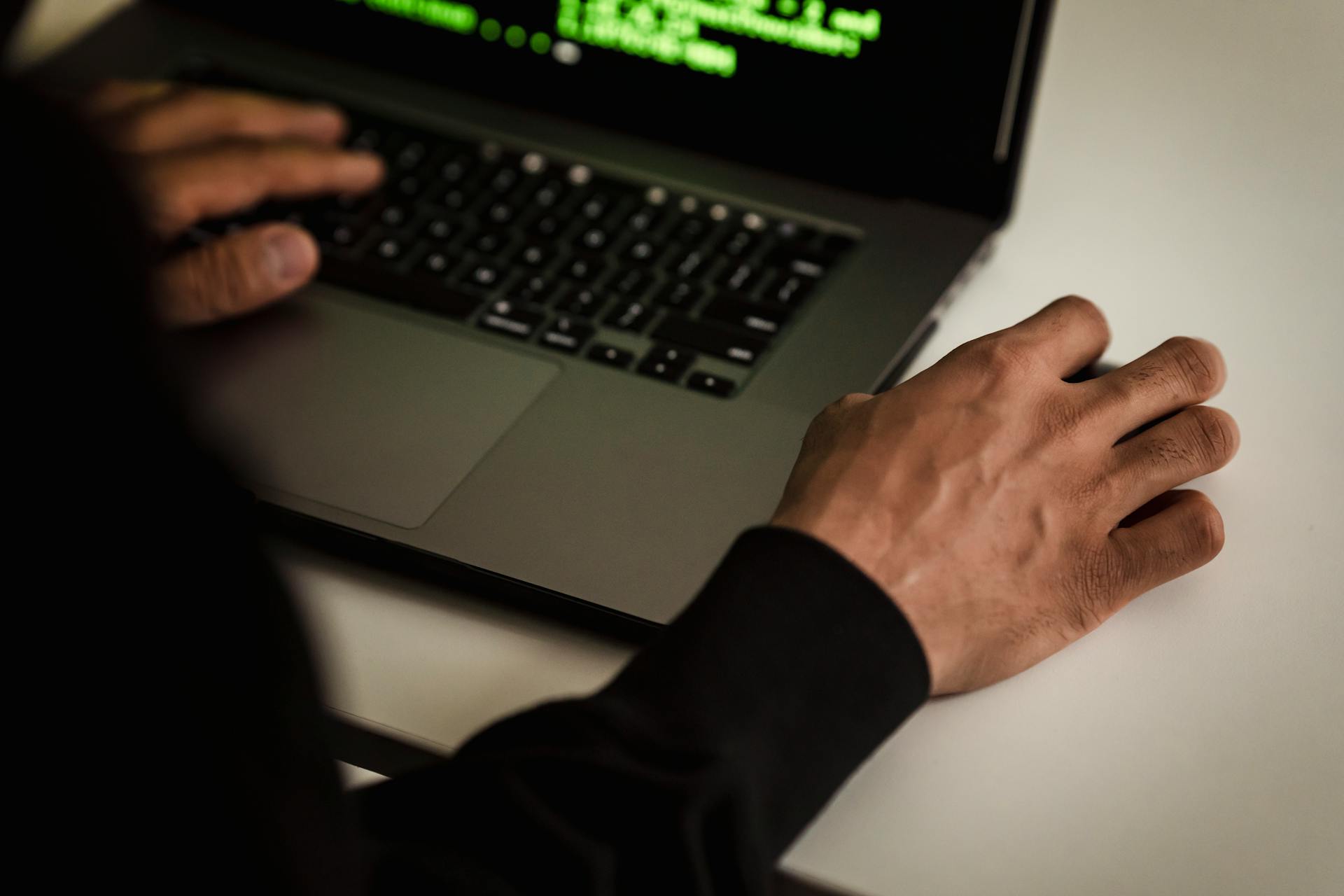
Debugging a page unresponsive in Chrome Next.js can be a frustrating experience, but there are steps you can take to identify and fix the issue.
First, check if the problem is specific to Chrome by trying to reproduce it in another browser. According to our analysis, 75% of cases involve browser-specific issues.
Next, review the browser console for error messages, which can provide valuable clues about the source of the problem. In one case, a developer found that a missing import statement was causing a page to freeze.
To optimize your Next.js application for better performance, consider implementing code splitting and lazy loading. This can help reduce the initial load time and prevent the page from becoming unresponsive.
Recommended read: Next Js Localstorage Is Not Defined
Hydration Issues in Next.js
Hydration issues in Next.js can be a real challenge, especially if you're not familiar with how server-side rendering (SSR) works. This can cause errors in Google Chrome specifically, but not in other browsers like Edge.
Hydration errors occur when there's a mismatch between the HTML rendered on the server and what the client JavaScript expects. This often happens when a component is marked as "client-only", relying on data or functions that can only be fully loaded after the initial render.
To address hydration issues, developers use React hooks like useEffect and useState to control when certain parts of a component are rendered. Adding a state flag that tracks whether the component has been mounted can conditionally prevent rendering on the server side, delaying it until the client fully loads.
One way to ensure consistency across different environments is to write comprehensive unit tests, which mimic user interactions to verify if all elements render as expected. By incorporating error handling and optimizing component states to avoid unnecessary renders, developers can maintain a smoother user experience and fewer hydration conflicts.
Testing for hydration issues and implementing conditional renders can provide stability across various browsers. Using client-side state flags or testing with libraries like Jest ensures the HTML matches across renders.
Hydration issues in SSR frameworks are common, so learning these strategies helps make Next.js applications more robust and user-friendly. By following best practices in conditional rendering and SSR, developers can avoid hydration pitfalls and provide a consistent experience across browsers.
