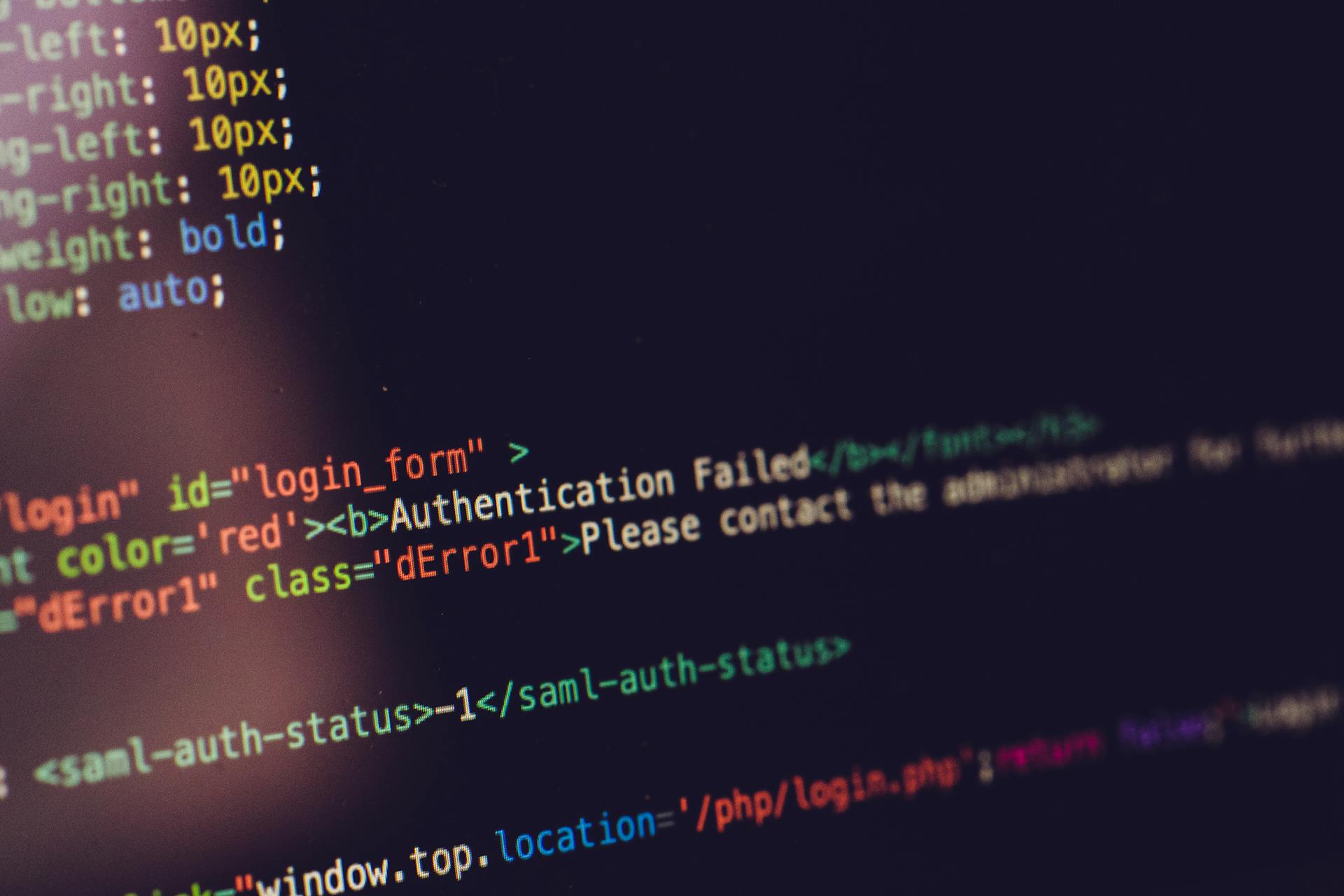
Setting up a dynamic router in Next.js is a crucial step in building a robust and scalable application. To begin, you'll need to create a new file in the pages directory, such as `[id].js`, which will serve as the base for your dynamic routes.
