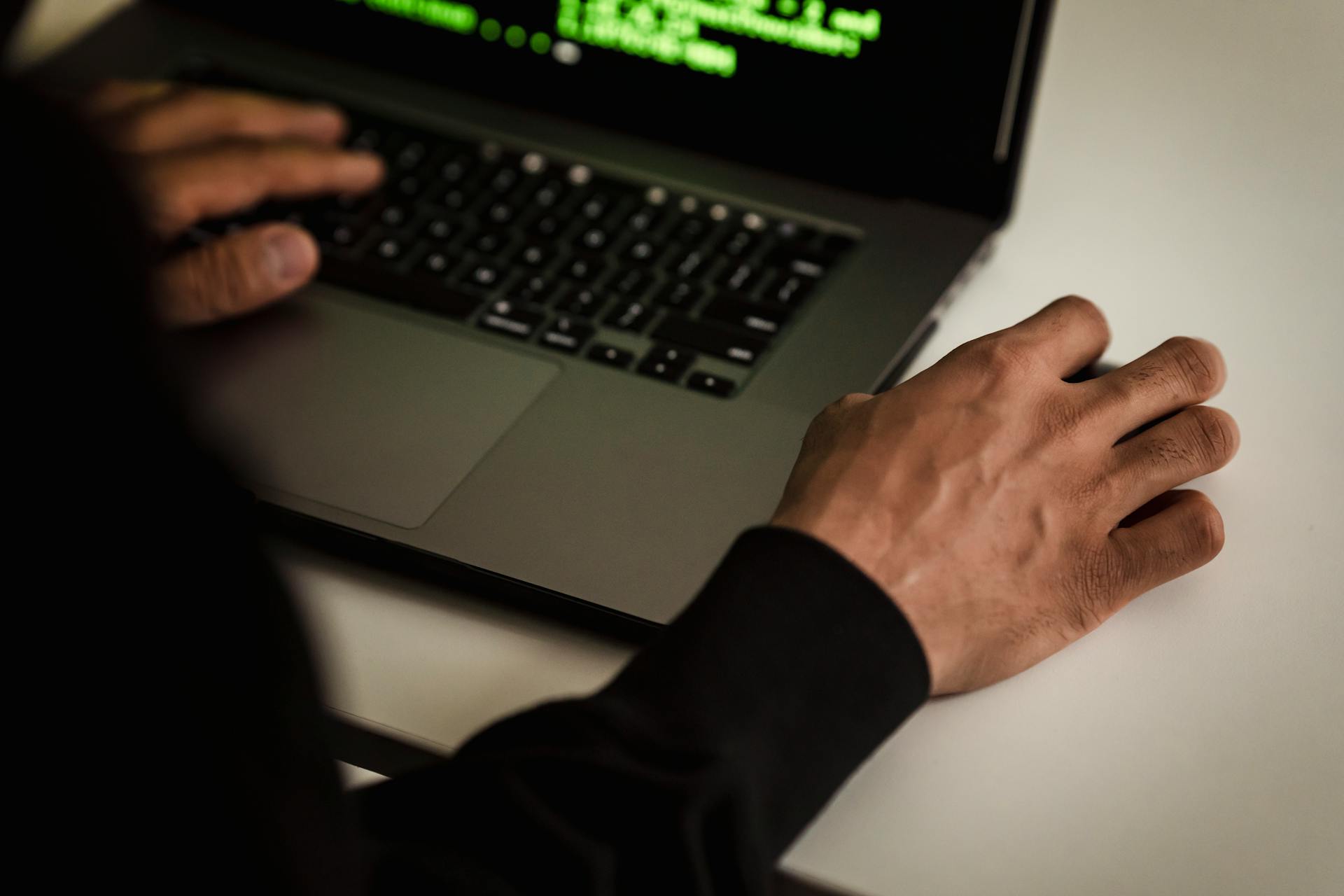
Getting the pathname in Next.js is crucial for building robust applications. The pathname is the URL of a page, and it's used to determine the current page being viewed.
Next.js provides a built-in API called `useRouter` to get the pathname. This hook returns the current pathname as a string.
The pathname can be used to display dynamic content, such as user profiles or product details. For example, if the pathname is `/users/john`, you can display John's profile information.
To get the pathname in a Next.js page, you can use the `useRouter` hook and access the `pathname` property. For instance, `const { pathname } = useRouter();` will give you the current pathname.
For more insights, see: Next Js Page Transition Animation Loader
Routing Basics
Routing in Next.js is based on a file system, where each route is defined by a separate file in the pages folder.
The structure of the pages folder determines the structure of your routes, with each file inside the folder mapping to a route in your application.
A unique perspective: Pages Router Next Js
To create a new route, you need to add a file in the pages folder, and the file name determines the route path.
For static routing, you can create a new route by adding an index.js or a named file like about.js.
The pages folder itself represents your root URL, meaning /.
To create a dynamic route, you can use brackets around parameters in the file name, such as [id].js.
Next.js handles dynamic routes by supporting brackets around parameters in the file name, such as [id].js.
The Link component in Next.js allows for navigation between pages, and it can accept an object for the href attribute.
The Link component takes the pathname and query parameters from the object and formats them into the right URL.
Suggestion: Dynamic Routing Nextjs
Dynamic Routing
Dynamic routing in Next.js is a powerful feature that allows you to create routes that are determined by user input or other dynamic factors.
You can create dynamic routes by using brackets around parameters in your file names, such as [id].js.
Worth a look: Intercepting Routes Next Js Example
For example, if you want to create a route for a blog post, you can create a [id].js file inside your posts folder.
