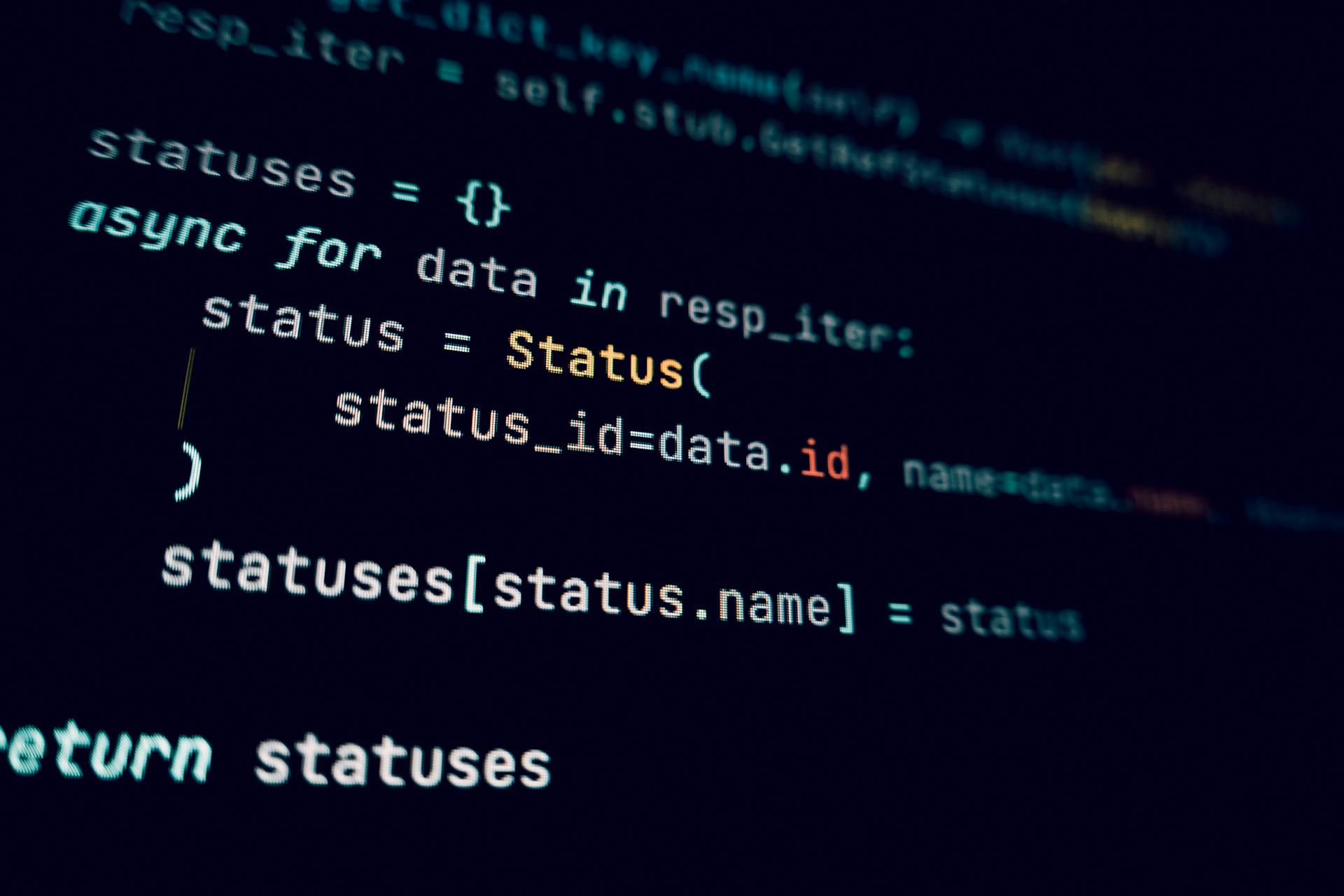
As you start building your Next.js API, security should be top of mind. Always use HTTPS (SSL/TLS) for all API endpoints to encrypt data in transit.
