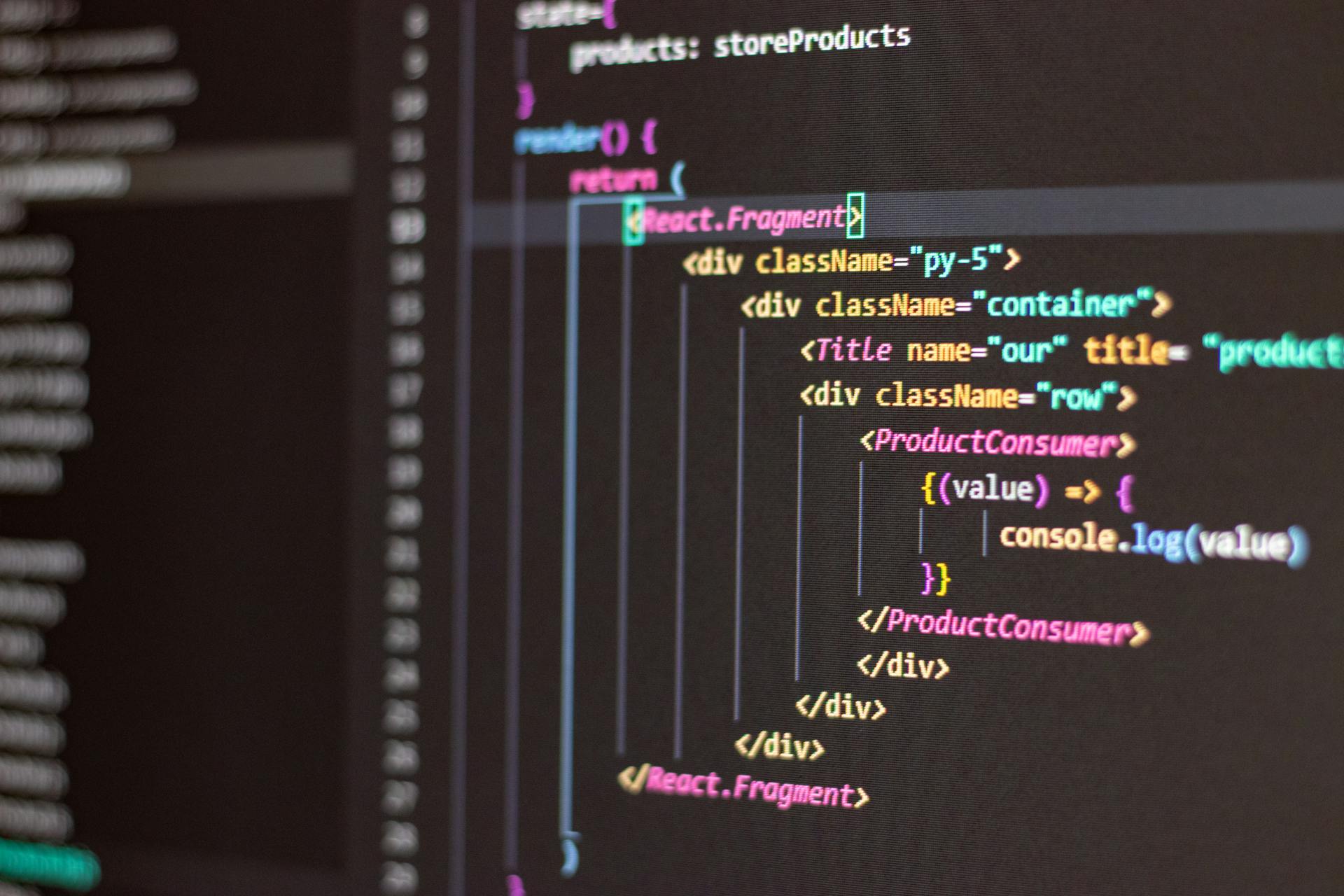
The infamous "Next Js Document Is Not Defined" error - a frustrating issue that can bring even the most seasoned developers to a halt. This error occurs when Next Js is unable to find the document object, which is a fundamental concept in Next Js.
The document object is crucial for Next Js to function properly, and its absence can lead to a range of problems, including failed page loads and errors.
