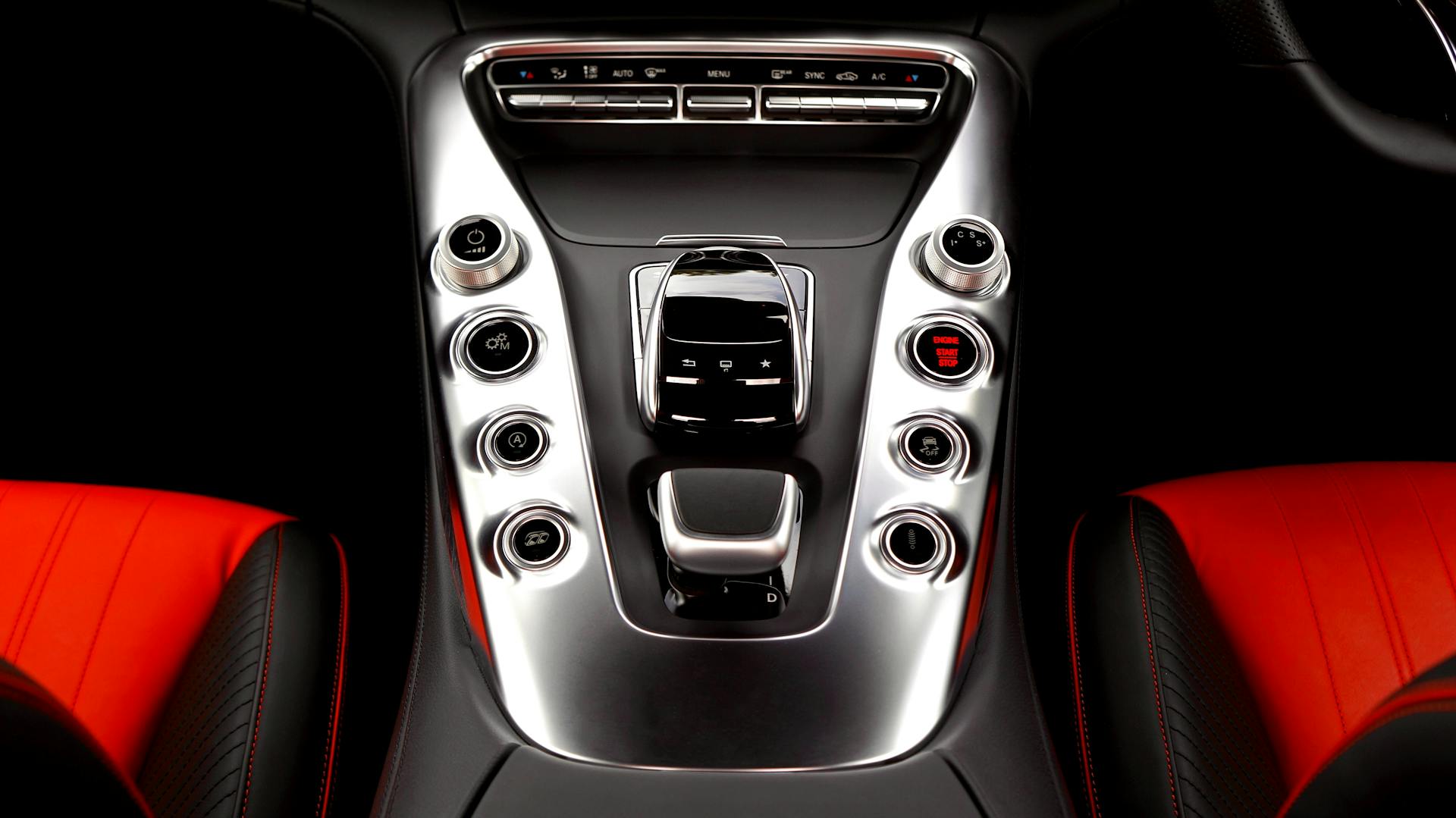
The Chrome Browser Console is a powerful tool that allows you to inspect and debug web pages.
It's accessed by pressing F12 or right-clicking on a page and selecting "Inspect" or "Inspect element".
The Console is divided into several panels, including the Elements panel, which allows you to inspect the HTML and CSS of a page.
The Console itself is where you'll find error messages, warnings, and other output from your code.
To use the Console effectively, you'll need to understand how to navigate its various features and tools.
