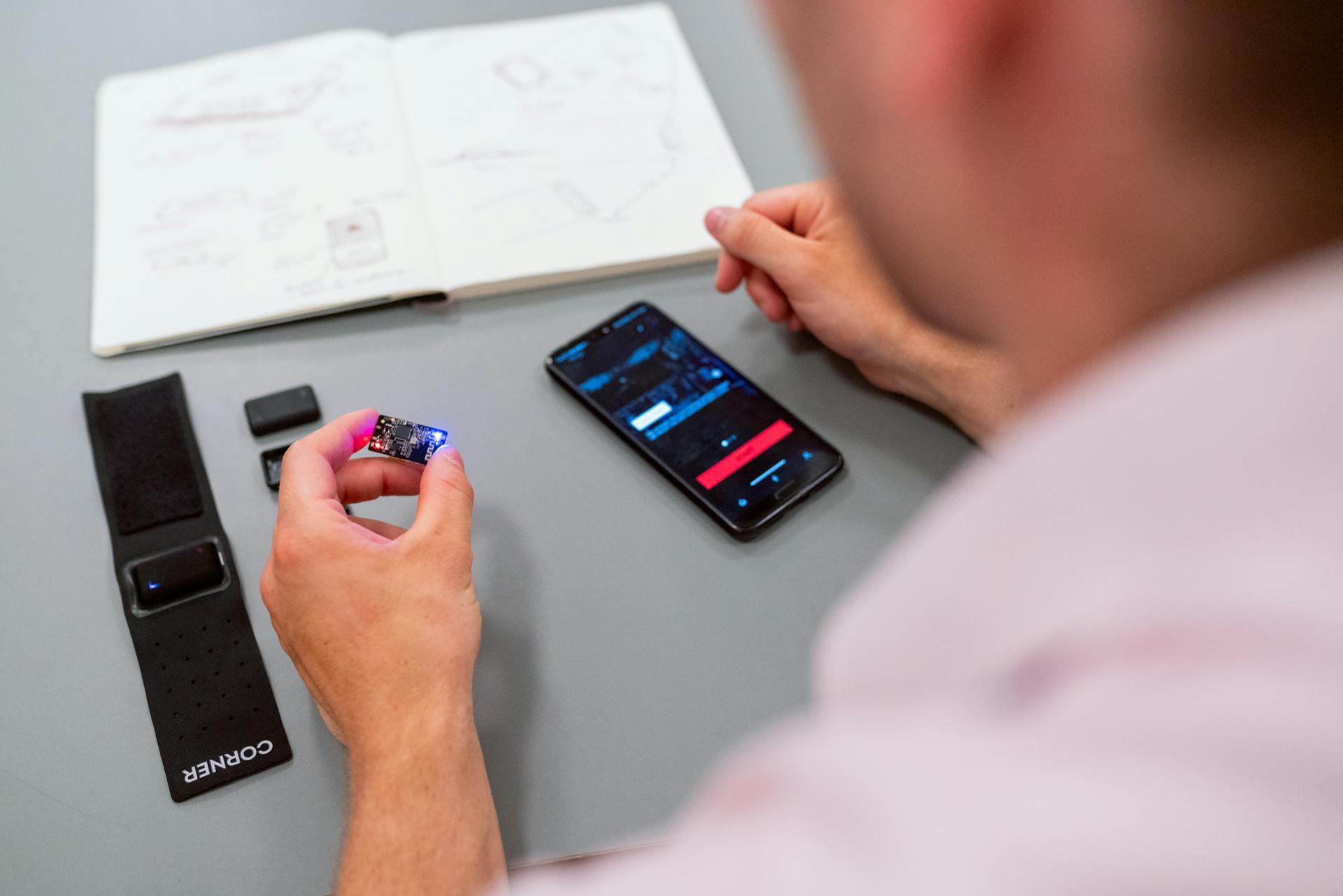
Dynamic Route Nextjs 13 is a powerful feature that allows you to create flexible and scalable routes for your Next.js application.
With Next.js 13, you can create dynamic routes using the `pages` directory and the `getStaticPaths` method. This method allows you to specify the paths for your dynamic routes at build time.
To create a dynamic route, you need to create a file in the `pages` directory with a name that matches the pattern of your route. For example, if you want to create a dynamic route for a blog post, you can create a file named `[slug].js` in the `pages` directory.
