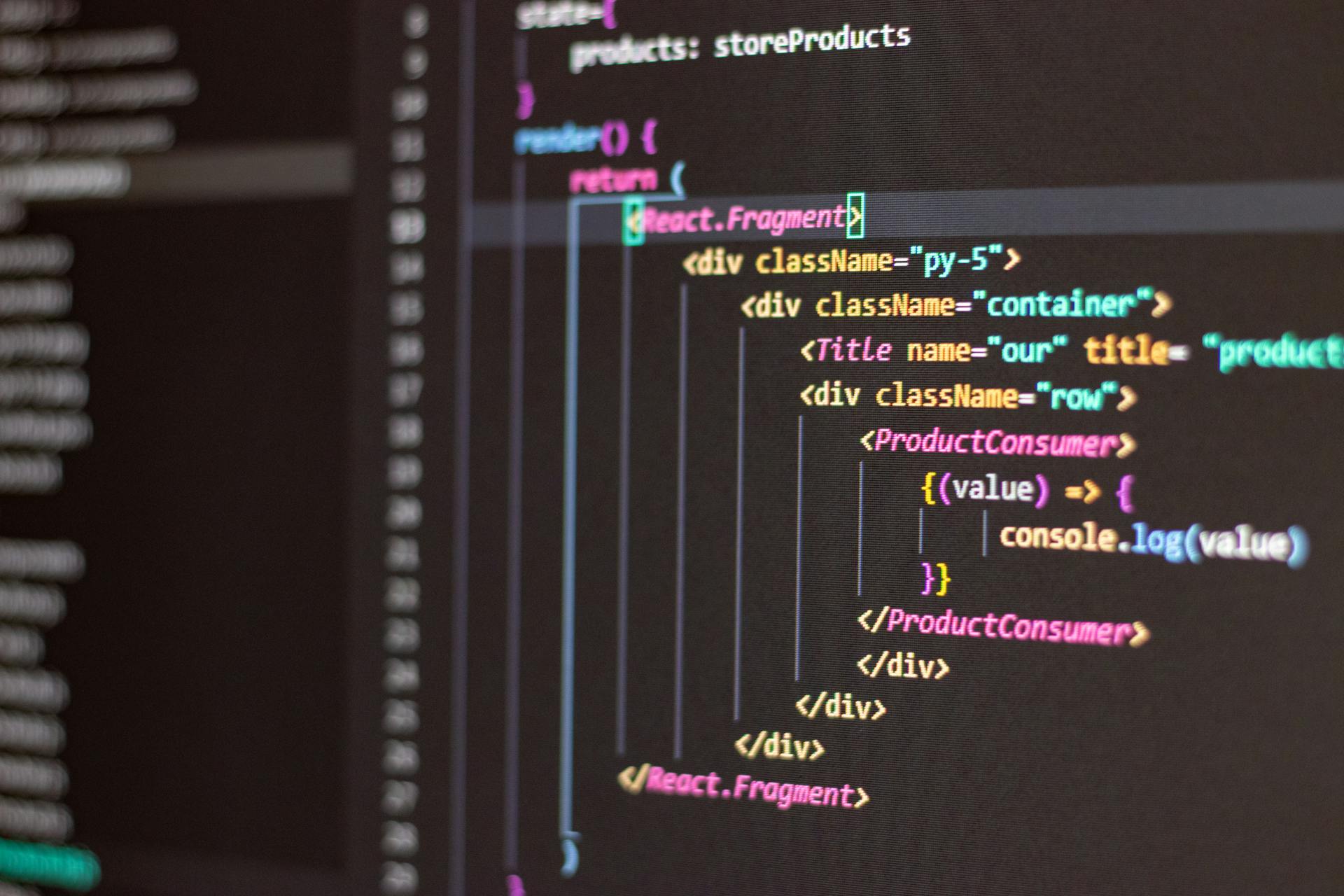
Next.js GetStaticPaths is a crucial step in creating a static site, allowing you to pre-render pages at build time.
Static site generation (SSG) is a technique that allows you to pre-render pages at build time, resulting in faster page loads and improved SEO.
GetStaticPaths is a function that helps you define which pages should be pre-rendered and how to generate the necessary data for those pages.
You can use GetStaticPaths to pre-render pages for a wide range of use cases, from blogs to e-commerce sites.
