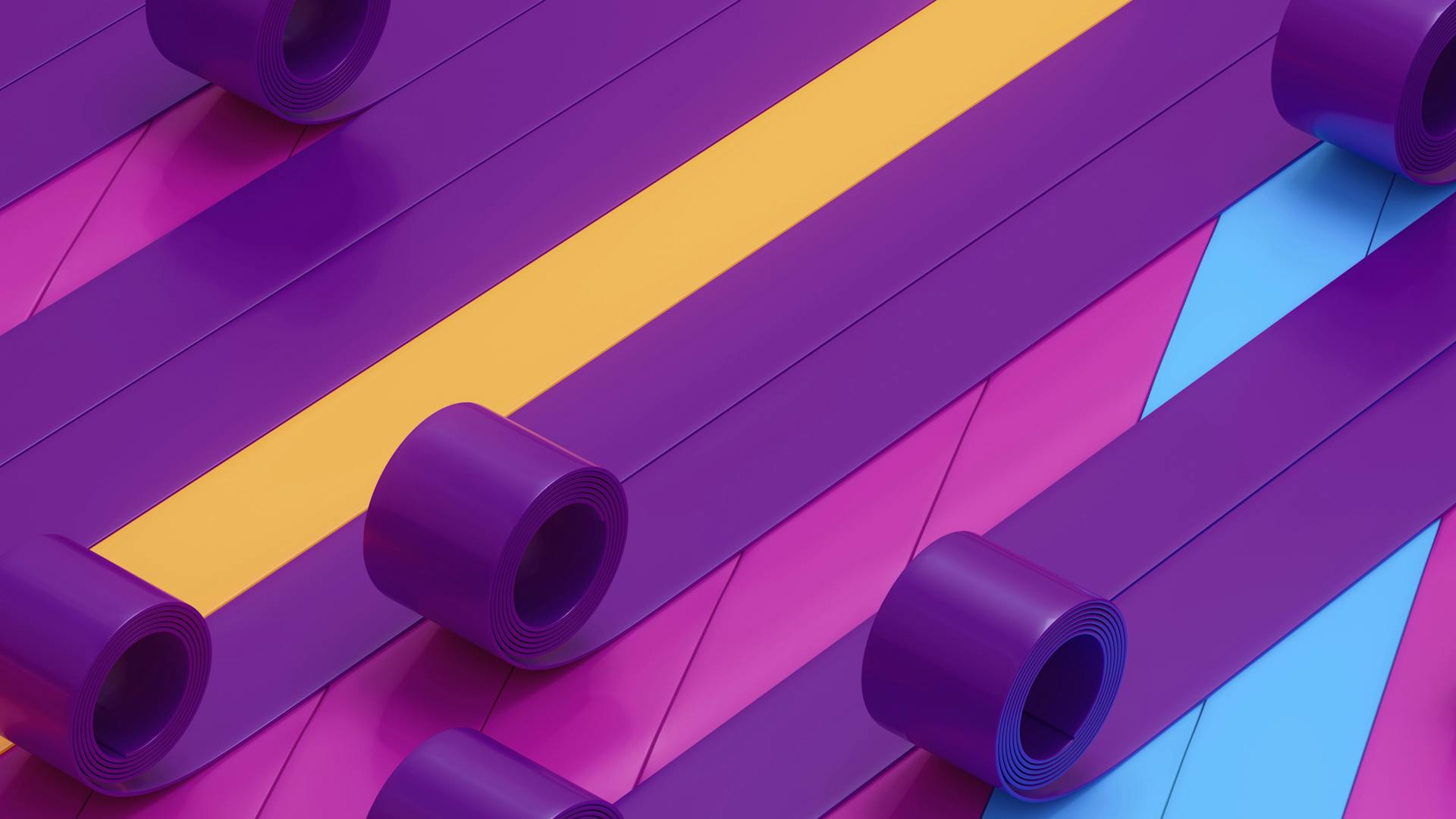
Lazy loading in Next.js can be a game-changer for performance, and we're going to dive into how to implement a preloader using Suspense and dynamic import.
