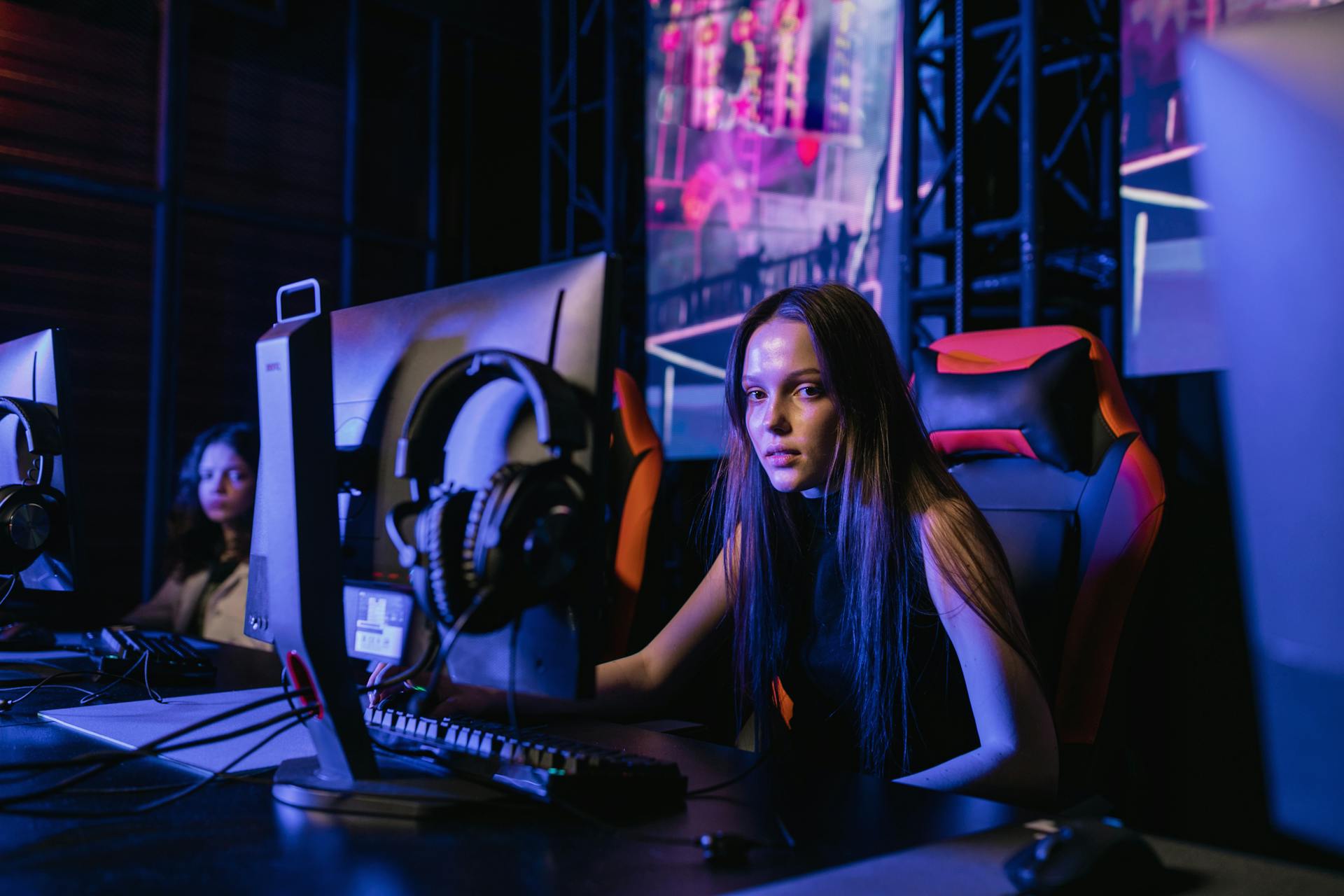
To run JavaScript code in the browser console, you need to open the developer tools.
To open the developer tools, right-click on a page and select Inspect or press F12.
The console is where you'll write and execute your JavaScript code.
You can also access the console by pressing Ctrl + Shift + J or Cmd + Opt + J on a Mac.
Now that you have the console open, you can start writing your JavaScript code.
For another approach, see: How to Open Browser Console Chrome
Console Functions
Console Functions are incredibly powerful, and they can be used to manipulate and inspect data in the browser. Steven was blown away by the power of the browser console, and for good reason - it's a game-changer.
You can use console functions to print color syntax highlighted code, which can be super helpful for old-school paper reviews. Darren was searching for a way to do this, and he was stumped because Atom and Chrome didn't support it directly. Luckily, there are some workarounds that can help.
Here are some examples of console functions that you can use to print color syntax highlighted code:
- console.log() with a string argument
- console.log() with a JSON object argument
- console.log() with a function argument
These functions can be used to print data in a variety of formats, and they can be customized to suit your needs.