Next.js provides a built-in API route feature that makes it easy to handle API requests. You can define API routes using the `api` directory.
To optimize performance, use caching for frequently accessed data. Next.js provides a built-in caching mechanism using the `cache` API.
Remember to validate user input to prevent common web vulnerabilities like SQL injection and cross-site scripting (XSS).
Intriguing read: Nextjs App Route Get Ssr Data
Getting Started
To use Next.js API routes effectively, you'll need to have some knowledge and tools.
You'll need to follow some steps to set up your project.
Getting started with Next.js API routes requires some knowledge and tools.
To begin, you'll need to have some knowledge and tools.
Having some basic knowledge of Next.js and its tools will make the process smoother.
You can set up your project by following the given steps.
Following these steps will help you get started with Next.js API routes.
Getting started with Next.js API routes can be a bit daunting, but with the right tools and knowledge, you'll be up and running in no time.
Server Actions
Server Actions are a powerful feature in Next.js that allow you to run server-side code in response to user interactions on the client. They've been around since Next.js version 13, but it wasn't until version 14 that they became stable and incorporated by default.
Server Actions are well-suited for use cases like fetching data from external APIs, performing business logic, and updating your database. They can even handle user input validation and payment processing.
You can use Server Actions to fetch data from external APIs without compromising performance or security, which is a huge plus. This way, you can keep your application fast and secure.
Here are some use cases for Server Actions:
- Feching data from external APIs
- Performing business logic
- Updating your database
Server Actions work by sending a request from the client to the server, where a function representing the Server Action is executed. The server then sends the response back to the client, where the promise resolves and the client-side execution continues.
For another approach, see: Next Js Client Side Rendering
Here's a step-by-step breakdown of how Server Actions work:
- A user action or business logic condition triggers a function call to a Server Action.
- Next.js serializes the request parameters and sends them to the server.
- The server deserializes the request parameters and executes a function that represents the Server Action.
- The server serializes the response and sends it back to Next.js.
- Next.js deserializes the response and sends it back to the client.
To use Server Actions effectively, follow these best practices:
- Decouple Server Actions from your components to keep your code easier to understand, maintain, reuse, and test.
- Don't disregard the client-side handling of UI, as it's still important for user experience.
- Cache the results of Server Actions to improve performance by avoiding unnecessary data fetching.
- Use Server Actions to handle errors gracefully and return meaningful error messages to the user.
- Protect your Server Actions from unauthorized access just like you would with API endpoints.
Server Actions Best Practices
Decouple Server Actions from your components to make your code easier to understand and maintain. This means separating the logic of your Server Actions into a separate file or component, rather than embedding it directly in your components.
Server Actions can be written in a separate file, but it's also possible to write them in a Server Component. However, as the book "Clean Code" suggests, separation of concerns is key to keeping your code maintainable.
Cache the results of Server Actions to improve performance. If a Server Action returns data that's not frequently changing, you can cache the results so that they don't have to be fetched from the server every time.
Use Server Actions to handle errors gracefully and return meaningful error messages to the user. This will make your application more user-friendly and help prevent frustration.
Here's an interesting read: Nextjs Code Block
Protect your Server Actions from unauthorized access by implementing security measures. This includes validating input data, authenticating and authorizing requests, and securing sensitive data.
Here are some specific security best practices to keep in mind:
- Enable HTTPS to encrypt data sent from the client to the server.
- Implement rate limiting to prevent abuse of your API.
- Validate input data to prevent injection attacks.
- Authenticate and authorize requests to ensure legitimate users have access rights to the resource.
- Secure sensitive data, such as API keys and passwords, by storing them in environment variables.
Middleware in
Middleware in Next.js API is a powerful tool for performing tasks such as authentication, logging, and request validation.
Middleware functions can be used to abstract reusable code that runs before the handler is invoked. This makes our routes more secure and maintainable.
