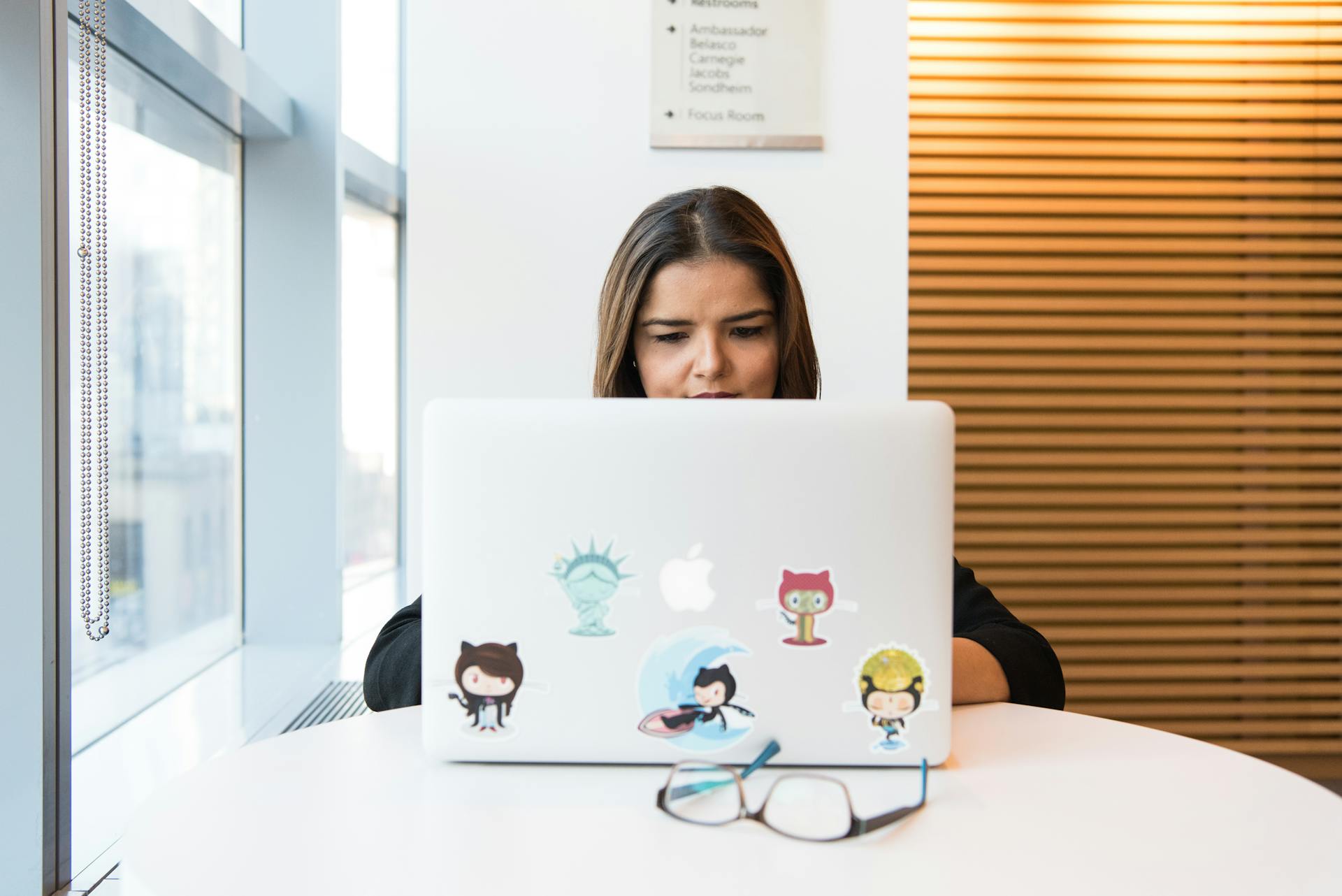
Routing to a page in Next.js can be a bit tricky, but don't worry, I've got you covered.
To start routing to a page in Next.js, you need to create a new file in the pages directory for each page you want to create. This is where things get interesting.