In Next Js, the document object is typically defined in the _document.js file, which is a special file that Next Js uses to render the HTML document. Without this file, Next Js will throw an error.
If you're experiencing this issue, the first step is to check if you have a _document.js file in your project.
What is the Error?
The "document is not defined" error in Next.js is a common issue that occurs when your code tries to access the document object during server-side rendering. This happens because Next.js uses Node.js for server-side rendering, and Node.js doesn't have access to document.
The document object is part of the browser's Window interface and represents the DOM (Document Object Model) of the current web page. This means that when Next.js renders pages on the server, there is no browser environment, so the document object is not defined.
This error typically occurs when code that references the document is executed on the server, especially in the component's render method or lifecycle methods. It's a disconnect between the client-side and server-side environments that can cause frustration for developers.
Preliminary Checks
Before you start troubleshooting, it's essential to perform some preliminary checks to ensure you're on the right track. Review your code to identify where you're accessing the document object, as it could be in lifecycle methods, outside components, or in third-party libraries.
Make sure the problematic code runs only on the client side by checking if you're in a browser environment with a simple if (typeof window !== 'undefined') check. This will help you determine if the issue is related to the client-side code.
Update your dependencies, including Next.js and other related packages, to ensure you're running the latest versions. Outdated dependencies can sometimes cause issues, so it's crucial to stay up-to-date.
Here are some key areas to check in your code:
- Lifecycle methods
- Outside components
- Third-party libraries
Basic Troubleshooting
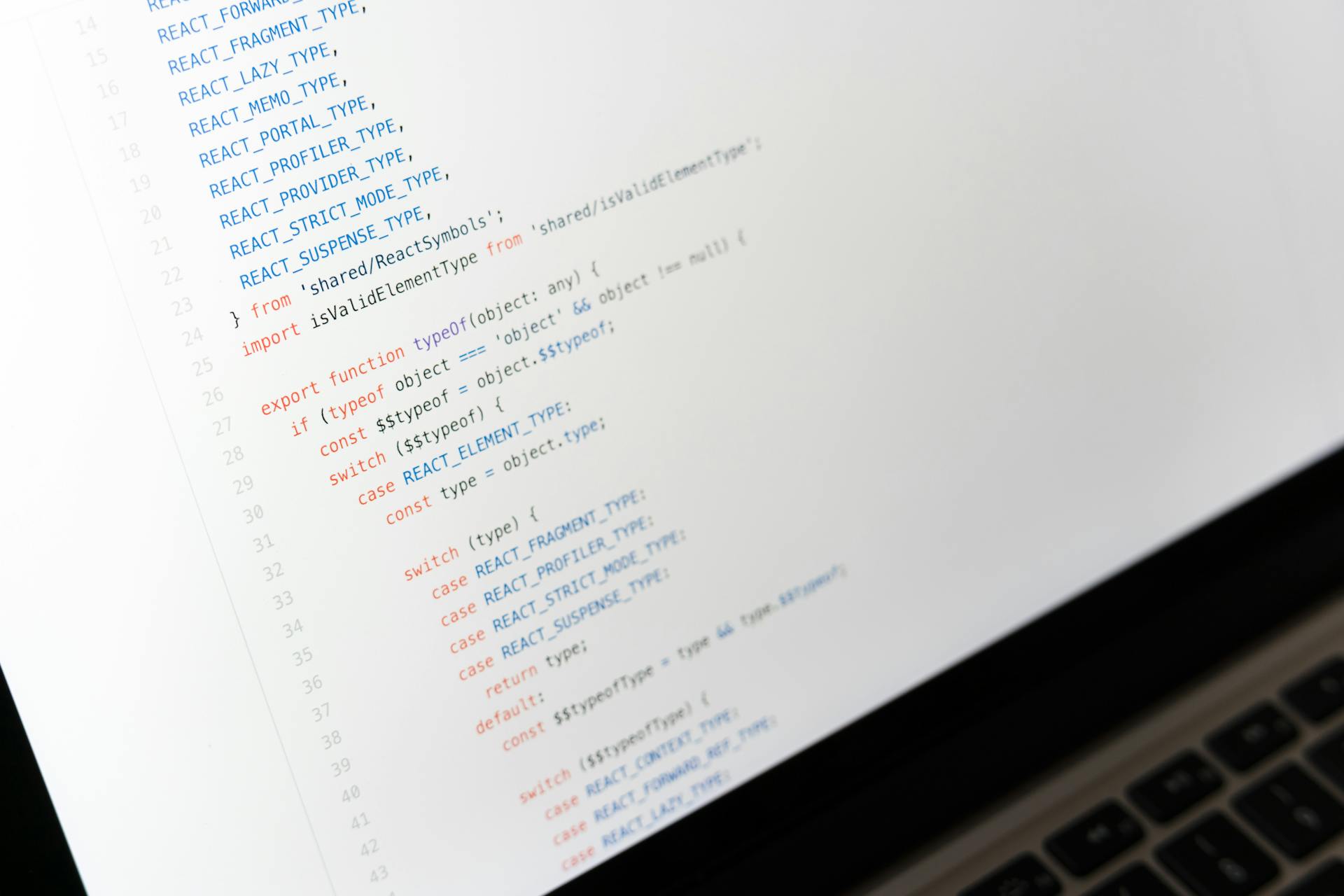
Check the power cord and ensure it's properly connected to both the device and the power source. A loose connection can cause a device to malfunction.
