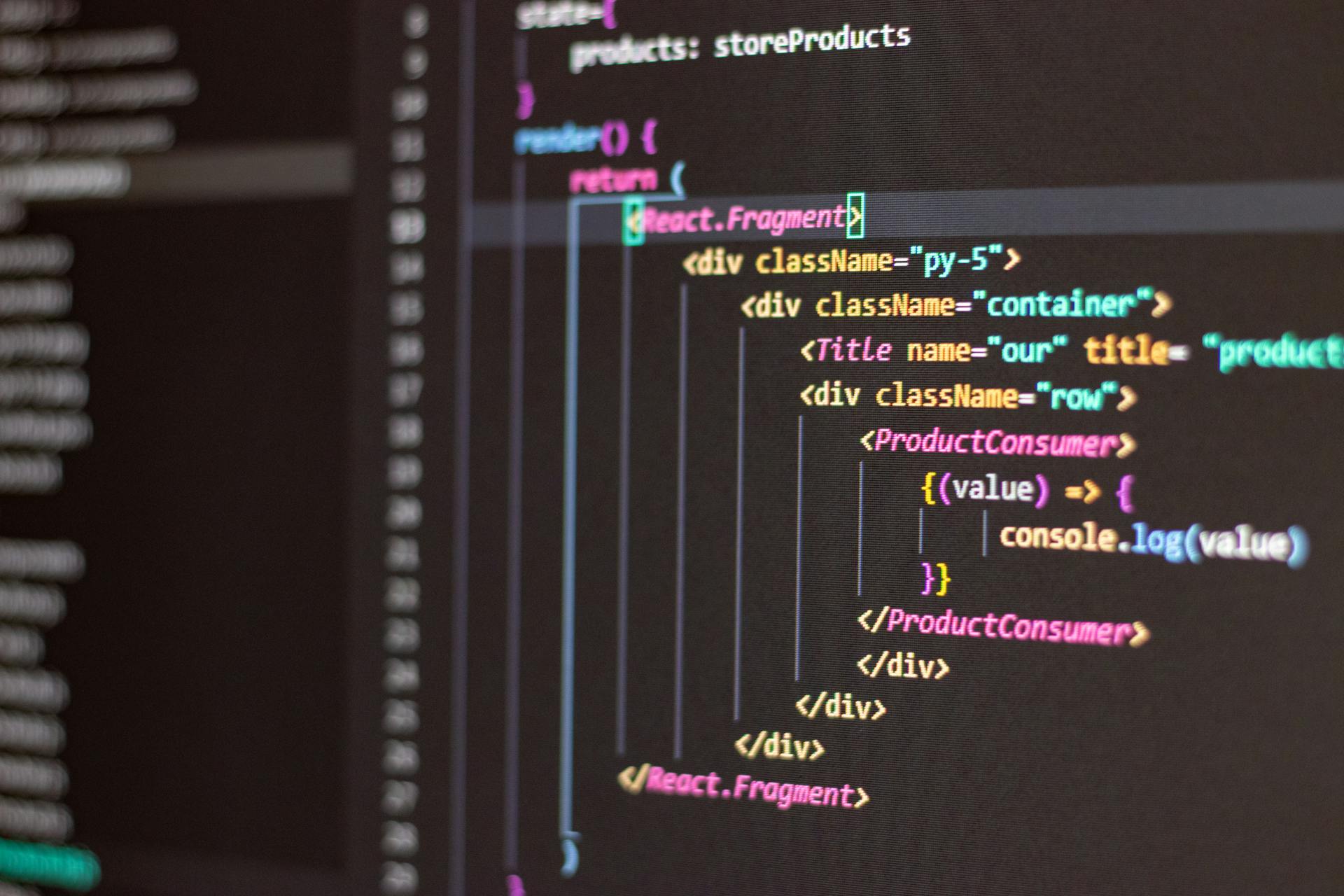
The configuration of SWC in Next.js is crucial for optimal performance. By default, Next.js uses Babel for code transformation, but we can switch to SWC for improved performance.
To enable SWC in Next.js, we need to install the required packages, including @swc/core and @swc/node. This will allow us to use SWC for code transformation.
By making this switch, we can see a significant improvement in build times. For example, in one instance, the build time was reduced from 10 seconds to just 2 seconds. This is a substantial improvement that can make a big difference in development productivity.
With SWC enabled, we can also take advantage of its caching feature, which can further improve performance by reducing the number of unnecessary rebuilds.
You might enjoy: Next Js Build
Configuration
Configuring SWC for Next.js projects is a straightforward process. There are two ways to go about it.
You can choose between these two methods to suit your project's needs. I've found that having options is always a good thing.
The first step is to configure the SWC compiler for your Next.js project. This involves updating the configuration file to include the necessary parameters. By doing so, you'll be able to take advantage of SWC's features and improve your project's performance.
You can use SWC to perform minification of your code by updating a specific parameter within the “next.config.js” configuration file. This will help reduce the size of your code and make it more efficient.