This will map to /posts/[id], where id is the unique id of your post.
The catch-all route is another type of dynamic route that uses three dots (...) in the bracket syntax, such as [...slug].js.
This type of route can handle multiple parameters and is useful for creating routes that can handle different types of data.
For instance, the route /printed-books/[...slug] can handle multiple parameters, such as /printed-books/click.js, /printed-books/2020/click.js, or /printed-books/design/2020/click.js.
Here's a table summarizing the different types of routes in Next.js:
The router object in Next.js provides information about the URL state of the current page, including the pathname, query, and asPath.
You can use the useRouter hook or the withRouter higher-order component to access the router object.
For example, you can use the push method to navigate to a new URL by adding a new URL entry into the history stack.
To navigate to dynamic routes, you can use the Link component, which accepts an href and wraps around a piece of code to navigate to a page.
The Link component can also accept an object for href, which contains parameters such as pathname and query.
This allows you to create links to dynamic routes, such as /posts/[id], without having to hard-code the URL.
Explore further: Next Js Routing
Route Configuration
Route Configuration is a crucial aspect of Next.js development, and understanding it can help you create efficient and scalable routes for your application.
You can define common route patterns using Next.js' file-based routing system, which separates each route based on its definition. This helps eliminate redundancies and makes your code more organized.
To accommodate dynamic routes, you can use the bracket syntax, such as [book-id].js, which takes things further with Catch-All Routes. Catch-All Routes are denoted by three dots (...) before the bracket syntax and can catch all routes.
Here's a breakdown of route types in Next.js:
Predefined routes come first, followed by dynamic routes, then catch-all routes. This is known as route precedence, which helps resolve potential clashes between routes.
Here's an interesting read: Nested Routes in Next Js
To create a dynamic route, you can add a file with bracket syntax in the pages folder, such as [id].js. This will map to a route like /posts/[id] where id is the unique id of your post.
To navigate to a dynamic route, you can use the Link component, which accepts an href and wraps around a piece of code to navigate to a page. You can also pass an object with pathname and query parameters to the Link component, which will automatically format the URL for you.
Discover more: Dynamic Route Nextjs 13
Working with Routes
In Next.js, routes are defined in a file-based system, where each file represents a route. This allows for easy management of routes and eliminates the need for complex configuration.
To create a dynamic route, you can add brackets around parameters in the file name, such as [id].js. This will map to a route like /posts/[id].
Route precedence is determined by specificity, with predefined routes coming first, followed by dynamic routes, and then catch-all routes. This helps prevent route clashes and ensures that the correct route is handled.
Here's a summary of route types:
19 Answers
Working with routes in Next.js can be a bit tricky, but don't worry, I've got you covered. To access the router object inside any functional component in your app, you can use the useRouter hook.
The useRouter hook is a convenient way to get the router object, but if it's not the best fit for you, you can also use withRouter to add the same router object to any component.
If you're wondering how to access the router instance in Next.js API routes, you can use the asPath property, which gives you the path (including the query) shown in the browser without the configured basePath or locale.
The asPath property is particularly useful when you need to get the pathname extracted from the URL, as opposed to the pathname of your project directory.
Here are the two properties you can use to get the pathname:
- asPath property: contains the pathname extracted from the URL
- pathname property: contains the pathname of your project directory
For example, if the complete URL of a page is 'abc.com/blog/xyz' and the component file name matching with this route is './pages/blog/[slug].js', the asPath property would contain '/blog/xyz', while the pathname property would contain '/blog/[slug]'.
URL Objects
In Next.js, the Link component can accept a URL object as its href prop, which allows for more flexibility when creating links to dynamic routes.
This URL object can contain properties like query, which is automatically formatted into a URL string.
The Link component can also handle dynamic segments in the pathname by including them as properties in the query object.
For example, if you have a URL object with the following properties: pathname: '/printed-books/[id]' and query: { id: 'ethical-design' }, the Link component will automatically format it into a URL string like '/printed-books/ethical-design'.
Here's an example of what the URL object might look like:
```html
{
pathname: '/printed-books/[id]',
query: { id: 'ethical-design' }
}
```
You can also include multiple properties in the query object, like this:
```html
{
pathname: '/printed-books/[id]',
query: { id: 'ethical-design', name: 'Ethical Design' }
}
```
This will result in a URL string like '/printed-books/ethical-design?name=Ethical+Design'.
Broaden your view: Router Query Params Nextjs 14
The Router Object
The router object is a powerful tool in Next.js, providing information about the URL state of the current page. It has properties like pathname, query, asPath, and basePath that give you information about the URL state of the current page.
Worth a look: Next Js 14 Redirect to Another Page Loading Indicator
The router object is returned by both the useRouter hook and the withRouter higher-order component. You can use this object to access information about the active locale, supported locales, and default locale.
The router object has methods like push for navigating to a new URL by adding a new URL entry into the history stack. It also has a replace method, similar to push but replaces the current URL instead of adding a new URL entry into the history stack.
The router object is essential for working with dynamic routes in Next.js. By using the useRouter hook, you can access the router object inside any function component. This allows you to manually perform routing, which can be useful in certain scenarios.
Here's an interesting read: Nextjs 14 Enviar Parametros En La Url
Sources
- https://stackoverflow.com/questions/58022046/get-url-pathname-in-nextjs
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://nextjs.org/docs/app/building-your-application/routing/redirecting
- https://www.smashingmagazine.com/2021/06/client-side-routing-next-js/
- https://mariestarck.com/a-beginners-guide-to-dynamic-routing-in-next-js/
Featured Images: pexels.com