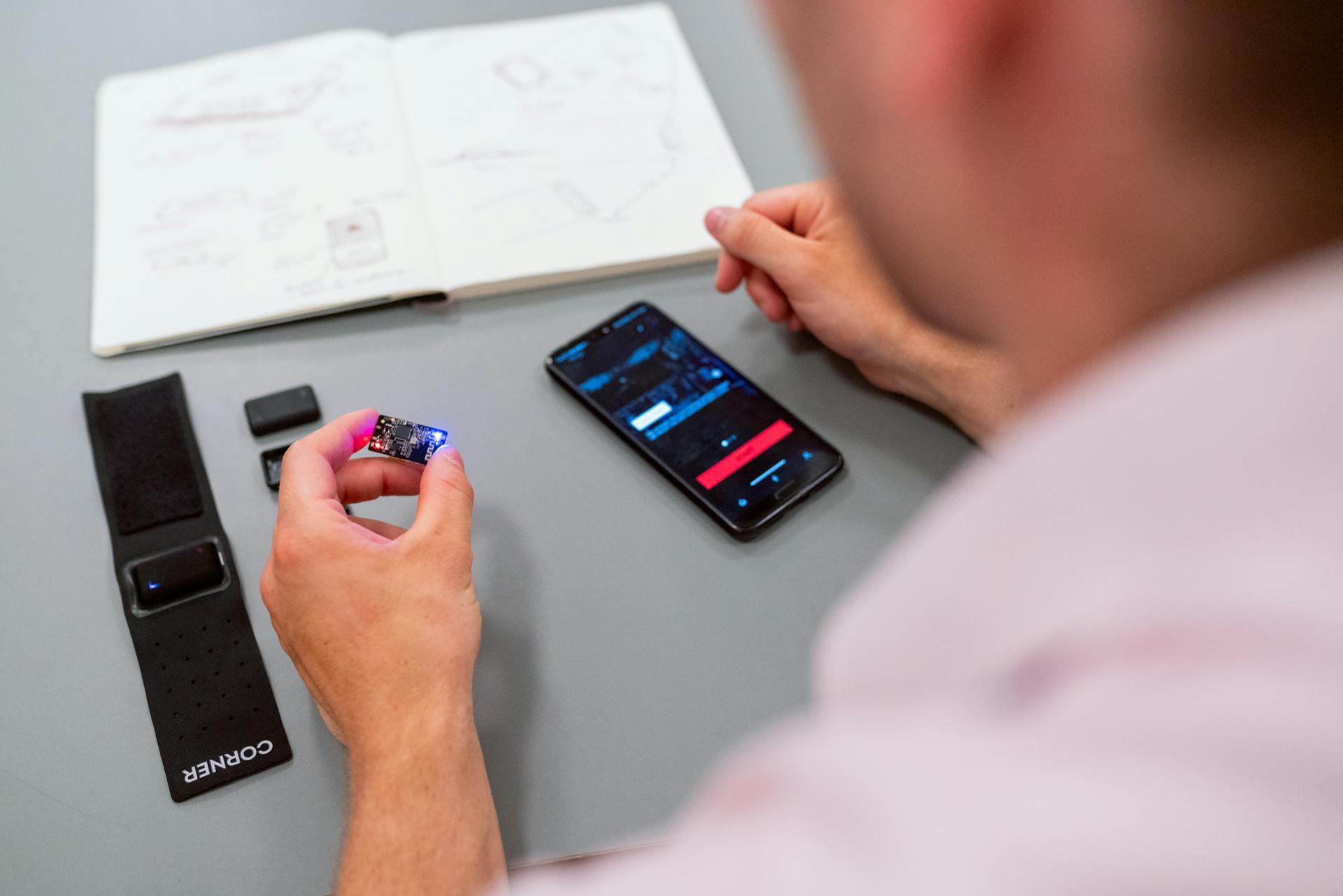
Next.js provides a powerful way to intercept routes with middleware, allowing you to execute code before a page loads. This is particularly useful for authentication, rate limiting, and caching.
Middleware functions can be used to authenticate users before they access a page, by checking if the user is logged in and redirecting them to the login page if necessary.
By using Next.js middleware, developers can also implement rate limiting to prevent abuse of their application. This is achieved by checking the number of requests made from a particular IP address within a certain time frame.
Middleware can be used to cache frequently accessed pages, reducing the load on the server and improving performance.
Understanding Route Interceptors
Route interceptors are middleware functions that allow you to intercept requests before routing occurs. They provide a centralized mechanism for executing logic and handling common tasks.
Unlike regular middleware, interceptors operate specifically on the routing level. This is what makes them so powerful in the Next.js framework.
Route interceptors are useful for executing logic and handling common tasks. You can think of them as a way to wrap your entire application with a layer of functionality.
In Next.js, route interceptors are created as custom middleware. This is the first step in implementing route interceptors in your application.
Route interceptors can modify the behavior of routes. For example, you can redirect a user from one route to another using the res.redirect() function.