Next.js uses a file-based routing system, which means that the file name and directory structure determine the URL of the page. For example, if you create a file called about.js in the pages directory, the URL of the page will be /about.
This approach makes it easy to create dynamic routes and nested routes, which can be really useful for complex applications.
Discover more: Nested Routes in Next Js
Routing Basics
Next.js routing is a file-based system that makes it easy to manage routes without complex configurations. Each file in the pages directory corresponds to a route in the application.
You can create dynamic routes using parameterized file names, allowing you to handle routes with dynamic segments, such as user profiles or blog posts. This is achieved by using the bracket syntax, like in the example [id].js.
Discover more: Next Js Dynamic Routes
Here's a breakdown of how Next.js treats files in the pages directory as routes:
This means that each file in the pages directory automatically becomes a route, eliminating the need for complex routing configurations.
To add a static route, you can create a file in the /pages directory, like the about.js file mentioned in Example 6.
A fresh viewpoint: Deploy Nextjs to Github Pages
Segments and Routing
Segments are used in Next.js routing to define dynamic routes. A dynamic route segment is denoted by square brackets around a name, such as [book-id].js. This syntax helps eliminate file creation redundancy for a single route to access multiple books with their ID.
Dynamic routes can be further extended with Catch-All Routes, denoted by three dots before the square brackets, like [...slug]. This syntax catches all routes and is useful for deeply nested routes.
Here are some examples of how to use dynamic route segments and Catch-All Routes:
Segments
Segments are a fundamental part of Next.js routing, allowing you to define dynamic routes and catch-all routes. You can use square brackets to define dynamic route segments, such as [book-id].js, which helps eliminate file creation redundancy for a single route to access multiple books with their ID.
Explore further: Intercepting Routes Next Js
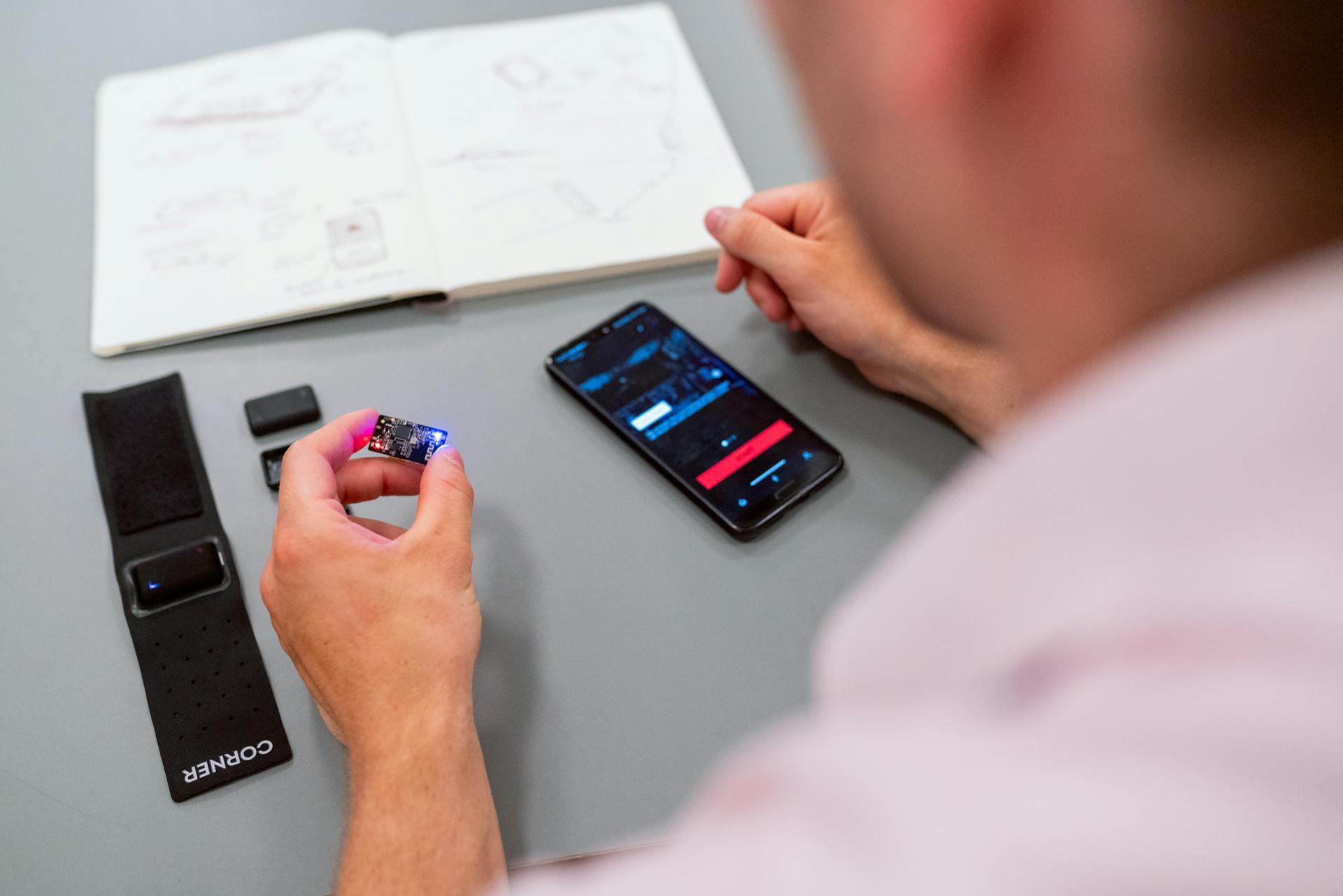
Dynamic route segments can be extended with catch-all routes, which use three dots inside the square brackets, like […slug].js. This allows you to catch all routes and eliminate the need for deeply nested routes.
You can also use optional catch-all routes, which use double square brackets with three dots inside, like [[…slug]].js. This makes the catch-all route optional, and if it's not available, it will fallback to the path /printed-books, rendered with [[…slug]].js route handler, without any query params.
