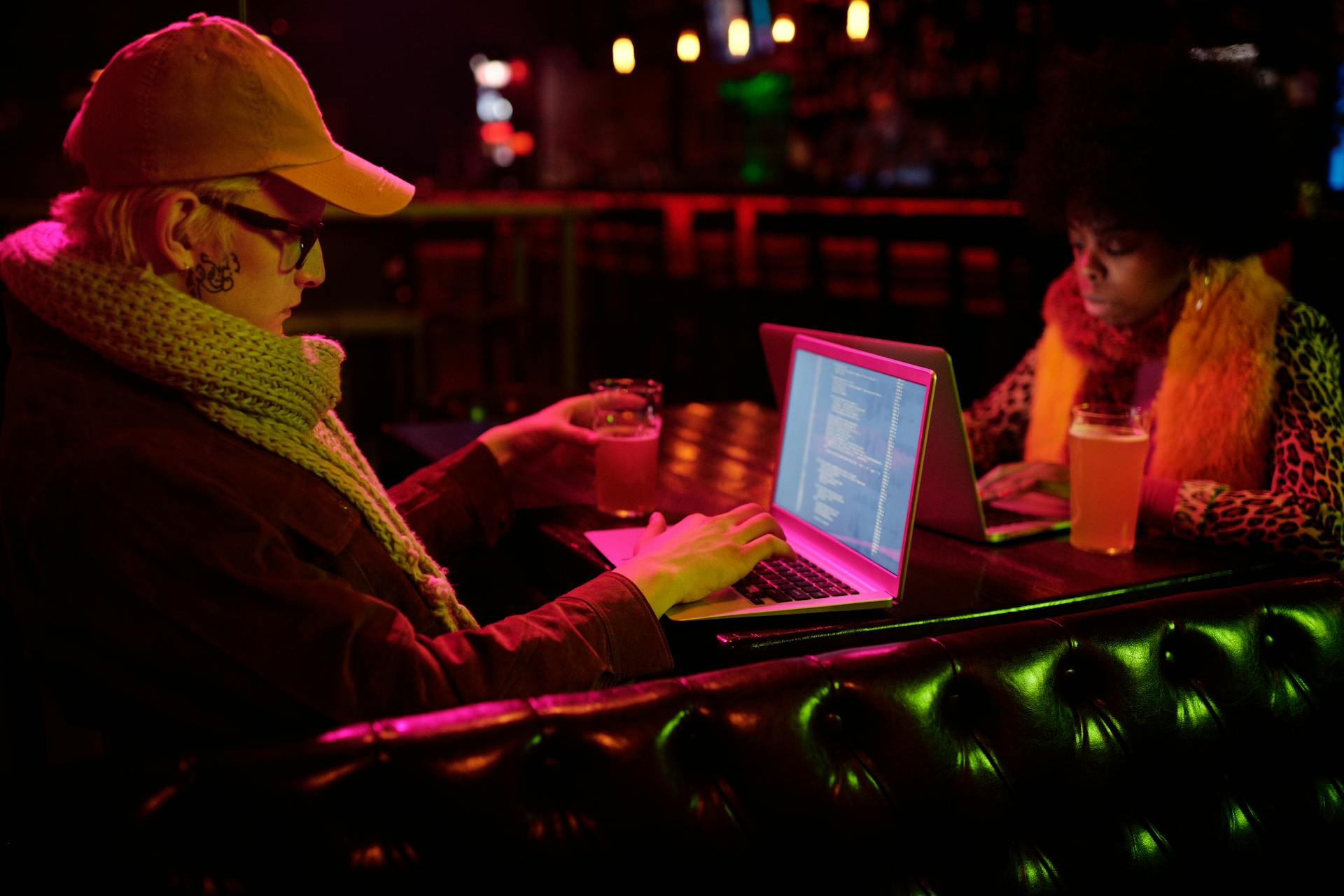
To set up a Next.js project with WebStorm, you'll need to install the necessary plugins and configure your environment. Install the Node.js and Next.js plugins in WebStorm by going to Settings > Plugins.
For debugging, you can use the built-in WebStorm debugger or a third-party tool like Next.js Debugger. The built-in debugger provides features like breakpoints, step-through execution, and variable inspection.
Next.js provides a built-in debugging tool that allows you to view and edit your application's state in real-time. This tool is accessible by going to the browser's developer tools and selecting the "Components" tab.
Setting Up Breakpoints
To set up breakpoints in WebStorm for Node.js and Next.js projects, you need to use the --inspect or --inspect-brk flag when starting your application.
You can add breakpoints in your code by setting the debugger; statement somewhere in your code. This is probably the easiest way to launch the built-in debugger.
In WebStorm, you can initiate a debugging session in two ways: start the debugger together with your application using a Node.js run/debug configuration, or attach the debugger to an already running application.
To debug a running application, use an Attach to Node.js/Chrome configuration, and make sure to select the Reconnect automatically checkbox.
