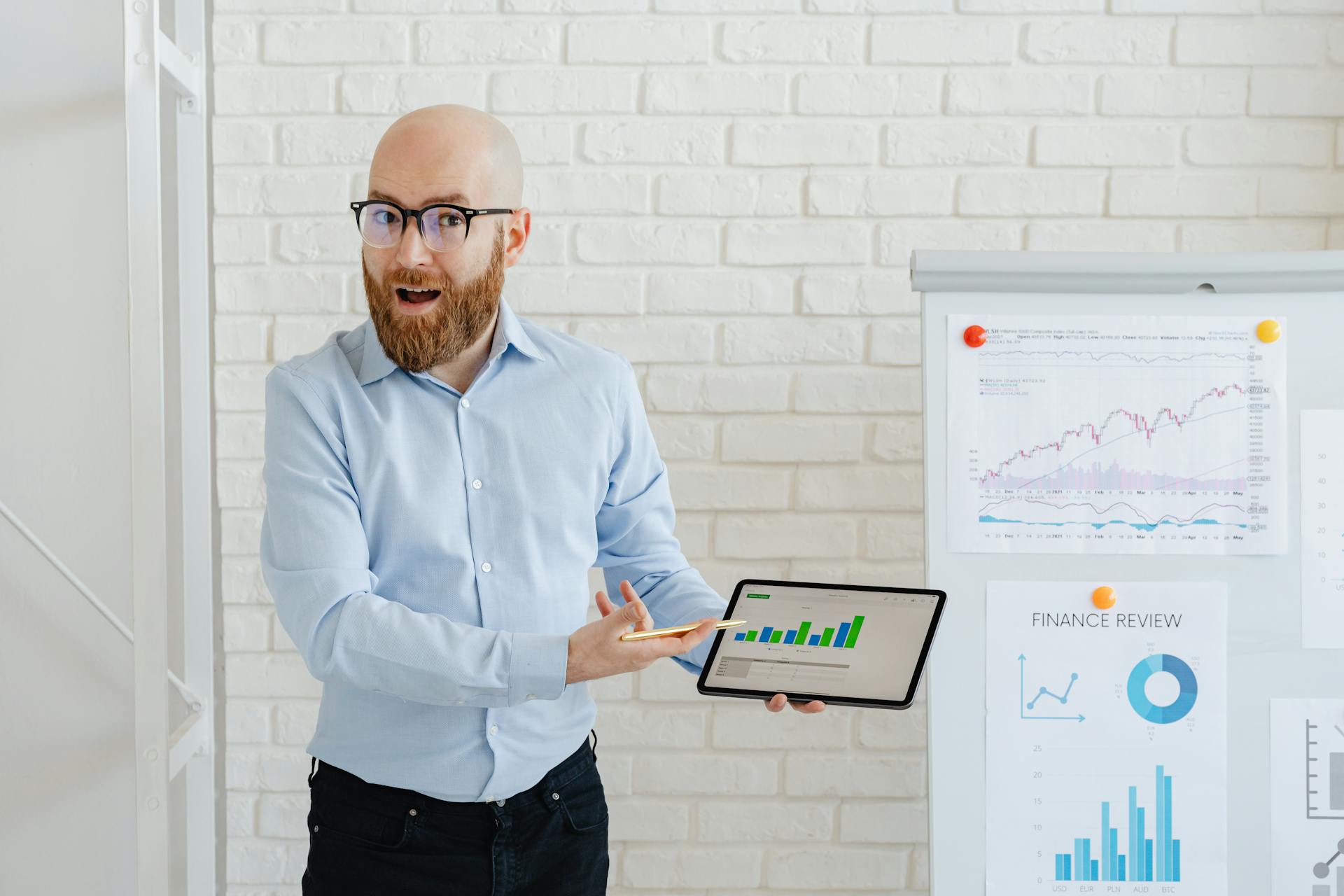
Using React Query with Next.js can simplify data management by providing a centralized way to handle data fetching, caching, and updating.
React Query can be used with Next.js to handle API calls and caching, making it easier to manage data across different pages and components.
One of the key benefits of using React Query with Next.js is that it automatically caches API responses, reducing the number of requests made to the server.
This can lead to a significant performance boost, especially for applications with complex data requirements.
Curious to learn more? Check out: Next Js Mysql
Getting Started
React Query is a powerful library that can be used with Next.js to manage data fetching and caching. It's a great tool to have in your toolbox, but before you can start using it, you need to set up your project.