Additional reading: Nextjs Github Pages
Setup and Configuration
To set up getStaticProps(), you'll need to create the function next, which enables pre-rendering for the specific fetched data for the paths in your dynamic route.
You can omit the type for getStaticProps(), and it can provide a context parameter that allows you to access params and the current identifier of your specific path.
This context is essential for reaching out to the backend data with your getData() function, which you'll use to return the foundItem to the frontend.
Explore further: Next Js Fetch Data save in Context and Next Route
For the frontend configuration, you'll need to access your backend data with getData(), and return the foundItem in the props object, keeping in mind that everything exposed to the frontend should be kept secure.
Remember to configure your page with the fallback from getStaticPaths() set to true or 'blocking' if you can't provide every dynamic route for pre-loading, to prevent your page from crashing.
You can use router.isFallback to return JSX with a loading indication for the user, and assuming the user reached a dynamic path with data, the actual intended JSX output will be returned.
Curious to learn more? Check out: Lazy Loading Preloader in Nextjs
Setup for GetStaticProps
To set up GetStaticProps, you'll need to create the function next. For JavaScript, you can simply omit GetStaticProps as the type for getStaticProps().
getStaticProps() can provide a context parameter, which offers helpful methods. This includes accessing params and the current identifier of the specific path.
You'll use getStaticProps() to enable pre-rendering for the fetched data in your dynamic route.
Remember that everything you return in the props object will be exposed to the frontend, so don't return any credentials like personal API keys.
Recommended read: Next Js Getstaticprops
Static Path Setup
Static path setup is crucial for generating static pages with dynamic paths in Next.js. This process optimizes performance and SEO by ensuring that HTML code for each page is generated in advance.
To set up static paths, you'll need to use the `getStaticPaths()` function, which defines which pages to pre-generate. You can use two options for defining paths: an array of objects with `params` or an array of path strings.
Here are the key differences between the two options:
For a small dataset, you can give every possible path to `getStaticPaths()`, but for larger datasets, you'll want to include only the necessary paths. The `fallback` property can be set to `true` to handle manual errors and loading sequences.
It's essential to return an array of paths with `fallback` set to `true` or `'blocking'` to prevent page crashes. This will allow the page to load on the fly and provide the necessary information.
Understanding GetStaticPaths
You'll encounter an error when using getStaticProps() alone on dynamic routing pages, and that's where getStaticPaths() comes in handy. It allows you to specify which paths of the dynamic routing should be pre-rendered.
Next.js looks for the getStaticPaths() function to generate all the static pages for a template, and it's essential to return the paths in the format that Next.js is looking for. This includes an object with fallback set to false, which will make it so that if you go to an unknown path, you'll get a 404 page.
Here are the two options for the paths within the return of getStaticPaths(): an array with an object for every path you want Next.js to pre-render, or path strings like '/test/St2-18', '...', '...'.
For your interest: Next Js Pages
What's the Purpose of?
GetStaticPaths is a crucial function in Next.js that helps resolve an error you'll encounter when using getStaticProps on dynamic routing pages. getStaticProps alone can't handle dynamic routes, and that's where getStaticPaths comes in.
getStaticProps relies on static-site generation, which means Next.js pre-renders the page at build time. However, it doesn't know which paths to pre-render for dynamic routes, so you need to specify those paths with getStaticPaths.
You can use getStaticPaths to pre-render specific paths of the dynamic routing and handle unknown paths accordingly. This is a necessary step to avoid getting an error message when using getStaticProps on dynamic routing pages.
Paths
Paths are the foundation of dynamic routing, and getStaticPaths() is the function that helps Next.js pre-render pages for these routes.
You can specify which paths of the dynamic routing should be pre-rendered using getStaticPaths(). This function is essential for dynamic routes, as Next.js doesn't know which paths to pre-render on its own.
The fallback property is particularly useful for handling cases where a user tries to access a path that isn't recognized by getStaticPaths(). There are three options for fallback: false, true, and 'blocking'.
Recommended read: Next Js Dynamic
Fallback set to false would automatically lead to a 404 error page whenever the user tried to access a path that doesn't exist in getStaticPaths(). Fallback set to true doesn't automatically lead to a 404 error page, but instead, you can handle the situation on the frontend by displaying a loading sequence or an error message. Fallback set to 'blocking' doesn't automatically lead to a 404 error page either, but it omits any manual loading processes, making the browser take a moment longer to fetch the data before displaying the page.
