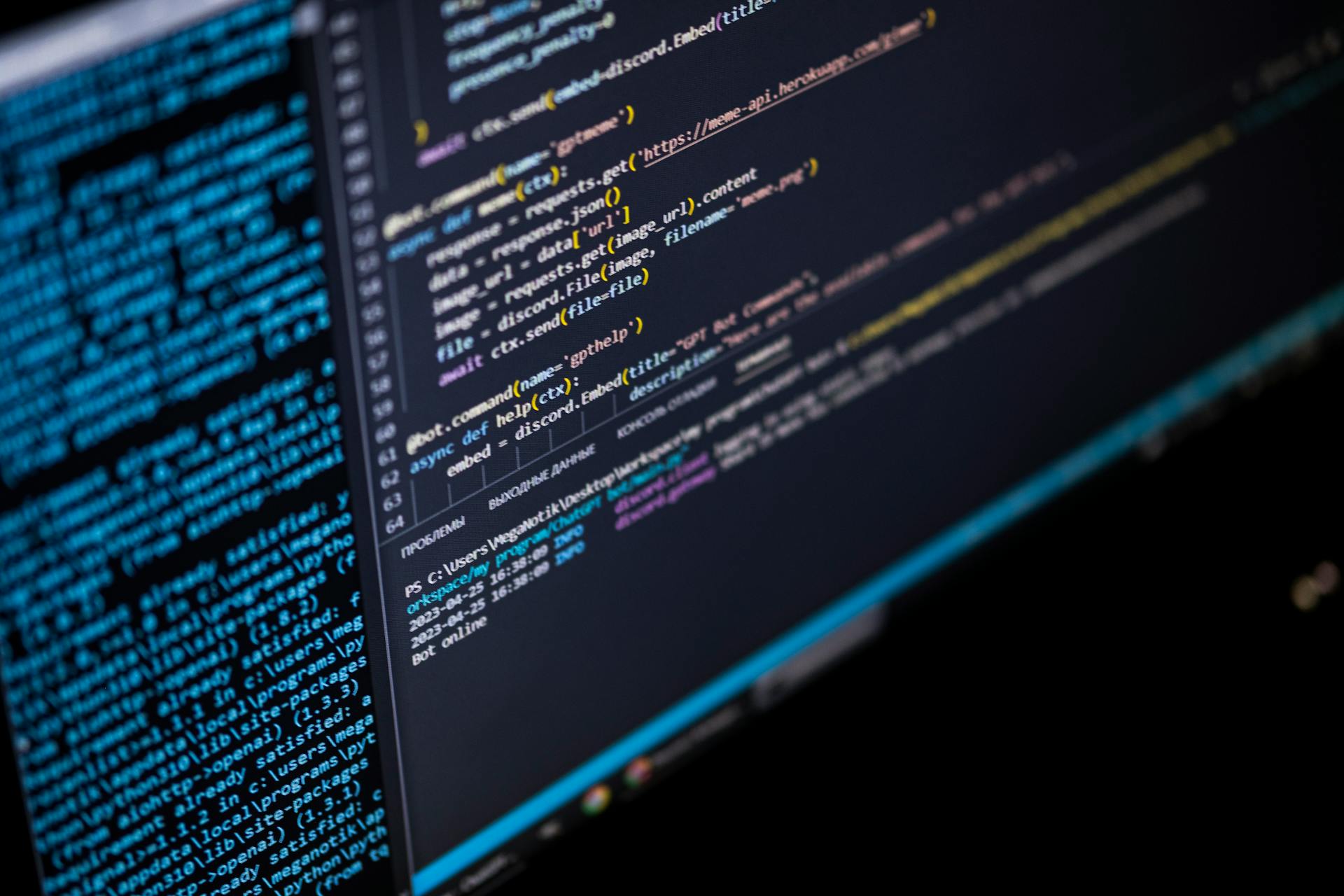
The most common cause of the "window is not defined" error in Next.js is the use of server-side rendering (SSR) in a browser environment. This occurs because the browser doesn't have a window object available on the server.
To solve this issue, you can use the `useEffect` hook to wait for the window object to be available before executing certain code. This is demonstrated in the example where the `useEffect` hook is used to wait for the window object to be available before calling the `fetch` function.
Another solution is to use the `typeof window` check to determine whether the window object is available before attempting to access it. This approach is shown in the example where the `typeof window` check is used to determine whether to call the `fetch` function or not.
Here's an interesting read: The Azure Window in Malta
The Problem
In Next.js version 13+, you may have encountered the error when trying to use the browser API window object.
This error occurs because components are server-rendered by default, meaning they're pre-rendered into HTML on the server before being sent to the client.
The window object is not available on the server, which causes the error.
Be careful about using this method as it can cause hydration errors if it causes a difference between the HTML that’s pre-rendered on the server and the HTML that’s first rendered on the client when the component is hydrated.
This error can be tricky to track down because it's inconsistent, and Next.js may render your app differently depending on how the user navigates to a given page.
The Solution
To fix the "window is not defined" issue in Next.js, you can use the "use client" directive in your component file, which will make it a client component. This will allow you to use the window object without any issues.
You can also use the useEffect hook to ensure that the code that uses the window object only runs on the client. Another option is to use an event handler or lazy load the component to achieve the same result.
To check if the window object is defined, you can simply do a check for the window object. This will help you prevent any errors that might occur when trying to access the window object on the server.
Readers also liked: Next Js Localstorage Is Not Defined
UseEffect to the Rescue
If you're dealing with the "nextjs window is not defined" issue, one potential solution is to use the useEffect hook.
Relying on useEffect can resolve this issue because hooks aren't run when doing server-side rendering.
Wrapping the usage of window inside a useEffect that is triggered on mount means the server will never execute it and the client will execute it after hydration.
This approach is convenient because it ensures that the code only runs on the client-side, avoiding any potential errors on the server.
Lazy Load Client Component
Lazy loading your client component can be a viable solution to the "window is not defined" issue in Next.js. This approach involves loading the client component only on the client-side, which can be achieved by disabling server-side rendering (SSR) and pre-rendering on the server.
By doing so, you ensure that the client component is only rendered on the client, making the window object accessible. However, keep in mind that this may make the initial page load slower.
This approach is particularly useful when using client-only libraries or when you only need to load the client component after a certain user action.
If this caught your attention, see: Nextjs App Loading Page Not Working
Frequently Asked Questions
Why is the window undefined in React?
The "window is not defined" error in React typically occurs when code that relies on the browser's window object is executed outside of a browser environment, such as during server-side rendering or testing. This is because React applications don't have access to the window object in these scenarios.
Sources
- https://blog.sethcorker.com/question/how-to-solve-referenceerror-next-js-window-is-not-defined/
- https://developer.mozilla.org/en-US/docs/Web/API/Window/open
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://sentry.io/answers/next-js-13-window-is-not-defined/
- https://www.geeksforgeeks.org/how-to-fix-next-js-document-is-not-defined/
Featured Images: pexels.com