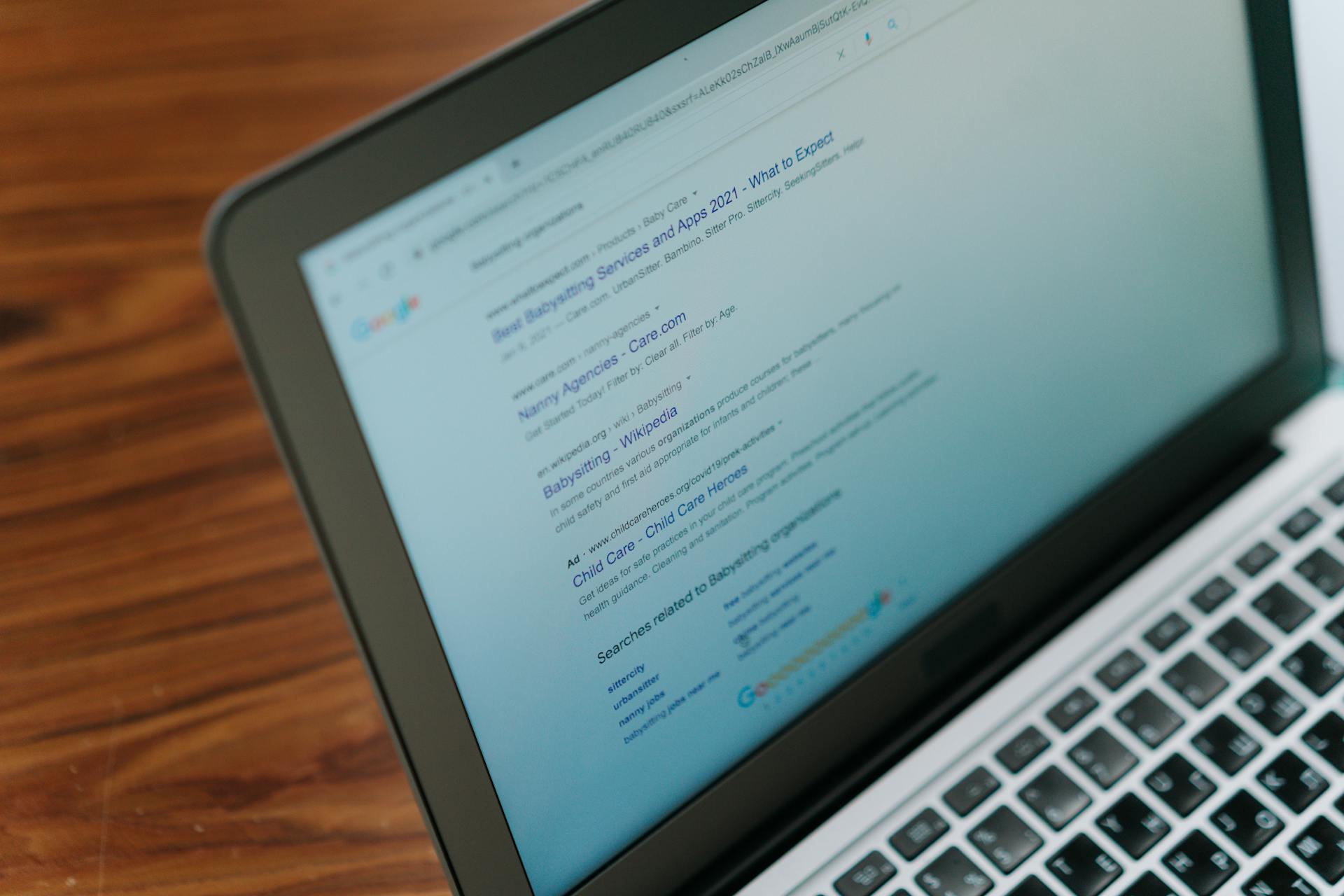
Creating a Next.js Chrome extension requires a solid understanding of the underlying technologies. This guide will walk you through the process, step by step.
First, you'll need to set up a new Next.js project using the command `npx create-next-app my-extension`. This will create a basic project structure for your extension.
Next, you'll need to create a new directory for your extension's code, and then run `npm install` to install the required dependencies. This includes the `chrome-extension` package, which provides a set of utilities for building Chrome extensions.
With your project set up, you can start building your extension's UI using Next.js's built-in components. For example, you can use the `Link` component to create a navigation menu.
Related reading: Using State in Next Js
Building the Extension
To create a Next.js Chrome extension, you first need to create your app with the Next.js template by executing the following command: `npx create-next-app my-extension --experimental-app`. Don't select the App router option, or you'll encounter errors.
