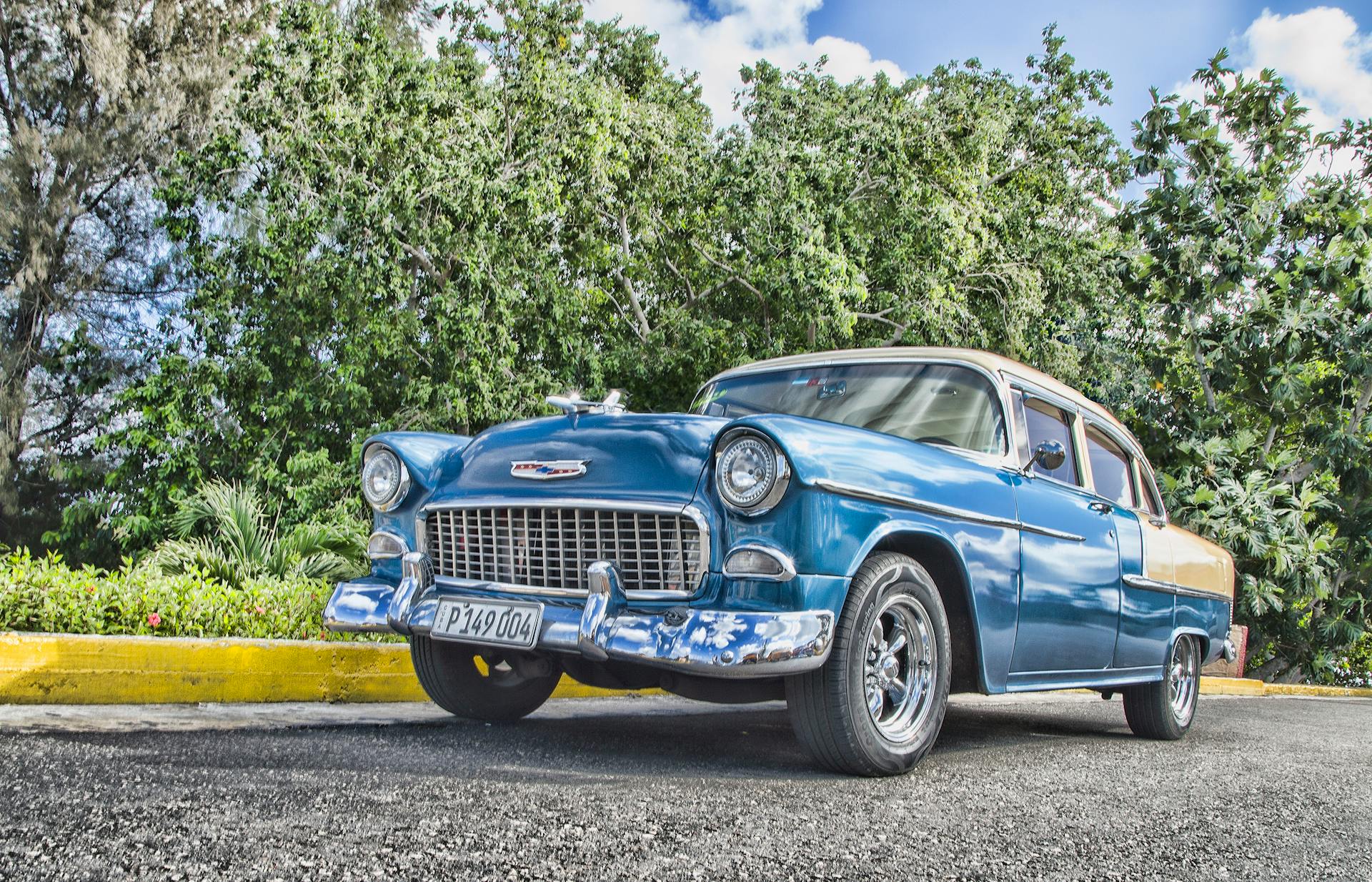
Building a Chrome DevTools Extension is a great way to customize your development experience and make your job easier.