By leveraging Suspense, we can create a seamless user experience by loading components on demand. This is particularly useful for large applications with many routes.
In Next.js, dynamic import allows us to import components only when they're needed, which can significantly reduce the initial load time. This is a key component of lazy loading.
With Suspense and dynamic import, we can create a preloader that loads components in the background while the user waits. This makes for a much more engaging user experience.
You might enjoy: Next Js Swc
Lazy Loading Techniques
Lazy loading techniques in Next.js are used to reduce the amount of JavaScript needed by a route, improving the initial load performance of the application.
There are two ways to implement lazy loading techniques in Next.js: using dynamic imports with the next/dynamic package, and using a combination of React.lazy() and Suspense.
Intriguing read: Router Prefetch Next Js
Lazy loading fetches resources asynchronously, allowing us to delay the loading of client components and imported libraries until they are needed.
Using dynamic imports with next/dynamic package is one way to implement lazy loading in Next.js. This approach works by decreasing the amount of JavaScript required for a route.
Here are the two methods of implementing lazy loading in Next.js:
- Using dynamic imports with the next/dynamic package.
- Using a combination of React.lazy() and Suspense.
Lazy loading applies to client components, not server components. If you dynamically import a server component, only its child client components will be lazy-loaded.
Dynamic Import and Next.js
In Next.js, you can use dynamic import to load components on demand. Dynamic import uses the functionalities of both React.lazy() and Suspense, making it a preferable choice for lazy loading in Next.js.
You can create a folder called components under the app/ directory and a React component called LazyComp.jsx with the following code.
Dynamic import works similarly in the app and page directories, allowing for incremental migration. This means you can start using dynamic import in some parts of your app and gradually migrate the rest of the app to use it.
If this caught your attention, see: Nextjs App Router Tailwind Loading Page
To use dynamic import, you can create a client component called DynamicLoad using the "use client" directive and import the useState hook for managing a toggle state, and the dynamic function from the next/dynamic for the lazy loading of the component.
The dynamic function accepts a function as an argument that returns the imported component. You can also add a custom loading message by passing an optional configuration object as an argument to the function.
Here are the steps to implement dynamic import in Next.js:
- Create a folder called components under the app/ directory
- Create a React component called LazyComp.jsx with the following code
- Create a client component called DynamicLoad using the "use client" directive
- Import the useState hook and the dynamic function from the next/dynamic
- Use the dynamic function to load the LazyComp component on demand
By following these steps, you can implement dynamic import in Next.js and lazy load your components on demand.
Optimizing Loading
Optimizing loading components is crucial for a seamless user experience. It can negatively impact performance if not implemented correctly.
To optimize loading components, we can break our application into smaller chunks that can be loaded on demand, a technique known as lazy loading. This can be achieved using the Next.js dynamic function.
Consider reading: Nextjs App Loading Page Not Working
Lazy loading can be implemented in two ways: using dynamic imports with the next/dynamic package or using a combination of React.lazy() and Suspense. Both methods can help reduce the amount of JavaScript needed by a route, making the initial load performance faster.
Using lazy loading, we can defer the load of client components and imported libraries until they are needed. This can be seen in action with the example of loading Tom's story, where the component is loaded only when needed, improving the overall user experience.
Here are some key benefits of lazy loading:
React and Next.js
React Suspense is a powerful tool for handling loading states in Next.js applications. It can be used to suspend components until their data is ready, but it can block the entire UI.
Next.js supports server-side rendering, which can cause UI loading delays. To address this, you can use Suspense to load the UI. In fact, the Next.js team has worked to keep support for React Suspense up-to-date and efficient.
