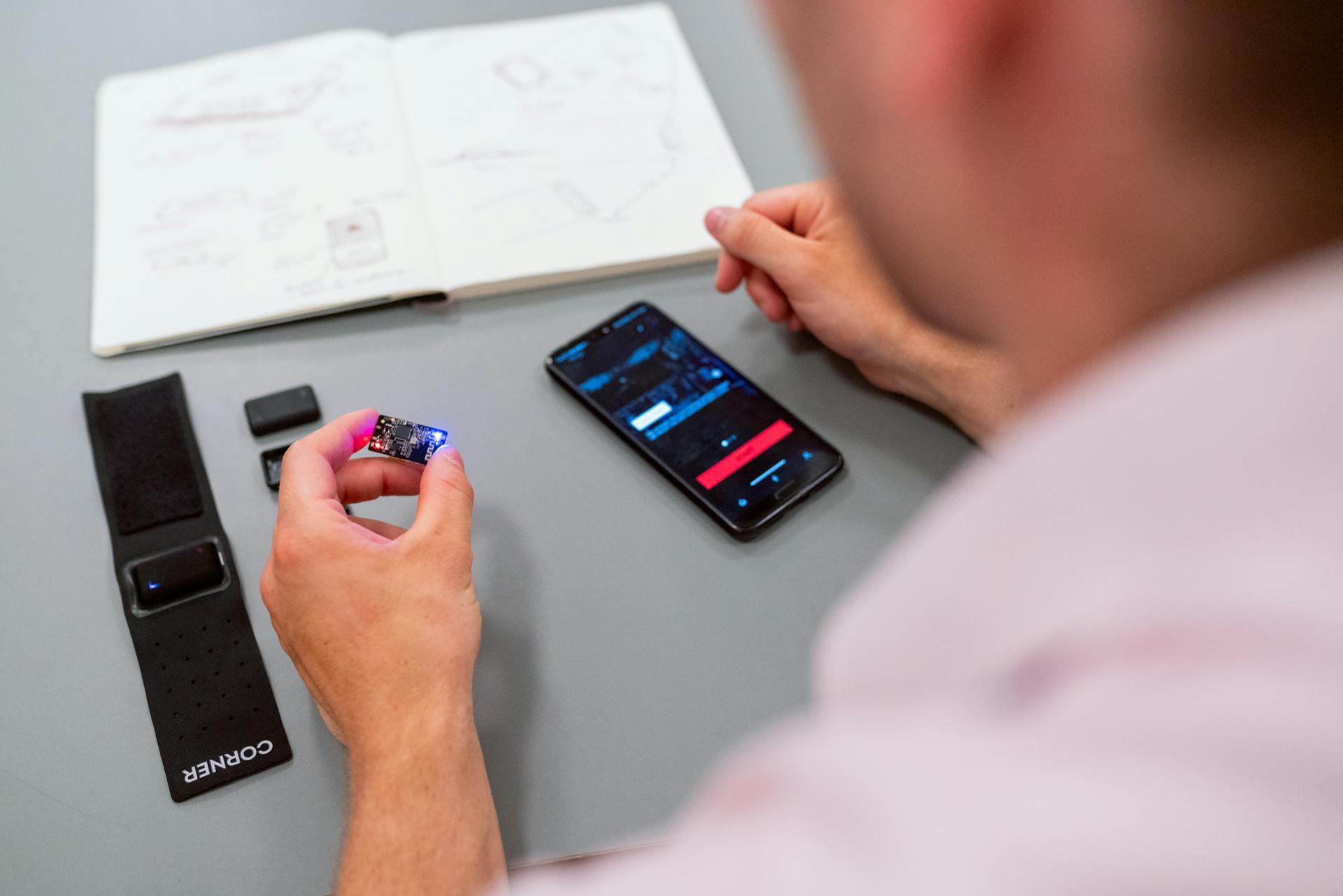
So you're having trouble with Next.js localstorage not being defined? This can be frustrating, especially when you're trying to store data locally for a user.
The issue often arises when you're trying to access localstorage in a server-side rendered (SSR) environment, which is the default behavior in Next.js. This is because localstorage is only available on the client-side.
To resolve this issue, you can use the useSession function from the next-auth library, which allows you to access the session on the server-side. This function can be used to store data in localstorage that can then be accessed on the client-side.
For another approach, see: Next Js Fetch Data save in Context and Next Route
Understanding the Issue
The "next js localstorage is not defined" error can be frustrating, but understanding the issue is key to resolving it. This error typically occurs when trying to access local storage in a Next.js application, which is not directly supported by the framework.
Next.js uses a different approach to handle client-side rendering, which can lead to unexpected behavior when trying to access local storage. This is because Next.js uses a technique called server-side rendering, which can cause local storage to be unavailable.
In Next.js, local storage is not defined because the framework doesn't directly support it, but there are workarounds to make it work.
Discover more: Next Js Document Is Not Defined
Causes of the Error
The causes of the error are multifaceted.
Inadequate maintenance is a common culprit, as seen in the case of the old factory's outdated equipment.
