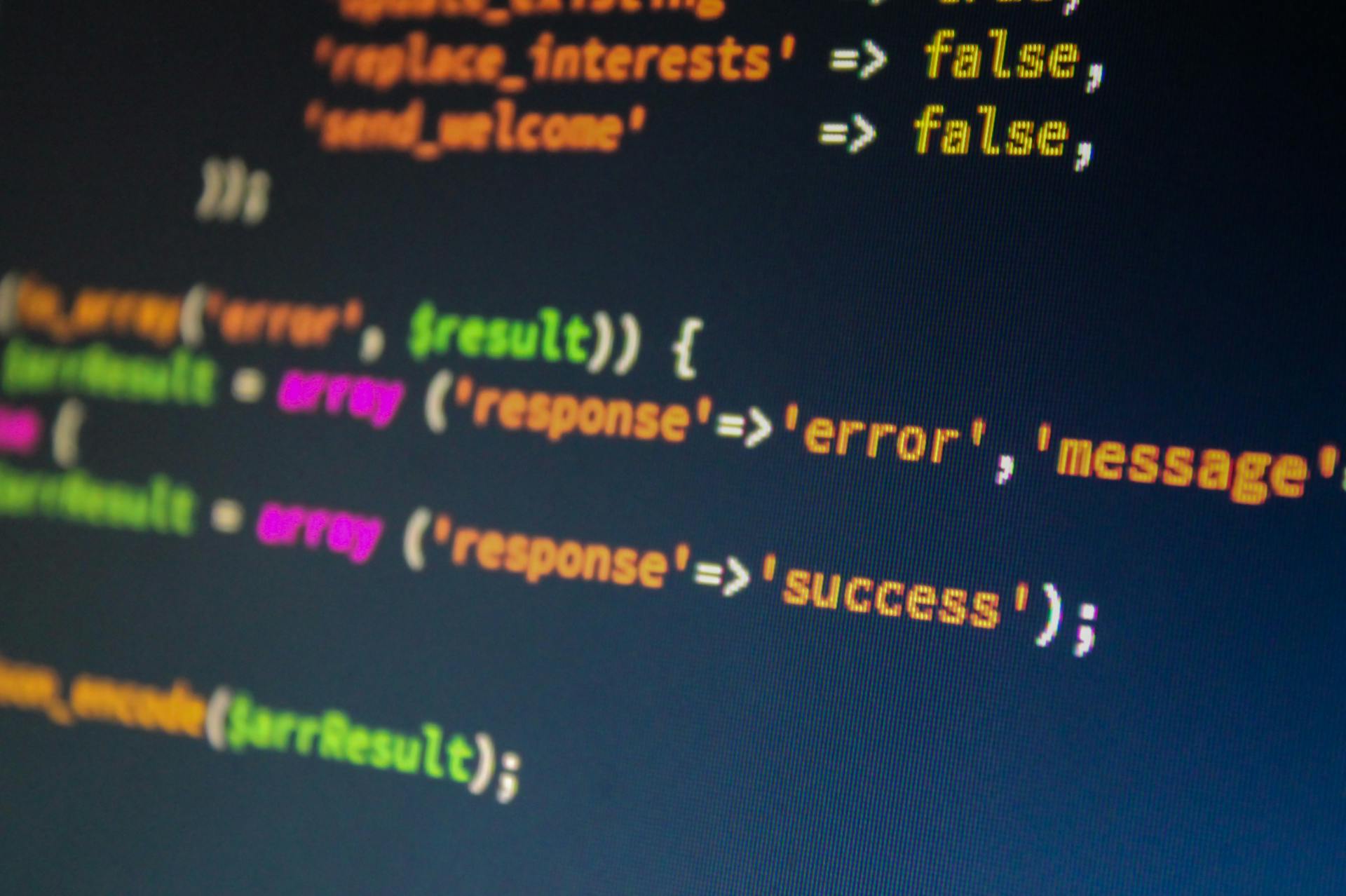
Browser console JavaScript logging and debugging is a crucial skill for any web developer.
The browser console is a powerful tool for debugging JavaScript code, and it's accessible in most modern browsers.
To log messages in the console, you can use the `console.log()` method, which is widely supported across browsers.
For example, `console.log('Hello World!');` will print the string 'Hello World!' in the console.
You can also use `console.error()` to log error messages, which can be useful for identifying and fixing issues in your code.
Suggestion: No Code Html Editor
Console Basics
Console Basics are essential for any JavaScript developer. You can use the Console web API to log messages into the browser's console. JavaScript is the universal language of web applications, and you can use it to log messages.
