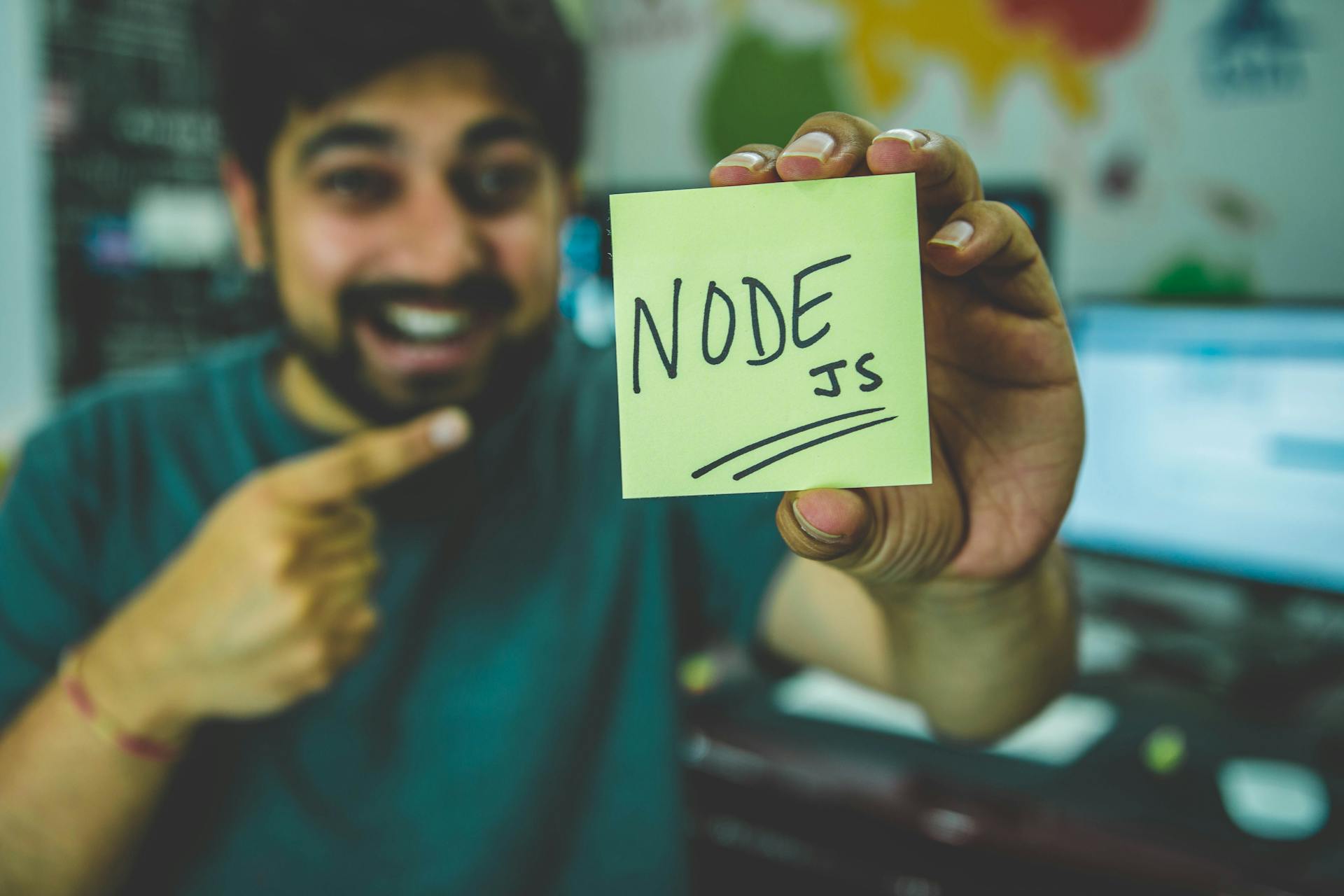
In Next Js, slugs are a crucial part of creating a JAMstack companion, as they provide a unique identifier for each page or post.
Slugs are created automatically by Next Js when you create a new page or post, but you can also customize them if needed.
A slug is a short, descriptive string that represents the URL of a page or post.
For example, if you create a new page with the title "Hello World", Next Js will automatically create a slug for it, which is usually the title in lowercase with hyphens instead of spaces.
In the example of creating a new page, we can see how Next Js automatically generates a slug for us, making it easy to create a JAMstack companion.
Suggestion: Gallary Page Next Js
Dynamic Routes
Dynamic Routes are a powerful feature in Next.js that allow you to create routes with dynamic segments. To access the value of a slug from a path, you use the params prop, which is directly destructured in the function component.
