
Nextjs frontend components are a game-changer for building fast and efficient apps. They enable server-side rendering, static site generation, and incremental static regeneration.
With Nextjs, you can create reusable UI components that can be easily shared across your app. This is made possible by the use of React components, which can be composed together to form more complex components.
By leveraging the power of Nextjs frontend components, you can significantly reduce the complexity of your codebase. This is evident in the example of the "Page" component, which can be used to render a static page without the need for a server-side API call.
The "useEffect" hook, on the other hand, is a powerful tool for managing side effects in your components. It allows you to run code when the component mounts or updates, making it easier to handle tasks such as API calls or data fetching.
Curious to learn more? Check out: Front End Web Dev
Next.js Frontend Components
Next.js offers two categories of components – React Server Components and Client Components, each playing a unique and essential role in building a Next.js application.
React Server Components are processed on the server side and transmitted to the client side, playing a critical role in performance enhancement and administrative simplification of Next.js applications.
Client Components, on the other hand, are rendered on the client side, pre-rendered on the server, and then hydrated on the client, providing an optimal balance of server-side rendering and client-side interactivity.
Reusable Next.js components can be created with ComponentProps, HTMLAttributes, functional or class components to maintain scalability and organization.
Here are some key characteristics of Next.js components:
- Next.js components are divided into React Server Components and Client Components for optimized performance and interactivity.
- Reusable Next.js components can be created with ComponentProps, HTMLAttributes, functional or class components to maintain scalability & organization.
- Layouts in NextJS can be tailored using the pages directory & UI enhanced via integration of the NextUI library.
Understanding Next.js
Next.js components are divided into two types: React Server Components and Client Components, which are optimized for performance and interactivity. This allows for efficient rendering of pages and improved user experience.
Reusable components can be created using ComponentProps, HTMLAttributes, functional or class components, making it easier to maintain scalability and organization in your application.
To create reusable components, you can use a combination of these approaches, depending on your specific needs and preferences.
Layouts in Next.js can be tailored using the pages directory, and UI can be enhanced via integration of the NextUI library. This allows for a high degree of customization and flexibility in your application's design.
Here are some key benefits of using Next.js layouts:
- Tailor your layouts using the pages directory
- Enhance your UI with the NextUI library
- Improve the overall user experience of your application
Implementing Layouts
In Next.js, layouts are used to create a uniform design throughout an application.
You can create a Layout.js file, import necessary dependencies, and define the component to create a layout component. This is done by using the export default function rootlayout in your file.
Incorporating common elements like headers and footers helps maintain a uniform aesthetic across your Next.js application.
Next.js provides the flexibility to incorporate multiple layouts, allowing you to tailor the layout for specific sections or pages within the application.
To create a layout component, you can create a file with a.js extension in the layouts directory of the project, such as DefaultLayout.js. This file can include common components like a header, footer, and navigation menu.
Additional reading: Next Js Single Page Application
The same layout component can be reused in multiple pages within a Next.js application, promoting consistency and streamlining the page designs.
You can also create nested layouts by creating a _layout.js file within a nested directory under the pages directory, allowing you to create a hierarchical structure of layouts.
Creating a directory under the pages directory for a specific route and then creating a _layout.js file within that directory can be a good approach for custom layouts tailored to specific routes.
Curious to learn more? Check out: Nextjs App vs Pages
Component Structure
Organizing components effectively is crucial for maintaining a well-organized project. This involves creating a 'components' folder for storing all reusable components and subfolders to sort components according to their functionality or purpose.
A well-organized 'components' folder is like a well-run library, where books are arranged by genre or author, making it easier to locate the exact component you need in your codebase. This practice helps in maintaining a scalable, organized, and efficient project, similar to a factory where each part is stored in a specific place and can be easily found when needed.
Readers also liked: What Is .next Folder in Next Js
This organization also facilitates collaboration among team members, allowing different team members to work on different aspects of the project simultaneously without stepping on each other's toes. By categorizing components according to their functionality or purpose, it becomes easier for team members to work together and maintain a cohesive project.
For more insights, see: Nextjs Team
Component Structure
Organizing components is crucial for maintaining a well-structured project. This involves creating a 'components' folder for storing reusable components and subfolders to sort them according to functionality or purpose.
Having a well-organized library of components makes it easier to locate the exact component you need in your codebase. This practice also helps in maintaining a scalable, organized, and efficient project, similar to a well-run factory where each part is stored in a specific place.
A good component structure facilitates collaboration among team members by allowing different team members to work on different aspects of the project simultaneously. This helps prevent conflicts and streamlines the development process.
Organizing components effectively can also speed up the development process and reduce the likelihood of errors. By having a clear understanding of where each component is located, you can quickly identify and modify components as needed.
You might like: Nextjs Pathname Server Component
Creating Reusable Components
Creating Reusable Components is a game-changer in web development, conserving time, ensuring application consistency, and resulting in cleaner, more manageable code. It's as simple as creating a file with a .js extension in the components directory of your project.