First, you'll need to create a new folder for your extension and add a `manifest.json` file, which is the backbone of your extension and contains metadata, permissions, and functionality.
A `manifest.json` file requires a unique ID, a name, and a version number, which you can specify in the `manifest_version`, `name`, and `version` fields respectively.
To start building your extension, you'll need to create a `popup.html` file, which is the user interface of your extension. This file will contain the HTML, CSS, and JavaScript code for your popup.
Here's an interesting read: Html File Extension
Creating a Extension
To create a DevTools extension, you'll need to add the devtools_page field to your extension's manifest. This field must point to an HTML page, and it's recommended to specify it using a relative URL.
The page loaded within the DevTools window has access to the members of the chrome.devtools API, but content scripts and other extension pages don't. This means you can only use these APIs while the DevTools window is open.
An instance of the devtools_page is created for every DevTools window opened, and it can add other extension pages as panels and sidebars to the DevTools window using the devtools.panels API.
On a similar theme: Web Page
Extend Developer Tools
To extend developer tools, you can use the browser's API to access the DOM and manipulate the UI. This allows you to create custom tools that fit your specific needs.
The browser's API provides a way to interact with the web page, including getting and setting elements, attributes, and styles. You can use this API to create custom UI elements that display information specific to your tool.
You can also use the browser's storage API to store and retrieve data, which is useful for storing user preferences or other data that needs to be persisted across sessions. The storage API provides a way to store key-value pairs in the browser's local storage.
By using the browser's API, you can create custom tools that are tightly integrated with the browser and provide a seamless user experience. This can help to increase user adoption and engagement with your tool.
Explore further: Edit Html in Chrome
Examples
Creating a DevTools extension requires inspiration from existing examples. The Polymer Devtools Extension uses many helpers running in the host page to query DOM/JS state to send back to the custom panel.
You can learn from the React DevTools Extension, which reuses DevTools UI components through a submodule of Blink.
The Ember Inspector has a shared extension core with adapters for both Chrome and Firefox.
If you're looking for more examples, check out the DevTools Extension Gallery and Sample Extensions, which have more worthwhile apps to install, try out, and learn from.
Here are some notable DevTools extension examples:
- Polymer Devtools Extension
- React Devtools Extension
- Ember Inspector
- Coquette-inspect
UI Elements: Panels & Sidebar
A DevTools extension can add UI elements to the DevTools window, including panels and sidebar panes. These elements are in addition to the usual extension UI elements like browser actions, context menus, and popups.
Each panel is its own HTML file, which can include other resources like JavaScript, CSS, images, and more. This means you can create a basic panel using HTML code.
JavaScript executed in a panel or sidebar pane has access to the same APIs as the DevTools page. This is a powerful feature that allows you to interact with the inspected page in a variety of ways.
A different take: Page Unresponsive in Chrome Nextjs
There are several ways to display content in a sidebar pane. You can use HTML content, JSON data, or JavaScript expressions to display information in the pane.
Here are the three ways to display content in a sidebar pane:
- HTML content: Call setPage() to specify an HTML page to display in the pane.
- JSON data: Pass a JSON object to setObject().
- JavaScript expression: Pass an expression to setExpression(). DevTools evaluates the expression in the context of the inspected page, then displays the return value.
For both setObject() and setExpression(), the pane displays the value as it would appear in the DevTools console. However, setExpression() lets you display DOM elements and arbitrary JavaScript objects, while setObject() only supports JSON objects.
Communicating Between Components
Communicating between components is crucial for a seamless DevTools extension experience. It allows different components to exchange data and work together efficiently.
There are helpful ways to allow DevTools extension components to communicate with each other, such as the sections that describe these scenarios.
In typical scenarios, communicating between different components of a DevTools extension is essential.
What's Not Working?
If a DevTools extension isn't working, it's likely because it's not being maintained or is incompatible with the latest Google Chrome version. We can't offer extra support for unsupported extensions.