A unique perspective: Cookies Nextjs
You'll then need to install the gsed package to replace underscores in filenames, which are prohibited in extensions. On Mac, you can install gsed by running `brew install gsed`.
To build and export your extension, use the `yarn run build:extension` command, which will call `lint:build` and utilize the gsed package to replace underscores in file names.
Readers also liked: Install Next Js 13
Building UI
Building UI for your Chrome extension requires a form to collect user data and a button to save it to the Notion database. This involves replacing the code in the index.js file in the pages directory with specific code.
You use the Chrome API to query the currently opened tab, getting its URL and title. The pageData state variable is then set.
To save bookmark data to Notion, you need to add a saveBookmarkToNotion method. This method is crucial for interacting with the Notion database.
The handleSubmit method is also essential, as it handles form submission and data processing. It gets the form's data, converts it to a JSON object, and modifies the bookmark object by converting tags into an array of objects.
See what others are reading: Nextjs Code Block
Here's a step-by-step guide to implementing the handleSubmit method:
- You get the form’s data (e.target) and convert it to a JSON object (Object.fromEntries).
- Since tags are a comma-separated string, you convert the tags into an array of objects where each object is of {name: tag} structure.
- You pass the modified bookmark object to the saveBookmarkToNotion function.
- Based on the result, you update the state variables isSaved and isSaving.
After implementing the handleSubmit method, save your progress and start the development server by running a specific command.
Configuring the Manifest
The manifest.json file is the configuration file of your extension, containing metadata and security permissions.
This file should be added to your Next.js app's /public directory. Think of it as the blueprint for your extension.
The manifest.json file contains essential fields like name and version, which are simply metadata for the extension. These fields are required.
The manifest_version field is crucial, defining which manifest.json version the browser should interpret. You can check the documentation for compatible versions.
The action or browser_action field is responsible for creating the actual icon and title of the extension within the browser's toolbar. It's also responsible for creating a pointer to your Next.js application.
Here's a breakdown of the key fields in the manifest.json file:
Next.js Integration
To integrate Next.js with Chrome, you need to create a Next.js app with the Next.js template. This can be done by executing the command `npx create-next-app my-app --ts`. However, make sure not to select App router, as it will cause errors.
Curious to learn more? Check out: Next Js App
You'll need to install the `gsed` package to replace underscores in filenames, which is prohibited for extensions. This can be done by running `brew install gsed` on Mac.
To build and export your extension, use the `build:extension` command, which will call `lint:build` and utilize the `gsed` package. This will replace the `_next` directory with `assets` and fix any reference issues.
A manifest.json file is required in the `/public` directory, where you need to modify the permissions to include history and storage. This is because your extension will save specific websites in the browser's storage and clear their history.
The `action` property in manifest.json should include `"default_popup": "index.html"` to display the default page upon clicking the extension. You'll be building your extension mainly from the `/pages/index.html`.
To use Chrome API to delete history and save data in browser storage, you need to install `chrome-types` by running `yarn add -D chrome-types`. Then, open `tsconfig.json` and add `["chrome-types"]` to the `compilerOptions`.
After configuration, run `yarn run build:extension` to produce an `/out` directory.
Consider reading: Text Html Chrome Extension
Customization
Customization is a crucial aspect of building a Next.js Chrome extension. You can customize the appearance of your extension by creating a custom popup HTML file.
By default, Next.js Chrome extensions use a standard popup template, but you can easily swap it out for a custom one. This allows you to tailor the look and feel of your extension to your brand.
The custom popup HTML file can be created using React components, making it easy to reuse code and maintain a consistent design. You can also use CSS to style the popup and make it more visually appealing.
To customize the behavior of your extension, you can use the Chrome extension API to interact with the browser and perform actions. This allows you to create a seamless user experience.
Next.js provides a built-in API to handle events and interactions, making it easy to respond to user input and update the extension's behavior accordingly. This API can be used to create custom event handlers and update the extension's state.
You can also customize the extension's behavior by using environment variables to store sensitive information. This allows you to keep your extension's configuration separate from its code.
Here's an interesting read: Nextjs Server Actions File Upload
Sources
- https://www.coditude.com/chrome-extension-boilerplate-with-next-js/
- https://birdeatsbug.com/blog/build-a-chrome-extension-in-next-js-and-notion-api
- https://christianpenrod.com/blog/developing-browser-extensions-with-nextjs
- https://thedatalife.com/building-a-nextjs-chrome-firefox-extension
- https://ui-lib.com/blog/nextjs-extensions/
Featured Images: pexels.com