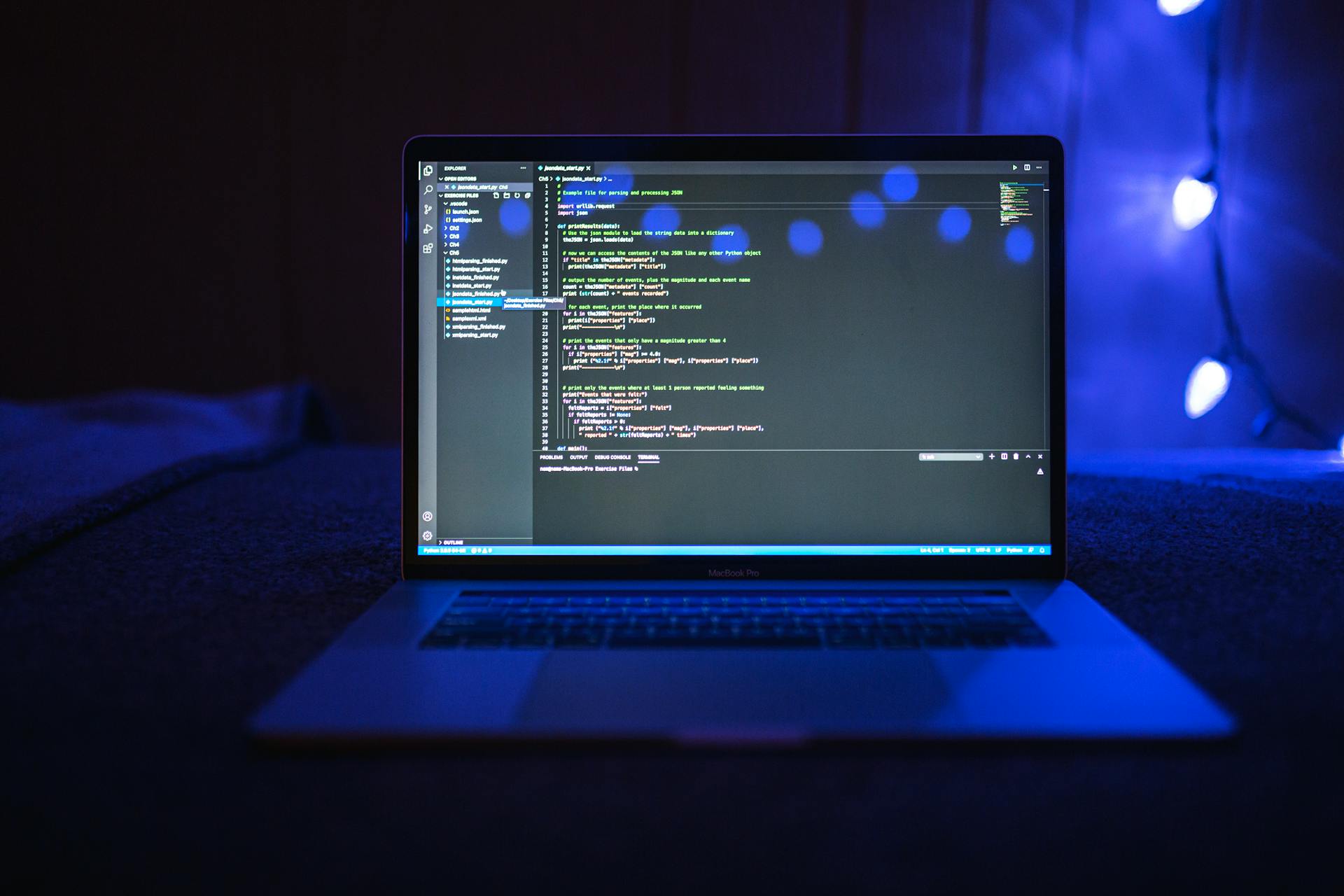
In Next.js App Router, query parameters are used to pass data from one page to another. They are represented as an object in the `useRouter` hook.