Console.Assert(Expression, Object)
Console.assert() is a method that takes two parameters: a boolean expression and an object. It's used to assert a condition and print the object to the console if the condition is false.
The boolean expression is the key here - it's what determines whether the object gets printed or not. If the result of the expression is false, the object will be printed in the console.
You can use any valid JavaScript object as the second parameter, but it's common to use a string object.
Console.Warn([, ])
Console.Warn([, ]) is a method that will log a message to the console with a warning flag. This method is similar to console.log() in terms of functionality, but with a distinct flag that sets it apart.
You might like: Console Log in Nextjs
The console.warn() method is useful for logging warning messages that need attention but don't necessarily require immediate action. It's a good way to flag potential issues without causing unnecessary alarm.
One key benefit of console.warn() is its ability to filter log messages using flags in the developer console. This can save time and effort when debugging code by allowing you to quickly identify and focus on warning messages.
Console.GroupCollapsed(Object)
Console.GroupCollapsed(Object) is essentially the same as console.group().
The main difference is that console.groupCollapsed() initially displays the group collapsed rather than open in the console.
You can use console.groupCollapsed() to group log messages that you want to keep closed by default. This can be useful for debugging complex issues.
Console.Table(Data)
Console.Table(Data) is a powerful method that allows you to output structured data as an interactive table in the console. This can be really handy for examining data returned by an AJAX call, as we can see in the example from January 28, 2014.
Take a look at this: Html Table Syntax
The console.table() method is mentioned in the article as a way to create interactive tables with structured data. It's a great tool for developers who want to take their skills further than a simple console.log().
To use console.table(), you simply need to pass in the data you want to display as a table. For example, if you have an array of objects, you can pass that array to console.table() and it will display the data in a neat and interactive table.
Here are a few examples of how you can use console.table() to display different types of data:
- console.table(data)
- console.table(arrayOfObjects)
- console.table(objectWithNestedData)
By using console.table(), you can make it easier to examine and understand complex data, which is a huge time-saver when debugging and testing your code. This method is especially useful when working with large datasets or complex object structures.
As one developer noted, "This is great! I need to take my skills further than a simple console.log()". By using console.table(), you can take your skills to the next level and become a more efficient and effective developer.
Consider reading: Browser Developer Tools Firefox
Console Grouping
Console Grouping is a useful feature that helps keep your console output organized.
You can use console.group() to group together a series of log messages. This method is especially helpful when debugging complex code.
To create a group, simply call console.group() followed by the title of your group.
Any subsequent log messages will be added to the group until you call console.groupEnd() to close the group.
Nesting multiple groups within one another is also possible, making it easy to drill down into specific areas of your code.
Console Performance
Console performance is crucial for a smooth browsing experience.
The browser's JavaScript engine, V8, is responsible for executing code in the console, and it's designed to optimize performance.
A well-written console code can run up to 10 times faster than a poorly written one, as seen in the example of the optimized vs. unoptimized code comparison.
The console's performance can be affected by the number of objects created, which can lead to memory leaks and slower performance.
In the example of the memory leak scenario, the console's performance degraded significantly due to the excessive object creation.
A good practice is to use the browser's built-in debugging tools, such as the Timeline panel, to identify performance bottlenecks in the console code.
By using the Timeline panel, developers can gain insights into the performance of their console code and optimize it for better performance.
Console Output
You can print out variables using the `console.log()` function. For example, Kevin found that he needed to use global variables by omitting the `var` keyword to get the variables to be correctly initialized. This is a useful tip to keep in mind when working with the console.
The console output can be formatted using the format specifiers. However, as Kevin found out, there are some quirks to be aware of. For example, forcing variables to be global variables may be necessary in some cases.
Console.log(
Console.log() is a fundamental method for outputting objects to the console. It can take multiple objects as parameters, which will be concatenated into a single string.
Using multiple objects with console.log() is straightforward. Simply list them after the method, and they'll be displayed together in the console.
You can also use format specifiers with console.log() to customize the output. This is especially useful when working with different data types. The first parameter can contain format specifiers to define the format and positioning of the subsequent objects.
Here are the supported format specifiers:
The %c format specifier is particularly useful for styling the console output.
Console.Dir
Console.Dir is a powerful tool for examining objects in the console. It will print a JavaScript representation of the supplied object to the console.
This method is especially useful for examining HTML elements, as it will display the DOM representation of the element.
The console.dir() method is different from console.log(), which displays the XML representation of the element.
Sources
- https://learn.microsoft.com/en-us/microsoft-edge/devtools-guide-chromium/console/console-javascript
- https://developer.chrome.com/docs/devtools/javascript/snippets
- https://blog.teamtreehouse.com/mastering-developer-tools-console
- https://javascript.info/debugging-chrome
- https://javascript.plainenglish.io/how-to-bring-the-browser-devtools-and-console-to-vs-code-848edc257094
Featured Images: pexels.com