You can apply middleware to an API route using Next.js, as seen in the example where a small instance of middleware is created and applied to an API route. The authenticate middleware verifies whether a valid token is included in the request headers.
Middleware functions allow us to execute code before the route handler is invoked, enabling us to perform various tasks, such as logging requests, authenticating users, and modifying responses.
Popular middleware libraries one can include with Next.js API routes to make working with middleware relatively easy are next-connect and Express middleware.
Related reading: Middleware Config Nextjs
Here are some benefits of using middleware in Next.js API:
- Makes our routes more secure and maintainable
- Enables us to perform various tasks, such as logging requests, authenticating users, and modifying responses
- Allows us to abstract reusable code that runs before the handler is invoked
However, there are some limitations to using Next.js API middleware, including code running on Edge runtime, limited bundle size, and memory and execution duration limitations.
Authentication
Authentication is a crucial aspect of Next.js API routes, allowing you to control who has access to your API.
You can use middleware to implement authentication by checking for authentication tokens or session cookies and denying access to unauthorized requests. Middleware makes it easy to enhance the security of your API routes.
Here's an example using JSON Web Tokens (JWT): a middleware function checks for a JWT in the Authorization header, attaching the user object to the request if the token is valid. If the token is missing or invalid, it returns an error response.
Middleware helps you make your Next.js API routes more robust and easier to maintain by handling authentication and other tasks. This makes it easier to manage complex API routes and reduce errors.
Suggestion: Nextjs Middleware Firebase Auth
Error Handling
Error handling is a crucial aspect of building reliable Next.js API routes. It ensures that your API endpoints can handle unexpected errors, provide clear error responses, and maintain a good user experience.
Clear error messages are essential for debugging and improving the user experience. To write clear error messages, follow these strategies:
Try-catch blocks are a fundamental concept in error handling. They allow you to catch and handle exceptions that occur during the execution of your API route handlers. By using try-catch blocks, you can provide custom error responses, log errors, and prevent your API from crashing.
Worth a look: Nextjs Error Page
Input Validation
Input Validation is a crucial step in building a secure Next.js API. It involves verifying and sanitizing user input data to prevent malicious data from being injected into your API.
You can use middleware functions to validate user input data, making it easier to catch and handle potential security threats.
Input validation is essential to prevent security vulnerabilities, such as SQL injection or cross-site scripting (XSS).
To validate user input, consider the following best practices:
- Validate and sanitize user input to prevent security vulnerabilities.
- Use middleware to handle errors and exceptions.
By following these guidelines, you can ensure that your Next.js API is secure and reliable.
Performance Optimization
Improving the performance of your Next.js API routes is crucial for delivering a seamless user experience.
Optimizing database queries can significantly improve performance.
Caching API routes can also reduce the number of requests to your API.
Next.js provides built-in support for caching API routes using the cache-control header.
You can cache API routes for a specified amount of time by adding specific lines to your now.json file.
For example, you can cache API routes for one hour by adding the following lines to your now.json file.
Here are some key strategies for improving performance:
- Optimize database queries
- Cache API routes
- Use efficient query techniques
Testing
Testing API routes in Next.js is crucial for ensuring they're reliable and maintainable. Unit testing verifies individual parts of the application work as expected, while integration testing checks how different parts work together.
Unit testing involves testing route handlers to ensure they return the correct responses for given inputs. You can use testing frameworks like Jest to write these unit tests. Here's a simple example of a unit test for an API route using Jest.
To write effective integration tests, you need to ensure the different parts of your application work perfectly together. This includes testing API routes with other parts of the application, such as the database connection or other APIs.
If this caught your attention, see: Next Js Route Handlers
Testing
Testing is a crucial part of ensuring your API routes are reliable and maintainable. It's essential to verify that individual parts of your application work as expected.
You can use testing frameworks like Jest to write unit tests for your API routes. These tests check that the route handlers return the correct responses for given inputs.
Unit testing is a great way to catch bugs early on and prevent them from causing problems later down the line. It's also a good practice to include typing in your unit tests to ensure that the request and response objects are correctly defined.
