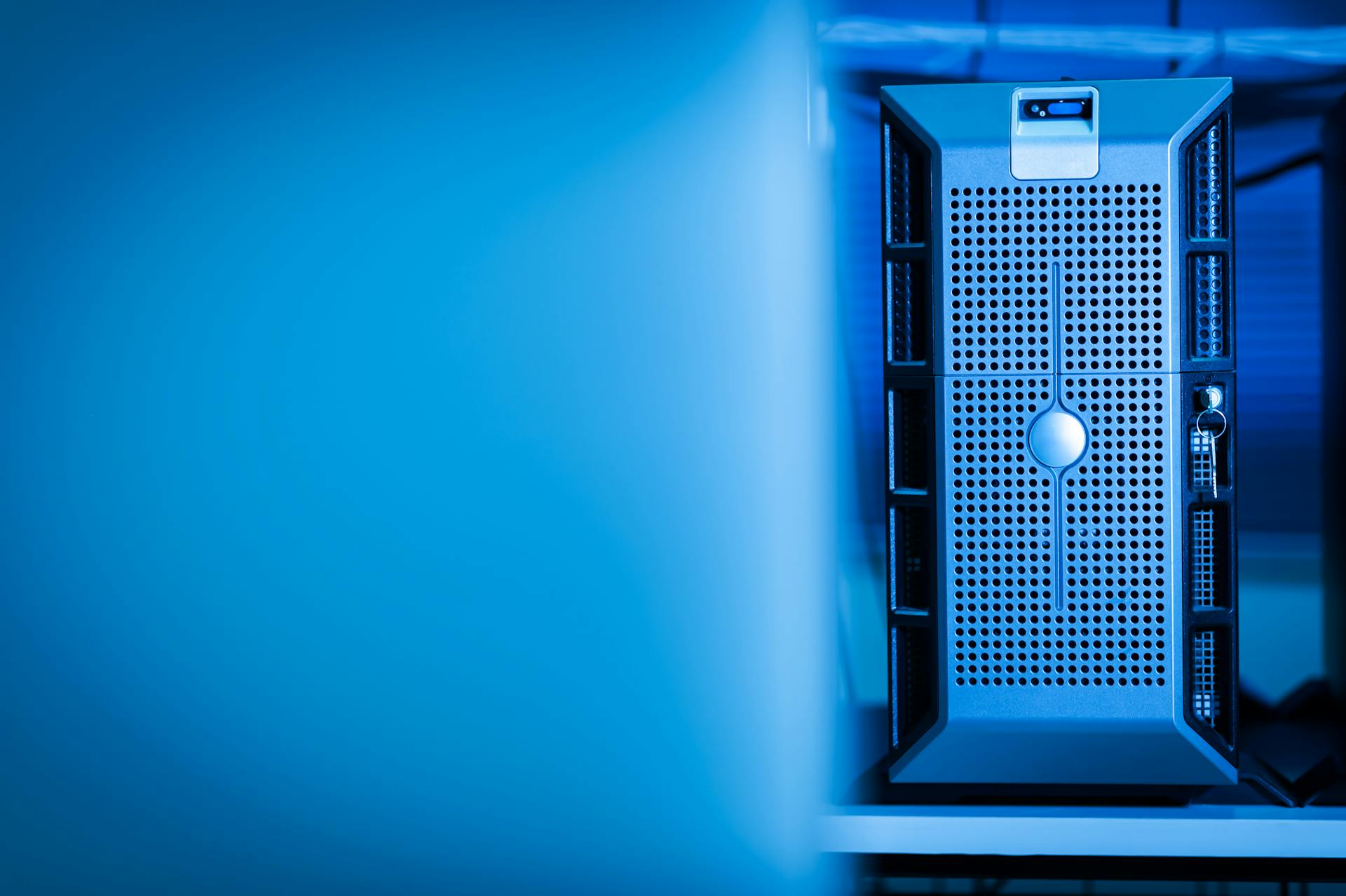
Server components in Next.js are a powerful tool for building scalable and performant applications. They allow you to render components on the server, reducing the amount of data sent to the client and improving page load times.
One of the key benefits of server components is that they can be reused across different pages, making it easier to manage layout and structure.
Layouts in Next.js can be server components, and this can be achieved by using the `use client` directive. This directive tells Next.js to render the component on the client-side, but only if the component is being used as a layout.
By using layouts as server components, you can take advantage of server-side rendering and improve the performance of your application.
Recommended read: Nextjs Nested Layout
Definition
Next.js allows developers to create reusable layouts using server components, which can greatly improve the performance and scalability of their applications.
Server components in Next.js are a new type of component that can be rendered on the server, rather than in the browser.
Discover more: Next Js Server Components
This means that server components can handle complex logic and rendering, freeing up the browser to handle other tasks.
Server components can also be reused across multiple pages, making it easier to maintain a consistent design and user experience.
Server components can be used to create reusable layouts that can be easily shared across multiple pages, reducing code duplication and improving maintainability.
Server components can also improve performance by reducing the amount of data that needs to be transferred between the server and the browser.
For another approach, see: Use Theme in Server Components Nextjs
Using Server Components in Next.js
Server components in Next.js offer a new way to build reusable UI components, and can be used in layouts. This approach allows for more efficient code splitting and faster page loads.
Server components can be used in layouts to render dynamic content on the server, reducing the amount of data transferred to the client.
If this caught your attention, see: Nextjs Frontend Components
Enabling Server Components
To enable server components in Next.js, you need to create a new file in the pages directory with a .js extension. This file will contain the server component code.
Suggestion: Can Amazon S3 Take in Nextjs File
Server components are enabled by default in Next.js 13, so you don't need to do anything extra in most cases. However, if you're using an older version of Next.js, you'll need to update to the latest version.