Intercepting routes introduces a flexible way to load content from one route without disrupting the current layout. This is especially helpful in scenarios where you want to maintain context while showcasing additional content.
Code Organization
Route interceptors in Next.js are a game-changer for code organization. They enable centralized logic for handling tasks before routing takes place.
By separating the logic into interceptors, you can keep your route handlers clean and focused on their specific responsibilities. This leads to better code organization and maintainability as the interceptor middleware becomes the single entry point for executing common tasks.
Route interceptors help you avoid cluttering your route handlers with unnecessary code, making them easier to read and maintain.
Route Interceptor Middleware
Route Interceptor Middleware is a powerful tool in Next.js that allows you to intercept requests before routing occurs. Unlike regular middleware, interceptors operate specifically on the routing level.
To create a custom middleware, you'll need to follow the process outlined in Section 5, "Creating a Route Interceptor Middleware". This will give you a basic interceptor middleware that you can build upon.
A route interceptor middleware is a centralized mechanism for executing logic and handling common tasks. You can use it to execute code before routing occurs, making it a great place to handle authentication, caching, or other tasks that require access to the request and response objects.
In Next.js, you can create a route interceptor middleware by following the example provided in Section 5. This example will give you a basic understanding of how to set up a route interceptor middleware.
By using route interceptors, you can modify the behavior of routes in Next.js. For example, you can redirect the user to a different route using the `res.redirect()` function, as shown in Section 4, "Modifying Route Behavior".
Implementing Route Interceptors in Next.js
Implementing route interceptors in Next.js is a straightforward process. You start by creating a custom middleware, as shown in Example 3. This middleware serves as the foundation for your route interceptor.
To implement route interceptors, you need to create a middleware that will handle the logic for intercepting routes. This middleware will be used to intercept requests before routing occurs, providing a centralized mechanism for executing logic and handling common tasks.
Once you have your middleware set up, you can implement route interceptors in Next.js by using the middleware to intercept routes. This can be done by creating folders and files to intercept routes and display different content based on navigation, as demonstrated in Example 7.
You can also conditionally intercept routes based on specific criteria, as shown in Example 8. This allows you to apply different logic to different routes, making it easier to manage complex routing scenarios.
By leveraging route interceptors, you can simplify the implementation of common functionalities such as authentication, authorization, error handling, and request validation. This reduces code duplication and ensures that these functionalities are consistently enforced across the application, as mentioned in Example 2.
Route interceptors also provide a flexible way to load content from one route without disrupting the current layout. This is especially helpful in scenarios where you want to maintain context while showcasing additional content, like displaying a photo in a modal without leaving the feed, as described in Example 6.
Soft Navigation
Soft navigation in Next.js intercepting routes is a powerful feature that allows users to interact with content without fully transitioning to a new page. This is especially useful in scenarios where you want to maintain context while showcasing additional content.
For example, if you click on a photo in a feed, the modal opens without fully transitioning to the photo page. This is because soft navigation masks the URL, but the route behaves as an overlay, giving the user access to the same content without navigating away from the feed.
According to the Next.js documentation, soft navigation is a key benefit of intercepting routes. It allows users to navigate between routes without disrupting the current layout.
Here are some key features of soft navigation in Next.js intercepting routes:
By using soft navigation in Next.js intercepting routes, you can create a seamless user experience that allows users to interact with content without fully transitioning to a new page.
Handling Asynchronous Tasks
In Next.js, route interceptors can handle asynchronous tasks with ease.
Route interceptors can perform asynchronous operations using functions like `performAsyncTask()`.
This allows you to execute tasks concurrently, enhancing the overall efficiency of your application.
Once the task is completed, the interceptor can proceed with the routing process, ensuring a seamless user experience.
As demonstrated in an example, route interceptors can handle asynchronous tasks with minimal code changes, making them a convenient solution for complex operations.
Testing and Debugging
Testing and debugging intercepted routes are essential for ensuring their correctness and effectiveness.
Unit testing with mocks and stubs is a great approach to test intercepted routes, as it allows you to isolate the code and test it in isolation.
Integration testing is also crucial, as it helps to ensure that the intercepted routes are working correctly with the rest of the application.
Utilizing debugging tools like logging and breakpoints can be incredibly helpful in identifying and fixing issues with intercepted routes.
Logging can provide valuable insights into what's happening with the intercepted routes, allowing you to identify and fix problems more efficiently.
Breakpoints can also be used to step through the code and see exactly what's happening with the intercepted routes.
Sources
- https://cloudinary.com/blog/image-gallery-next-js-parallel-intercepting-routes
- https://stackoverflow.com/questions/76439415/intercepting-routes-nextjs-not-rendering-modal
- https://blog.stackademic.com/leveraging-route-interceptors-in-next-js-13-4-a-step-by-step-guide-with-code-snippets-f142900aaecd
- https://mediusware.com/blog/details/intercepting-routes-in-nextjs-a-new-routing-paradi
- https://www.chaindesk.ai/ja/tools/youtube-summarizer/next-js-14-tutorial-31-intercepting-routes-nr_kRfTJfKc
Featured Images: pexels.com