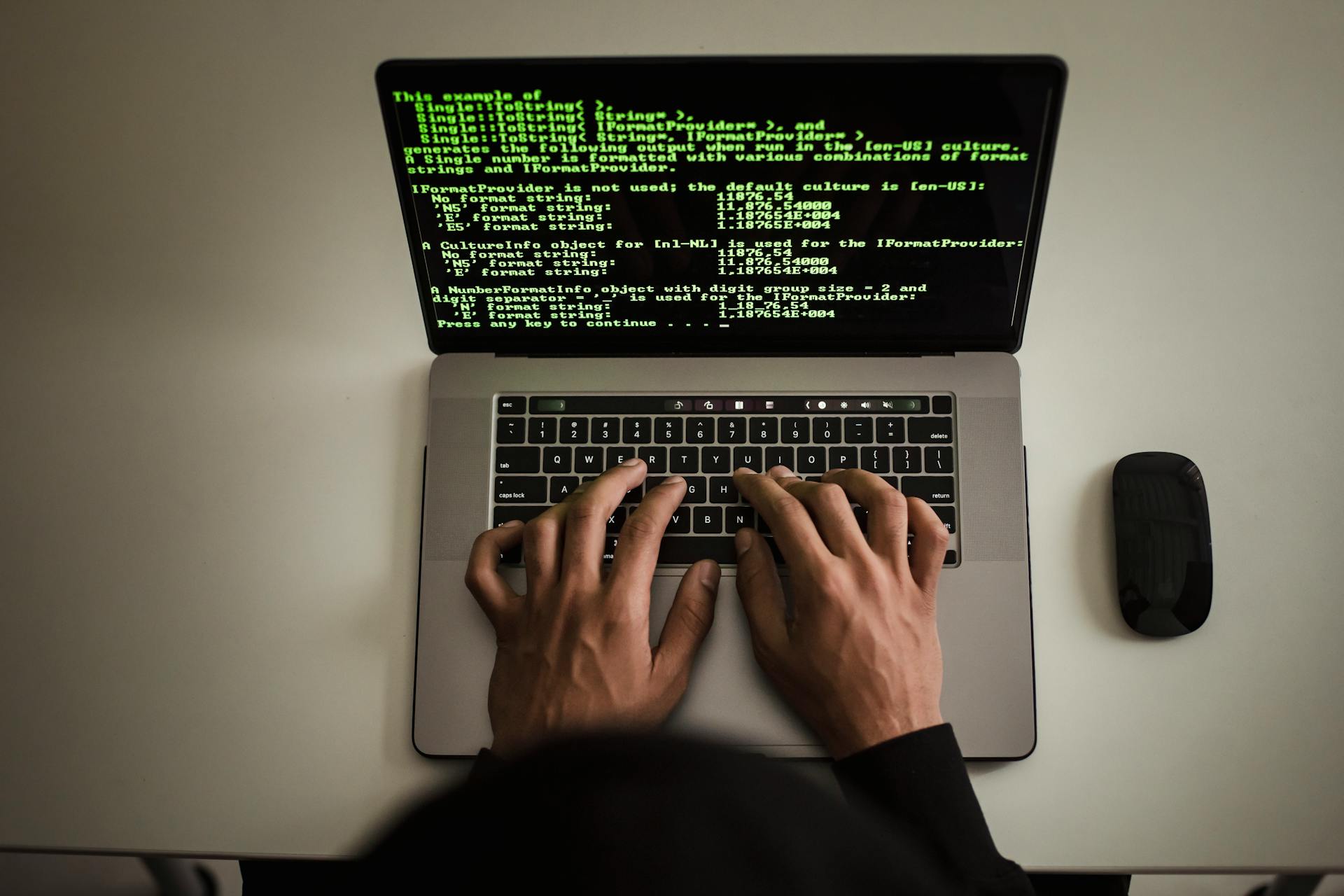
Next.js 13 Middleware is a game-changer for developers, allowing you to write server-side code in JavaScript.
With Next.js 13 Middleware, you can create custom middleware functions to handle requests and responses, giving you more control over your application's behavior.
Middleware functions can be used to authenticate users, validate requests, and perform other tasks before the request reaches the application.
A key feature of Next.js 13 Middleware is its ability to handle errors, making it easier to catch and handle exceptions in your application.
By using Next.js 13 Middleware, you can write more efficient and scalable code, reducing the load on your server and improving overall performance.
Broaden your view: Nextjs Pathname Server Component
Middleware Basics
Middleware in Next.js is a function that runs before a request is completed, allowing you to modify the request, perform certain actions, or even redirect users based on specific conditions.
Middleware functions in Next.js are defined using a standard structure that involves importing necessary types and functions from next/server and then exporting the middleware function.
Additional reading: Next Js Function Handlers to Filter Data
You can split complex middleware logic into separate modules and import them as needed to keep your code clean and modular.
The middleware function can be applied to specific routes or all API routes under a certain path, such as /api/*, by specifying the configuration object.
Here's a typical structure for defining middleware functions:
- Modular Middleware Logic: The customMiddlewareLogic function is imported from another file, allowing you to organize your middleware code more effectively.
- Configuration: The config object specifies that the middleware should apply to all API routes under /api/*.
Middleware Configuration
Middleware Configuration is crucial for controlling the flow of requests and responses in Next.js 13. You can place your middleware functions in the middleware.ts file, which should be in the root of your project.
To manage incoming requests, you can filter and process them based on various criteria, such as request paths, query parameters, or request headers. This allows you to apply specific logic before requests reach your route handlers.
Here are some key considerations for managing incoming requests:
- Filtering by Path: You can process requests that start with /api and log the request path.
- Using Config Object: The config object can specify that the middleware should apply to all API routes.