Here are some key points to consider when unit testing API routes:
- Use Jest to write unit tests for your API routes
- Check that route handlers return the correct responses for given inputs
- Include typing in your unit tests to ensure correct request and response objects
Integration testing takes it a step further by ensuring that different parts of your application work perfectly together. This means testing your API routes with other parts of your application, such as the database connection or other APIs.
Consider reading: Next Js Spa
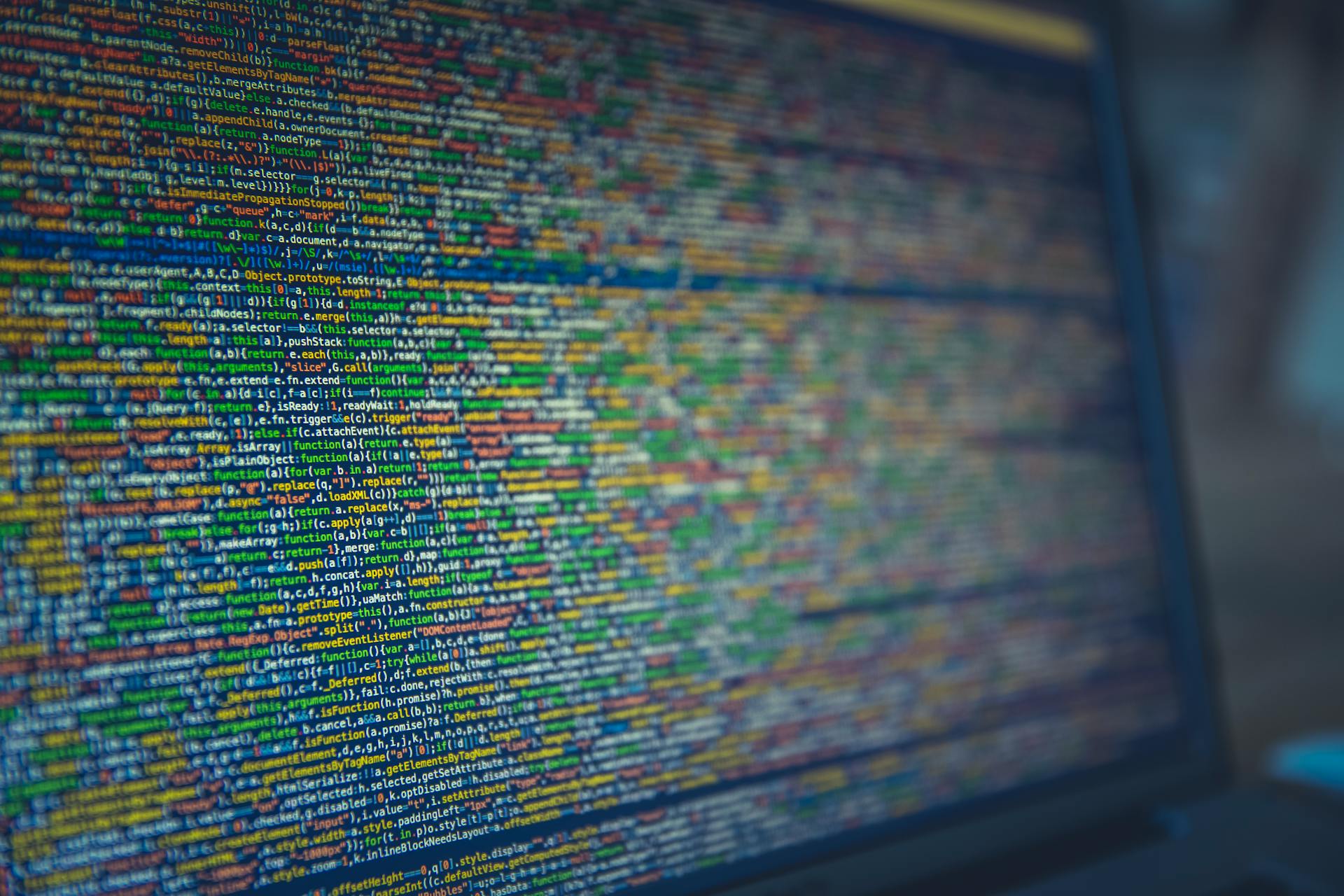
Integration testing is a great way to catch issues that might arise when multiple parts of your application interact with each other. By testing these interactions, you can ensure that your API routes are sturdy and trustworthy.