This file will contain the basic structure for your dynamic routes, including the `getStaticProps` method, which fetches data at build time. For example, the `getStaticProps` method can be used to fetch data from an API, such as the `fetch` function.
In your `[id].js` file, you can define the dynamic route pattern using the `useRouter` hook from the `next/router` package. This hook provides access to the router's context, allowing you to manipulate the URL and fetch data accordingly. For instance, you can use the `useRouter` hook to fetch data based on the dynamic route parameter, `id`.
Next, you'll need to configure the dynamic router by creating a new instance of the `DynamicRouter` class in your `pages/_app.js` file. This instance will be responsible for rendering the dynamic routes.
Recommended read: Pages Router Next Js
Next.js Dynamic Router Fundamentals
Dynamic route segments can be extended with catch-all routes, which eliminate redundancy in deeply nested routes. This is achieved by prefixing the bracket syntax with three dots, like the JavaScript spread syntax.
You can access the category and release year in two ways: either by attaching the metadata to each book info, or by returning them as an array of query parameters. In the case of the printed-books example, the end goal is the book.
Here's how the query parameter is structured for different routes:
Catch-all routes are "strict" and will throw a 404 error unless you provide a fallback index route.
Segments
Segments are a powerful feature in Next.js that allow you to create flexible and dynamic routes. You can extend dynamic route segments with catch-all routes, which eliminate the need for deeply nested routes.
Catch-all routes use the same bracket syntax as dynamic routes, but with three dots (...) instead of a single bracket. Think of the dots like the JavaScript spread syntax. This syntax is used to catch all routes, and it's especially useful when you have multiple parameters in your route.
Related reading: Nested Routes in Next Js
For example, if you have a route like /printed-books/:category/:release-year/:book-id, you can use catch-all routes to eliminate the need for deeply nested routes.
Here's an example of how you can use catch-all routes:
As you can see, catch-all routes return the slug segments as an array of query parameters. You can access these parameters in your route handler using the query object.
However, if you use a catch-all route, the route /printed-books will throw a 404 error unless you provide a fallback index route. This is because the catch-all route is "strict" and either matches a slug or throws an error.
If you'd like to avoid creating index routes alongside catch-all routes, you can use optional catch-all routes instead. Optional catch-all routes use double square brackets [[…slug]] instead of three dots. This makes the slug parameter optional, and if it's not provided, the route will fallback to the path /printed-books, rendered with [[…slug]].js route handler, without any query params.
Related reading: Nextjs Slug
Prior to Next.js 9.5.3, linking to dynamic routes required providing both the href and as prop to Link. However, this was tedious, error-prone, and somewhat imperative. With the release of Next.js 10, this has been fixed for the majority of use-cases. To adopt the new syntax, simply discard the href prop and its value, and rename the as prop to href.
Internationalized (i18n)
Internationalized routing is a powerful feature in Next.js that allows you to create different routes for different locales.
You can set up your application to handle multiple languages and Next.js will automatically route users based on their locale. This means you can create a global application without requiring separate setups for each locale.
Internationalized routing is simple and powerful in Next.js, making it easy to create a multi-language application.
You can configure locales in next.config.js, which is a key step in setting up internationalized routing in Next.js.
Internationalized routing opens the door to global applications without requiring separate setups for each locale.
Custom Redirects in Next.js
Custom Redirects in Next.js are a game-changer for SEO and user experience improvements. You can configure custom routing rules and redirects directly in next.config.js, eliminating the need for manual management or middleware.
This centralized routing control helps you manage large projects with clean, readable configurations. It's a huge time-saver and reduces the risk of errors.
To take it a step further, you can use middleware to execute code before a request is completed. This allows you to dynamically handle routing or permissions on any request.
Here are some key benefits of using middleware for route control:
- Custom routing logic
- Authentication
- Logging
- A/B testing
By using middleware, you can precisely control routing, making it perfect for security checks or A/B testing. This is especially useful for redirecting old paths to new ones, providing a seamless user experience.
Router Configuration
When configuring the frontend in Next.js, you don't have to mention the starInterface as well as boolean within the function's arguments for JavaScript.