Here's a summary of the different types of segments:
By understanding and using these different types of segments, you can create robust and flexible routing systems in your Next.js applications.
Nested
Nested routes allow for more organized and scalable routing in Next.js.
Creating a nested folder structure in the pages directory enables nested routes to be structured in the same manner. For example, if we create a new folder called users and create a new file called about.js within it, we can access this file by visiting http://localhost:3000/users/about.
For your interest: What Is .next Folder in Next Js
Here's a list of how nested routes can be structured:
- pages/users/about.js will be accessible on http://localhost:3000/users/about
- pages/posts/index.js will be accessible on http://localhost:3000/posts
- pages/posts/my-first-post.js will be accessible on http://localhost:3000/posts/my-first-post
Nested routes can also be created using a nested folder structure in the pages directory. To create a nested route, let's create a posts folder in the pages directory and also create an index.js file in the posts folder.
Recommended read: Pages Router Next Js
Route Parameters and Redirects
Route parameters allow you to pass data to a page and access it using the useRouter hook or getInitialProps. This is useful for dynamic routes like my-folder/[id].js, where the data is stored in the query element.
You can access the data by using the useRouter hook, which gives you access to the router object that contains the params. This is especially useful for dynamic routes like my-folder/[id].js.
Redirects in Next.js can be done using several methods, including the useRouter/push method, which redirects pages on the client side based on user actions. This method is useful for creating a new page that loads in response to a user action.
For your interest: Dynamic Route Nextjs 13
Here are the different methods for redirects in Next.js, along with their uses:
Passing Route Parameters
Next.js allows you to pass route parameters and then get back the data using the useRouter hook or getInitialProps. It gives you access to the router object that contains the params.
To pass route parameters, you can use the Link component and pass an object with a pathname property. For example, instead of providing a string to the href attribute, you can pass in an object like this: { pathname: '/my-folder/[id]', query: { id: 1 } }.