To log messages, you can use the following console methods: console.log: outputs a message to the browser's consoleconsole.info: logs an informational message with a small "i" icon in Firefoxconsole.warn: logs a warning message in a distinctive color with an exclamation point icon in Chrome and Firefoxconsole.error: logs an error message in redconsole.debug: outputs a message at the debug log level, but it may be hidden if the console is configured to do so
You can also log messages by passing a relevant message and the value as comma-separated arguments to the log() method, or by wrapping the variable in curly brackets and passing it to the log() method.
Additional reading: Inspect Element Firefox
Logging Basics
You can use the Console web API to log messages into the browser's console. JavaScript is the universal language of web applications.
The Console web API has several methods for logging messages, including console.log, console.info, console.warn, console.error, and console.debug.
Console methods display data differently: console.log outputs a message, console.info shows a small "i" icon, console.warn displays a distinctive color and an exclamation point icon, console.error displays a message in red, and console.debug outputs a message that may be hidden if the console is configured to hide debug messages.
You can log complex data structures as tabular data into the console using the console.table() method.
Here are some common console methods and their uses:
These methods can help you understand where values come from and what they relate to by providing context.
Grouping Messages
You can group console messages contextually so they appear together, making it easier to understand the flow of your code. This can be especially helpful when debugging an issue.
The JavaScript console API provides two ways to group messages: console.group and console.groupEnd. You can create as many groups as you want, but remember to provide a unique contextual message to the console.group() method for every group, so you can identify them without confusion.
console.group() creates an inline group of console messages, and all subsequent messages will be indented by a level until you call console.groupEnd(). This helps keep your log messages organized and easier to read.
Here are the two methods:
- console.group: creates an inline group of console messages
- console.groupEnd: helps order the current group of messages
You can also use console.groupCollapsed() to create a group, but in this case, the newly created group will be collapsed by default.
$: Query All
The $ utility command is like a superpower for selecting DOM elements. It works just like document.querySelector() and returns the reference to the first DOM element with the specified CSS selector.
You can use the $ command to select a specific element, like an image, and get a reference to it. This is useful when you need to access a single element on the page.
The $$ utility command is similar but returns an array of elements that match the CSS selector. This is helpful when you need to work with multiple elements at once.
If you need to select all the image elements on the page, the $$ command is the way to go. It's like a shortcut to getting all the images in one go.
Logging
Logging is a crucial part of web development, and JavaScript makes it easy with its Console API.
You can use the Console API to log messages into the browser's console, which is useful for debugging and troubleshooting your code. The most basic method is console.log(), which outputs a message to the browser's console.
There are several other methods, including console.info(), console.warn(), console.error(), and console.debug(). Each method has a specific purpose, such as logging informational messages, warnings, or errors.
For example, console.log() is used for general logging, while console.error() is used for logging error messages. You can also use console.info() to log informational messages, and console.warn() to log warning messages.
Intriguing read: Console Log in Nextjs
Here are the different console methods:
You can also use console.log() to log a message with a specific format, such as logging a user's name and value. For example, console.log("The user name is", atapas) will output "The user name is atapas".
It's also a good practice to provide context to your logs, so you can easily understand what the value represents. You can do this by passing a relevant message and the value as comma-separated arguments to the log() method.
Custom Logs
Custom logs are a powerful way to make your console logs stand out. You can add styling to your logs using CSS.
To do this, you simply add the CSS styling as a parameter to the logs, replacing %c in the logs. This will give you custom logs that are easy to read and understand.
You can also pass a relevant message and the value as comma-separated arguments to the log() method. This will output a concatenated message, which may look better than simply logging the value.
Discover more: Jquery Add Css Class
For example, you can log a user's name by passing the name and a message to the log() method, like this: console.log("The user name is", atapas). This will output "The user name is atapas".
Alternatively, you can wrap the variable in curly brackets and pass it to the log() method. This will output a JavaScript object, with the key as the variable name and the value in a console message.
Here are some examples of how to use custom logs:
Note that you can use these methods to log different types of messages, and customize the output to suit your needs.
Frequently Asked Questions
How to run JavaScript in browser console?
To run JavaScript in the browser console, right-click in a webpage and select Inspect, or use a keyboard shortcut like Ctrl+Shift+J (Windows/Linux) or Command+Option+J (macOS). This will open the DevTools console where you can enter and execute JavaScript statements and expressions.
How to open browser JavaScript console?
To open the browser JavaScript console, press Ctrl+Shift+J on Windows or Linux, or Cmd+Opt+J on Mac. This will allow you to view and interact with the console.
Featured Images: pexels.com