You can set the fallback from getStaticPaths() to true or 'blocking' if you're not able to provide every dynamic route for pre-loading. This will prevent your page from crashing and instead load the page on the fly as the user accesses the dynamic route.
To indicate a loading sequence, you can use router.isFallback to return JSX with a loading indication for the user.
Precedence
Precedence is crucial in routing, and Next.js has a clear approach to resolving route clashes.
In Next.js, predefined routes come first, followed by dynamic routes, then catch-all routes. This means that if you have multiple routes defined, the most specific one will be chosen.
For example, if you have a route like /printed-books/[book-id].js and another like /printed-books/[…slug].js, the former will win because it's more specific.
Route clashes can occur when you have multiple dynamic routes on the same level, which is an error. This is why it's essential to understand the precedence rules to avoid conflicts.
For more insights, see: Intercepting Routes Next Js Example
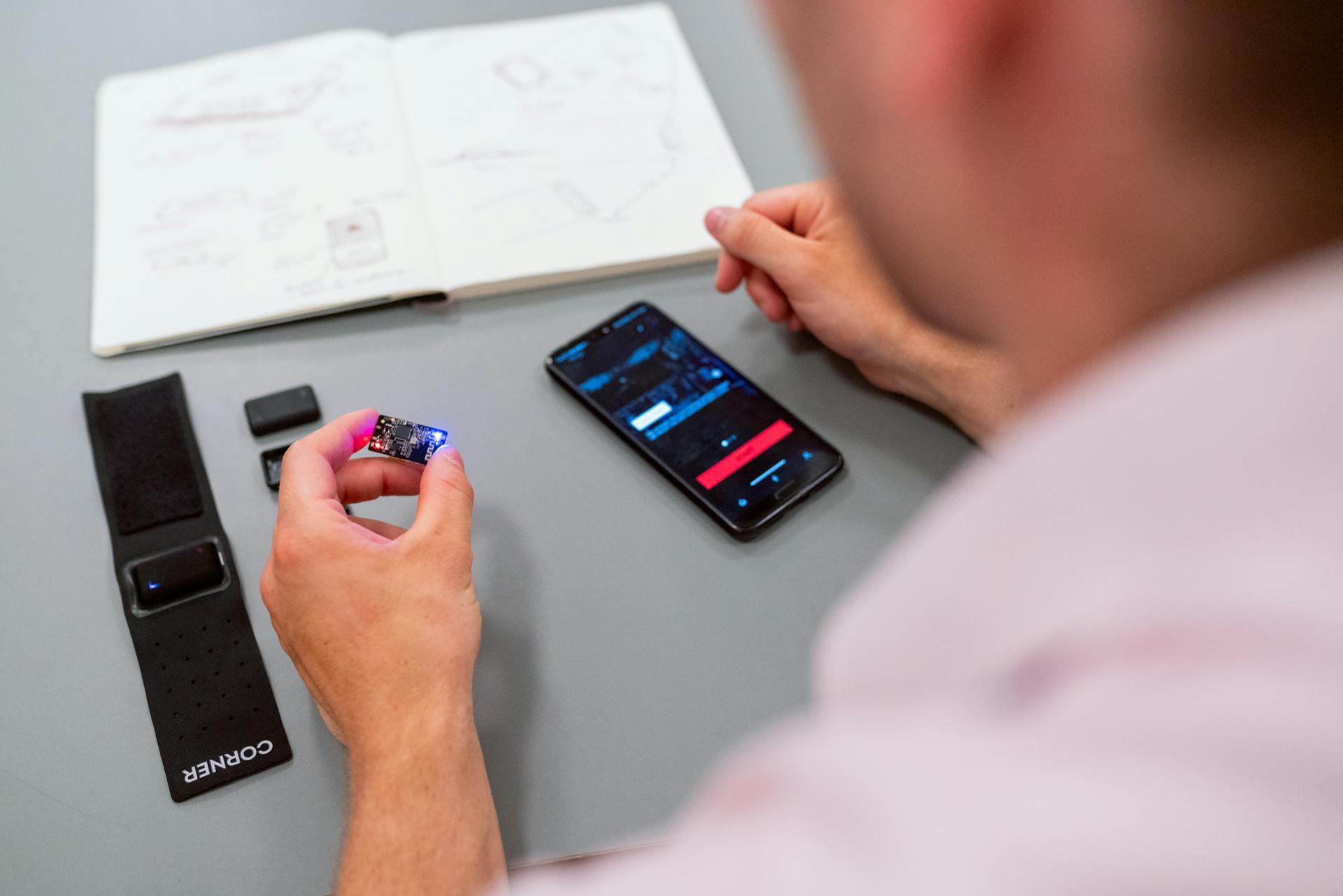
Here's a summary of the route precedence model:
This pseudo-code model helps you understand the route request/handling process:
Is there a predefined route handler that can handle the route?
