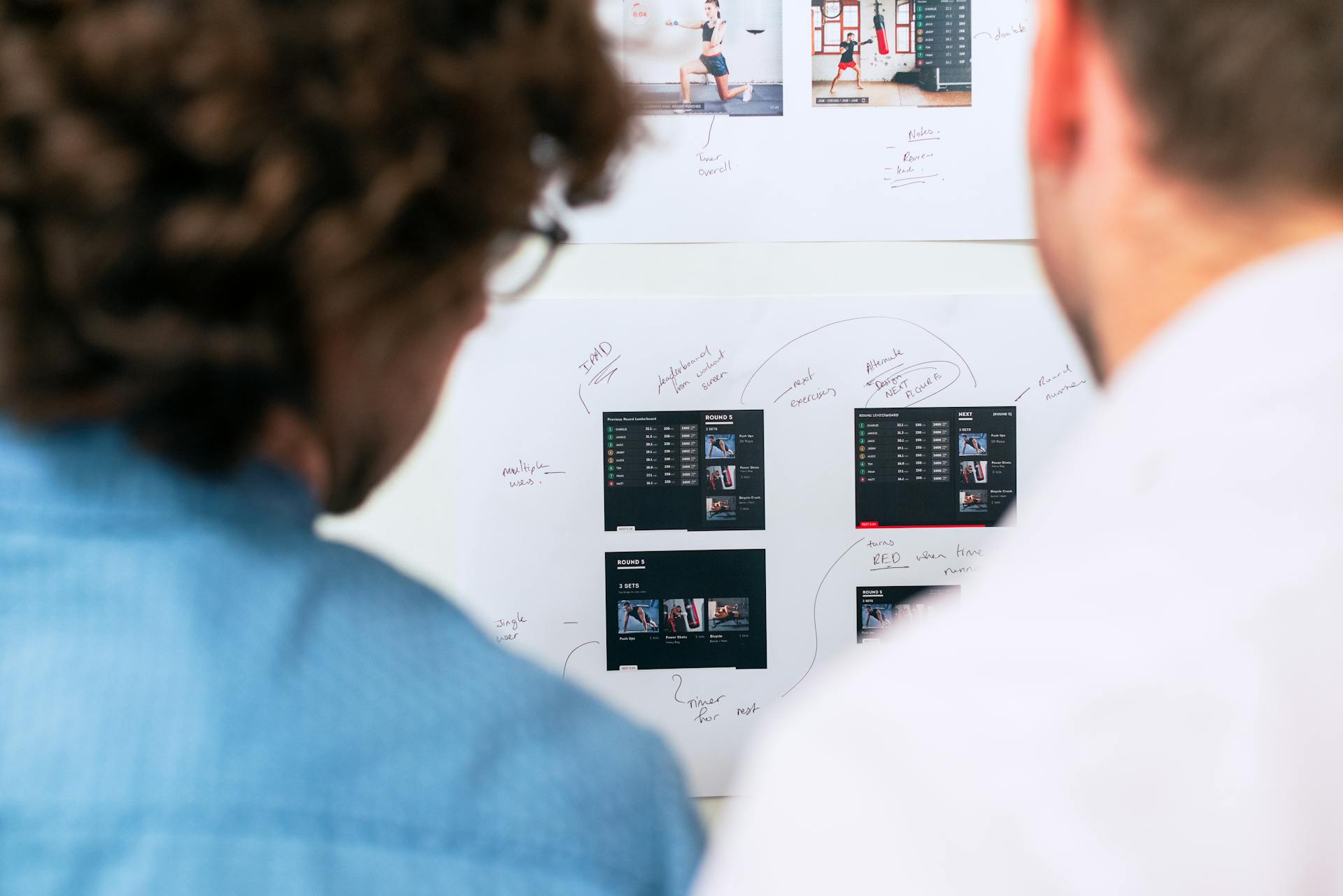
Nextjs is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications.
To get started with Nextjs, you'll need to have Node.js installed on your computer.
In a Nextjs project, the `pages` directory is where you'll write your React components that will be rendered on the server.
The `getServerSideProps` method is a special method in Nextjs that allows you to pre-render pages on the server.
This method returns an object that contains the props that will be passed to the page component.
The `res.json()` method is used to send a JSON response to the client.
For example, if you have a page component that uses the `getServerSideProps` method, you can use `res.json()` to send a JSON response to the client.
This is useful for sending data to the client in a format that can be easily consumed by the frontend.
Suggestion: Next Js Client Side Rendering
In a Nextjs project, you can use the `res.json()` method to send a JSON response to the client by returning an object from the `getServerSideProps` method.
This object will be converted to a JSON response by the `res.json()` method.
By using the `res.json()` method, you can easily send data to the client in a format that can be easily consumed by the frontend.
Curious to learn more? Check out: Next Js Use Client
Next.js Basics
To create API Routes in Next.js, you simply need to create a file in the api folder, and Next.js will treat it as an API endpoint.
API Routes are created in the api folder, which is found in the pages folder of your application.
Every API route must export a default function that handles the requests made to that endpoint.