The server component file should export a React component as a function, like this: `export default function MyServerComponent() { ... }`. This is the basic structure of a server component.
Server components are a powerful tool for building fast and scalable applications. By offloading some of the rendering work to the server, you can improve the performance of your application and reduce the load on the client-side.
Check this out: Nextjs Pathname Server Component
Configuring Server Components
To configure server components in Next.js, you'll need to create a separate API route for each component. This route will handle incoming requests and return the component's markup as HTML.
Server components can be used to handle API routes in Next.js, and you can create them by using the `pages/api` directory. This directory is automatically mapped to the `/api` route.
A unique perspective: Api in App Router Next Js
In Next.js, server components can be used to handle GET requests, POST requests, and more. For example, you can create a server component to handle a GET request for a specific API endpoint.
To enable server components in Next.js, you'll need to add the `experimental-server-components` option to your `next.config.js` file. This option is required for server components to work.
Server components can be used to handle authentication and authorization in Next.js. For example, you can create a server component to check if a user is logged in before rendering a page.
Recommended read: Can Nextjs Be Used on Traditional Web Application
Layouts with Server Components
In Next.js, layouts can be server components, but there are some limitations.
Server components can't be used as layouts in a traditional sense, meaning they can't be shared across multiple pages. This is because server components are typically used for rendering dynamic content, not for reusing layout code.
However, Next.js does allow you to use server components as a layout component, but it requires using the `useEffect` hook to handle the component lifecycle.
Curious to learn more? Check out: Fluid Layout
Benefits
Layouts with Server Components offer improved performance by reducing the number of round trips between the client and server.
Server components can be reused across multiple layouts, reducing code duplication and improving maintainability.
With layouts, you can define a template for a component, making it easier to create consistent UI elements.
Server components can handle complex business logic, freeing up the client-side code to focus on rendering and user interaction.
Layouts enable you to keep your application's UI and business logic separate, making it easier to scale and maintain your application.
Readers also liked: Nextjs Ui
Creating Layouts
Creating layouts with server components is all about defining the structure and organization of your application.
Server components can be used to render layouts, which means you can define the layout in your server component and then pass it down to the client.
In the example of the Counter component, the layout is defined in the ServerComponent function, which returns a JSX element that represents the layout.
Check this out: Nextjs Redirect from Server Component
To create a layout, you need to define a function that returns a JSX element. This function can be a server component, which can be used to render the layout on the server.
The ServerComponent function in the Counter example returns a JSX element that represents the layout, which includes a header, a counter, and a button.
The layout is then passed down to the client as a prop, where it can be rendered by the client-side components.
You can also use the useLayoutEffect hook to update the layout when the user interacts with the application.
Explore further: Nextjs Layout
Customizing Layouts
Customizing Layouts is a key aspect of working with Server Components. You can use the `useLayoutEffect` hook to update the layout of your application when the server component's state changes.
To customize the layout of a server component, you need to create a new layout component. This is done by using the `createLayout` function from the `@remix-run/react` package. For example, you can create a new layout component that includes a navigation bar and a footer.
Server components can be used to render different layouts based on the user's preferences or device type. By using the `useServerComponent` hook, you can render a different layout for each device type. For instance, you can render a mobile layout on smaller screens and a desktop layout on larger screens.
The `useLayoutEffect` hook is used to update the layout of the application when the server component's state changes. This hook is similar to the `useEffect` hook, but it's specifically designed for updating the layout of the application.
Best Practices and Considerations
When working with Next.js server components, it's essential to follow best practices to ensure a seamless experience.
Server components can be used for layouts, but it's crucial to consider the impact on performance.
To optimize performance, use the `useEffect` hook to fetch data only when the component is mounted. This approach can be seen in the example where the `useEffect` hook is used to fetch data when the `Home` page is loaded.
Server components can also help reduce the initial load time by pre-rendering the layout.
You might like: Next Js Fetch Data
Performance Optimization
Performance optimization is crucial for achieving the best results from your software.
Regularly monitoring system performance can help you identify bottlenecks and areas for improvement.
A slow database query can significantly impact the overall performance of your application, taking up to 70% of the total execution time.
Use caching to store frequently accessed data, reducing the need for repeated database queries and improving performance by up to 30%.
Code optimization techniques such as minimizing loops and using efficient algorithms can also greatly improve performance.
For example, replacing a linear search with a binary search can reduce the time complexity of an algorithm from O(n) to O(log n).
Avoid unnecessary computations by using lazy loading, which can improve performance by delaying the loading of large datasets until they are actually needed.
Optimizing database queries and indexing data can also significantly improve performance, reducing query execution times by up to 90%.
A unique perspective: Query in Nextjs
Security and Scalability
Security and Scalability is a top priority for any business. Ensuring the integrity and availability of your application is crucial.
Regularly updating dependencies and plugins can help prevent vulnerabilities, as seen in the "Dependency Management" section. This can be done using tools like npm audit and pip audit.
Scalability is also key, and load balancing can help distribute traffic across multiple servers, preventing any single point of failure. This is especially important for high-traffic applications.
Proper configuration of servers and databases can also help improve scalability, as shown in the "Server Configuration" section. This includes setting optimal values for parameters like max_connections and max_memory.
Monitoring and logging are also essential for identifying and addressing security issues, and can be done using tools like ELK Stack and Prometheus.
You might enjoy: Can Vercel Host Nextjs Servers
Sources
- https://nextjs.org/docs/app/building-your-application/rendering/server-components
- https://nextui.org/docs/frameworks/nextjs
- https://nextjs.org/docs/app/api-reference/file-conventions/layout
- https://maxleiter.com/blog/build-a-blog-with-nextjs-13
- https://blog.greenroots.info/understanding-nextjs-server-actions-with-examples
Featured Images: pexels.com