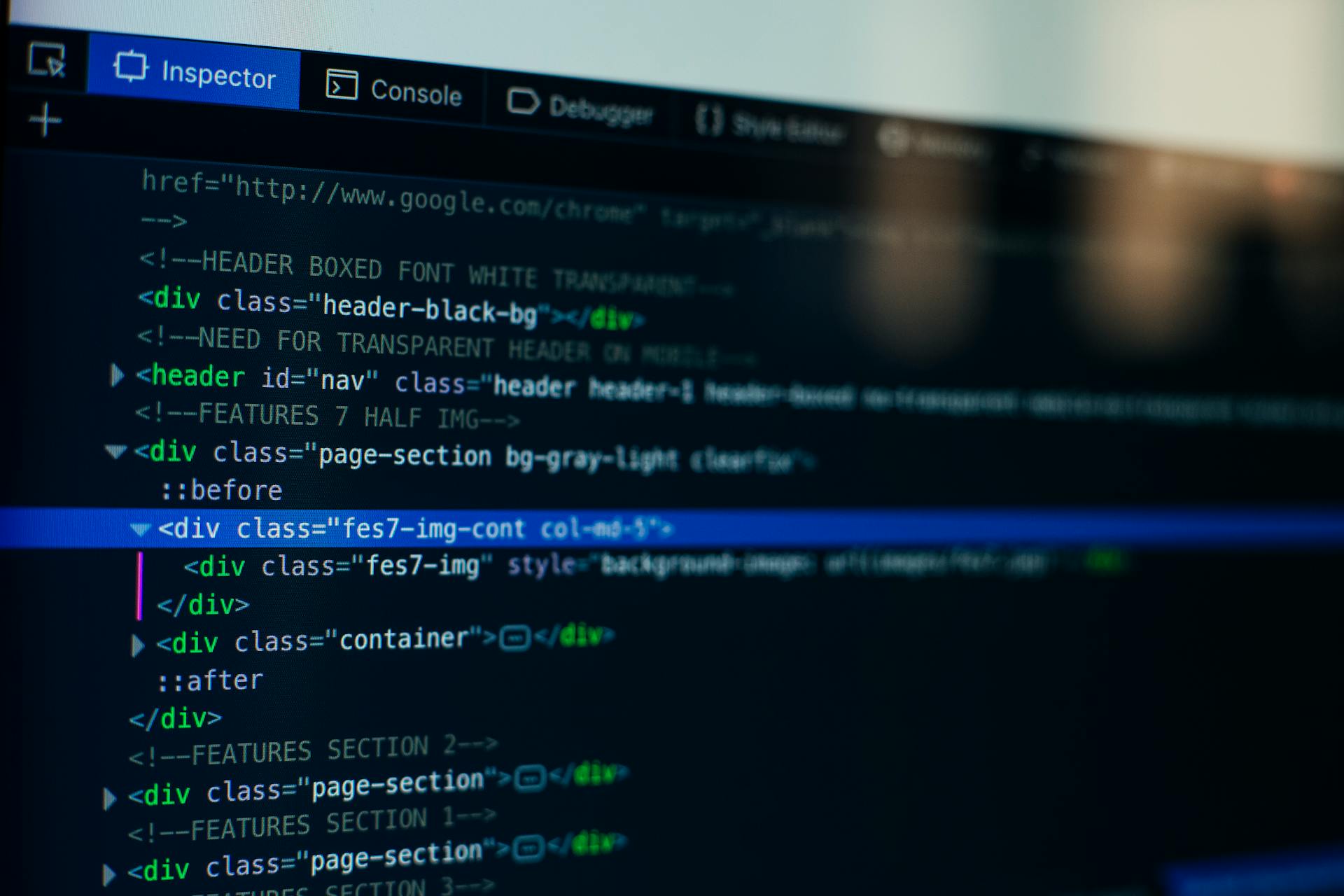
Debugging Next.js in VSCode can be a daunting task, but don't worry, I'm here to guide you through it.