To implement getStaticPaths(), you can use either the paths: [{ params: { something: star.id } }] or the paths: ['/test/St2-18', '...', '...'] approach. You don't need to include every path that should be pre-rendered, especially when you have a lot of cases to consider.
Here are the two options for specifying paths in getStaticPaths():
- The first option is paths: [{ params: { something: star.id } }]. This approach should be an array with an object for every path you want Next.js to pre-render.
- The second option is paths: ['/test/St2-18', '...', '...']. This approach uses path strings.
You can also use getStaticPaths() to generate paths for your app by reading files from your file system or fetching data from an external API.
Implementing GetStaticPaths
To implement GetStaticPaths, you need to understand how to return an array of possible values for each dynamic segment of the URL in the getStaticPaths function.
The getStaticPaths function should return an array of objects with the params key, which contains the actual values to be used for path parameters. This is where you define dynamic paths for your static pages.
You can return paths as an array of objects with the params key, like this: `paths: [{ params: { something: star.id } }]`. Alternatively, you can use path strings like this: `paths: ['/test/St2-18', '...', '...']`.
Here are the two options for returning paths in the getStaticPaths function:
You don't need to include every path which should be pre-rendered, especially when you have a lot of cases to consider and don't want everything to be pre-rendered. Set the fallback property to true to handle manual errors and loading sequences that might occur.
Implementing GetStaticPaths
Implementing GetStaticPaths is a crucial step in creating a dynamic SSG setup. You can pre-render dynamic routes using the getStaticPaths functionality offered by Next.js.
To start, you need to export an async function called getStaticPaths from a page that uses dynamic routes. This function specifies the paths that Next.js will statically pre-render.
The getStaticPaths function allows you to define which pages to pre-generate. You can use it to create pages for every link returned from Storyblok, except for any folder or the home story itself.
In your [...slug].js file, you can use the getStaticPaths function to create a page for every link except for any folder or the home story itself. This is because the home story is already created in your index.js file.
You can also make the catch-all routes optional by putting the ...slug in double brackets: [[...slug]].js. This main difference between catch-all and optional catch-all routes is that with optional, the route without the parameter is also matched.
It's a good idea to create the correct folder structure in Storyblok and use the catch-all routes. This way, you won't have to change the real path & slugs constantly.
Intriguing read: What Is .next Folder in Next Js
Dynamic Route Generation
Dynamic route generation is a powerful feature in Next.js that allows you to pre-render pages at build time using getStaticPaths and getStaticProps. This process is crucial for optimizing performance and SEO by ensuring that HTML code for each page is generated in advance.
You can use getStaticPaths to define which pages to pre-generate, and getStaticProps to fetch the necessary data for each page. For example, in a blog setup, you can use getStaticPaths to pre-render all the paths for your blog posts at build time, and each page will have the necessary data fetched and passed to the page component.
To implement dynamic route generation, you need to understand how to implement path parameters in the getStaticPaths function. This function should return an array of possible values for each dynamic segment of the URL.
Here's an example of how you can do it:
```html
getStaticPaths() {
const allPosts = getAllPosts();
Related reading: Next Js Build
return allPosts.map(post => ({
params: { slug: post.slug }
}));
}
```
In this code, getAllPosts would be a function that fetches all the blog posts from your data source, and you map over these posts to create an array of paths that Next.js will use to pre-render pages.
You can also use the catch-all route feature in Next.js to make your dynamic routes optional. This is useful when you don't want to hardcode every possible path in your getStaticPaths function.
Here's an example of how you can use the catch-all route feature:
```html
getStaticPaths() {
const allPosts = getAllPosts();
return allPosts.map(post => ({
params: { slug: post.slug }
}));
}
```
In this code, the catch-all route feature is used to make the slug parameter optional, so that the route without the parameter is also matched.
By using dynamic route generation with getStaticPaths and getStaticProps, you can create a seamless user experience and improve the performance of your Next.js application.
Discover more: Searchparams Nextjs
Sources
- https://welearncode.com/beginners-guide-nextjs/
- https://www.freecodecamp.org/news/how-to-setup-dynamic-routing-in-nextjs/
- https://www.dhiwise.com/post/next-js-dynamic-routing-how-to-handle-content-in-next-js
- https://www.storyblok.com/tp/render-storyblok-stories-dynamically-in-next-js
- https://nextjs.org/docs/pages/api-reference/functions/get-static-paths
Featured Images: pexels.com