Here are the steps to set up breakpoints in WebStorm:
1. Set the breakpoints in your code as necessary.
2. Create a new Attach to a Node.js/Chrome configuration and select the Reconnect automatically checkbox.
3. From the Run widget list on the toolbar, select the newly created Attach to Node.js/Chrome configuration and click next to it.
By following these steps, you can effectively set up breakpoints in WebStorm for Node.js and Next.js projects.
Debugging Tools
The built-in debugger in Node.js is a great place to start when debugging your application. You can add a `debugger;` statement to your code and use commands like `cont` (shortcut `c`) to continue execution, `exec()` to evaluate an expression, or `next` (shortcut `n`) to step to the next line.
To use the built-in debugger, you'll need to have command line access to your app. If you're using a different way to launch Next.js, you can configure it to start in debug mode by adding `NODE_OPTIONS='--inspect'` before the next command.
WebStorm makes it easier to debug Node.js applications. You can put breakpoints right in your JavaScript or TypeScript code without needing a debugger or console.log() statements.
To debug a Node.js application with WebStorm, you can start the debugger together with your application using a Node.js run/debug configuration. Alternatively, you can attach the debugger to an already running application.
The WebStorm debugger recognizes the `--inspect`, `--inspect-brk`, and `--debug` flags, so you can make any application accessible for debugging. You can also debug Node.js applications that are running in Vagrant boxes, in Docker containers, or on remote hosts accessible via various transfer protocols or via SSH.
Here are some ways to debug a Node.js application with WebStorm:
- Set breakpoints in your code as necessary
- Create a new Attach to a Node.js/Chrome configuration and select the Reconnect automatically checkbox
- From the Run widget list on the toolbar, select the newly created Attach to Node.js/Chrome configuration and click next to it
- Perform the actions that will trigger the code at the breakpoint
You can also debug a Node.js application that uses nodemon by starting it in the debug mode (with the `--inspect` or `--inspect-brk` flag) and then connecting to it using the Attach to a Node.js/Chrome debug configuration with the Reconnect Automatically option on.
The Node.js inspector is a powerful tool for debugging your application. You can execute a program with a debug server using the `node --inspect` command, which listens on TCP port 9229. This allows you to connect to the server using an Inspector Client and debug your app using a full-featured UI.
You can also use the Node.js inspector via Chromium DevTools. Simply go to `chrome://inspect`, add `localhost:9229` as a target, and click "inspect" to see a familiar UI. This works just like debugging your app in the browser.
Next.js Debugging
Debugging Next.js applications can be challenging, but with the right tools and techniques, you can streamline the process and find bugs quickly.
To start debugging, configure Next.js to start in debug mode by setting the NODE_OPTIONS flag to '--inspect'. This will allow you to set breakpoints and inspect variables in your code.
In VS Code, you can use the built-in debugger to set breakpoints, step through code, and inspect variables. Next.js development integrates well with VS Code, making it a powerful tool for debugging.
If you're using WebStorm, you can debug a running Node.js application by attaching the debugger to the process. This can be done by creating a new Attach to Node.js/Chrome configuration and specifying the host and port where the application is running.
To debug a running Node.js application, you can also use the Chrome Debugging Protocol. This involves running the application with the --inspect flag and then attaching the debugger to the process using an Attach to Node.js/Chrome configuration.
Server-Only
Server-Only Debugging can be a challenge because some of the code is executed only on the server, like getServerSideProps, getStaticProps, and getStaticPaths, which can't be debugged in the browser at all.
The good news is that the Next.js server is fundamentally a Node.js process, so it can be debugged like any other Node.js process.
Configure Next.js for Debug Mode
To configure Next.js for debug mode, you need to set the NODE_OPTIONS flag. This flag is a standard Node.js flag that allows you to add options for Node.js. Specifically, you need to set it to '--inspect' before running the Next.js development command.
You can do this by modifying your package.json file to include the following script:
```json
"scripts": {
"dev": "NODE_OPTIONS='--inspect' next dev",
"build": "next build",
"start": "next start"
},
```
This will start the Next.js development server in debug mode, allowing you to set breakpoints and inspect variables.
Alternatively, you can also set the NODE_OPTIONS flag when running the development command directly. However, be aware that this can lead to issues with multiple debuggers trying to start on the same port, resulting in an error like "address already in use".
It's also worth noting that using `NODE_OPTIONS='--inspect' npm run dev` or `NODE_OPTIONS='--inspect' yarn run dev` won't work, as it will try to start multiple debuggers on the same port.
By setting the NODE_OPTIONS flag to '--inspect', you'll be able to start the Next.js development server in debug mode, making it easier to find and fix issues in your code.
Here are some common issues you may encounter when debugging Next.js apps, and how to troubleshoot them:
Debugging Techniques
To debug a Node.js application, you can use the built-in debugger or a third-party tool like WebStorm.
You can initiate a debugging session in WebStorm by starting the debugger together with your application using a Node.js run/debug configuration or by attaching the debugger to an already running application.
The WebStorm built-in debugger can automatically reconnect to running Node.js processes, making it ideal for debugging applications that use the nodemon utility.