Here's a list of ways to pass route parameters:
- my-folder/[id].js
- my-folder/[…slug].js
- pages/authors/[…slug].js
The useRouter hook gives you access to the router object, which contains the params. You can use this hook to get the dynamic data from the path. For example, if you have a file named [postId].js, you can use the useRouter hook to get the post ID from the path.
The useRouter hook is useful for getting the dynamic data from the path. You can use it to display the data to the user. For example, in the [postId].js file, you can use the useRouter hook to get the post ID and display it to the user.
Optional catch-all routes in Next.js allow you to handle routes with a variable number of segments, including the option of no segments at all. You can make catch-all routes optional by adding three dots inside the double square brackets in the name of the file. For example: [[…slug]].js.
Expand your knowledge: Next Js Build Collecting Page Data
Redirects Using Next.config.js
You can define Next.js redirects in the next.config.js file, located in your project directory. This is best for permanent redirects on the server side, where you need to inform search engines of a changed URL.
These redirects apply to the entirety of your application and all users accessing it. You can create redirects in next.config.js by adding them to the return value of the redirects function exported by the module.
Discover more: Next Config Js
For example, you can define a basic redirect from one page to another, or redirect everything under the old_blog path to new_blog. You can even use regex to do the same thing only if the post_id is numeric.
Here are some examples of redirects you can create in next.config.js:
Adding Next.js redirects to the config is a great way to keep all your static redirects in one place. This is especially useful when you have a page that was created early in development with a misspelled URL, and you need to correct it.
Advanced Routing
So you want to get advanced with Next.js routing? Let's dive into the details.
Next.js uses the file system to enable routing in the app, which means it automatically treats every file with the extensions .js, .jsx, .ts, or .tsx under the pages directory as a route.
Routing is based on file names, which is pretty cool. For example, if you have a file named index.js, it's treated as the home page accessible on http://localhost:3000.
Here's a breakdown of how routing works in Next.js:
- index.js is the home page accessible on http://localhost:3000
- contact.js is the contact page accessible on http://localhost:3000/contact
- my-folder/index.js is the page located on the sub-folder my-folder accessible on http://localhost:3000/my-folder
- my-folder/about.js is the about page located on the sub-folder my-folder accessible on http://localhost:3000/my-folder/about
You can create sub-folders to organize your pages and Next.js will automatically route to them. Just remember to keep the file name as the route name.
Best Practices and SEO
To route to a page in Next.js effectively, you need to understand the best practices for SEO. Pages in your app should have unique URLs.
For SEO purposes, you should use permanently redirected URLs to pass their SEO score on to the new page. This is good for maintaining SEO.
Temporary redirects won't pass on their SEO score, making them suitable only for short-lived redirects like temporarily hiding a page while it's updated.
Each redirect requires reloading content, which will disrupt your users' experience. Try to minimize redirects to get the best performance for your Next.js app.
Understanding Next.js API
Next.js provides two main APIs for handling routes: the next/link API and the next/router API. The next/link API exposes the Link component as a declarative way to perform client-side route transitions.
For another approach, see: Nextjs 13 Api
The Link component will resolve to a regular HTML hyperlink. This means you can use it to create links that will navigate to different pages in your Next.js application.
The href prop is the only required prop to the Link component. You'll need to include this prop when using the Link component to specify the destination of the link.
The next/router API is imperative, meaning it allows you to manually perform routing. This is useful in scenarios where the next/link API isn't enough, or you need to "hook" into the routing.
To use the next/router API, you'll need to use the useRouter hook, which gives you access to the router object inside any function component. This hook is a React hook, so you won't be able to use it with class components.
If you do need to use the router object in a class component, you can use the withRouter method instead.
On a similar theme: Nextjs Link
Frequently Asked Questions
Does Next.js use client-side routing?
Next.js uses client-side routing, but it doesn't require any additional routing libraries. Instead, you create routes as files under the 'pages' directory and use the built-in Link component.
How does Next.js do routing?
Next.js uses a file-system based router, where routes are defined by nested folders and a final page.js file. This approach allows for a simple and intuitive way to manage routes in your application.
Featured Images: pexels.com