For another approach, see: Page Unresponsive in Chrome Nextjs
Console Navigation
The Chrome browser console is a powerful tool for debugging and testing web applications. It's divided into several panels, including the Elements, Sources, and Console panels.
The Console panel is where you'll spend most of your time, as it's where you can execute JavaScript code, view errors, and inspect the console's output. You can also use the Console panel to debug your code by setting breakpoints and stepping through your code line by line.
Related reading: How to Run Code in Browser Console
To navigate the Console panel, you can use the keyboard shortcuts, such as Ctrl + Shift + I (Windows/Linux) or Command + Option + I (Mac) to open the console, and Ctrl + Shift + E (Windows/Linux) or Command + Shift + E (Mac) to focus on the Elements panel.
Intriguing read: Linux Console Web Browser
Watch and Breakpoints
In the Watch and Breakpoints section, you can see a list of watch expressions and breakpoints you've added to your code. This is shown in the right-hand pane of your debugger.
The Watch expressions section lists the variables you've added, such as the listItems variable in the example. You can expand the list to view the values in the array.
Breakpoints are listed in the Breakpoints section, and in the example, a breakpoint has been set on the statement listItems.push(inputNewItem.value);.
The Call stack section shows you what code was executed to get to the current line, including the function that handles a mouse click. The code is currently paused on the breakpoint.
The Scopes section shows what values are visible from various points within your code. For example, in the image, you can see the objects available to the code in the addItemClick function.
You can access the console in any browser by opening the developer tools and selecting the Console tab.
Here are the debuggers available on different browsers:
- Firefox JavaScript Debugger
- Chrome Debugger (Opera and Edge's debugger is the same)
- Safari Sources tab
In the console, you can try entering snippets of code to see what happens. For example, try entering the following code into the console one by one and pressing Enter:
Browser Tools
Browser Tools are a must-have for any web developer. You can use them to debug your web applications and achieve a lot through the developer console.
Some of the console methods you can use for debugging include the ones you've learned about in this post. You'll find that you use some of these methods more than others.
Browser Developer Tools offer a lot of useful features, including dev tools tips and tricks from the Google Developers website. This is a great resource to check out for more information.
A unique perspective: Browser Developer Tools
Label
You can specify a label for your timeline recordings using console.timeline() and console.timelineEnd() methods. This label must be the same in both calls.
The label helps identify the new timeline in the Chrome developer tools. You can think of it as a bookmark or a title for your timeline.
The label is also used when manually adding events to the timeline with console.timeStamp() method. This method can be passed a label to help identify the marker in the developer tools.
In Chrome, the label is required for console.timeStamp() method, and it was previously called console.markTimeline().
A unique perspective: Browser Developer Tools Firefox
Open the
You can open the Console as a panel or as a tab in the Drawer. To open it as a panel, you'll need to follow the instructions provided in the feature reference.
The Console can be opened as a tab in the Drawer, giving you more flexibility in how you navigate your workspace.
To learn more about opening the Console in the Drawer, check out the feature reference for detailed instructions.
Recommended read: Browser Dev Console
Console Functions
The Console is a powerful tool in the Chrome browser that allows you to interact with the page you're inspecting. You can run JavaScript directly in the Console to try out new code or experiment with existing functions.
One of the most useful features of the Console is its ability to pause your code on specific functions, making it easier to debug and inspect your code. For example, you can use the `debug()` function to pause your code on the first line of a function like `hideModal`.
The Console also has a range of utility functions that make it easier to inspect a page, including `debug()`. These functions can be accessed through the Console Utilities API Reference.
You might like: Web Page
Dir(Object)
When you need a more detailed look at an object, use console.dir(). This method prints a JavaScript representation of the object to the console.
The console.dir() method is particularly helpful for examining HTML elements, as it displays the DOM representation of the element, unlike console.log() which shows an XML representation.
The console.dirxml() method is the opposite, printing the XML representation of an object.
This is useful when you want to see the XML structure of an object.
Error()
The `Error()` method is similar to `console.log()`, but it also prints a stack trace from where the method was called.
The output of `Error()` will be flagged as an error in the console, making it a useful tool for writing error handlers.
You can use `console.error()` to log errors with a stack trace, which can be really helpful for debugging.
This method takes one or more objects and prints them to the console, similar to `console.log()`.
Intriguing read: Console Log in Nextjs
Console Functions
The console.info() method is a handy way to log messages with an info flag, allowing you to filter log messages in the developer console.
