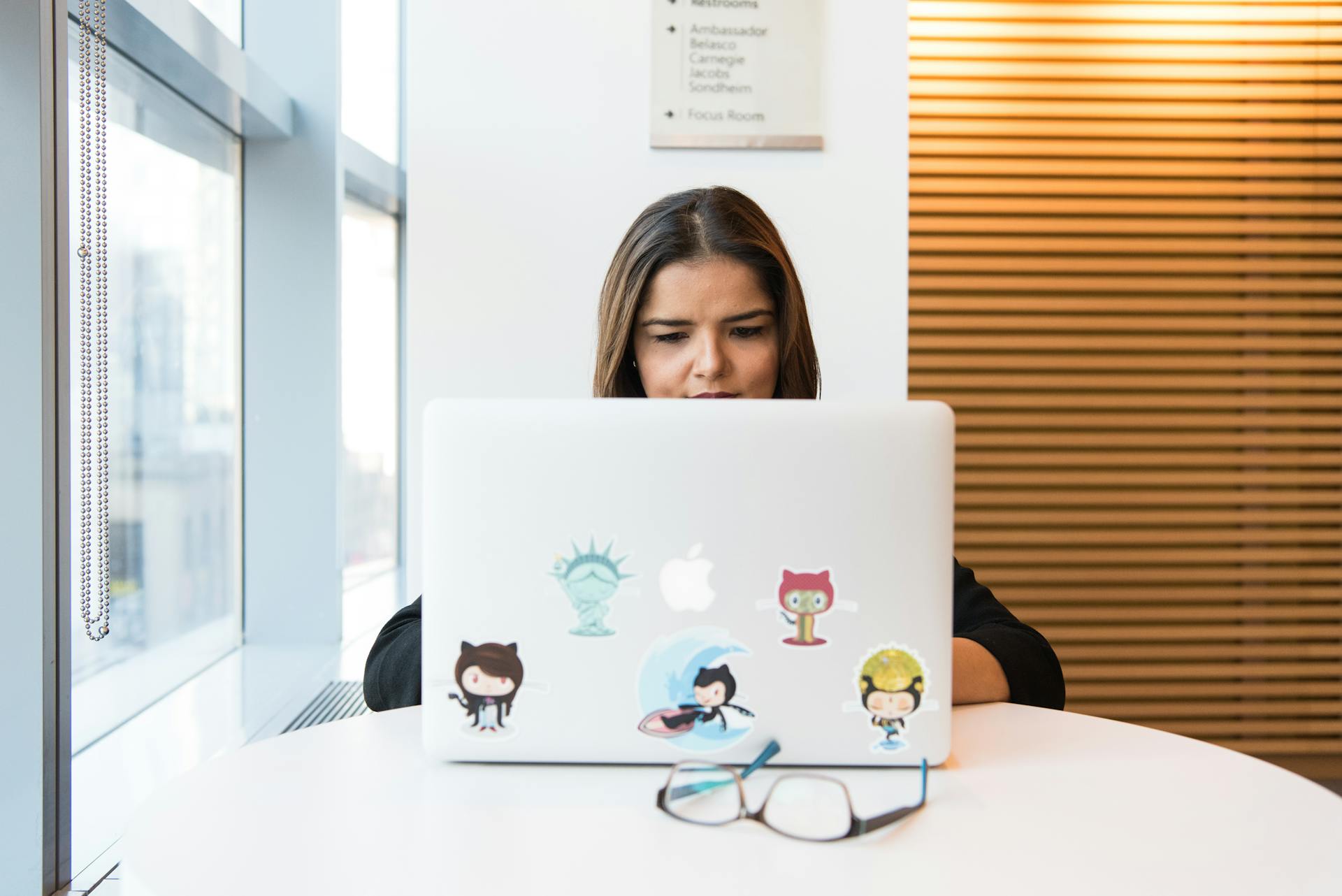
Console logging in Next.js can be a real pain, but don't worry, we've got you covered.
To make console logging easier, Next.js provides a built-in middleware solution. This allows you to log messages at different stages of the page lifecycle, such as during page loading or when a page is unmounted.
You can also use a custom solution to log messages in Next.js. By creating a custom middleware, you can log messages at specific points in your application's lifecycle. For example, you can use this solution to log errors that occur during page loading.
One key advantage of using a custom solution is that you have complete control over what gets logged. This can be particularly useful when debugging complex issues.
Here's an interesting read: Nextjs Custom Server
Error Handling
Error handling is a crucial aspect of Next.js development, and it's essential to understand how to handle both client-side and server-side errors effectively.
Client-side errors can be a real pain, but Next.js provides a solution through error boundaries. These boundaries act as a safety net, catching errors that occur anywhere within their child component tree and preventing the entire application from crashing.
A unique perspective: Next Js Use Client
To handle client-side errors, you can use the App Router, which has greatly improved the developer experience. With it, you can create a nested route page to implement error boundaries in your Next.js application.
If an error occurs, an error boundary will provide a fallback UI and log the error information, making it easier to diagnose and fix the issue. This is a game-changer for developers, as it prevents the entire application from crashing and provides a better user experience.
Server-side errors, on the other hand, can be handled using try/catch blocks. By catching errors and returning appropriate error responses to the client, you can ensure that your API routes are secure and provide a good user experience.
A unique perspective: Next Js Single Page Application
Handling Client-Side Errors
Client-side errors can stop your application from running if not handled effectively.
