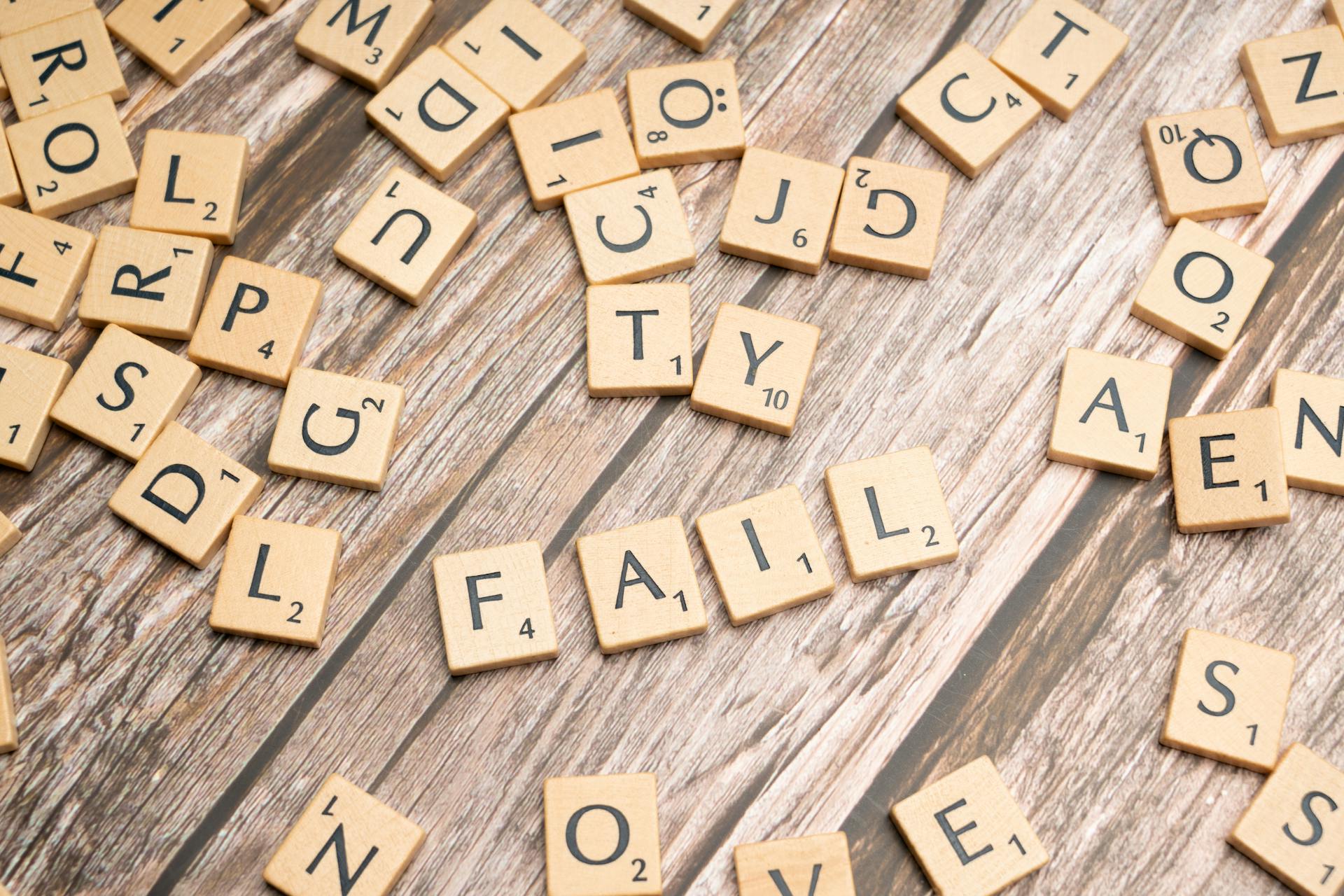
Error boundaries in Next.js are a crucial feature for handling errors in a robust and user-friendly way. They allow you to catch and display errors in a way that's both informative and visually appealing.