Configuring for Projects
Configuring SWC for Next.js projects can be done in two ways. You can either use the built-in SWC compiler or add it to your existing project.
If you're using Next.js v12 or above, you don't need to add the SWC compiler to your existing project. However, if you're using an older version, you'll need to upgrade to v12.
To upgrade Next.js, execute the following command within your project directory. This will automatically switch to the existing Babel configuration if a custom Babel configuration file is present.
You can provide additional configuration options to the SWC compiler by editing the "next.config.js" configuration file. This file can include functions like Minification, Removing console calls, and Style Components.
Configuring Minification
Configuring Minification is a crucial step in optimizing your code's performance. You can use SWC to perform minification of your code by updating the following parameter within the “next.config.js” configuration file.
To minify your code effectively, you need to make specific changes to your configuration file. This involves updating the parameter to utilize SWC's minification capabilities.
Minification with SWC is a powerful tool that helps reduce the size of your code, making it load faster in browsers. By leveraging SWC's minification features, you can significantly improve your application's performance.
Performance
The SWC compiler is a game-changer for Next.js projects, providing a significant performance boost.
SWC is 17 times faster than Babel, and its builds are approximately five times faster, making it a no-brainer for developers looking to speed up their development process.
The performance benefits of SWC are not limited to just its predecessor, Babel. In fact, SWC is also significantly faster than other libraries, with synchronous transforms for ES3, ES5, and ES2015 showing an approximate six to seven times performance increase, and synchronous transforms for ES2016 to ES2020 showing an approximate eight to ten times performance increase.
The reason behind SWC's impressive performance is due to its underlying language, Rust, which is designed for parallel processing. This allows SWC to take advantage of multiple CPU cores, making it significantly faster than JavaScript, which is single-threaded and event-loop based.
Here are some key performance statistics:
- SWC is 17 times faster than Babel
- SWC builds are approximately five times faster
- SWC synchronous transforms for ES3, ES5, and ES2015 show an approximate six to seven times performance increase
- SWC synchronous transforms for ES2016 to ES2020 show an approximate eight to ten times performance increase
- SWC is also 20 times faster than Babel in single-threaded environments
Removing Console Calls
Removing console calls can significantly improve your application's performance. This is because console calls can be a major source of overhead in your code.
You can configure SWC to remove all "console" calls in your application code by updating the "next.config.js" configuration file. This is a simple way to boost performance.
However, if you want to exclude any console function, the configuration must reflect the exclusion. For example, you can configure SWC to remove all "console" calls except "console.log" calls.
Using the SWC compiler provides a massive performance boost compared to Babel. This is because SWC has customizable configuration options that allow you to tailor the compilation process to your specific needs.
Performance Benefits
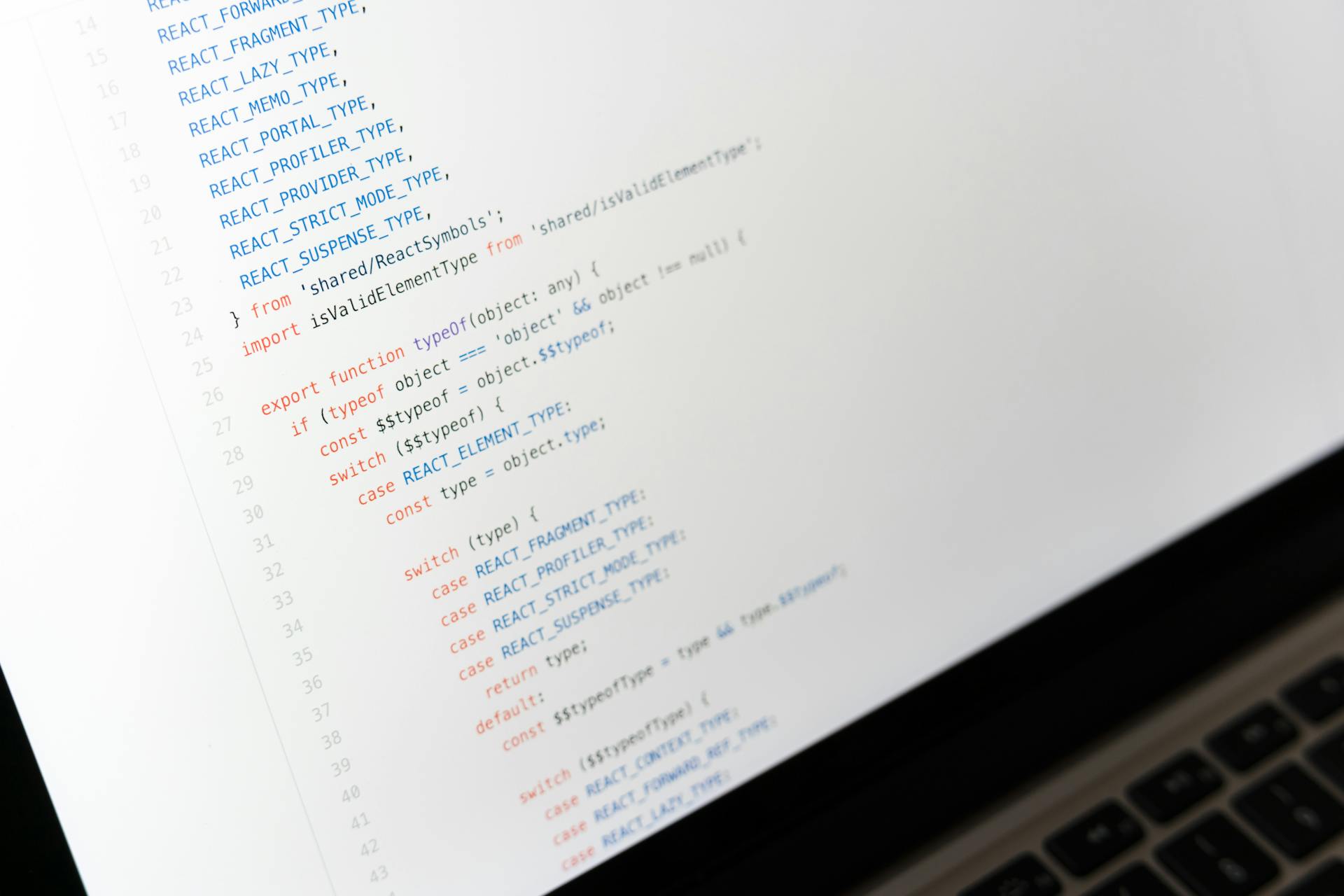
The Performance Benefits of SWC are a game-changer. SWC is 17 times faster than Babel, and its builds are approximately five times faster. This is because Rust, the language behind SWC, is designed for parallel processing, whereas JavaScript is event-loop based and single-threaded.