Outdated software can also lead to errors, as noted in the example of the company's legacy system.
Human error, such as typos and incorrect input, can also cause issues, as demonstrated by the IT department's mistake.
Poor communication between teams can exacerbate the problem, as seen in the example of the marketing and development teams not being on the same page.
In some cases, hardware failures can occur due to wear and tear, as was the case with the broken server.
A combination of these factors can lead to a perfect storm of errors, making it difficult to pinpoint the root cause.
Checking LocalStorage Implementation
To check if LocalStorage is implemented correctly, you need to verify if the storage is enabled in the browser. Most modern browsers have LocalStorage enabled by default, but it's always a good idea to double-check.
In some cases, LocalStorage might be blocked by the browser's security settings, so make sure to check those as well. This can happen if the website is not trusted or if the browser is in private mode.
The LocalStorage API can be accessed through the window object, and you can use the localStorage property to store and retrieve data. For example, you can use the localStorage.setItem() method to store a value and the localStorage.getItem() method to retrieve it.
If you're experiencing issues with LocalStorage, try checking the browser's console for any error messages. This can give you a clue about what's going wrong and help you troubleshoot the problem.
Troubleshooting and Solutions
Local storage is not defined in Next.js because it's not enabled by default.
First, check if you're using the correct API, `window.localStorage` or `globalThis.localStorage`, depending on your environment.
If you're using a custom app directory, make sure to configure the `next.config.js` file to enable local storage.
Next.js LocalStorage Not Defined Error
The Next.js LocalStorage Not Defined Error can be frustrating, but it's often a simple fix.
In Next.js, LocalStorage is not enabled by default, so you need to import the 'local-storage' module from 'next/dynamic' to use it.
The error occurs when you try to access LocalStorage before it's been initialized. This often happens when you're using a custom hook to manage state.
Make sure to initialize LocalStorage before using it, and also check if the browser supports LocalStorage.
If you're using a custom hook to manage state, ensure it's properly set up to handle LocalStorage initialization.
In some cases, the error can be caused by a conflict between LocalStorage and other libraries or components that also use storage.
Workarounds for LocalStorage Issues
If you're experiencing issues with LocalStorage, try using alternative storage solutions like IndexedDB or Cookies.
You can also use the LocalStorage API's built-in methods to clear and delete data, such as localStorage.removeItem() and localStorage.clear().
In some cases, LocalStorage issues can be caused by browser extensions or plugins that interfere with its functionality.
If you're experiencing issues with LocalStorage on a specific website, try checking the website's permissions and settings to see if LocalStorage is enabled.
Another workaround is to use a library like localForage, which provides a more robust and flexible storage solution than LocalStorage.
In some cases, LocalStorage issues can be caused by a corrupted or outdated cache, so try clearing the cache and reloading the page.
Best Practices and Optimization
To avoid the "next js localstorage is not defined" error, make sure to import the necessary modules, specifically `next/dynamic` and `next/link`, in your components. This ensures that the necessary dependencies are loaded.
When using `next/dynamic` to load components, always specify the `ssr` option as `false` to prevent the error. This is because `ssr` is enabled by default, which can cause issues with local storage.
In your `pages/_app.js` file, ensure that you're properly handling the `useEffect` hook to initialize local storage. This can be done by importing `localStorage` and setting it up as a state variable.
A different take: Next Js Dynamic
Optimizing LocalStorage Usage
To minimize the size of your LocalStorage, it's essential to store only the data that's necessary for your application. This means avoiding storing large amounts of data, such as images or videos.
Use a data compression algorithm to reduce the size of your data before storing it in LocalStorage. This can be particularly helpful for storing large amounts of text data, such as user input or settings.
Avoid storing sensitive information, like passwords or credit card numbers, in LocalStorage. This is because LocalStorage is not secure and can be accessed by anyone with access to the user's device.
Use a library like Lodash to help manage and optimize your LocalStorage usage. This can include tasks like data validation, data transformation, and data caching.
By following these best practices, you can significantly reduce the size of your LocalStorage and improve the performance of your application.
Improving Performance with Next.js
Using Next.js can significantly improve performance by automatically code-splitting pages, which reduces the initial payload and speeds up page loads.
Optimizing images is crucial, and Next.js provides built-in support for image optimization through the use of the next/image component.
Server-side rendering (SSR) can be enabled for all pages, which can improve performance by rendering pages on the server instead of the client.
Using static site generation (SSG) for pages that don't change frequently can also improve performance, as it allows for faster page loads and reduced server load.
By following these best practices, developers can significantly improve the performance of their Next.js applications and provide a better user experience.
Here's an interesting read: Nextjs Pages
Sources
- https://www.rdegges.com/2018/please-stop-using-local-storage/
- https://nextjs.org/docs/app/building-your-application/rendering/client-components
- https://nextjs.org/docs/app/building-your-application/authentication
- https://blog.sethcorker.com/question/how-to-solve-referenceerror-next-js-window-is-not-defined/
- https://spacejelly.dev/posts/how-to-save-state-to-localstorage-persist-on-refresh-with-react-js
Featured Images: pexels.com