You can use console.info() to log messages with an info flag, similar to console.log() but with an added layer of organization.
The console has a feature that allows you to run JavaScript, making it a REPL (Read-Eval-Print Loop) environment.
This means you can interact with the page you're inspecting and even modify it using JavaScript.
Explore further: Browser Javascript Console
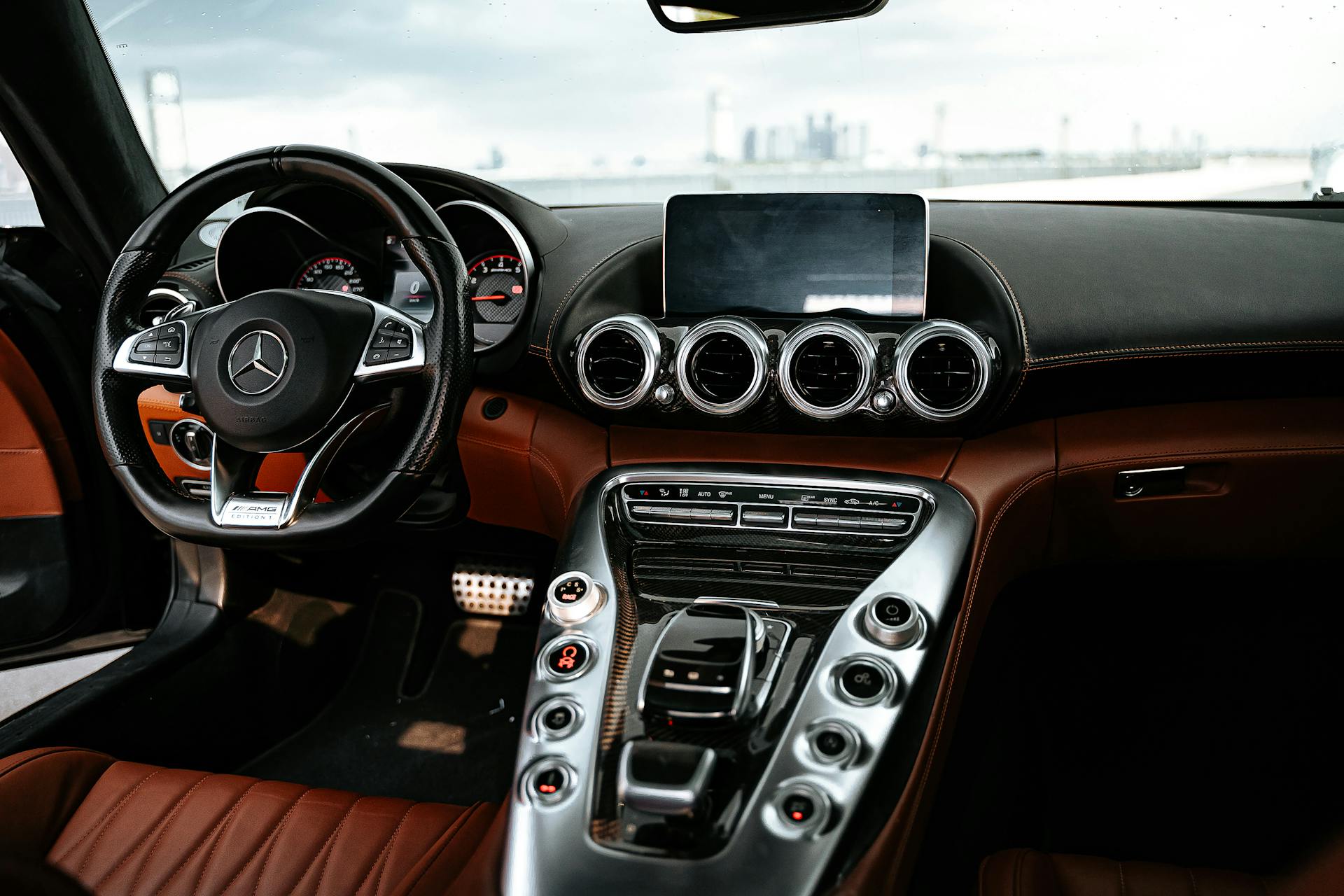
Running JavaScript in the console is possible because it has full access to the page's window, giving you a lot of power to experiment and learn.
