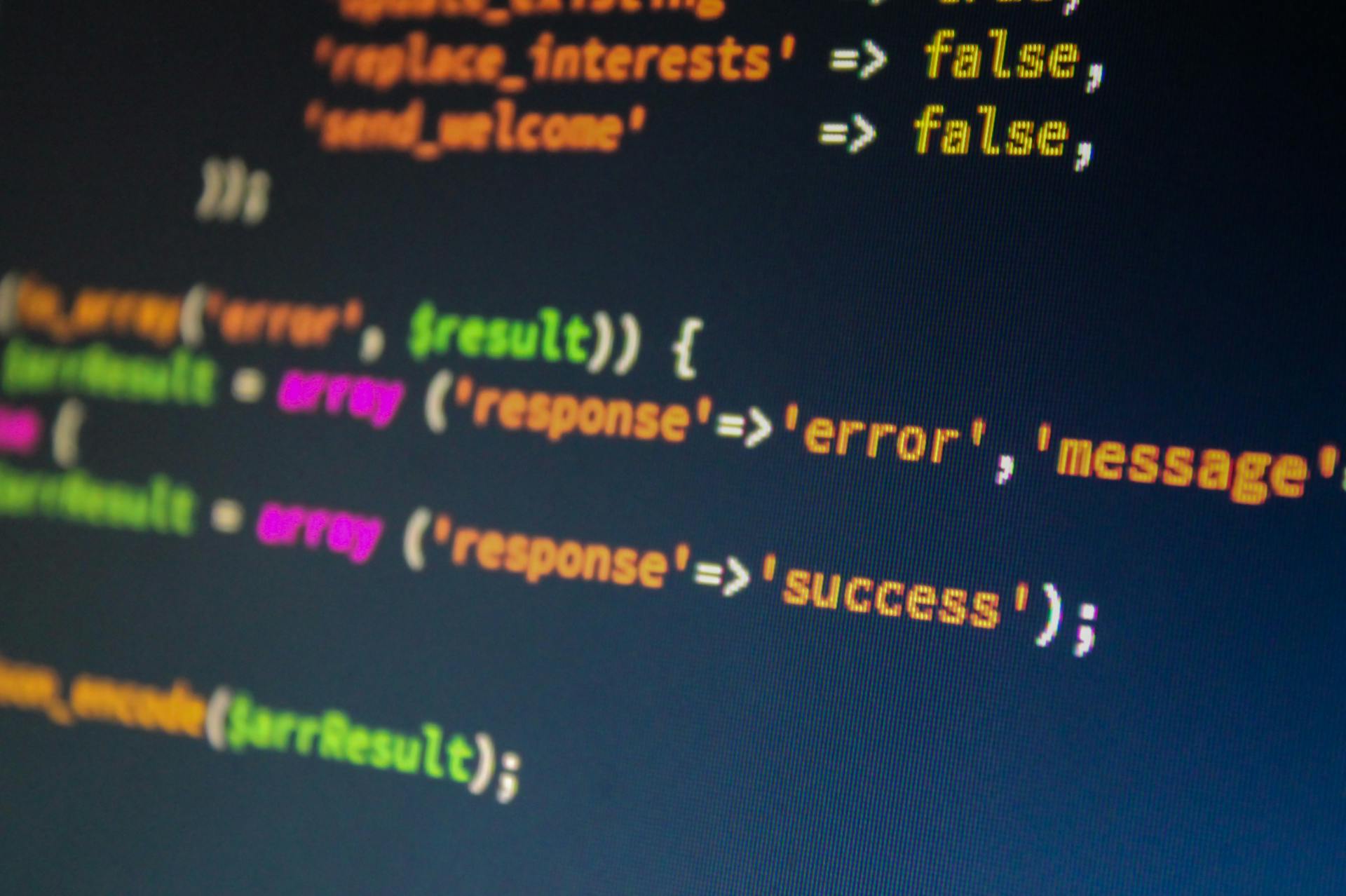
As a Next.js developer, you know how important it is to have a solid logging system in place. Next.js logging allows you to track errors, debug your application, and even collect analytics data.
With Next.js logging, you can use the built-in logging library, which provides a simple way to log messages at different levels of severity. This includes error, warn, info, and debug levels, which can be configured using environment variables.