First, make sure you have the Next.js extension installed in VSCode, which allows you to create and debug Next.js applications with ease.
To start debugging, open the Command Palette in VSCode and type "Next.js: Start Server" to launch the development server.
Next, configure your launch.json file to specify the debug configuration for your Next.js application.
In the launch.json file, add a new configuration for Next.js, specifying the "type" as "next" and the "protocol" as "http".
Check this out: Next Js Debugging
Setting Up Next.js in VSCode
To set up Next.js in VSCode, you need to have the latest version of Visual Studio Code installed on your system. Install the necessary extensions, as they are crucial for efficient debugging.
The debugger; keyword is a game-changer for identifying potential bugs or issues in your code. Place it strategically in your JavaScript or TypeScript code to pause execution and inspect variables.
For another approach, see: Nextjs Code Block
Edit the .vscode/launch.json file in VSCode to define configurations for your Next.js project. This file lets you specify launch configurations for debugging, such as attaching to a running server or launching a dev script modification.
Start your Next.js project from scratch by setting up a configuration in the launch.json file. You can also connect the debugger to a running server for flexibility in how you debug your app.
Call stack navigation and the watch variables feature are essential debugging tools in VSCode. They will help you analyze code, inspect variables, and find bugs in your Next.js apps.
For your interest: Nextjs File Structure
Debugging Basics
To debug Next.js in VSCode, you'll need to understand the basics of debugging, including setting breakpoints and inspecting variables.
You can use the debug panel in VSCode to set breakpoints, step through code, and analyze runtime behavior.
Knowing how to set breakpoints is key. You need to inspect variables and step through code. These skills are vital in debugging Next.js applications.
Discover more: Nextjs Build Fails Environment Variables
You can use breakpoints to pause execution at specific points and track down bugs.
Here are the steps to set up debugging in VSCode:
- Update your package.json file to include a debug script.
- In your .vscode/launch.json file, add a new configuration for attaching the debugger to the Node.js process.
By following these steps, you can start debugging your Next.js application in VSCode.
Common Issues
Debugging Next.js apps can be tricky, especially when dealing with asynchronous code, which can make breakpoints hit unpredictably.
One common issue developers face is server-side debugging, where ensuring code executes correctly and handling errors requires careful attention.
Breakpoints may not hit as expected due to timing issues, making it hard to pinpoint the source of bugs.
Reviewing open issues on the Next.js GitHub repository can provide valuable insights and workarounds from the community.
Environment variables, such as NODE_OPTIONS, can aid in debugging and optimizing server-side code.
Running commands like npm run dev or yarn dev can start the development server and monitor its output for any issues during the development process.
Debugging client-side code within the Next.js framework presents its own set of challenges, particularly in understanding data flows between components and tracking state changes.
Discover more: Nextjs Dev Rel
Advanced Debugging Techniques
To take your debugging skills to the next level, you can optimize your workflow by utilizing advanced features in Visual Studio Code. Setting conditional breakpoints can simplify your debugging process by pausing code only when specific conditions are met.
Using the watch window in VS Code allows you to track variables and expressions in real-time, providing valuable insights into your application's behavior. This feature is particularly useful when troubleshooting complex issues.
In addition to these features, the terminal in VS Code can be used to enter debug mode and interact with your Next.js project directly. This streamlines your development process and eliminates the need to switch between tools.
Here are some key features to explore:
- Conditional breakpoints: Pause code only when specific conditions are met.
- Watch window: Track variables and expressions in real-time.
- Terminal: Enter debug mode and interact with your Next.js project directly.
By incorporating these advanced features into your debugging toolkit, you can work more efficiently and effectively when troubleshooting issues in your Next.js applications.
Optimizing with Advanced Features
Using advanced features in Visual Studio Code can significantly streamline debugging for your Next.js apps. This can lead to faster issue resolution and better code.
One key feature is the ability to set conditional breakpoints, which allows you to pause code only when you meet specific conditions. This can simplify your debugging and focus on key code areas.
