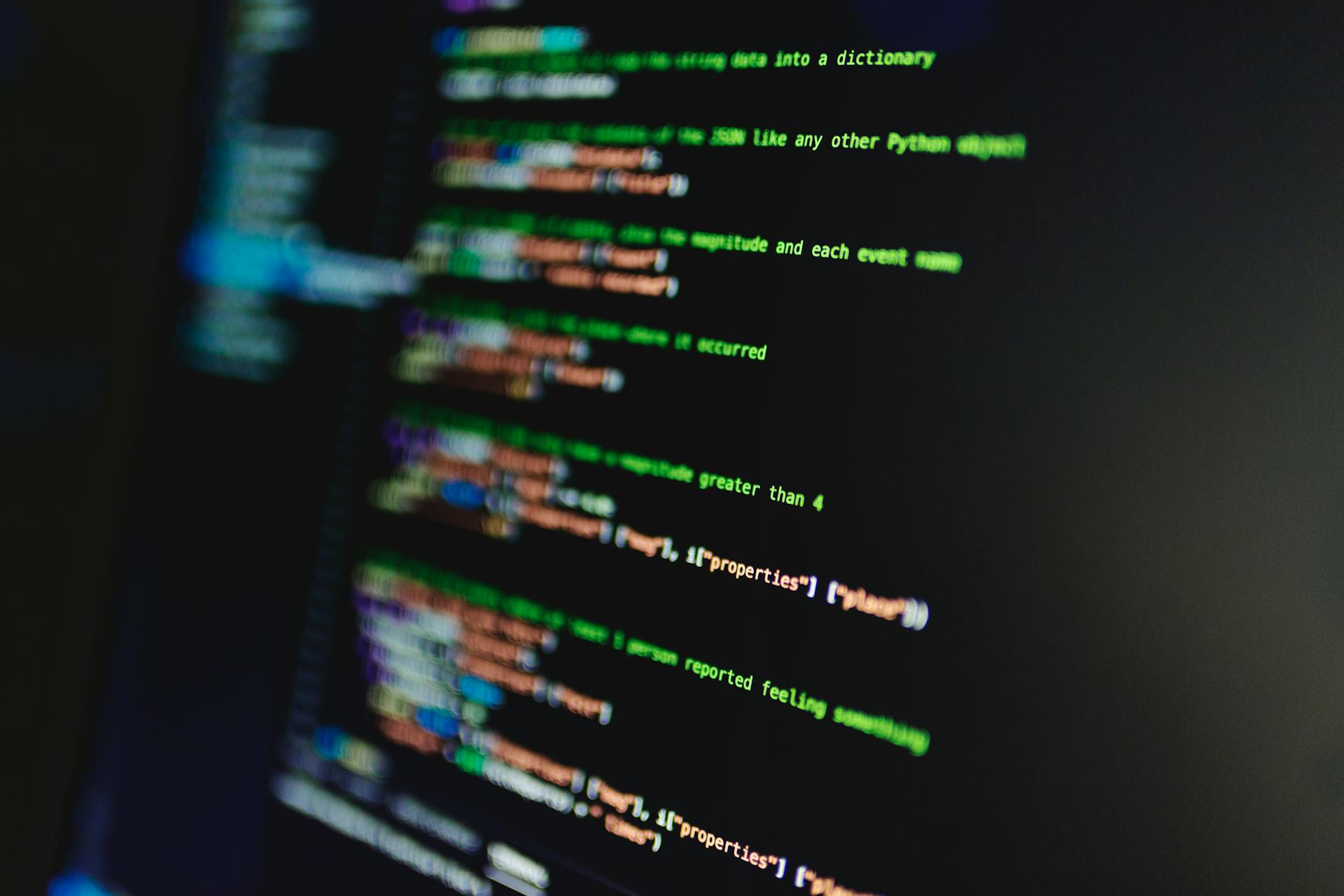
Nextjs provides a built-in support for search parameters and routing, making it easy to create dynamic and interactive routes.
You can access search parameters using the `useRouter` hook and the `query` object.
For example, if you have a route like `/users?name=John&age=30`, you can access the `name` and `age` parameters using `router.query.name` and `router.query.age`.