Reusable components are essential for maintaining a well-organized project, just like a well-run factory where each part is stored in a specific place and can be easily found when needed. This practice helps in maintaining a scalable, organized, and efficient project.
To create reusable components, you should adhere to best practices like using ComponentProps and HTMLAttributes to specify the props and attributes that can be passed to the component. This helps in making your code reusable, scalable, maintainable, and efficient.
Breaking down your application into smaller components is another key practice in creating reusable components. This makes it easier to manage and organize components within your Next.js project.
Here are some key practices to keep in mind when creating reusable components:
- Use ComponentProps and HTMLAttributes to specify props and attributes
- Break down your application into smaller components
- Utilize functional or class components, similar to regular React development
Component Isolation
Component Isolation is crucial for security and architectural integrity in web applications built with Next.js. This means making a clear distinction between server-only and client-accessible code to prevent sensitive data leakage.
Declaring 'server-only' at the top of a file will enforce isolation, causing a build error if client components attempt to import server-only code. This is a key aspect of robust component isolation.
Middleware in Next.js establishes a well-defined boundary between server and client components, ensuring only necessary information reaches the client. This is a safeguard against unintended server logic from reaching the client.
React Server Components protocol dictates that any data passed to client components is serialized in a superset of JSON, excluding complex data instances like classes. This prevents unintended server logic from reaching the client.
Server components should fetch data server-side using Next.js's data fetching methods and pass serialized data to client components. This is a better practice than passing complex data instances directly.
The experimental React Taint APIs introduced in Next.js 14 can be used to further protect sensitive operations from crossing into the client's domain. These APIs can help you lay the foundation for secure, maintainable, and well-structured web components in Next.js.
See what others are reading: Next Js React Fundamentals
Component Interaction
In Next.js, components can receive data and functionality from their parent components through props.
Props are like messengers that carry information from the top of the component tree to the bottom, allowing components to customize their behavior and appearance.
To define props in a component, you simply pass them as parameters to the component function, just like you would with any other function.
For example, in the MyComponent function, you can extract values like name and age from the props using dot notation.
This enables components to be highly customizable and reusable, which is a key benefit of building with Next.js.
You can pass as many props as you need to a component, making it easy to create complex and dynamic user interfaces.
Discover more: Next Js Props
Performance and Security
Leveraging Server-Side Rendering (SSR) and React Server Components (RSC) can significantly trim the time it takes for content to be seen by users and crawled by search engines.
SSR excels at transmitting fully-rendered pages from the server, enhancing initial load times, while RSC operates in a distinct module system than SSR, safeguarding the application by preventing server-side code from being exposed to the client.
Consider reading: Rsc Nextjs
Developers should strategically use SSR to serve static content and pieces critical for SEO, while RSC is best suited for handling dynamic elements that don't require immediate client-side loading.
Server-Side Rendering merely enhances the upfront rendering stage without stifling the potential for client-side dynamics, allowing components to evolve into fully dynamic elements upon client-side hydration.
RSC permits state management server-side, streamlining client-side architecture and curbing resource utilization, breaking down responsibilities clearly: SSR lays out the main structure and metadata, while RSC enriches specified zones with complex state management or intensive calculations.
By marrying SSR and RSC within your application, you can initiate with SSR to deliver a swift, SEO-optimized access point before supplementing with RSC which overlays progressive enhancements on the webpage, fortifying the security posture and rigorously dictating component access to server-side resources.
Consider reading: Next Js Client Side Rendering
Optimizing Performance with SSR and RSC
Using Server-Side Rendering (SSR) and React Server Components (RSC) can significantly boost your application's performance. SSR excels by transmitting fully-rendered pages from the server, enhancing initial load times—a boon for both user experience and search engine discoverability.
For your interest: Nextjs App Route Get Ssr Data
SSR and RSC collaborate to create a seamless user experience. RSC operates in a distinct module system than SSR, safeguarding the application by preventing server-side code from being exposed to the client.
Developers should strategically use SSR to serve static content and pieces critical for SEO, while RSC is best suited for handling dynamic elements that don't require immediate client-side loading. This approach enables quicker initial engagement with the web page.
SSR merely enhances the upfront rendering stage without stifling the potential for client-side dynamics. It's paramount to comprehend that static components can evolve into fully dynamic elements upon client-side hydration.
RSC permits state management server-side, streamlining client-side architecture and curbing resource utilization. This fine-tuned approach breaks down responsibilities clearly: SSR lays out the main structure and metadata, while RSC enriches specified zones with complex state management or intensive calculations.
By initiating with SSR to deliver a swift, SEO-optimized access point and supplementing with RSC, you can overlay progressive enhancements on the webpage. This stratagem advocates for superior application speed and fortifies the security posture.