To debug a running Node.js application, you can start it in the debug mode with the --inspect or --inspect-brk flag and then connect to it using an Attach to a Node.js/Chrome debug configuration.
Breakpoints are a powerful tool in debugging Next.js applications. Strategically place breakpoints in your code to pause execution at specific points and inspect variables.
You can use the debug panel in your IDE to set breakpoints, step through code, and analyze runtime behavior. Breakpoints can be customized based on conditions or expressions to provide more detailed insights.
To maximize breakpoint usage, place them in your codebase to find issues and monitor the program's flow in real-time.
Here are some common debugging techniques:
- Set breakpoints in your code to pause execution at specific points
- Use the debug panel in your IDE to step through code and analyze runtime behavior
- Customize breakpoints based on conditions or expressions
- Use the WebStorm built-in debugger to automatically reconnect to running Node.js processes
- Start a debugging session in WebStorm by starting the debugger together with your application or attaching to an already running application
Advanced Debugging
You can debug a running Node.js application with WebStorm using the Attach to Node.js/Chrome configuration and the Chrome Debugging Protocol.
To start debugging, run your application with an --inspect or --inspect-brk flag. This flag allows WebStorm to initiate a debugging session.
You can set breakpoints as necessary before running your application. Then, run your application with the --inspect or --inspect-brk flag and hold Ctrl+Shift to click the link in the Debugger listening message.
Alternatively, you can copy the port number from the Debugger listening message and paste it later in the Attach to Node.js/Chrome configuration.
Debugging a Node.js application that uses nodemon is also possible with WebStorm. You can start your application in debug mode with the --inspect or --inspect-brk flag and then connect to it using the Attach to a Node.js/Chrome debug configuration with the Reconnect Automatically option on.
Here's a summary of the steps to debug a Node.js application with WebStorm:
Debugging with Tools
You can debug a Next.js application using the VS Code debugger, which integrates seamlessly with Next.js projects. This allows you to set breakpoints, pause and inspect variables, and step through code.
To debug a Node.js application, you can use WebStorm, which makes it easy to put breakpoints in your JavaScript or TypeScript code. You can initiate a debugging session by starting the debugger together with your application using a Node.js run/debug configuration.
With WebStorm, you can also debug a running Node.js application by attaching the debugger to an already running process. This is done by creating an Attach to Node.js/Chrome configuration and selecting the Reconnect automatically checkbox.
You can debug a Node.js application that uses nodemon by starting it in the debug mode (with the --inspect or --inspect-brk flag) and then connecting to it using the Attach to a Node.js/Chrome debug configuration with the Reconnect Automatically option on.
Here are the different ways to start a debugging session in WebStorm:
- Start the debugger together with your application using a Node.js run/debug configuration.
- Attach the debugger to an already running process using an Attach to Node.js/Chrome configuration.
- Debug a running Node.js application using the Attach to Node.js/Chrome configuration.
You can debug a Node.js application that uses nodemon by starting it in the debug mode (with the --inspect or --inspect-brk flag) and then connecting to it using the Attach to a Node.js/Chrome debug configuration with the Reconnect Automatically option on.
Using the VS Code debugger, you can set breakpoints, pause and inspect variables, and step through code. This helps you find and fix issues in your Next.js applications faster.
You can use the following flags to start a debugging session:
- --inspect
- --inspect-brk
- --debug (now deprecated)
Make sure to specify the host and port where the target application is running when creating an Attach to Node.js/Chrome configuration.
Debugging with Nodemon
You can debug a Node.js application that uses nodemon with WebStorm's built-in debugger. This lets you automatically reconnect to running Node.js processes.
To start, you need to run your application in the debug mode with the --inspect or --inspect-brk flag. This will enable the debugger to listen for connections.
Once your application is running, you can connect to it using an Attach to a Node.js/Chrome debug configuration in WebStorm. Make sure to select the Reconnect Automatically option to ensure the debugger reconnects to the process when it's restarted.
With nodemon, your application will automatically reload when you save changes to your code. The debugger will also reconnect to the restarted process, allowing you to continue debugging without interruption.
Here are the steps to debug a Node.js application that uses nodemon:
1. Run your application in the debug mode with the --inspect or --inspect-brk flag.
2. Create an Attach to a Node.js/Chrome debug configuration in WebStorm with the Reconnect Automatically option selected.
3. Save your changes to the code and wait for the application to reload.
4. The debugger will reconnect to the restarted process, and you can continue debugging as usual.
Sources
- https://dev.to/spe_/debugging-next-js-applications-46b6
- https://dev.to/vvo/5-steps-to-debugging-next-js-node-js-from-vscode-or-chrome-devtools-497o
- https://nomadicsoft.io/advanced-debugging-techniques-for-next-js-applications-with-vs-code-breakpoints/
- https://www.jetbrains.com/help/webstorm/running-and-debugging-node-js.html
- https://stackoverflow.com/questions/54354389/how-does-one-debug-nextjs-react-apps-with-webstorm
Featured Images: pexels.com