By using error boundaries, you can prevent your application from crashing and provide a better user experience. This is especially important for applications with complex logic or multiple components.
In Next.js, error boundaries are implemented using a special component called "ErrorBoundary". This component is responsible for catching and displaying errors in a way that's customizable and flexible.
Worth a look: Nextjs Usecontext
What Are Error Boundaries?
Error boundaries are a feature in Next.js that allows developers to handle better errors during the components' rendering phase.
They help prevent the entire application from crashing, providing a fallback UI in case of errors.
Error boundaries are implemented as higher-order components that wrap around a collection of components, creating an isolated error-catching zone.
This approach is especially useful for complex applications with multiple components, as it isolates the error and prevents it from bringing down the entire application.
Check this out: Next Js Spa
In Next.js, error boundaries can be implemented using an error.js file in the project structure, which works as a component in React that helps detect and contain errors.
This error.js file creates a fallback UI for specific route segments or components that experience issues, preventing the entire application from crashing.
Next.js automatically assigns error boundaries to each route segment using the file system hierarchy based on the project structure, allowing developers to control the level of granularity in error handling.
You might like: Next Js Project Structure
Setting Up Error Boundaries
To set up error boundaries in your Next.js application, the first step is to create an ErrorBoundary component. This component will catch JavaScript errors in its child component tree, log them, and display a fallback UI instead of letting the error crash the application.
You can create a basic ErrorBoundary component using the following code. This component has a constructor, a static getDerivedStateFromError method, and a componentDidCatch method.
You might enjoy: Next Js Cookie
The constructor is used to initialize the component's state. The static getDerivedStateFromError method is called when an error is caught and updates the state to show a fallback UI. The componentDidCatch method logs the error and can be used to send it to an error reporting service console.
To integrate the ErrorBoundary component into your Next.js application, you can wrap your application's root component with it. This ensures that any error within the application gets caught by the error boundary.
By wrapping the Component with ErrorBoundary, you ensure that all errors within your app's component tree are caught and handled, improving error handling in your Next.js app.
Explore further: Using State in Next Js
Components and Fallback UI
Error boundaries in Next.js are a great way to handle errors that occur in client and server components. By wrapping these components with an error boundary, you can catch errors and display a fallback UI instead of breaking the entire app.
Curious to learn more? Check out: Nextjs Error Page
Client components, which run in the browser, are prone to client-side errors. Using an error boundary, you can handle these errors gracefully by wrapping them with an error boundary component.
Server components handle server-side logic and rendering, and errors in these components can also be managed using error boundaries. To use a custom fallback component in your error boundary, modify the render method to display the custom component when an error occurs.
A fallback error component provides a user-friendly message when an error occurs. This component takes an error object as a prop and displays a user-friendly error message. You can use a custom fallback component to provide more informative and user-friendly error UI.
To create a custom fallback component, you can modify the render method to display the custom component when an error occurs. This allows you to provide a more informative and user-friendly error UI, enhancing the overall user experience when errors occur.
You can make the fallback UI more informative or user-friendly by adding retry buttons, error logging services, or even suggestions to contact support.
Consider reading: Nextjs Custom Server
Error Handling and Reporting
Error handling is a crucial aspect of any Next.js application. Integrating an error reporting service like Sentry can help you track and log errors effectively.
You can integrate Sentry into your Next.js app by installing it, configuring it in sentry.client.config.js and sentry.server.config.js, and wrapping your application with the Sentry.ErrorBoundary component.
To send errors to your reporting service, use the componentDidCatch method in your ErrorBoundary component. This method captures the error and sends it to the service.
Here are some popular error-reporting services you can consider:
- Sentry
- LogRocket
- Bugsnag
Reporting and Logging
Reporting and logging are essential parts of error handling. Integrating an error reporting service console in your Next.js application allows you to track and log errors effectively.