First, you'll need to install React Query using npm or yarn. This can be done by running the command `npm install react-query` or `yarn add react-query` in your terminal.
Next, you'll need to import the QueryClient and QueryClientProvider components from the react-query library. This will allow you to wrap your app with the QueryClientProvider, which will enable React Query to manage your data fetching and caching.
Recommended read: Figma to Next Js
Setting Up React Query
To set up React Query, you'll need to import QueryClient and QueryClientProvider from react-query and create a client. This client is then provided to your application.
You can use ReactQueryDevtools in development to help with debugging.
In a typical setup, you'll create a separate hook file for maintaining the React Query integration. This hook file is where you'll use the UseQuery hook for GET methods and the UseMutation hook for POST, PUT, and DELETE methods.
Here's a quick rundown of what you'll typically see in your hook file:
In the hook file, "posts" is a query key that must be unique to the query's data. This query key is used to manage query caching by React Query.
When using the hook, you'll need to pass the ID dynamically for GetById and DeleteById methods, and the data dynamically for POST and PUT methods from where the hook is used.
Discover more: Nextjs save Data Pulled from Api Using State Context
Creating Queries
Creating queries is a key part of building a robust application with React Query and Next.js.
To write reusable queries, define them in a central place, such as a queries folder, so you can use them across both your server and client components. This makes your code more organized and easier to maintain.
You can create a simple query function that takes in either the browser or server Supabase client and the id of a country, and returns a supabase-js query.
For React Query setup in your application, import QueryClient and QueryClientProvider from react-query, create a client, and provide the client to your application. This is a crucial step in getting React Query up and running with Next.js.
In development, you can use ReactQueryDevtools to help with debugging and optimization.
You might enjoy: Use Theme in Server Components Nextjs
Database and Client Setup
To set up React Query with Next.js, you'll need to install the required packages, including `@tanstack/query` and `@tanstack/react-query`.
First, create a new Next.js project using the `npx create-next-app` command. Next, install the required packages by running `npm install @tanstack/query @tanstack/react-query` in your terminal.
A fresh viewpoint: Next Js Send Email
With the packages installed, you can now configure React Query by creating a new instance of the QueryClient in your Next.js project. This can be done by adding the following code to your pages/_app.js file: `import { QueryClient, QueryClientProvider } from '@tanstack/react-query';`.
You can also use the `useQuery` hook from React Query to fetch data in your components. This hook allows you to specify the query function, the initial data, and the options for the query.
Fetching Data
You can fetch data server-side with React Query, which means the data will be immediately available when rendered in a client component. This is done using the prefetchQuery method in server components.
To write reusable queries, define them in a central place, such as a queries folder, where you can easily access and reuse them across both server and client components. This approach makes your code more organized and efficient.
In a Next.js application, you need to set up React Query by importing QueryClient and QueryClientProvider from react-query, creating a client, and providing the client to your application.
Consider reading: Nextjs Can Layouts Be Server Components
CRUD Operations
To perform CRUD (Create, Read, Update, Delete) operations with React Query in a Next.js application, you'll need to use hooks. For POST and PUT methods, the mutate function is used, and data is passed dynamically to the hook.
When implementing the DELETE function, you'll need to pass the Id of the data to be deleted dynamically to the hook.
In a separate hook file, you'll use the UseQuery function for GET methods and UseMutation for POST, PUT, and DELETE methods. The "posts" query key is used in the example hook file code and must be unique to the query's data.
For GetById and DeleteById methods, you'll need to pass the Id as dynamically, while for POST and PUT methods, you'll pass the data as dynamically from where the hook has been used.
Consider reading: Can Nextjs Be Used on Traditional Web Application
Frequently Asked Questions
Can you use React and Next.js together?
Yes, you can use React and Next.js together to build dynamic web applications. This powerful combination enables interactive and high-performance web development.
How to use React Query with Next 14?
To use React Query with Next 14, create a Next.js project and set up a React Query Client Provider to manage data fetching and caching. This involves wrapping the provider around your root children and implementing prefetching strategies for optimal performance.
Sources
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://brockherion.dev/blog/posts/setting-up-and-using-react-query-in-nextjs/
- https://prateeksurana.me/blog/mastering-data-fetching-with-react-query-and-next-js/
- https://supabase.com/blog/react-query-nextjs-app-router-cache-helpers
- https://medium.com/@aalam-info-solutions-llp/react-query-integration-from-the-scratch-in-next-js-and-react-js-91d585a0a65e
Featured Images: pexels.com