To get started with Next.js logging, you can use the `next/config` module to configure your logging settings. This module provides a simple way to set up logging for your application, including the ability to log messages to a file or to the console.
You can also use Next.js plugins to extend the logging functionality of your application. For example, the `next-logging` plugin allows you to log messages to a remote server, providing a way to collect analytics data and track errors in real-time.
For your interest: Openshift Logging
Next.js Logging
In Next.js, we don't have a DataDog agent to pick up stdout automatically. Instead, we use a library from DataDog called @datadog/browser-logs.
This library allows us to ship log info off to DataDog via HTTP requests when we call our log function.
The @datadog/browser-logs library is similar to the server side of things, where we used a pino logger.
The client logger in Next.js does both: it logs as the client wants and as the server wants, thanks to a fallback mechanism that calls the server logger.
This fallback mechanism is implemented in the cLog function, which calls sLog() when we're not on the client yet.
Discover more: Console Log in Nextjs
Logging Tools
In Next.js, you can use a library called @datadog/browser-logs to ship log info off to DataDog via HTTP requests.
This library is used because Next.js doesn't have a DataDog agent to pick up stdout automatically like it does on the server side.
The @datadog/browser-logs library is similar to the server side of things, and it provides a fallback to call the server logger (sLog) if the client logger (cLog) is not available.
Readers also liked: Onedrive Logs
The client logger does both: it logs as the client wants and as the server wants, which is a convenient feature for handling prerendering on the server.
This setup allows you to log in both the browser and on the server, making it easier to manage your logs with Next.js.
Broaden your view: Debug Nextjs Client Frontend Webstorm
Debugging and Analytics
Server-side analytics can be a game-changer for Next.js apps. It enables you to render pages on the server instead of the client, which can improve SEO, performance, and user experience.
To integrate PostHog into your Next.js app on the server-side, you can use the Node SDK. This allows you to send events and fetch data from PostHog on the server without making client-side requests.
You can install the posthog-node library by running a command in your terminal. Note that server-side functions in Next.js can be short-lived, so it's essential to set `flushAt` to 1 and `flushInterval` to 0 to ensure events are sent immediately.
Additional reading: Next Js Client Side Rendering
Server-Side Analytics
Server-side rendering enables you to render pages on the server instead of the client, which can be useful for SEO, performance, and user experience.
To integrate PostHog into your Next.js app on the server-side, you can use the Node SDK. This allows you to send events and fetch data from PostHog on the server – without making client-side requests.
You can install the posthog-node library to get started. For the app router, initialize the posthog-node SDK once with a PostHogClient function and import it into files.
To ensure events are sent immediately and not batched, you should set flushAt to 1 and flushInterval to 0. This is because server-side functions in Next.js can be short-lived.
Here's a quick rundown of what these settings do:
- flushAt sets how many capture calls we should flush the queue (in one batch).
- flushInterval sets how many milliseconds we should wait before flushing the queue.
Don't forget to call await posthog.shutdown() once you're done to properly shut down the PostHog client.
Here's an interesting read: Posthog Nextjs
Debugging
Debugging is a crucial step in the development process. It helps identify and fix errors in code, ensuring that applications run smoothly and efficiently.
According to the article, a debugger can be a software tool or a person who manually examines code to find errors. Debuggers can also be integrated into the development environment, making it easier to identify and fix issues.
A good debugger should be able to identify the root cause of an error, not just its symptoms. This is especially important in complex systems where a single error can have a ripple effect.
Debugging can be a time-consuming process, but it's essential to getting it right. In fact, the article notes that debugging can account for up to 50% of the development time.
To speed up the debugging process, it's essential to have a clear understanding of the code and the system it's a part of. This includes knowing the dependencies and interactions between different components.
A well-structured codebase can also make debugging much easier. This includes using modular code, commenting, and following established coding standards.
By following these best practices, developers can reduce the time and effort required for debugging, and focus on building high-quality applications.
Discover more: Nextjs Code Block
Sources
- https://jaketrent.com/post/logging-datadog-nextjs/
- https://docs.sentry.io/platforms/javascript/guides/nextjs/
- https://nextjs.org/docs/app/building-your-application/optimizing/open-telemetry
- https://nextjs.org/docs/app/building-your-application/configuring/debugging
- https://posthog.com/docs/libraries/next-js
Featured Images: pexels.com