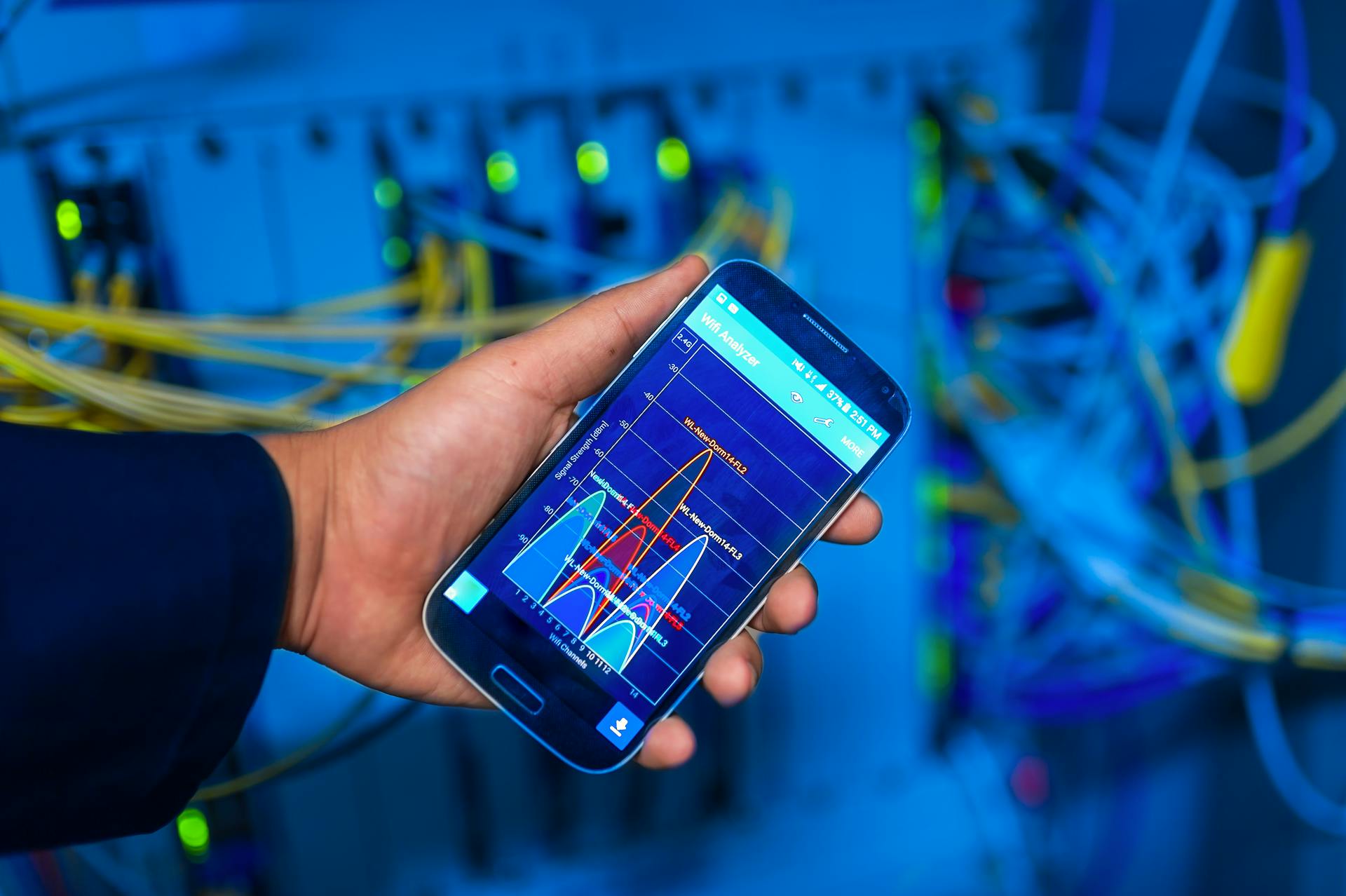
Next.js Pathname Server Component is a powerful tool that allows you to handle client-side routing in a more efficient way.
It's built on top of the Next.js server-side rendering (SSR) capabilities, which means you can render pages on the server before sending them to the client.
This allows for faster page loads and better SEO, as search engines can crawl and index your pages more easily.
The Pathname Server Component is particularly useful when you need to handle dynamic routes, such as blog posts or product pages, where the URL path can change based on the content.
Recommended read: Nextjs Get Pathname
What are Server Components
Server components are a type of component in Next.js that can be rendered on the server.
They allow for server-side rendering of components, which can improve performance and SEO.
Server components are built on top of the React framework and can be used to create reusable UI components.
Server components can be used to render dynamic content, such as user profiles or search results.
A fresh viewpoint: Can Nextjs Be Used on Traditional Web Application
Server components can be used to render static content, such as pages and layouts, which can improve performance.
Server components can be used to create APIs, which can be used to fetch data from external sources.
Server components can be used to create reusable UI components, such as buttons and forms.
Server components can be used to handle server-side logic, such as authentication and authorization.
Server components can be used to create dynamic routes, which can be used to render different content based on the URL.
Server components can be used to create reusable UI components, such as navigation menus and footers.