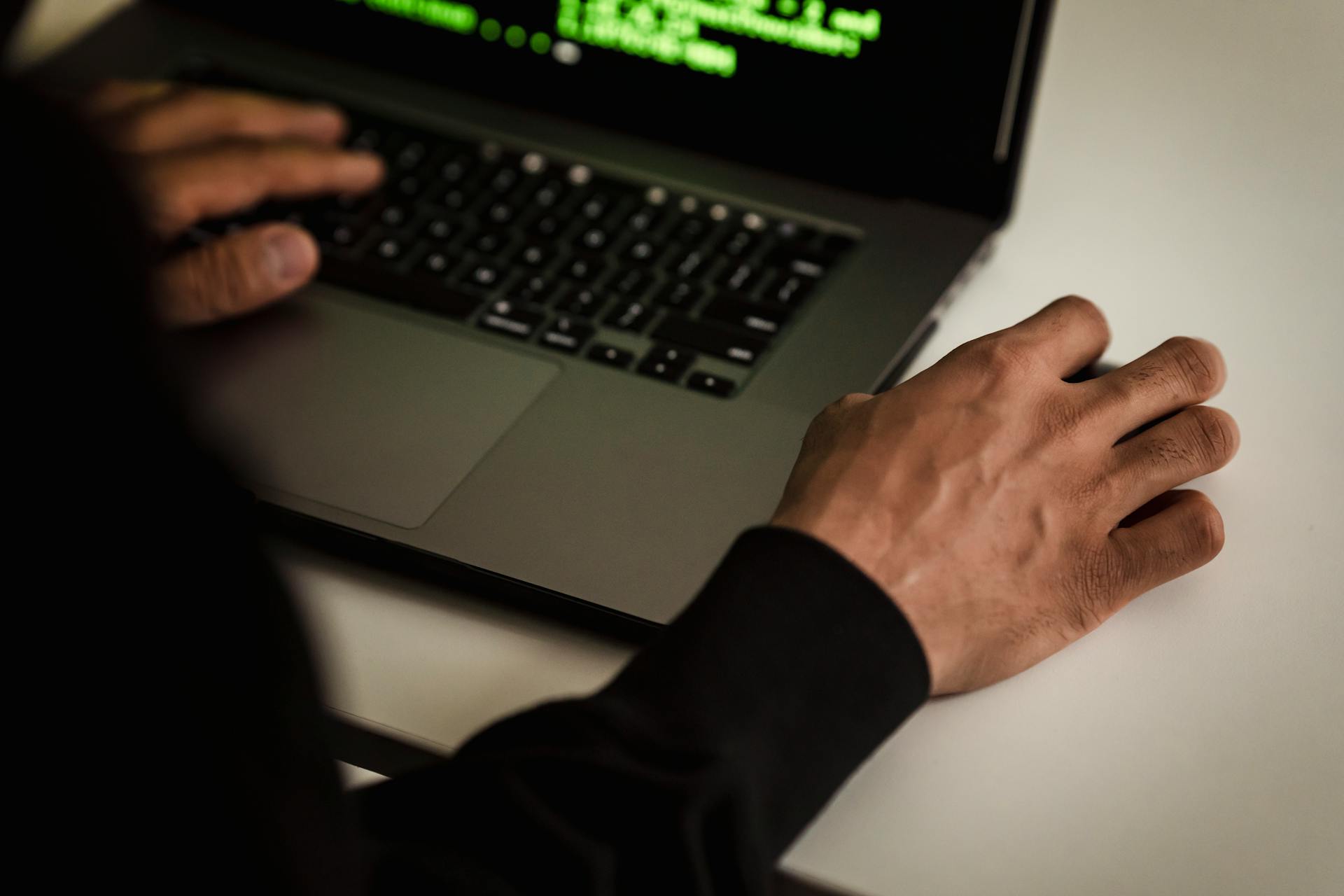
First, check if the extension is still supported and compatible with the latest Chrome version. If it's not, it's best to look for an alternative.
If the extension works on Chrome but not on Electron, it's time to file a bug in Electron's issue tracker. Describe which part of the extension isn't working as expected.
Communicating Between Components
Communicating between components is crucial for a seamless extension experience.
DevTools extension components can communicate with each other in various ways, as described in the sections that follow.