To access query parameters, you can use the `query` property of the `useRouter` hook. For example, if you have a page with the following code: `const { query } = useRouter(); console.log(query.name);`, it will log the value of the `name` query parameter.
Query parameters can be accessed directly in the page's props, using the `useRouter` hook.
Explore further: Next Js Userouter
Dynamic Routing
Dynamic routing is a powerful feature in Next.js that allows you to create pages that respond to query parameters.
To create a dynamic page, you first define the dynamic route using the file naming convention mentioned above. This means creating a file in the 'pages' directory that corresponds to the dynamic segment of the URL.
For example, if you create a file named [category].js, it will match routes like /shoes or /accessories, and the dynamic segment will be available as a query parameter under the key category.
You might like: Next Js Pages vs App
You can access the dynamic segments of the URL as query parameters using the useRouter hook or the context object provided to getServerSideProps or getStaticProps.
In the example of an e-commerce application, a URL like /shoes?price=under-100&brand=nike would render the ProductsByCategory component, and within that component, you could fetch and display products that are shoes under $100 from Nike.
To access the dynamic segment and any additional query parameters, you can use the useRouter hook within the page component.
Here's a step-by-step guide to creating a dynamic route:
- Create a file in the ‘pages’ directory that corresponds to the dynamic segment of the URL, for example, [category].js.
- Within this file, use the useRouter hook to access the dynamic segment and any additional query parameters.
Using the Router
You can access the router object within your page components using the useRouter hook, which is part of the Next.js router. This hook allows you to access the query object, which holds the query parameters of the current URL.
The useRouter hook is a client-side hook, meaning it runs on the client-side, not on the server-side. This is useful for accessing query parameters without making an extra server request.
To use the useRouter hook, you import it from the Next.js router library and use it to retrieve the router object. You can then destructure the query object from router to access the query parameters.
For example, if you have a dynamic route like /shoes?price=under-100&brand=nike, you can use the useRouter hook to access the category and any additional query parameters.
You can also use the router.push method to navigate to a new page and add a new entry to the browser's history stack. This means that when the user clicks the back button, they will return to the previous page.
Here are some key router methods:
- useRouter: a client-side hook that allows you to access the router object within your page components.
- router.push: a method used to navigate to a new page and add a new entry to the browser's history stack.
- router.replace: a method used to update the URL and navigate to a new page without a full page refresh.
These methods allow you to update the URL and navigate to a new page without a full page refresh, providing a smoother user experience.
Query Parameters
Query parameters are a crucial part of any web application, and Next.js app router is no exception. They're used to sort, filter, or search data on a page, and can be passed as part of the URL string.
In a URL, query parameters come after the pathname and can be used to pass additional information to the page components. This is particularly useful when you need to retrieve or display data based on user input or when you want to create dynamic routes that respond to changes in the query string.
To preserve query parameters during navigation, you have several strategies at your disposal. You can pass them manually when using router.push or router.replace, or use a global state management library like Redux or Zustand.
Here are some strategies for preserving query parameters during navigation:
These strategies can help you manage query parameters effectively and ensure a seamless user experience in your Next.js app router.
Action
In a Next.js app, query parameters in action are used to fetch data that matches the specified category and color.
Query parameters are key-value pairs that are appended to a URL, such as category=shoes and color=black. These parameters can be used to highlight the active filters on the current page.
To access query parameters in a Next.js page component, you can use the useRouter hook to get the router object, which contains the query object. The query object holds the query parameters from the current URL.
The query object can be used to log the values of the query parameters, such as category and color. This can be useful for debugging or rendering specific components on the page.
Sources
- https://nextjs.org/docs/pages/building-your-application/routing/api-routes
- https://www.dhiwise.com/post/navigating-nextjs-query-params-a-comprehensive-guide
- https://egghead.io/lessons/next-js-access-route-params-from-props-inside-a-next-js-dynamic-app-router-route
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://developer.auth0.com/resources/guides/web-app/nextjs/basic-authentication
Featured Images: pexels.com