This file will be used to render the blog post for any slug that matches the pattern.
You might enjoy: Intercepting Routes Next Js Example
Building Routes
Building routes in Next.js is a breeze, especially with the introduction of Route Handlers in v13.2. You can create dynamic routes by wrapping file or folder names in square brackets, like this [segmentName]. This allows you to create routes like /post/1, /post/2, etc.
See what others are reading: Nested Routes in Next Js
To create a dynamic route component, you can create a file at pages/post/[id].js. This will match requests like /post/1, /post/2, /post/a. You can render a simple template with the required fields, such as title, description, and date.
Next.js also supports nested routes, which can be created by defining a parent API route in app/api and then nesting a dynamic route within it. For example, you can create a username route and nest a dynamic route [user] within it. This allows you to access the parent route via the URL localhost:3000/api/username and the nested route via localhost:3000/api/username/[your username].
Here's a quick rundown of the key benefits of Next.js route handling:
- Automatic code splitting: Next.js loads only the necessary code for each page, improving performance.
- Built-in API routes: Next.js integrates API route handling within the project structure, making it easy to manage server-side logic.
- Dynamic and static route generation: Next.js supports both dynamic and static route generation, allowing for efficient handling of various content pages.
Building Nested
Building Nested Routes is quite simple in Next.js. To create a nested route, first define the parent API route in app/api. Any subfolders added to the created parent route are treated as nested routes rather than separate routes.
You can access the parent route via the URL localhost:3000/api/username. Accessing the defined nested route http://localhost:3000/api/username/[your username] will produce the earlier result.
Check this out: Nextjs Proxy Api Route
To create a nested route, create a username folder for the route and define a dynamic route. For the purpose of this example, we will create a dynamic route [user] in the app/api/username directory.
Here's a table summarizing the key points about creating nested routes:
Nested route support is also available in Remix, which is built on top of React Router. This means that both frameworks have similar patterns for handling routing in applications.
Imports and Interface
You'll need to import the necessary modules to create a dynamic route in Next.js. This includes importing the GetStaticProps and GetStaticPaths functions from 'next'.
If you're using TypeScript, you'll also need to import the interface starInterface.
In addition to these imports, you'll also want to import the required components and modules for your dynamic route.
Here are the specific imports you'll need:
- GetStaticProps and GetStaticPaths from 'next'
- starInterface if you're using TypeScript
These imports will provide you with the necessary functions and interfaces to create a dynamic route in Next.js.
Routing Concepts
Next.js uses a file-system approach for routing, unlike React which uses code-based routing. This means you set up routes directly through page files.
You can define routes using TypeScript/JavaScript files that contain handler functions, just like Remix. This allows for route-based code splitting, which boosts site performance by loading only the necessary code for each page.
Route-based code splitting is a key feature of both Remix and Next.js v13.2. This means each route loads only the code necessary for that particular page, reducing the amount of code that must be loaded on the initial execution of the application.
Next.js has built-in support for nested routes, allowing pages and components to be created within folders. This means components created with a nested folder structure within the directory will be treated as nested routes of a defined parent route.
Here are the key similarities between Remix and Next.js v13.2 route handling:
- Defining and managing routes
- Route-based code splitting
- Nested route support
In Next.js, you can use dynamic routing by using square brackets for variable parameters in your page file names. For example, `[id].tsx` or `[something].tsx`. This allows you to insert any input you like for `[id]` and the page will load for this specific path.
Take a look at this: Nextjs Page Transition Loader
Use Cases and Setup
Dynamic routes in Next.js 13 allow us to create custom routing logic and handle incoming requests efficiently.
We can use Route Handlers to create dynamic routes that contain variable parameters, which can be used to generate dynamic content based on user actions.
A simple frontend blog application can use Static Site Generation to dynamically create any number of routes, such as post/1, post/post2, and so on.
The number of routes can vary and come from an external data source, like a database.
To enable pre-rendering for the specific fetched data for the paths in our dynamic route, we'll use getStaticProps().
getStaticProps() provides a context parameter that allows us to access params and the current identifier of our specific path.
If the user reaches out to /test/St2-18, the itemID would hold the value of St2-18.
We can access our backend data with getData() in getStaticProps(), and return the found item to the frontend.