You might enjoy: Nextjs State Management
Client-Side Component Security Audit Techniques
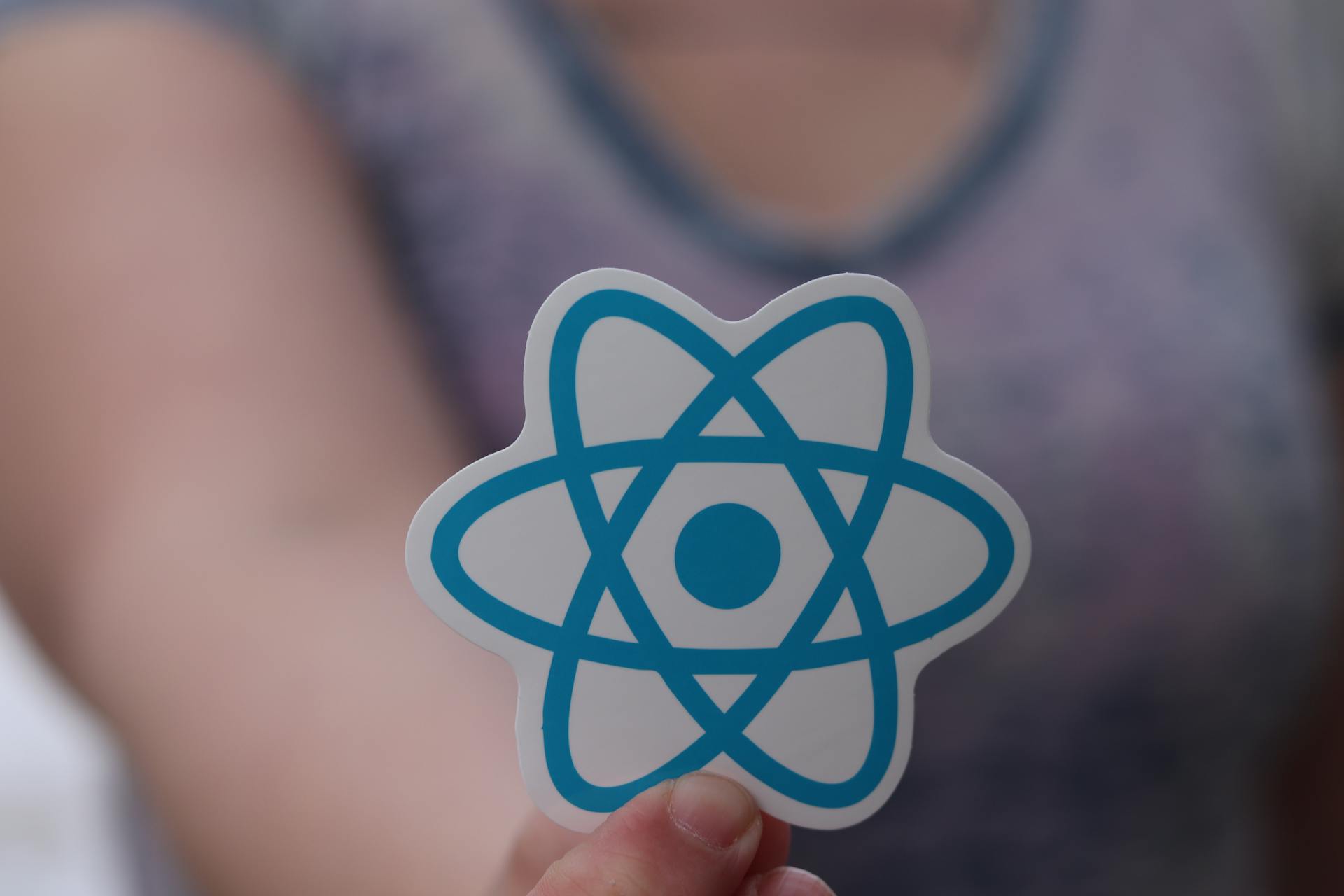
A thorough client-side component security audit is essential in Next.js applications. This involves reviewing client-side code to ensure sensitive data manipulations are securely guarded.
Client components must be designed to operate assuming they live entirely in the user's browser environment, where access to server environment variables or direct database interactions is strictly prohibited for security reasons.
A focused audit of Middleware and Route Files like middleware.js and route.tsx is critical, as they wield significant authority in controlling page access and request handling. Apply penetration testing and vulnerability scanning tailored to your development lifecycle to spot security oversights.
Server-side validation is crucial for dynamic route parameters, designated by bracketed folder names like /[param]/. This prevents vulnerabilities often associated with incorrect or malicious user input.
Client files should not expect props to contain private data, and server files should contain strict validation logic to sanitize action arguments. Authorization checks should always be performed server-side to avoid exposing sensitive operations.
Explicit permissions for HTTP methods, particularly the POST method, should be set to ensure they are selectively accessible based on user authorizations. This bolsters the security of Server Actions.
Explore further: Debug Nextjs Client Frontend Webstorm
Featured Images: pexels.com