In Nextjs, you can also use the `useParams` hook to access route parameters.
A fresh viewpoint: Nextjs App Router Query Params
Next.js Routing
Dynamic routes in Next.js allow you to create pages that can match a variety of URL structures. This flexibility is particularly useful when you want to create pages that adapt to different content based on the URL parameters provided.
To create dynamic routes, you place square brackets around a file or folder name within the ‘pages' directory. For example, a file named [id].js would match any route like /posts/1 or /posts/abc.
You can use the router.push method to navigate to a new page and add a new entry to the browser's history stack. This means that when the user clicks the back button, they will return to the previous page.
In Next.js, the router.push method is used to update the current URL with new query parameters, while preserving existing ones. This is demonstrated in an example where a handleSearch function is called, which uses router.push to update the URL with the new search term.
Check this out: Router Prefetch Next Js
Server-Side Rendering
Server-Side Rendering is a powerful feature in Next.js that allows you to render pages on the server. This enables you to fetch data based on query parameters during server-side rendering.
You can use the getServerSideProps function to achieve this, which runs on the server for each request and receives the context object. The query object is extracted from the context, allowing you to log the query parameters and fetch data accordingly.
Server-side filtering is a key feature of modern enterprise web applications, and Next.js makes it possible with the help of nuqs. You can implement a basic server-side string filter based on the q search parameter using this approach.
However, you may face an issue on the initial load of your app, where the default value of a parameter is undefined. To circumvent this, you can use a nuqs utility that helps you parse the search params with their default values automatically loaded. This will provide type-safe access to the parameters through all its properties.
For more insights, see: Router Query Params Nextjs 14
Static Generation
Static Generation is a powerful feature in Next.js that allows you to pre-render pages at build time. This is done using the getStaticProps and getStaticPaths functions.
getStaticPaths is used to specify dynamic routes that should be pre-rendered, including query parameters. In getStaticPaths, we define the paths that include the query parameters we want to pre-render.
We access these parameters through the params object provided by the context in getStaticProps. This allows us to fetch data at build time and make it available to the page.
Check this out: Next Js Getstaticpaths
Re-Running Server Components
Re-running server components based on search params updates is a crucial aspect of static generation. We can check if this is happening by adding a simple console.log on our Page component.
For now, URL updates do not rerun our server components, which can be a problem. We need to fix this issue.
To fix this, we set the shallow option to false in our useQueryState hook. This will ensure that our server components are re-run when the search params update.
Static Generation with GetStaticProps
Static generation with getStaticProps is a powerful way to pre-render pages at build time. This approach allows you to fetch data at build time, making it ideal for pages that don't need to be updated frequently.
GetStaticProps is used to fetch data at build time, which means you can pre-render pages with dynamic content. In Next.js, getStaticProps is a function that receives the context and returns an object with the pre-rendered data.
To use getStaticProps, you need to define the paths that include the query parameters you want to pre-render in getStaticPaths. This is a crucial step in setting up static generation with getStaticProps.
By using getStaticProps, you can improve the performance of your application by reducing the number of server requests. This is especially important for pages that require a lot of data to be fetched on every request.
Managing Search Params
Managing search params in Next.js is a breeze, thanks to the useQueryState hook. This hook lets you easily manage a specific search param state by passing your parameter name, making it similar to using the useState hook.
For more insights, see: Google Search Console Nextjs
By default, this state will be parsed as a string, and you can see that updating the search bar updates the appropriate search param on the URL. However, there's one problem with our code here: nuqs doesn't re-render server components by default, which means we won't see any updates if we do filtering on the server.
To access query parameters in URLs, you can use the useRouter hook from Next.js to access the router object, which contains the query object. This query object holds the query parameters from the current URL, allowing you to log their values and potentially use them to fetch data or render specific components on the page.
There are two ways to get access to URL parameters: the searchParams page prop in the root of a route returns an object with the parameters, and the useSearchParams hook returns a readonly URLSearchParams interface.
Here are the possible values you can expect from searchParams:
You'll need to validate searchParams to ensure it contains the expected values. You can create a type to represent searchParams and use it to validate the incoming search parameters.