If this caught your attention, see: Nextjs Loading Page Ssr
Some key improvements to using Suspense with Next.js include:
- Use of React Server Components with Suspense
- Partial pre-rendering
- Refactored streaming logic
Concurrent rendering, introduced in React 18, allows React to work on multiple tasks simultaneously. This can improve the performance and responsiveness of React applications, and it can also help Suspense manage loading states more effectively.
Next.js Image Best Practices
To get the most out of Next.js image optimization, ensure you import the Image component from 'next/image'.
Optimize your image formats by using modern formats like WebP and AVIF that are optimized for the web.
Set static width and height props to reserve space before loading, and consider the aspect ratio of your images.
Images should load lazily as the user scrolls, rather than all images loading at once.
You can leverage the default loader or configure a custom one for resizing and optimization, and point to optimized image files or use relative URL paths with configured loader.
Monitor core web vitals like LCP related to images loading correctly, and display placeholders while images are loading to prevent layout shift.
Readers also liked: Next Js Getstaticpaths
Here are some key considerations for Next.js image optimization:
Next.js and React
Next.js and React are a match made in heaven. Next.js supports server-side rendering, so your UI will take some time to load. This is where React Suspense comes in, allowing you to load the UI and provide a better user experience.
The Next.js team has worked to keep support for React Suspense up-to-date and efficient, as well as to enhance the DX of using Suspense with Next. Some of the key improvements include the use of React Server Components with Suspense, partial pre-rendering, and refactored streaming logic.
You can implement Suspense in Next.js by creating a loading.js file in the route directory. This file defines a functional component that can be exported as the default.
Concurrent rendering is a key feature introduced in React 18 that can improve the performance and responsiveness of React applications. With concurrent rendering, React can selectively suspend parts of the component tree, keeping the rest of the UI interactive.
Here are some benefits of using React Suspense with Next.js:
- Improved performance and responsiveness
- Enhanced user experience with loading UI
- Better support for concurrent rendering
Memoization
Memoization is a powerful technique that can significantly improve the performance of your React and Next.js applications.
By memoizing expensive computations, you can cache their results and prevent unnecessary re-renders.
This is especially useful during suspenseful loading, as it can help reduce the number of unnecessary computations and improve the overall user experience.
Memoization can be particularly beneficial in complex applications where computations are involved, such as in data processing or calculations.
By using memoization, you can ensure that your application remains fast and responsive, even when dealing with large amounts of data.
Create a File
To create a file for lazy loading in Next.js, start by creating a loading.js file in the root directory. This file contains the code that will be used to display a loading component when data is being fetched.
The loading.js file is used by Next.js to understand when data is loading, and it will display the loading component accordingly. You can add different loading components separately in every route with the addition of the loading.js file.
This approach is useful for displaying a loading animation or message while data is being fetched, improving the user experience.
In the root directory, create the loading.js file with the code that will be used to display a loading component.
React Suspense and Concurrent Rendering
React Suspense and Concurrent Rendering are key features that work together to improve the performance and responsiveness of React applications.
Concurrent rendering is a feature introduced in React 18 that allows React to work on multiple tasks simultaneously and prioritize important updates. This means React can pause or interrupt low-priority rendering processes to handle urgent tasks, like responding to user input.
With concurrent rendering, Suspense can better manage loading states by selectively suspending parts of the component tree, keeping the rest of the UI interactive. This is a significant improvement over the previous behavior, where Suspense would block the entire UI.
To get the most out of React Suspense and concurrent rendering, plan the way you nest Suspense and error boundaries carefully. This will help you avoid unpredictable behavior and make it easier to trace errors or manage loading states.
Here are some key takeaways to keep in mind:
- Suspense should have a fallback, like a loading spinner, to provide a clear loading state.
- Error boundaries should be placed where they can catch errors effectively.
Image Optimization Strategies
Image optimization is crucial for a smooth user experience, especially when using lazy loading. You should import the Image Component from 'next/image' to utilize it in your app.