To allow extension components to communicate, you can use the methods outlined in the sections that describe helpful ways to do so.
In typical scenarios, components of a DevTools extension need to communicate with each other to function properly.
The sections that describe typical scenarios for communicating between components provide valuable insights into how this can be achieved.
To facilitate communication between components, you can refer to the sections that describe some typical scenarios for communicating between the different components of a DevTools extension.
Injecting Content Script
To inject a content script, you can use scripting.executeScript(). You can retrieve the tab ID of the inspected window using the inspectedWindow.tabId property.
If a content script has already been injected, you can use messaging APIs to communicate with it. This allows you to pass data back and forth between the content script and the DevTools page.
Here are the general steps to follow:
- Get the tab ID of the inspected window using inspectedWindow.tabId.
- Use scripting.executeScript() to inject the content script.
Note that you can't call tabs.executeScript directly from the DevTools page. Instead, you must retrieve the tab ID and send a message to the background page, which then calls tabs.executeScript to inject the script.
If this caught your attention, see: Devtools Pause Page
Manually Loading
Manually loading an extension is a viable option if you don't want to use the tooling approach.
To load an extension in Electron, you need to download it via Chrome and then load it into your Session by calling the ses.loadExtension API.
You'll need to install the extension in Google Chrome first, which can be done by visiting the Chrome Web Store.
Recommended read: Devtools Failed to Load Sourcemap: Could Not Load Content
Finding the extension ID is the next step - it's a hash string like fmkadmapgofadopljbjfkapdkoienihi, which can be located on the chrome://extensions page.
The filesystem location used by Chrome for storing extensions varies depending on the operating system, but you can find it by navigating to the extensions directory.
Once you have the extension ID and filesystem location, you can load the extension into your Session by passing the location to the ses.loadExtension API.
Here's an example of how to do this in code:
```
const { app, session } = require('electron')
const path = require('node:path')
const os = require('node:os')
// on macOS
const reactDevToolsPath = path.join(
os.homedir(),
'/Library/Application Support/Google/Chrome/Default/Extensions/fmkadmapgofadopljbjfkapdkoienihi/4.9.0_0'
)
app.whenReady().then(async () => {
await session.defaultSession.loadExtension(reactDevToolsPath)
})
```
Note that loadExtension returns a Promise with an Extension object, which contains metadata about the extension that was loaded. This promise needs to resolve before loading a page.
Also, be aware that loadExtension cannot be called before the ready event of the app module is emitted, nor can it be called on in-memory (non-persistent) sessions.
Finally, loadExtension must be called on every boot of your app if you want the extension to be loaded.
Injecting a Content Script
Injecting a content script is a crucial step in extending the functionality of a web page. You can retrieve the tab ID of the inspected window using the inspectedWindow.tabId property.
To inject a content script, use scripting.executeScript(). This method allows you to execute a script in the context of the current tab.
If a content script has already been injected, you can use messaging APIs to communicate with it. This is a powerful feature that enables seamless interaction between your DevTools extension and the content script.
You can't call tabs.executeScript directly from the DevTools page. Instead, you must retrieve the ID of the inspected window's tab using the inspectedWindow.tabId property and send a message to the background page. From the background page, call tabs.executeScript to inject the script.
Here are the general steps to inject a content script:
- Retrieve the tab ID of the inspected window using inspectedWindow.tabId property.
- Send a message to the background page to inject the script.
- Call tabs.executeScript from the background page to inject the script.
By following these steps, you can successfully inject a content script and extend the functionality of a web page.
Evaluating JavaScript in the Inspected Window
Evaluating JavaScript in the inspected window allows you to execute code in the context of the inspected page.
You can use the inspectedWindow.eval() method to do this, which is the same as typing code into the DevTools console. This method uses the same script execution context and options, giving you access to DevTools Console Utilities API features.
By default, the expression is evaluated in the context of the main frame of the page. You can also set the useContentScriptContext to true when calling inspectedWindow.eval() to evaluate the expression in the same context as content scripts.
To use this option, you need to load a context script before calling eval, either by calling executeScript or by specifying a content script in the manifest.json file. This allows you to inject additional content scripts once the context script context exists.