In integration testing, you can use mock functions to simulate different return scenarios. This helps you test how your API route behaves in different situations.
Here's an example of an integration test for a route to an API, including a database:
- Mock the function getUser to make different return scenarios
- Test that the API route returns a different status and response if a user exists or doesn't exist in the database
Video Tutorials
Testing API routes in Next.js requires a solid understanding of the basics. Next.js API Routes Crash Course is a beginner-friendly video tutorial that covers the fundamentals.
To create a RESTful API, you'll need to follow a step-by-step guide. Building a REST API with Next.js API Routes is a resource that will walk you through the process.
Error handling is a crucial aspect of API routes. Next.js API Routes: Error Handling and Middleware teaches you how to implement error handling and middleware functions.
For another approach, see: Error Boundary Nextjs
Here are some video tutorials to help you deepen your understanding of Next.js API routes:
Best Practices
As you build your Next.js API, it's essential to follow best practices to ensure security and performance. Enable HTTPS to encrypt data sent from the client to the server, protecting against eavesdropping and tampering.
To protect your API from abuse, implement rate limiting to restrict the number of requests within a certain period. This can be achieved using middleware like express-rate-limit.
Validate input data to establish the first perimeter defense against injection attacks. Libraries like joi or yup can be used to validate request data.
Authentication and authorization are crucial to ensure legitimate users have access rights to resources. Establish an authentication mechanism with JWT or OAuth, and verify user roles and permissions.
You can protect sensitive data like API keys and passwords by storing them as environment variables instead of hardcoding them into your codebase.
Check this out: Nextjs save Data Pulled from Api Using State Context
Here are the best practices summarized:
Creating and Setting Up
To create API routes in Next.js, you'll need to define them in the pages/api directory. Each file in this directory corresponds to a specific route.
Creating a new file in the pages/api directory with a name that matches the desired API route is a great way to start. For example, users.js for a route handling user data.
In the file, export a default function that will handle the route. This function takes two parameters: req (the incoming request object) and res (the response object).
The route handler sends a JSON response with a status code of 200 and a message. To create a dynamic API route, you can use square brackets ([]) in the file name to define a parameter.
For instance, pages/api/users/[id].js would match requests to /api/users/1, /api/users/2, and so on. This makes your API more versatile and easier to use.
Worth a look: Nextjs Pages
Here's a list of benefits of dynamic API routes:
By using dynamic API routes, you can create more flexible and scalable APIs that can handle a wide range of requests and parameters.
Handling Requests and Responses
In Next.js API routes, you can type request and response objects using NextApiRequest and NextApiResponse respectively. This helps catch errors and ensures your API endpoints are properly formatted.
To handle GET requests, you need to check the req.method property and respond accordingly, sending a JSON response with a 200 status code if it's a GET request, and setting the Allow header to indicate that only GET requests are allowed, and returning a 405 (Method Not Allowed) status code if it's not.
You can also type the response data returned from an API endpoint by adding types to res: NextApiResponse. This is especially useful when working with dynamic routes, as it allows you to specify the exact data structure that should be returned.
Broaden your view: Nextjs Response Json
Sending External Data
To send data to an external API, you can use Server Actions, like the addComment function that expects a single parameter of type FormData.
This function can be triggered from a form submission, which is different from other functions triggered by JavaScript function calls.
You'll notice that the user is not aware that something is happening, as there is no "loading" or "saving" activity shown, so you'll need to add a loading or saving indicator to fix this.