You can use the console to try out new code that's not related to the page, like experimenting with the built-in JavaScript Array method map().
Additional reading: Devtools Pause Page
Console Grouping
Console Grouping is a powerful tool in the Chrome browser console that allows you to organize log messages into groups, making it easier to read and understand complex debugging information.
You can create a group with console.group() or console.groupCollapsed(), both of which allow you to specify an optional title to help identify the group.
To close a group, use the console.groupEnd() method, which will stop adding new messages to the group.
Group & GroupEnd()
Group & GroupEnd() is a powerful tool for organizing console messages. It allows you to group together a series of log messages with a title.
You can specify an optional title to make it easier to find the group in the console. This can be especially helpful when working on complex projects with many log messages.
The console.group() method is used to create a new group, and console.groupEnd() is used to close it. This is done by executing console.groupEnd() after you're done logging messages.
You can nest multiple groups within one another, which is useful for further organizing your log messages. This can be done by calling console.group() multiple times, creating a hierarchy of groups.
GroupCollapsed
The console.groupCollapsed() method is a variation of console.group() that initially displays groups collapsed in the console.
This method is useful for grouping log messages that you don't need to see immediately, but can still be useful for debugging purposes.
console.groupCollapsed() is essentially the same as console.group(), with the only difference being the initial display state of the group.
You can use multiple arguments with console.groupCollapsed() to group related log messages together.
This can help keep your console output organized and make it easier to find the information you need.
Console Timing
Console Timing is a powerful tool in the Chrome browser console. It allows you to measure the execution time of specific code blocks.
You can use the console.time() and console.timeEnd() methods to achieve this. Both methods should be passed the same label parameter, which will be used to identify the time measurement.
For example, you can use console.time("myCode") to start the timer and console.timeEnd("myCode") to stop it. This will give you a precise measurement of how long your code took to execute.
Profile End
The console.profileEnd() method is a crucial part of creating a JavaScript CPU profile. It completes the profile that was started with console.profile().
To stop a profile, you need to call console.profileEnd() after the code you want to profile has run. This will give you a detailed breakdown of the performance of your code.
console.profileEnd() is typically used in conjunction with console.profile() to create a comprehensive profile of your code's performance.
time(label) & timeEnd(label)
The console.time() and console.timeEnd() methods are super useful for timing how long it takes for a piece of code to execute. Both methods should be passed the same label parameter.
You can use these methods to give you a way of timing how long a piece of code takes to execute. This is especially helpful when you're trying to optimize your code and want to see where the bottlenecks are.
The label parameter is used to identify the specific piece of code you're timing. It's like a name tag for your timer. Both the time() and timeEnd() methods should be passed the same label parameter.
This allows you to keep track of multiple timers and see how long each piece of code takes to execute. It's like having a dashboard for your code's performance.
Console Viewing
You can view logged messages in the Chrome browser console to see what's going on with your JavaScript code. This is a great way to make sure everything is working as expected.
Web developers log messages for two main reasons: to check that code is executing in the right order, and to inspect the values of variables at a specific moment in time.
To log a message, you insert an expression like `console.log('Hello, Console!')` into your JavaScript code. This will display the message in the console.
You can log messages for debugging purposes, and it's a good idea to remove them before deploying your code to production. The main difference between console methods is how they display the data you're logging.
Web developers often log messages to the Console to make sure that their JavaScript is working as expected.
Frequently Asked Questions
How do I press F12 to open the browser console?
Press F12 or use the keyboard shortcut CTRL+SHIFT+J (CMD+OPT+J on Mac) to open the browser console
How do I open the browser console in Chrome?
To open the browser console in Chrome, press Option + ⌘ + J on macOS or Shift + CTRL + J on Windows/Linux. This will open the console within your existing Chrome window or in a new one.
What is the command for the console in Chrome?
To open the Console panel in Chrome, press Control + Shift + J on Windows or Command + Option + J on a Mac. This shortcut allows you to access Chrome's debugging tools and inspect web page issues.
Sources
- https://developer.mozilla.org/en-US/docs/Learn/Common_questions/Tools_and_setup/What_are_browser_developer_tools
- https://blog.teamtreehouse.com/mastering-developer-tools-console
- https://support.google.com/chrome/a/answer/14989974
- https://www.digitalocean.com/community/tutorials/how-to-use-the-javascript-developer-console
- https://developer.chrome.com/docs/devtools/console
Featured Images: pexels.com