Here's an interesting read: Nextjs Force Dynamic
Static Site Generation
Static Site Generation is a powerful feature in Next.js that allows us to pre-render our pages at build time, making our application faster and more efficient. This is achieved through the use of getStaticProps and getStaticPaths methods.
Next.js will pre-render the respective page at build time, but with dynamic routes, it doesn't know which paths to pre-render, that's where getStaticPaths comes in handy. It specifies which paths of the dynamic routing should be pre-rendered and/or how unknown paths should be handled.
getStaticPaths gets the list of paths from our mocked API function getPostIdList() and Next.js will generate the respective routes and also pass the context ahead to getStaticProps. This allows us to access params and then reach out to the current identifier of our specific path.
getStaticProps() can provide us with a context parameter through which we can reach some helpful methods, including params, which holds the value of the specific path identifier. For example, if the user reaches out to /test/St2-18, itemID would hold the value of St2-18.
To use getStaticProps(), we need to return the found data in the format shown above, so that Next.js can ensure our component function will get the postData prop. This is especially important when using dynamic routes and getStaticProps.
You might like: Router Query Params Nextjs 14
Data Fetching and Setup
To fetch data in Next.js with dynamic routing, you can use the Link element to lead the user to a dynamic route with a valid parameter, such as a product ID. This is especially useful when listing multiple items on a shop page.
Dynamic routing is the best approach for such cases, and it's essential to understand the getStaticProps() function, which you can learn more about in the official Next.js documentation.
The getData() function is crucial in fetching data from the backend, and it's used in conjunction with getStaticProps() and getStaticPaths() to fetch data for specific paths in the dynamic route. This function reads a JSON file, extracts the data, and converts it into a usable format.
A different take: Nextjs Dynamic Routing
Mocking API Data
Mocking API Data is a crucial step in setting up data fetching for your application.
To create mock data, you can add functions like getPostIdList() and getPostDetails() to your code. For example, a function like getPostIdList() can return a list of posts in a specific format, like this: id: 1, title: 'Post 1', content: 'This is post 1.'id: 2, title: 'Post 2', content: 'This is post 2.'
Next.js requires the value corresponding to the id key to be always a string. This means that in the response array, the id should be a string, not a number.
In a real-world application, you would replace this dummy data with actual API calls to get data from your data source.
See what others are reading: Next Js 13 Api Routes
Data Fetching
To start fetching data, you need to create functions that will provide data to your application. For demo purposes, you can use mocked data, but in a real-world application, you would want to replace this dummy data with actual API calls to get data from your data source.
You can create a function like getPostIdList() that returns a list of posts in a specific format. This format should have the same structure as shown in the example, with the id as the inner key.
The response array should have the same format as shown above, this will help next.js to generate paths for our routes dynamically.
Next.js requires the value corresponding to the id key to be always a string.
You can also create another function like getPostDetails() that returns some dummy data for each post.
Data fetching in Next.js with dynamic routing is a powerful approach, especially when you have a shop page with multiple items, each with a link for more information.
Take a look at this: Nextjs App Route Get Ssr Data
You can use the Link element to lead the user to a dynamic route with a valid parameter, such as the product id.
To create the data fetching function, you can prepare an async function called getData(), which will be helpful for the getStaticProps() and getStaticPaths() functions.
The getData() function will look for the file where you have your JSON data placed, read it, and extract the actual JSON data.
You can use the getData() function to reach out to your backend data and extract the specific information you need.
To fetch data for a specific path, you can pull the specific identifier from the URL and combine it with the extracted getData() result data.
You can use the itemID variable to search for the specific object in your backend data array and return the object that includes the itemID as an id.
Here's a table summarizing the steps to fetch data:
To setup getStaticProps(), you can use the getStaticProps() function to enable pre-rendering for the specific fetched data for the paths in your dynamic route.
You can access the current identifier of your specific path for which something is the placeholder through the context parameter.
If there is data, you can return your foundItem to the frontend.
Remember to access your backend data with getData() and return the props object to the frontend.
Sources
- https://nextjs.org/docs/app/building-your-application/routing
- https://blog.logrocket.com/using-next-js-route-handlers/
- https://hygraph.com/blog/nextjs-dynamic-routing
- https://www.freecodecamp.org/news/how-to-setup-dynamic-routing-in-nextjs/
- https://nextjs.org/docs/app/building-your-application/routing/route-handlers
Featured Images: pexels.com