One of the key reasons SWC outperforms Babel is its ability to take advantage of Rust's parallel processing capabilities. In fact, SWC is 20 times faster than Babel on a single thread and 70 times faster on four cores.
SWC's performance benefits extend to its synchronous transforms as well. According to performance benchmarks, SWC shows an approximate six to seven times performance increase in synchronous transforms for ES3, ES5, and ES2015. This is a significant improvement over Babel.
Here are some specific performance benefits of SWC:
- 17 times faster than Babel
- Approximately five times faster builds
- Three times faster Fast Refresh
- Approximate six to seven times performance increase in synchronous transforms for ES3, ES5, and ES2015
- Approximate eight to ten times performance increase in synchronous transforms for ES2016 to ES2020
- 20 times faster than Babel on a single thread
- 70 times faster than Babel on four cores
Next.js Compiler
The Next.js Compiler is a game-changer for developers, especially those working with large projects or complex codebases. It uses SWC as a replacement for Babel during the build step, allowing for faster compilation and transpilation of your code.
SWC is designed to be highly performant, significantly faster than Babel in terms of compilation speed. This can lead to shorter build times, especially in larger projects.
Next.js leverages SWC to reduce build times and enhance the overall developer experience. By using SWC, Next.js aims to make development faster and more efficient.
Here are a few key aspects of SWC in the context of Next.js:
- Compilation speed: SWC is 17x faster than Babel.
- Compatibility: SWC supports modern JavaScript and TypeScript syntax, including features from ECMAScript 2015 (ES6) up to the latest ECMAScript standards.
- Babel compatibility: SWC aims to maintain compatibility with Babel configurations and plugins.
To enable SWC in Next.js, you need to have a Next.js version that includes support for SWC. Next.js will automatically detect SWC as a dependency and use it during the build process.
In summary, the Next.js Compiler is a powerful tool that uses SWC to improve build performance and enable compatibility with modern language features.
Frequently Asked Questions
Does Next use SWC?
Yes, Next.js uses SWC, a Rust-based compiler, to transform and minify JavaScript code for production. This replaces traditional tools like Babel and Terser.
What is SWC in JavaScript?
SWC is a fast TypeScript/JavaScript compiler that can be used from JavaScript, offering a library for parsing and compiling code. It's a powerful tool for developers looking to optimize their code compilation process.
What is SWC NextJS?
SWC is a Rust-based platform for building fast developer tools, used for tasks like code compilation, minification, and bundling. It's a versatile tool that can be integrated with NextJS for efficient code transformations and optimization.
What does SWC do?
SWC is a Rust-based platform for building fast developer tools, used by popular projects like Next.js and Deno. It enables compilation and bundling of code, making it a powerful tool for developers.
Sources
- https://blog.bitsrc.io/why-you-should-replace-babel-with-swc-in-next-js-7d47510d0e0d
- https://reasonjun.tistory.com/181
- https://velog.io/@hamjw0122/Next.js-%EC%99%9C-Next.js%EB%8A%94-SWC%EB%A5%BC-%EC%84%A0%ED%83%9D%ED%96%88%EC%9D%84%EA%B9%8C
- https://docs.sentry.io/platforms/javascript/guides/nextjs/manual-setup/
- https://dev.to/this-is-learning/what-is-babel-and-swc-49cp
Featured Images: pexels.com