Creating a new Server Action, like addComment, is a great way to send data to a third-party API, and it's fully functional, but it's not very user-friendly.
By using Server Actions to send data to an external API, you can create a seamless user experience, even if the process takes a little time.
Suggestion: Next Js Server Action
Request and Response Objects
You can provide types for the request and response objects using NextApiRequest and NextApiResponse respectively. This helps catch errors and make your code more maintainable.
The NextApiRequest object represents the incoming request, while NextApiResponse represents the outgoing response. By adding types to these objects, you can ensure that your API endpoints are handling requests and responses correctly.
Here are some key properties of the NextApiRequest and NextApiResponse objects:
By understanding these properties, you can write more effective API endpoints that handle requests and responses correctly.
Processing and Modifying
In Next.js API, the processing and modification of responses is a crucial aspect of building robust APIs.
Middleware can modify the response before it's sent back to the client, allowing for custom headers to be set.
This middleware sets a custom header and a cookie on the response before passing it to the next middleware or route handler.
Custom headers can be used to provide additional information about the response, such as caching instructions or authentication tokens.
By modifying the response in this way, developers can add a layer of complexity to their APIs without affecting the underlying functionality.
Suggestion: Why Are Apis Important
Securing and Protecting
Securing and Protecting your Next.js API is crucial for building a reliable and trustworthy application. API routes are vulnerable to various security threats, including unauthorized access, data tampering, and denial-of-service (DoS) attacks.
To protect your API routes, you can use authentication and authorization mechanisms, such as API Keys and Access Tokens. API Keys are a simple and effective way to protect APIs, and they can be used to identify and authenticate an application making requests to an API.
API Keys are typically used to protect APIs from unauthorized access and to track usage. However, they have a significant security limitation: they do not identify the individual user making the request, allowing anyone with the API Key to access the API.
To mitigate this drawback, you can use OAuth 2.0, which is recommended in the article. The implementation of API Keys varies from project to project, so you should check how your target API implements them.
Securing individual API endpoints is also essential, and this can be achieved using authentication and authorization mechanisms. You can use middleware to protect your API routes, which can be applied to specific routes or to all routes in your application.
Here are some key points to consider when securing your Next.js API:
- API Keys are a simple and effective way to protect APIs.
- API Keys do not identify the individual user making the request.
- OAuth 2.0 can help mitigate the security limitations of API Keys.
- Middleware can be used to protect specific API routes or all routes in your application.
By implementing these security measures, you can protect your Next.js API from unauthorized access and ensure that your application is reliable and trustworthy.
Frequently Asked Questions
What is the Next.js API?
Next.js API is a feature that allows you to create API endpoints alongside your frontend code, eliminating the need for separate backend codebases. It combines backend and frontend code in a single directory, streamlining development and deployment.
Is Next.js API serverless?
Next.js API routes are deployed as serverless functions, offering a scalable and cost-effective solution. This means your API can handle traffic spikes without worrying about server maintenance.
Can Next.js be used as a backend?
Yes, Next.js can be used as a backend framework for web applications, making it a versatile tool for both frontend and backend development. Its API routes and middleware enable seamless backend functionality.
Is Next.js good for REST API?
Yes, Next.js is well-suited for building REST APIs due to its built-in API routes support. It simplifies the process of creating server-side rendered applications with REST API functionality.
What is the difference between Express js and Next.js API?
Express.js is ideal for building lightweight APIs and web servers, while Next.js excels at high-performance websites with server-side rendering and SEO optimization. Choose Express.js for simplicity and Next.js for advanced features and dynamic websites.
Sources
- https://next-auth.js.org/configuration/nextjs
- https://auth0.com/blog/using-nextjs-server-actions-to-call-external-apis/
- https://refine.dev/blog/next-js-api-routes/
- https://nextjsstarter.com/blog/nextjs-api-routes-get-and-post-request-examples/
- https://blog.tericcabrel.com/protect-your-api-routes-in-next-js-with-middleware/
Featured Images: pexels.com