The watch window in VS Code is another powerful tool that lets you track variables and expressions in real-time as you debug. This feature provides valuable insights into your application at different points during execution.
Using the terminal in VS Code helps you enter debug mode and interact with your Next.js project during debugging, removing the need to switch between tools.
Consider reading: Next Js Feature Flags
Maximizing Breakpoint Usage for Inspection
Breakpoints are a powerful tool in debugging Next.js applications. Strategically place breakpoints in your code to pause execution at specific points and inspect variables.
Using breakpoints can help you find issues by showing how components interact. This level of granularity is essential for identifying hard-to-find bugs and optimizing performance.
To use breakpoints effectively, place them in your codebase, especially in key areas where issues may arise. This will help you narrow your debugging efforts and pinpoint problems.
Explore further: Breakpoints Webstorm Nodejs Nextjs
You can customize debugging by setting breakpoints based on conditions or expressions. This step helps you understand your code better in various situations.
Here are some advanced features of the VS Code debugger that you can use to maximize breakpoint usage:
- Conditional breakpoints: Pause code only when you meet specific conditions.
- Watch window: Track variables and expressions in real-time as you debug.
- Terminal: Enter debug mode and interact with your Next.js project during debugging.
By using these features and strategically placing breakpoints, you can streamline debugging, save time and effort, and ensure smooth debugging for your Next.js apps.
Using the Debugger
To use the debugger in VS Code, you'll need to attach it to the Node.js process. Update your package.json file to include a debug script, and then add a new configuration for attaching the debugger to the Node.js process in your .vscode/launch.json file.
To start debugging, use the command "npm run debug" or "yarn debug" to start your Next.js application in debug mode. Then, open the "Run" panel in VS Code and click the "Run" button next to the "Attach to Node.js" configuration.
A fresh viewpoint: Next Js vs Node Js
You can now set breakpoints and debug your server-side code, including API routes and getServerSideProps functions. Breakpoints let you pause code execution at specific points, allowing you to check variables and monitor the program's flow in real-time.
Here are the steps to follow:
1. Update package.json file
2. Add new configuration to launch.json file
3. Start Next.js application in debug mode
4. Attach to Node.js process in VS Code
By following these steps, you'll be able to use the debugger in VS Code to streamline your debugging process and improve your coding skills.
Take a look at this: Nextjs Server Actions File Upload
Server and Browser Debugging
Debugging server-side code in a Next.js application is a bit different from debugging client-side code. You'll need to attach the debugger to the running Node.js process.
To do this, update your package.json file to include a debug script. This will allow you to start your Next.js application in debug mode using npm run debug or yarn debug. I've found this step to be crucial in getting the debugger attached correctly.
If this caught your attention, see: Next Js Client Side Rendering
Once you've updated your package.json file, you'll need to add a new configuration for attaching the debugger to the Node.js process in your .vscode/launch.json file. This configuration will allow you to attach the debugger to the running process.
After adding the configuration, start your Next.js application in debug mode, and then open the "Run" panel in VSCode. Click the "Run" button next to the "Attach to Node.js" configuration to attach the debugger to the process. You can now set breakpoints and debug your server-side code, including API routes and getServerSideProps functions.
Alternatively, you can use the Debugger for Chrome extension to debug both server and client execution from a single VSCode launch command. This can be a more streamlined approach to debugging your Next.js application.
Expand your knowledge: How to Run Next Js App
Frequently Asked Questions
How to debug js file in VS Code?
Debug a JavaScript file in VS Code by using a launch config or attaching to a process via the auto attach feature in the integrated terminal
Sources
- https://codedamn.com/news/nextjs/profiling-debugging-nextjs-advanced-tools
- https://www.nextjs.cn/docs/advanced-features/debugging
- https://nomadicsoft.io/advanced-debugging-techniques-for-next-js-applications-with-vs-code-breakpoints/
- https://elliott.dev/posts/debugging-nextjs-applications
- https://avirup-dev.vercel.app/articles/debug-next-js-with-vs-code
Featured Images: pexels.com