To programmatically navigate between pages and manipulate query parameters, you can use the Next router methods router.push and router.replace. These methods allow you to update the URL and navigate to a new page without a full page refresh, providing a smoother user experience.
Parsers are a set of primitive functions that help you implement type-safe read/write access to your search parameters. They can convert traditional string-only parameters to other primitives like integers, floats, booleans, string and number literals, typescript enums, dates, arrays, etc. You can use parsers to avoid null-checking hell and history behavior.
Dynamic Server-Side Filtering
Dynamic Server-Side Filtering is a key feature of modern enterprise web applications, and thanks to nuqs, we'll always have access to the latest search params on our server components.
With nuqs, we can implement server-side filtering based on the q search parameter, which is a basic string filter. This approach, however, faces an issue on the initial load of our app, since the defaultValue used in the client-side hooks are not enforced on our server code.
To circumvent this, we can use another nuqs utility that helps us parse the search params with their default values automatically loaded, even if they don't appear in the current search params URL. This utility transforms the incoming params without applying any validation.
You might like: Nextjs Params
By using this cache, we'll find that our parsedParams variable now holds all our parameters with their respective default values, providing type-safe access through all its properties. This is particularly useful when we need to retrieve or display data based on user input or create dynamic routes that respond to changes in the query string.
URL Parameters and Navigation
URL parameters are used to sort, filter, or search data on a page, and they can be accessed within a Next.js page component using the useRouter hook.
In Next.js, you can programmatically navigate between pages and manipulate query parameters using the Next router methods router.push and router.replace. These methods allow you to update the URL and navigate to a new page without a full page refresh.
Query parameters can be accessed within a Next.js page component using the useRouter hook, which returns the router object containing the query object. This query object holds the query parameters from the current URL.
You might enjoy: Nextjs Swc
To create a dynamic page that responds to query parameters, you can define a dynamic route using the file naming convention mentioned above. Within the page component, you can access the dynamic segments of the URL as query parameters using the useRouter hook or the context object provided to getServerSideProps or getStaticProps.
Here are some strategies to preserve query parameters during navigation:
- Passing Query Parameters Manually: When using router.push or router.replace, you can manually include the query parameters you want to preserve in the new URL.
- Using a Global State Management Library: Libraries like Redux or Zustand can help manage the application state globally, including the state represented by query parameters.
- Storing in Local Storage or Session Storage: For more persistent state management across page reloads, you can store query parameters in local storage or session storage and retrieve them when needed.
- Custom Hooks: Creating custom hooks to manage query parameters can abstract the logic of preserving parameters during navigation, making your components cleaner and more reusable.
The useRouter hook is a client-side hook that allows you to access the router object within your page components. This router object contains the query object, which holds the query parameters of the current URL.
You can use the router.push method to navigate to a new page and add a new entry to the browser's history stack. This means that when the user clicks the back button, they will return to the previous page.
Parsing and Handling
Parsers are a set of primitive functions that help you implement type-safe read/write access to your search parameters.
You can use parsers to convert traditional string-only parameters to other primitives like integers, floats, booleans, string and number literals, and typescript enums.
Parsers are also configurable, which can help you avoid null-checking hell and history behavior.
The `parseAsString` function can be used with a default value to avoid doing null checks on the JSX directly.
For our application, setting a default value with `parseAsString` helps us avoid the hassle of null checks on the JSX.
Adding Search Functionality
You can easily manage a specific search param state using the useQueryState hook, which works similarly to a useState hook by passing your parameter name.
This state will be parsed as a string by default, but you can learn more about parsers later. As you type in the search bar, you can see that the appropriate search param on the URL is updating in real-time.
However, there's a problem with our code here: nuqs doesn't re-render server components by default, which means that if you do any sort of filtering on the server, you won't see any updates.
Sources
- https://www.dhiwise.com/post/navigating-nextjs-query-params-a-comprehensive-guide
- https://medium.com/@Jaimayal/how-to-properly-manage-search-params-in-nextjs-app-router-leverage-the-power-of-nuqs-the-right-way-9f7238cff76a
- https://dev.to/peterlidee/using-searchparams-usesearchparams-and-userouter-in-next-13-4623
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://nextjs.org/learn/dashboard-app/adding-search-and-pagination
Featured Images: pexels.com