Is there a dynamic route handler that can handle the route?
Is there a catch-all route handler that can handle the route?
What Is the Fallback Property?
The fallback property is a useful feature in router configuration that helps handle situations where a user tries to access a path that doesn't exist in getStaticPaths().
Fallback set to false will automatically lead to a 404 error page, while fallback set to true doesn't automatically lead to a 404 error page, allowing you to handle the situation on the frontend by displaying a loading sequence or error message.
Fallback set to 'blocking' is similar to true, but it omits manual loading processes, letting the browser take a moment longer to fetch the data before displaying the page.
Take a look at this: Nextjs Page Transition App Router Tailwind
Here are the different behaviors of the fallback property:
By trying out different fallback values, you can find out how your application's behavior changes and choose the best approach for your use case.
Dynamic Routing
Dynamic routing in Next.js is a powerful feature that allows you to create pages that can handle dynamic URL segments. You can use catch-all routes to capture multiple dynamic URL segments, which is useful for building paths with variable depth.
Catch-all routes are defined using square brackets, for example, pages/blog/[...slug].js. This route will match any URL starting with /blog/, such as /blog/post1, /blog/2024/08/post, or /blog/category/subcategory/post. The captured segments are available as an array.
Optional catch-all routes provide more flexibility in matching URLs with or without dynamic parameters. They are defined using double square brackets, for example, pages/blog/[[...slug]].js. This route will match URLs like /blog, /blog/post1, /blog/category/post, etc.
Here's a comparison of catch-all and optional catch-all routes:
Rendering
Rendering is a crucial aspect of Next.js, and it's closely tied to routing. Next.js pre-renders each page in advance, alongside minimal JavaScript code, through a process called hydration.
This process is highly dependent on the form of pre-rendering used, which can be either Static Generation or Server-side rendering. Both of these methods are highly coupled with the data fetching technique used.
Next.js has built-in data fetching functions like getStaticProps, getStaticPaths, and getServerSideProps that you can use for pre-rendering purposes. You can also use client-side data fetching tools like SWR, react-query, or traditional data fetching approaches like fetch-on-render, fetch-then-render, render-as-you-fetch with Suspense.
Pre-rendering is complementary to routing and highly coupled with data fetching, making it a crucial topic to understand in Next.js.
Consider reading: Next Js Fetch Data save in Context and Next Route
Catch-All
Catch-all routes are a powerful tool in Next.js for capturing multiple dynamic URL segments. They allow you to build paths with variable depth, making them super useful for a wide range of applications.
To create a catch-all route, you use the syntax `pages/blog/[...slug].js`. This route will match any URL starting with `/blog/`, such as `/blog/post1` or `/blog/2024/08/post`. The captured segments are available as an array, which can be accessed in your route handler.
Here's an interesting read: Nextjs 14 Enviar Parametros En La Url
Here's a breakdown of what the `slug` array contains in a catch-all route: it's a list of all the URL segments following the initial `/blog/` path. For example, if the URL is `/blog/2024/08/post`, the `slug` array would contain `['2024', '08', 'post']`.
Catch-all routes can be used to match URLs with variable depth, making them a great choice for building dynamic routes. For instance, you could use a catch-all route to create a blog with categories and subcategories.