In Next.js, syntax, runtime, and data fetching errors can be prevented and fixed using an error boundary.
An error boundary acts like a safety net for your application, catching errors that occur within its child component tree and preventing the entire application from crashing.
A unique perspective: Error Boundary Nextjs
In previous versions of Next.js, custom error pages like 404 and 500 pages helped with error handling, but they have limitations, such as limited customization and outdated support for React error boundaries.
The new App Router in Next.js has greatly improved the developer experience for handling errors.
To handle errors in your application, you can simulate a network error in a specific route, like the profile page route.
You can create a simulateNetworkError function that creates a promise to simulate a network error after a delay, which will create a runtime error for your application.
The error.js file automatically creates a React error boundary that wraps a nested child segment or page.js component and provides a fallback UI in case of an error.
Some errors can be temporary and resolved by trying again, which is where the reset function comes in, prompting the user to recover from the error by re-rendering the error boundary's contents.
Here's an interesting read: Nextjs Error Page
Handling Server-Side Errors
Handling server-side errors is crucial for a smooth user experience. Next.js will forward an Error object to the nearest error.js file when an error occurs in a server component.
For security reasons, Next.js sends only generic messages to the client, avoiding the leak of potentially sensitive information. This is a deliberate design choice to protect user data.
To handle API route errors, use try/catch blocks to catch errors and return appropriate error responses to the client. This is demonstrated in an example where a GET request is made to an external API endpoint, and an error is thrown if the response is unsuccessful.
A generic error message is returned to the client if the server-side error is not caught and handled properly. This is because Next.js does not leak potentially sensitive information to the client.
Recommended read: Next Js Client Side Rendering
Logging and Debugging
Custom logging libraries like pino offer flexibility and customization for logging in Next.js. Pino is a high-performance logging library for Node.js that supports log levels, different output formats, and has a handy pretty-print plugin for development environments.
Related reading: Openshift Logging
You can integrate pino with Next.js by adding it to the serverComponentsExternalPackages array in your next.config.js file. This allows you to customize your logs and make them more readable.
For debugging middleware, use debugging tools like console.log or dedicated debugging tools to inspect the flow of your middleware. Debug statements can be added at the entry and exit points of the middleware to show when it starts and finishes its execution.
Here are some tools you can use for debugging middleware:
- console.log
- Built-in debug module
- External tools like Node.js's built-in inspector or third-party tools such as ndb or node-inspect
These tools allow you to set breakpoints, inspect variables, and step through code, providing a more in-depth understanding of your middleware's execution flow.
Debugging
Debugging is a crucial step in ensuring your middleware behaves as expected. Debug statements can be added at the entry and exit points of middleware to show when it starts and finishes its execution.
The console.log statements provide valuable information about the request method and URL. This helps you understand the flow of your middleware.
Check this out: Nextjs Middleware Firebase Auth
To take debugging to the next level, consider using dedicated debugging tools like the built-in debug module or external tools like Node.js's built-in inspector. These tools allow you to set breakpoints and inspect variables.
Here are some popular debugging tools:
- Node.js's built-in inspector
- Third-party tools like ndb or node-inspect
By using these tools, you can gain a more in-depth understanding of your middleware's execution flow and identify any issues.
Logger
The Logger is a crucial part of any Next.js application, allowing you to track and debug your code. You can override the default logger levels, which are console, to suit your needs.
You can use the logger to send logs to a third-party logging service. The logger levels are undefined by default and will use the built-in logger if not defined.
The logger levels that can be overridden are:
- debug
- info
- warn
- error
If the debug level is defined by the user, it will be called regardless of the debug: false option. This means you can still use the debug level even if you've set debug to false.
Broaden your view: How to Debug Next Js in Vscode
Middleware and Custom Solutions
You can create custom middleware to log specific information in Next.js, such as incoming requests.
To write your first middleware, create a file like middleware/logger.js and add a simple logging function.
This middleware can be added to your next.config.js file to log every incoming request to the console. You can easily extend this middleware to include more details or modify it for other purposes.
For example, you can extend the logging middleware to log not only requests but also the user making the request, by adding a middleware/logger.js file and a few tweaks to your next.config.js file.
For your interest: Next Js Cookie
Creating Middleware
You can create a simple middleware that logs information about incoming requests, like logging the user making the request.
This middleware can be extended to include more details or modified for other purposes, as seen in the example where it logs not only requests but also the user making the request.
To use this middleware, you need to add it to your next.config.js file, which is where you can configure your Next.js application.
Adding a middleware to your next.config.js file is as simple as requiring the middleware file and passing it to the Next.js function.
By adding middleware to your next.config.js file, you can easily extend the functionality of your Next.js application without having to make changes to your existing code.
Consider reading: Onedrive Logs
Custom Logs with Pino
Pino is a high-performance logging library for Node.js that offers the flexibility and customization we needed at Arcjet. It supports log levels, different output formats, and has a handy pretty-print plugin for development environments.
To integrate pino with Next.js, you need to add it to your next.config.js file, specifically to the serverComponentsExternalPackages array. This allows pino to work seamlessly with your Next.js application.
Pino's pretty-print plugin is particularly useful for local logging, as it provides colorized output. This makes it easier to read and understand your logs during development.
You can also use pino to redact fields by JSON path, which is a great feature for protecting sensitive data.
For another approach, see: Nextjs Development Company
Featured Images: pexels.com