The eval method is powerful but can be dangerous if used inappropriately, so use it with caution. If you don't need access to the JavaScript context of the inspected page, you can use the tabs.executeScript method instead.
Recommended read: Chrome Browser Console
Passing Selected Element
You can pass the selected element to a content script using the inspectedWindow.eval() method. This method allows you to execute code in the context of the DevTools console and command-line APIs.
To do this, you need to create a method in the content script that takes the selected element as an argument. This method will be called from the DevTools page.
The method name is arbitrary, but it's common to use a name like setSelectedElement. The key is that it takes the selected element as an argument.
To call this method, you use inspectedWindow.eval() with the useContentScriptContext: true option. This option specifies that the expression must be evaluated in the same context as the content scripts, so it can access the setSelectedElement method.
Here's a step-by-step guide to passing the selected element to a content script:
- Create a method in the content script that takes the selected element as an argument.
- Call the method from the DevTools page using inspectedWindow.eval() with the useContentScriptContext: true option.
The useContentScriptContext: true option is crucial here, as it allows the evaluated code to access the setSelectedElement method. Without it, you would get an error.
Reference Panel Window
To get a reference to a panel's window object, you'll need to use the panel.onShown event handler. This event handler is triggered when the panel is shown, and it provides a reference to the panel's iframe window.
You can then use this reference to call postMessage() from the devtools panel. This is a crucial step in communicating with the panel's window object.
In fact, to postMessage from a devtools panel, you'll need a reference to its window object. This is why getting a reference to the panel's window is so important.
Here are the ways to get a reference to a panel's window object:
- panel.onShown event handler
Remember, the panel.onShown event handler is the key to getting a reference to the panel's window object.
Messaging
Messaging is a crucial aspect of Chrome DevTools extensions. You can send messages from injected scripts to the DevTools page using the window.postMessage() method, which is a recommended approach.
Combining an injected script with a content script can act as an intermediary, allowing messages to flow from the injected script to the background script and finally to the DevTools page.