You might like: Nextjs Dynamic
You can then access the slug directly and fetch data based on the dynamic parameter. This eliminates the need for deeply nested routes and reduces redundancy in your code.
For example, if you have a route /printed-books/:book-id, you can access the book-id directly in your component. But what if you want to add more segments to your route? That's where Catch-All Routes come in.
Catch-All Routes use the same bracket syntax as dynamic routes, but with three dots (...) before the slug. This allows you to catch all routes and eliminate the need for deeply nested routes.
Here's an example of how Catch-All Routes work:
As you can see, the slug is returned as an array of query parameters. This makes it easy to access the category and release year in your component.
But what if you want to avoid creating index routes alongside catch-all routes? That's where Optional Catch-All Routes come in. They use the same syntax as Catch-All Routes, but with double square brackets instead.
With Optional Catch-All Routes, the catch-all route is optional and will fallback to the path /printed-books if not available. This makes it easy to avoid creating index routes and still take advantage of the flexibility of catch-all routes.
JAMstack Companion
Next.js is a popular framework for building server-side rendered (SSR) and statically generated websites. It's perfect for the JAMstack architecture.
The JAMstack is a modern web development approach that combines JavaScript, APIs, and Markup to create fast, secure, and scalable websites. It's a great choice for building modern web applications.
Next.js and the JAMstack work well together, making it easier to build fast and secure websites. This combination is perfect for building modern web applications.
The JAMstack architecture is composed of three main components: JavaScript, APIs, and Markup. These components work together to create a fast, secure, and scalable website.
By using Next.js with the JAMstack, developers can build websites that are both fast and secure. This makes it a great choice for building modern web applications.
For another approach, see: Next Js Component
Routing and Rendering
Routing and rendering are complementary concepts in Next.js, and understanding how they work together is crucial for building efficient and scalable applications.
Each page in Next.js is pre-rendered in advance, alongside minimal JavaScript code, through a process called hydration. This process is highly dependent on the form of pre-rendering, which can be either Static Generation or Server-side rendering.
Check this out: Nextjs Rendering
Pre-rendering is closely related to data fetching, which can be done using built-in functions like getStaticProps, getStaticPaths, or getServerSideProps, or client-side data fetching tools like SWR or react-query.
Routing in Next.js is based on a file-system-based router, which uses the concept of pages to navigate between routes. This means that each route is represented by a separate file, typically named after the route path.
Here's a comparison of how dynamic route segments and catch-all routes work:
Catch-all routes use the same bracket syntax as dynamic routes, but with three dots (…) to indicate that it's a catch-all route. This eliminates the need for deeply nested routes and allows for more flexibility in routing.
Dynamic Route Segments
Dynamic Route Segments are a powerful tool in Next.js that allow you to create dynamic routes with ease.
You can access the value of the slug from the path using the params prop, which is directly destructured in the function component.
The params object allows you to access the slug directly and fetch data based on the dynamic parameter. This is especially useful for creating routes that can handle multiple books with their ID.
To avoid redundancy in your directory structure, you can use Catch All Routes, which eliminate the need for deeply nested routes. This is done by prefixing the bracket syntax with three dots, similar to the JavaScript spread syntax.
With Catch All Routes, you can access the category and release year using the same bracket syntax. Two ways to do this are by attaching the metadata to the book info or by returning the "slug" segments as an array of query parameter(s).
Here's an example of how the route /printed-books/[…slug] works:
Keep in mind that the route /printed-books will throw a 404 error unless you provide a fallback index route. However, you can use optional catch-all routes to avoid creating index routes alongside catch-all routes.
Expand your knowledge: Next Js Fetch Data save in Context and Next Route
Frequently Asked Questions
What is the difference between slug and path?
A slug is a unique identifier within a path, helping visitors remember and find content. Think of a slug as a descriptive label within a URL's path.
What is a slug in React?
A slug in React is a human-readable identifier that makes it easy to understand what a resource is, rather than using a less descriptive ID. It's a unique label that helps with readability and clarity in your application.
Sources
- https://egghead.io/lessons/next-js-set-up-dynamic-pages-in-next-js-13-app-directory-and-query-with-page-slug
- https://vercel.com/blog/common-mistakes-with-the-next-js-app-router-and-how-to-fix-them
- https://www.contentful.com/blog/integrate-contentful-next-js-app-router/
- https://staticmania.com/blog/how-to-start-with-nextjs-app-from-scratch
- https://www.smashingmagazine.com/2021/06/client-side-routing-next-js/
Featured Images: pexels.com