You can choose from popular error-reporting services like Sentry, LogRocket, and Bugsnag. To integrate Sentry, you'll need to install it and configure it in sentry.client.config.js and sentry.server.config.js.
To send errors to your reporting service, use the componentDidCatch method in your ErrorBoundary component. This method captures the error and sends it to the service.
Worth a look: Sentry Nextjs
In production, Next.js forwards an Error object to the nearest error.js file as the error prop, but only sends a generic message and a digest property to the client. The digest is a hash of the error, useful for matching with server-side logs.
Here are some popular error-reporting services you can use:
- Sentry
- LogRocket
- Bugsnag
Handling
Handling errors effectively is crucial for any Next.js application. You can integrate an error reporting service console to track and log errors, and popular services include Sentry, LogRocket, and Bugsnag.
To send errors to your reporting service, use the componentDidCatch method in your ErrorBoundary component. This method captures the error and sends it to the service.
If an API call fails, it's essential to manage the failure smoothly without affecting the rest of the app. Use try/catch blocks with asynchronous functions and provide fallback messages to users when errors occur.
To handle server errors, Next.js forwards an Error object (with sensitive details removed in production) to the nearest error.js file as the error prop. In development, the Error object sent to the client includes the original error message for easier debugging.
Here are some key steps to handle errors in a Next.js application:
- Install Sentry and configure it in sentry.client.config.js and sentry.server.config.js
- Wrap your application with the Sentry.ErrorBoundary component
- Use the componentDidCatch method in your ErrorBoundary component to send errors to the reporting service
- Use try/catch blocks with asynchronous functions to handle API errors
Best Practices and Troubleshooting
Proper error handling in Next.js involves using error boundaries strategically to catch and manage errors effectively. This includes using error boundaries sparingly, wrapping only those components that are prone to errors, such as client components with user input or third-party integrations.
Centralized logging is essential, as it helps in identifying and addressing issues promptly. This can be achieved by integrating an error reporting service console to centralize error logging and monitoring.
Custom error pages are also crucial, as they provide a better user experience. For example, creating a custom error page for 404 errors can be done by wrapping the component with an error boundary.
Avoid overusing error boundaries, as it can make debugging harder and affect performance. Similarly, ignoring server-side errors can lead to issues that are not properly addressed.
Here are some key takeaways for effective error handling in Next.js:
- Use error boundaries sparingly
- Centralize logging
- Create custom error pages
- Avoid overusing error boundaries
- Don't ignore server-side errors
By following these best practices, you can ensure that your Next.js application handles errors effectively, providing a better user experience and making it easier to debug and maintain your code.
Testing
Testing error boundaries in Next.js is crucial to ensure your application can handle errors gracefully under different scenarios.
Some popular testing libraries for Next.js include Jest and React Testing Library.
To test error boundaries effectively, you need to simulate errors and verify that the fallback UI is displayed correctly.
Here's an example of how to test an error boundary using Jest and React testing library:
throw new Error('Test error');
test('renders fallback UI when an error occurs', () => {
expect(screen.getByText('Something went wrong.')).toBeInTheDocument();
This test ensures that the ErrorBoundary component catches the error thrown by ProblemChild and displays the fallback UI.
To ensure error boundaries are working as expected, testing the error scenarios in your application is crucial.
Some popular testing libraries for error boundary testing include Jest and React Testing Library.
Here are some popular testing libraries for error boundary testing:
- Jest: A JavaScript testing framework
- React Testing Library: A library for testing React components
Sources
- https://www.dhiwise.com/post/nextjs-error-boundary-best-practices
- https://dev.to/rajeshkumaryadavdotcom/understanding-error-boundaries-in-nextjs-a-deep-dive-with-examples-fk0
- https://alerty.ai/blog/nextjs-error-boundary
- https://www.creowis.com/blog/error-handling-in-nextjs-app-router
- https://www.saffrontech.net/blog/error-handling-in-nextjs
Featured Images: pexels.com