Optional catch-all routes provide even more flexibility by allowing you to match routes with or without dynamic parameters. They use the syntax `pages/blog/[[...slug]].js` and will match URLs like `/blog`, `/blog/post1`, or `/blog/category/post`.
Explore further: Dynamic Route Nextjs 13
Advanced Features
Next.js offers advanced features that enable developers to create flexible applications.
Beyond basic routing, Next.js allows for dynamic routing, which is a game-changer for building scalable applications.
Next.js routing enables developers to create high-performance applications.
The advanced features of Next.js routing make it an ideal choice for building complex and dynamic applications.
With Next.js, developers can create flexible and scalable applications that meet the demands of modern web development.
For your interest: Nextjs Dynamic
Optimize
Shallow routing is a game-changer for dynamic UIs, especially when it comes to search filters.
It allows you to change the URL without triggering a page re-render, improving navigation speed and avoiding unnecessary re-renders.
This is especially helpful in dynamic UIs like search filters.
Shallow routing enables efficient URL state management, making it a valuable optimization technique.
By using shallow routing, you can create a smoother user experience without sacrificing performance.
Pre-fetching routes can also be used to optimize performance, especially when hovering over buttons or menu items.
This allows for faster page transitions and a more seamless user experience.
By pre-fetching routes programmatically, you can ensure a smoother user experience for your users.
Intriguing read: Next Js Routing
API and Data Fetching
Dynamic routing is not limited to page routes, you can also apply it to API routes to build flexible and reusable back-end functionality.
With dynamic routing, API routes can dynamically serve content based on the URL, perfect for product or user management systems.
Broaden your view: Nextjs 13 Api
API routes can be set up with dynamic route segments, allowing for a more flexible and scalable approach to building back-end functionality.
For example, an API route like /api/users/:id can serve different user data based on the id parameter.
This approach is particularly useful for product or user management systems where data needs to be served dynamically based on the URL.
Data Fetching
In Next.js, you can use dynamic routing to fetch data for a specific page based on a unique identifier in the URL. This is particularly useful for displaying information about a particular item, such as a product.
The Link component can be used to lead the user to a dynamic route with a valid parameter, like a product id. This is shown in Example 5, where a Link element is used to link to a dynamic route with a valid parameter.
API routes can also be used for dynamic routing, allowing you to build flexible and reusable back-end functionality. This is demonstrated in Example 3, where an API route is created with a dynamic segment, such as /api/users/:id.
On a similar theme: Next Js App Router Example
For dynamic routing to work, you need to set up a backend JSON data file, like the some-backend-data.json file shown in Example 4. This file contains dummy data that can be used to reproduce a situation where you have access to data in the backend.
You can use the useRouter hook from the next/router API to manually perform routing and fetch data for a specific page. This hook allows access to the router object inside any function component, making it easy to fetch data based on the current route.
The useRouter hook is a React hook and cannot be used with classes. If you need the router object in a class component, you can use the withRouter higher-order component instead.
Setup for GetStaticPaths()
Setup for GetStaticPaths() is crucial when working with dynamic pages in Next.js. You can omit the type for getStaticPaths() in JavaScript, and you can also delete starInterface.
To tell Next.js which paths should be pre-rendered, you need to implement the getStaticPaths() function. You have two options for the paths within the return: an array with an object for every path, or path strings like '/test/St2-18', '...', '...'.
You don't need to include every path that should be pre-rendered, especially when dealing with a large dataset. For small datasets, you can give every possible path to getStaticPaths().
If this caught your attention, see: Next Js Getstaticpaths
Sources
- https://nextjs.org/docs/app/building-your-application/routing
- https://www.smashingmagazine.com/2021/06/client-side-routing-next-js/
- https://medium.com/@farihatulmaria/mastering-next-js-routing-an-in-depth-guide-to-advanced-features-5aa10e15ec7f
- https://javascript.plainenglish.io/different-ways-of-handling-dynamic-routing-in-next-js-312006f5ba1b
- https://www.freecodecamp.org/news/how-to-setup-dynamic-routing-in-nextjs/
Featured Images: pexels.com