The function receives two parameters: req, an instance of http.IncomingMessage, and res, an instance of http.ServerResponse.
You can return an HTTP status code and JSON data using the res server response object.
If this caught your attention, see: Next Js Project
To handle different HTTP request methods, you can use a switch statement in your handler function.
You can also use methods like if/else statements to handle different HTTP request methods.
API Routes can be accessed by making a request to /api/endpoint, where endpoint is the name of the file in the api folder.
For example, if you have a file named example.js in pages/api, you can access the API route by making a request to /api/example.
Readers also liked: Next Js Project Structure
Data Fetching and Caching
Data Fetching and Caching is a crucial aspect of Next.js, especially when working with JSON responses.
Next.js uses the built-in `fetch` API to make HTTP requests, which is a great way to fetch data from APIs.
However, you can also use third-party libraries like Axios to make HTTP requests.
To improve performance, Next.js uses caching to store frequently accessed data.
Caching is particularly useful when dealing with APIs that have rate limits or require authentication.
You might enjoy: Nextjs App Route Get Ssr Data
By using caching, you can reduce the number of requests made to the API, which can help prevent rate limiting issues.
In Next.js, you can use the `useSWR` hook to implement caching for your API requests.
`useSWR` is a popular library that provides a simple way to implement caching and suspense for your API requests.
With `useSWR`, you can easily cache API responses and revalidate them when the data changes.
This can help improve the performance and user experience of your Next.js application.
Server Actions
Server Actions are a powerful feature in Next.js that allows you to run server-side code in response to user interactions on the client. They've been a part of Next.js since version 13, but became stable and incorporated by default in version 14.
Server Actions are well-suited for use cases such as fetching data from external APIs, performing business logic, and updating your database. This is because they can be executed on the server without compromising performance or security.
Here's an interesting read: Check Nextjs Version
Here are some key benefits of using Server Actions:
- Fetch data from external APIs without compromising performance or security
- Perform business logic that requires server execution
- Update your database without creating separate API routes
Server Actions work by executing asynchronous JavaScript functions on the server in response to user interactions on the client. The basic process involves a user action or business logic condition triggering a function call to a Server Action, which is then executed on the server. The server then serializes the response and sends it back to Next.js, which deserializes the response and sends it back to the front end.
In summary, Server Actions provide a convenient way to run server-side code in response to user interactions on the client, making it easier to build scalable and secure applications.
You might enjoy: Next Js Debugging
Request and Response
When working with API Routes in Next.js, it's essential to understand how to type request and response objects to ensure your code is type-safe.
Next.js provides automatic types for API Routes, which involves zero configuration to set up. This is a huge time-saver and helps catch errors early on.
To provide types for the request and response objects, you can use NextApiRequest and NextApiResponse respectively. This is a straightforward process that makes your code more maintainable.
You need to have integrated TypeScript in your Next.js project before using these features. Don't worry, it's easy to add TypeScript to your Next.js project.
Testing and Configuration
API Routes in Next.js can be customized with a config object, allowing you to tailor their behavior to your application's needs.
This customization is achieved by exporting a config object in the same file as the API route, where the "api" property contains all specific configuration options.
You can use this feature to fine-tune your API Routes and make them more efficient or secure.
Next.js also allows you to test your API Routes, which is crucial for ensuring their reliability and maintainability.
Testing in Next.js
Testing in Next.js is crucial for ensuring our application is reliable and maintainable.
Unit testing is essential for verifying that individual parts of our application work as expected, and for API routes, this means testing the route handlers to ensure they return the correct responses for given inputs.
Take a look at this: Web Application Programming Interface
We can use testing frameworks like Jest to write these unit tests. Jest is a popular testing framework for JavaScript, and it's widely used in the industry.
Here’s a simple example of a unit test for an API route using Jest, which creates mock request and response objects with node-mocks-http to test the API handler.
A correct unit test for an API route will assert that a correct response status and body have been sent. This helps us catch bugs early and make sure our application works as expected.
Custom Configuration
Custom Configuration is a powerful feature in Next.js that allows you to tailor the default configuration of API Routes to your specific needs.
You can customize the default configuration of every API route in Next.js by exporting a config object in the same file.
This config object contains all the configuration options that are specific to every API route, including the "api" configuration options.
Here's an interesting read: Title of Specific Page Nextjs
Dynamic
You can create dynamic API routes in Next.js, similar to how regular dynamic pages work. This allows you to send responses for different queries to an API endpoint.
To create a dynamic API route, create a folder named trivia in the /pages/api folder of your application. Then, in the trivia folder, create a file named [number].js.
In this file, you can return a random fact from an external API about every number query you receive on the API endpoint. You can use a library like superagent to make requests to the external API.
You can install superagent with the command npm install superagent. Then, add the following code to the [number].js file to return a random trivia for a given number.
If you navigate to http://localhost:3000/api/trivia/34, you'll see a random trivia for that number. If you use an invalid number, you'll get a 400 Bad Request.
A unique perspective: Next Js Npm Install
Featured Images: pexels.com