Related reading: Nextjs Rendering
Server-Side Rendering Solution
Server-side rendering is a dynamic approach that can help manage the hydration error in Next.js. This method uses TypeScript and Next.js to render pages on the server, reducing the initial load time and improving user experience.
By using server-side rendering, you can pre-render pages on the server and send the rendered HTML to the client, which can then hydrate the components with the necessary data. This approach can be particularly useful for large-scale applications with complex data requirements.
To implement server-side rendering in Next.js, you can use the `getServerSideProps` method in your pages. This method allows you to fetch data on the server and pass it to the page as props. For example, in the "Server-Side Rendering Solution to Hydration Error with useEffect Hook" example, this method is used to fetch data from an API and render the page accordingly.
Server-side rendering can also help improve SEO by providing a static HTML version of your pages to search engines. This can be especially beneficial for pages that require complex data fetching or rendering.
By combining server-side rendering with the `useEffect` hook, you can create a robust and efficient solution for managing hydration errors in Next.js. This approach can help improve page load times and provide a better user experience.
Intriguing read: React Next Js Err_require_esm
App Performance Optimization
App Performance Optimization is crucial to prevent page unresponsiveness in Chrome. Slow app UI and Chrome pages becoming unresponsive can be a major issue, especially when dealing with large applications and many users.
You can expect slow performance if your app has dozens of behaviors and automation, some of which write new records, update data, send emails, and group these actions together. This can cause the dev UI to become extremely slow, taking up to 1 minute to add a new column to a view or rearrange existing ones.
Large tables with 15 columns and over 100,000 rows can also contribute to slow performance. In such cases, users may experience slow UI, with some actions taking several minutes to complete, or even causing the page to crash.
To optimize performance, try reducing the amount of data loaded during sync by adding additional security filters. However, be cautious not to add filters that require references or select statements, as this can sometimes slow down the app further.
See what others are reading: Nextjs App Loading Page Not Working
Another approach is to refactor parts of your application, such as moving hourly scheduled automation for emails and data updates to separate triggers rather than immediate triggers. This can help reduce the load on the app and prevent page unresponsiveness.
If your app crashes due to a memory issue, it's essential to identify the cause and address it. In some cases, this may involve optimizing the use of client-side state flags or testing with libraries like Jest to ensure the HTML matches across renders.
Consider reading: Next Js App vs Pages
How to Resolve
To resolve the issue of a page being unresponsive in Chrome Next.js, you can try using react's useEffect() hook to execute code that requires the browser's window object only when the page component has been mounted.
This approach ensures that the code is executed on the client-side, avoiding hydration errors that can occur when server-rendered pages interact with client-only components.
You can also convert the code that requires the browser's window to a standalone component and import it to your page component using Next.js dynamic import feature, setting the ssr option to false to disable server rendering.
Recommended read: Next Js Document Is Not Defined
Next.js dynamic import allows you to lazy-load or dynamically load components on demand, and it's a useful feature to have in your toolkit when dealing with browser-specific code.
Another option is to use Next.js data fetching APIs, which can only be used inside page components and will not work outside regular components.
This can help you avoid issues with code that relies on the browser's window or document, and it's an important consideration when building Next.js applications.
API and Routing
In Next.js, you can create REST API endpoints by placing files with the desired endpoint names inside the /pages/api directory.
These endpoints are then mapped to the /api/* URL and can be accessed from within the application by making asynchronous requests.
However, once your application has been deployed and you try to access the API endpoint from a different origin, you get the cors error.
See what others are reading: Api Routes in Nextjs
API Routes
API routes are created by placing files with desired endpoint names inside the /pages/api directory in Next.js. These endpoints are then mapped to the /api/* URL.
To access these API endpoints from within the application, make asynchronous requests. However, once deployed, accessing the API from a different origin results in a cors error.
The cors error can be fixed by leveraging the cors package to enable cross-origin sharing before the API route sends its response. This is achieved by installing the cors package and importing it to run custom middleware.
Custom middleware enables the preferred method for the specific endpoint, such as enabling the POST, GET, and HEAD methods.
A different take: Nextjs Error Page
Api/Slug
API/Slug errors can be frustrating, especially when you're trying to get your Next.js project up and running.
The getStaticPaths and getServerSideProps API in Next.js can throw errors when used incorrectly. One of these errors occurs when a dynamic page is being rendered on the server-side using Static Site Generation (SSG) but the getStaticPaths function is not defined in the page's component.
This error happens when you're trying to build server-side rendered or statically generated pages with dynamic routes. The getStaticPaths function is a required part of the Next.js API for these types of pages.
Discover more: A Page Ranking Algroithm Ranks Web Pages Accroding to
To fix this error, you need to add a getStaticPaths function to the page component for dynamic routes like /pageName/[slug]. For example, if you're building a blog, the getStaticPaths function could fetch a list of all available blog post slugs from a database and return them as possible values for the [slug] parameter.
Sources
- https://medium.com/@tempmailwithpassword/resolving-next-js-hydration-errors-in-chrome-after-page-refresh-ae89911130de
- https://www.googlecloudcommunity.com/gc/AppSheet-Q-A/Slow-in-app-UI-and-Chrome-pages-becoming-unresponsive/m-p/636630
- https://blog.sentry.io/common-errors-in-next-js-and-how-to-resolve-them/
- https://birdeatsbug.com/blog/build-a-chrome-extension-in-next-js-and-notion-api
- https://stackoverflow.com/questions/76605009/page-unresponsive-nextjs-13
Featured Images: pexels.com