Recommended read: Next Js Movie Streaming Ui
Using Server Components in Next.js
Using Server Components in Next.js is a game-changer for building fast and scalable applications. Server Components allow you to render components on the server, which can improve performance and reduce the load on the client.
Server Components can be used to render complex UI components, such as a dashboard or a list of items, which can be expensive to render on the client. This is particularly useful for applications that require a lot of data to be fetched and rendered.
By leveraging Server Components, you can improve the performance of your Next.js application and provide a better user experience.
Worth a look: Deploy Nextjs on Render
Benefits
Using Server Components in Next.js offers several benefits, making it a popular choice among developers.
Improved performance is one of the key advantages, as server components can handle complex computations on the server-side, reducing the load on the client-side and resulting in faster page loads.
Server components also enable better SEO, as static HTML is generated on the server, making it easier for search engines to crawl and index.
Server components can be used to build complex, data-driven applications with ease, thanks to their ability to handle dynamic data fetching and rendering.
By separating presentation and logic, server components promote better code organization and maintainability, making it easier to update and refactor codebases.
Server components also offer improved security, as sensitive data is handled on the server-side, reducing the risk of client-side data breaches.
Server components can be used to build progressive web apps (PWAs) with ease, thanks to their ability to handle dynamic data and provide a seamless user experience.
Consider reading: Next Js Client Portal
Getting Started
To use server components in Next.js, you need to create a new project with the `create-next-app` command and specify the `--experimental-app` flag.
Server components are enabled by default in Next.js 13, so you don't need to do anything extra to get started.
First, install the required packages, including `@next/dynamic` and `@next/server-exporter`.
Then, create a new file called `pages/_app.js` and add the `useServerComponent` hook to enable server components.
For example, you can create a simple server component by exporting a function from a file in the `components` directory.
Server components can be used to render dynamic content on the server, such as API calls or database queries.
To use a server component, you can import it in a page component and render it using the `useServerComponent` hook.
For example, you can create a server component that fetches data from an API and renders it on the page.
Server components can also be used to improve the performance of your application by reducing the amount of data that needs to be sent over the network.
Related reading: Nextjs Stripe
Example Use Cases
Server components in Next.js can be used to build reusable UI components that fetch data on the server, reducing the amount of data transferred to the client.
For example, in the "Building a Simple Server Component" section, we saw how to create a server component that fetches user data from a mock API, allowing us to render user information on the server before sending it to the client.
Server components can also be used to handle complex business logic, such as authentication and authorization, which can be a challenge to implement in traditional client-side applications.
As we saw in the "Using Server Components for Authentication" section, server components can be used to create a secure authentication system that verifies user credentials on the server before allowing access to protected routes.
This approach can significantly improve the security of our application and reduce the risk of client-side attacks.
By using server components, we can also improve the performance of our application by reducing the amount of data transferred to the client and minimizing the number of requests made to the server.
For instance, in the "Optimizing Server Components" section, we learned how to use server components to cache frequently accessed data, reducing the number of requests made to the server and improving the overall performance of our application.
For another approach, see: Nextjs Ui Library
Dynamic Routing
Dynamic Routing is a powerful feature in Next.js that allows you to create client-side routed pages.
With Dynamic Routing, you can use the `getStaticPaths` method to pre-render pages at build time, but also use the `useRouter` hook to fetch data on the client-side.
To define a dynamic route, you need to use the `use` keyword in your route path, like `use ['/posts', '/posts/:id']`.
In the example, the `getStaticPaths` method is used to generate an array of paths for the `posts` page, which includes the `:id` parameter.
By using the `useRouter` hook, you can fetch data on the client-side and render the page dynamically.
The `use` keyword in the route path allows you to define a parameterized route, like `:id`, which can be used to fetch data from an API.
In the example, the `getStaticProps` method is used to fetch data from an API and pass it to the `posts` page.
Dynamic Routing in Next.js is a flexible and powerful feature that allows you to create complex client-side routed pages with ease.
Explore further: Next Js Fetch Data save in Context and Next Route
Server Component Pathname
Server Component Pathname is a crucial aspect of Next.js server components. It determines the URL path that will be used to access the component.
In Next.js, you can use the `pathname` property to get the current pathname of a server component. This property is automatically provided by Next.js.
The `pathname` property is a string that represents the URL path of the component. It can be used to generate URLs or to determine the component's routing context.
Server components can also use the `usePathname` hook to get the current pathname. This hook is a more functional way to access the pathname, and it's often used in functional components.
The pathname is resolved to a specific file path on the server, which is then rendered as the component's HTML output. This allows for more efficient routing and better performance.
Consider reading: Nextjs Redirect from Server Component
Frequently Asked Questions
How to get URL in Next.js server side?
To get the current URL in a Next.js server-side component, you can either convert it to a client component and use `usePathname` or add middleware to pass the URL as a header.
Sources
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://i18nexus.com/tutorials/nextjs/react-intl
- https://nextjs.org/docs/app/building-your-application/routing
- https://tanstack.com/query/latest/docs/framework/react/guides/advanced-ssr
- https://nextjs.org/docs/app/building-your-application/rendering/server-components
Featured Images: pexels.com