Verify that the device is turned on and that the power button is functioning correctly. This might seem obvious, but it's surprising how often it's overlooked.
Check for any blown fuses or tripped circuit breakers. This can be a common issue, especially in older homes or buildings with outdated electrical systems.
Make sure all cables and connections are secure and not damaged. A loose or damaged cable can cause a device to malfunction or not work at all.
Check the device's manual or online documentation for troubleshooting tips specific to the device. Sometimes, the solution is as simple as resetting the device or restarting it in a different mode.
Testing and Validation
Testing and Validation is a crucial step in ensuring the accuracy and reliability of your preliminary checks. It's like double-checking your math homework to make sure you got the answers right.
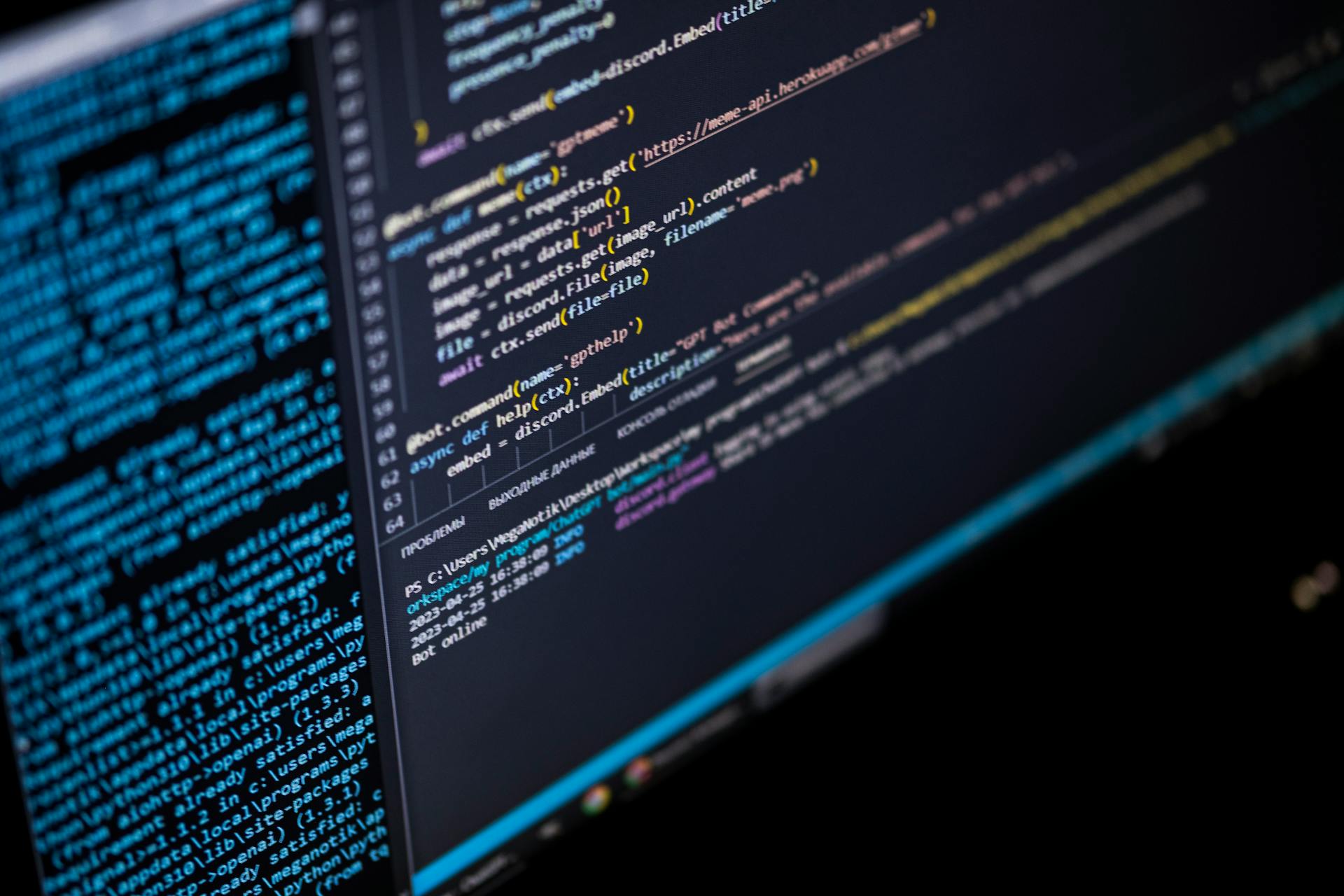
You've already identified potential issues with your data, such as missing or duplicate entries. Now, it's time to verify that your assumptions are correct and your findings are consistent.
To start, review your data for any inconsistencies or errors. For example, if you're checking for missing values, make sure to account for any null or blank entries. This will help you avoid making incorrect conclusions.
Use statistical methods to validate your results, such as comparing your findings to known benchmarks or using regression analysis to identify trends. This will give you a better understanding of the underlying patterns and relationships in your data.
Remember, testing and validation is an ongoing process that requires continuous monitoring and refinement. By regularly reviewing and updating your preliminary checks, you'll be able to identify and address any issues before they become major problems.
When Does the Error Occur?
The "document is not defined" error in Next.js can be a bit tricky to understand, but it's actually quite straightforward. This error occurs when your code tries to access the document object during server-side rendering.