To pass messages to the content script, you can use the window.postMessage API. This is a different strategy than using runtime.sendMessage, which won't work for injected scripts.
Here are two alternative message passing techniques:
In some cases, you may need to pass the selected element to a content script. To do this, you can use the inspectedWindow.eval() method with the useContentScriptContext: true option.
To pass the selected element to a content script, you can create a method in the content script that takes the selected element as an argument and call it from the DevTools page using inspectedWindow.eval().
For more insights, see: How to Inspect Element on Discord
Detecting Open and Close Times
To track whether the DevTools window is open, add an onConnect listener to the service worker and call connect() from the DevTools page.
Each tab can have its own DevTools window open, so you might receive multiple connect events. This can make it tricky to track whether any DevTools window is open.
Related reading: How to Open Devtools Chrome
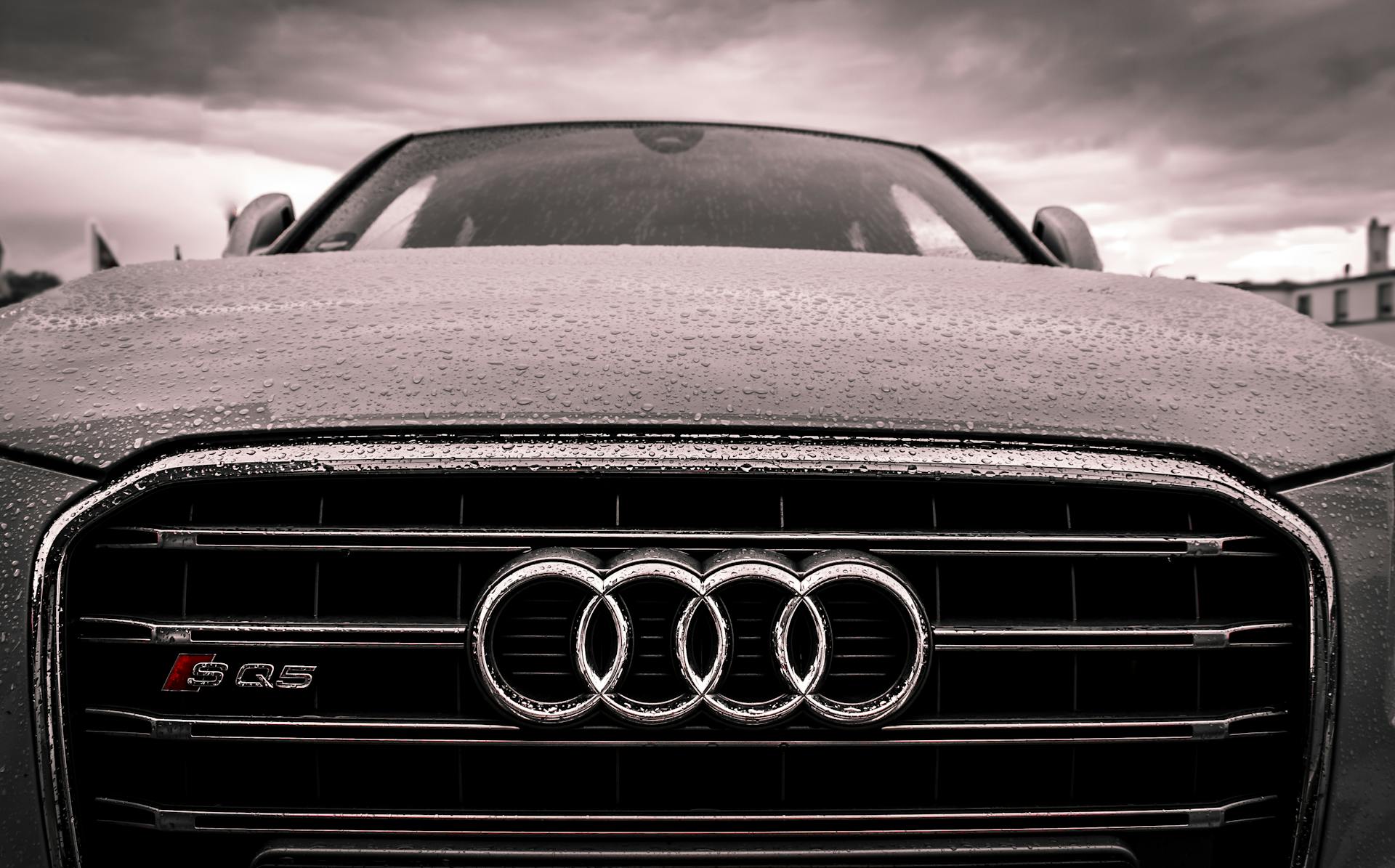
The DevTools page creates a connection by calling connect. You need to count the connect and disconnect events to track whether any DevTools window is open.
Since each tab can have its own DevTools window open, you may receive multiple connect events. This means you need to keep track of each tab's connection status.
To track whether any DevTools window is open, count the connect and disconnect events. This will give you a clear picture of when the DevTools window is open or closed.
A unique perspective: How to Open Html File in Chrome
Frequently Asked Questions
What is the DevTools extension for Chrome?
Chrome DevTools is a built-in set of web developer tools that helps you build better websites by editing pages on-the-fly and diagnosing problems quickly. It's a powerful tool for web developers to create and refine websites efficiently.
How to install DevTools extension?
To install the DevTools extension, download and install it in Google Chrome, then locate the extension's filesystem location in %LOCALAPPDATA%\Google\Chrome\User Data\Default\Extensions. Use the ses.loadExtension API to pass this location to load the extension.
Sources
- https://developer.chrome.com/docs/extensions/how-to/devtools/extend-devtools
- https://chromewebstore.google.com/detail/react-developer-tools/fmkadmapgofadopljbjfkapdkoienihi
- https://developer.chrome.com/docs/extensions/mv2/devtools
- https://electronjs.org/docs/latest/tutorial/devtools-extension
- https://developer.mozilla.org/en-US/docs/Mozilla/Add-ons/WebExtensions/Extending_the_developer_tools
Featured Images: pexels.com