Additional reading: Nextjs Image Loader
Optimizing image formats is a no-brainer. Use modern formats like WebP and AVIF that are optimized for the web. These formats can significantly reduce image file sizes.
Specify width and height props to reserve space before loading images. This ensures a seamless user experience and prevents layout shifts.
Lazy loading images is a must. Images load lazily as the user scrolls instead of all images loading at once. This approach can improve page load times and user engagement.
To optimize images, consider using a high-performance content delivery network (CDN). This can reduce latency and improve image loading times.
You should also compress and use appropriate resolutions on original image assets. This can significantly reduce file sizes and improve image loading times.
Measuring performance is essential to ensure that images are loading correctly. Monitor core web vitals like LCP (largest contentful paint) to identify areas for improvement.
See what others are reading: Why Use Nextjs
Dynamic Loader Component
To create a dynamic loader component in Next.js, you can use the `next/dynamic` package. This package uses the functionalities of both `React.lazy()` and `Suspense` to lazy load components.
You can import components that were exported with default export using the `next/dynamic` package. For example, you can create a component called `LazyComp.jsx` with the following code:
In this example, we have created a simple React component called `LazyComp`. We have also default exported the component to import it elsewhere.
Alternatively, you can import named export components using the `next/dynamic` package. For example, you can create a component called `NamedLazyComp.jsx` with the following code:
In this example, everything's the same as in the previous `LazyComp`. But, Instead of the default export we have used the named export.
To use the `next/dynamic` package, you can create a component called `DynamicLoad.jsx` with the following code:
This code imports the `useState` hook for managing a toggle state, and the `dynamic` function from the `next/dynamic` package for the lazy loading of the component. The `dynamic` function returns the lazily loaded component instance, which is `DynamicLoad` (could be any name). We also added a custom loading message by passing an optional configuration object as an argument to the function.
You can also use the `React.lazy()` and `Suspense` combination to lazy load components. However, the `next/dynamic` package is more preferable as it works similarly in the app and page directories to allow for incremental migration.
Here's a summary of the steps to create a dynamic loader component:
- Create a component with the `next/dynamic` package
- Import the `useState` hook for managing a toggle state
- Import the `dynamic` function from the `next/dynamic` package
- Use the `dynamic` function to lazy load the component
- Add a custom loading message by passing an optional configuration object as an argument to the function
Table of Contents
In this article, we'll explore the concept of lazy loading in Next.js, a technique used to optimize page loading times.
Lazy Loading is a technique used to optimize page loading times by loading components only when they are needed.
We'll also discuss various techniques for implementing lazy loading in Next.js, including dynamic import and next/dynamic.
There are several techniques for implementing lazy loading in Next.js. Here are some of them:
- Lazy Loading with dynamic import and next/dynamic
- Lazy Loading with React.lazy() and Suspense
- Lazy Loading Your Server Components
Each of these techniques has its own advantages and disadvantages, and we'll explore them in more detail throughout this article.
Memoization
Memoization is a powerful technique that can significantly improve the performance of your Next.js application. By caching the results of expensive computations, you can prevent unnecessary re-renders and improve the performance of components during suspenseful loading.
Memoization works by storing the results of expensive computations, so if the same computation is needed again, it can be retrieved from the cache instead of being recalculated. This can greatly reduce the load on your application and improve overall performance.
In Next.js, memoization can be particularly useful during suspenseful loading, where components are being re-rendered multiple times while data is being fetched. By caching the results of these computations, you can reduce the number of re-renders and improve the overall loading experience for your users.
Curious to learn more? Check out: Nextjs Performance
Sources
- https://staticmania.com/blog/implementing-lazy-loading-for-components-in-nextjs
- https://blog.logrocket.com/using-next-js-react-suspense-create-loading-component/
- https://blogs.purecode.ai/blogs/nextjs-image/
- https://meje.dev/blog/building-a-nextjs-preloader-the-right-way
- https://www.freecodecamp.org/news/next-js-performance-optimization/
Featured Images: pexels.com