The document object is part of the browser's Window interface and represents the DOM (Document Object Model) of the current web page. This means that when Next.js renders pages on the server, there is no browser environment, so the document object is not defined.
This error typically occurs when you're using code that references the document object in a component's render method or lifecycle methods. It's not a matter of whether your code is correct or not, but rather that it's being executed in the wrong environment.
Here are some scenarios where this error might occur:
Remember, this error is not unique to your code, but rather a result of the way Next.js handles server-side rendering.
Steps for Fixing
Fixing the "document is not defined" issue in Next.js can be a real challenge. But don't worry, I've got you covered.
To start, you need to ensure that code accessing the document object only runs after the component mounts on the client side. This is where the useEffect hook comes in - it's a game-changer for client-side code.
One of the most common mistakes is trying to access the document object before it's available. So, always perform a conditional check to ensure it exists before trying to use it. This simple step can save you a lot of headaches.
Here are some key steps to follow:
- Use the useEffect hook for client-side code.
- Perform conditional checks for the document object.
- Dynamically import components that rely on the document object.
- Avoid direct DOM manipulation in SSR (Server-Side Rendering).
- Use conditional rendering to ensure code runs in the correct context.
By following these steps, you'll be well on your way to fixing the "document is not defined" issue in Next.js. Remember, it's all about being mindful of the environment and using the right tools for the job.
Sources
- https://codedamn.com/news/nextjs/how-to-fix-document-is-not-defined-in-next-js
- https://www.geeksforgeeks.org/how-to-fix-next-js-document-is-not-defined/
- https://blog.sethcorker.com/question/how-to-solve-referenceerror-next-js-window-is-not-defined/
- https://next-auth.js.org/errors
- https://stackoverflow.com/questions/60629258/next-js-document-is-not-defined
Featured Images: pexels.com