By controlling incoming requests, you can set custom request headers to include additional information or modify the request before it reaches the server components.
Convention
Convention plays a crucial role in Next.js middleware configuration. The middleware.ts file is where you define your middleware functions, and it should be placed in the root of your Next.js project.
You can define middleware functions to handle specific routes or patterns. For example, you can redirect users to the /home route if they try to access the /about route, as shown in the example.
The middleware.ts file is where you define your middleware functions.
To specify the route pattern that the middleware should apply to, you can use a matcher. This allows you to control the flow of requests and apply specific logic before they reach your route handlers.
Here are some key things to keep in mind when defining middleware functions:
- Place the middleware.ts file in the root of your Next.js project.
- Use a matcher to specify the route pattern that the middleware should apply to.
By following these conventions, you can set up your Next.js middleware configuration to handle incoming requests and outgoing responses effectively.
Session Management and Access Control
Session Management and Access Control is a crucial aspect of middleware configuration. Middleware can be used to protect sensitive data by checking user authentication and authorization before granting access to certain routes.
Middleware can manage user sessions by inspecting cookies or session objects and ensuring users are authenticated before accessing protected routes. This is achieved by checking for a user session cookie. If the user is not authenticated, it redirects them to the login page.
You can set up session token checks to verify user authentication. The middleware checks for a session token in the cookies. If the token is missing, it redirects the user to the login page.
Here's an example of how to implement session token checks:
- Session Token Check: The middleware checks for a session token in the cookies. If the token is missing, it redirects the user to the login page.
By implementing these checks, you can ensure that only authenticated users have access to sensitive data and routes. This is a best practice for protecting user data and maintaining application security.
Managing Incoming Requests
Managing incoming requests in Next.js middleware allows you to filter and process requests based on various criteria such as request paths, query parameters, or request headers.
You can filter incoming requests based on their path, for example, by processing requests that start with /api and logging the request path.
The config object can be used to specify that the middleware should apply to all API routes.
Here's a table summarizing how to filter incoming requests by path:
You can also set custom request headers to include additional information or to modify the request before it reaches the server components.
Request and Response Management
In Next.js 13 middleware, you can directly respond from the middleware by returning a Response or NextResponse instance, a functionality introduced in version 13.1.0.
You can filter and process incoming requests based on various criteria like request paths, query parameters, or request headers, allowing you to control the flow of requests and apply specific logic before they reach your route handlers.
The middleware can process requests that start with /api and logs the request path.
Here are some ways to filter incoming requests:
- Filtering by Path: The middleware processes requests that start with /api and logs the request path.
- Using Config Object: The config object specifies that the middleware should apply to all API routes.
You can set custom request headers to include additional information or to modify the request before it reaches the server components.
Directly returning responses or error messages from the middleware can be useful for early exits or error handling, such as returning a 401 Unauthorized response with an error message if the Authorization header is missing.
The middleware can also be used to protect sensitive data by checking user authentication and authorization before granting access to certain routes.
Here are some ways to manage user sessions:
- Session Token Check: The middleware checks for a session token in the cookies. If the token is missing, it redirects the user to the login page.
- User Authentication: The middleware checks for a user session cookie. If the user is not authenticated, it redirects them to the login page.
API Routes and Security
API routes are a crucial part of any web application, and Next.js 13 middleware provides a powerful way to secure them.
In Next.js, all API routes must lie in a subfolder "api" in the "app" directory.
To protect sensitive data, middleware can inspect incoming requests to verify user authentication and enforce access control policies. This is crucial for securing API routes.
The middleware is executed before the route's logic is reached, and it can be used to protect routes like /api/users and /api/admin.
The properties matcher in the middleware config variable supports the wildcard syntax, so you can match a group of routes like /api/users/:path*.
Here's a breakdown of the middleware config variable:
If the authorization value in the request header doesn't match, the middleware redirects the user to the unauthorized route that will return a 401 status code.
The code of the API route /api/auth/unauthorized is straightforward, and it returns a 401 status code when the authorization value is invalid or missing.
The middleware checks the Authorization header for a valid token and returns a 401 Unauthorized response if the token is invalid or missing.
On a similar theme: Next Js Fetch Data save in Context and Next Route
Middleware Use Cases
Middleware can be used to check if a user is authenticated before allowing them to access certain routes.
You can use middleware to log requests, responses, or other information related to the application.
Middleware can be used to handle errors that occur during the request processing.
Here are some common use cases where you might want to use middleware:
- Authentication: Checking if a user is authenticated before accessing certain routes.
- Logging: Logging requests, responses, or other information related to the application.
- Error Handling: Handling errors that occur during the request processing.
- Caching: Caching responses or data to improve performance.
- Request Processing: Modifying or processing requests before they reach the final destination.
- Bot Detection: Detecting and blocking bots or malicious requests.
Protecting API routes with middleware is crucial for protecting sensitive data and ensuring that only authorized users can access specific endpoints.
Middleware can inspect incoming requests to verify user authentication and enforce access control policies.
To focus on middleware implementation, you will do it in one place, and it works for all the routes, unlike adding the authorization check on each route.
Error Handling and Logic
You can implement custom logic in middleware to handle specific scenarios, such as logging or modifying requests based on custom rules.
Middleware allows you to catch errors and return a meaningful response to users when something goes wrong. This is crucial for providing a good user experience.
The middleware can log a message when a specific route is accessed, like the /special route.
Here are some key aspects of error handling and custom logic in Next.js 13 middleware:
This approach ensures that users receive feedback when something goes wrong, rather than just a generic error message.
Best Practices and Optimization
To get the most out of Next.js 13 middleware, it's essential to follow some best practices.
Minimize middleware complexity by keeping functions lightweight and reducing latency. This will ensure your application remains fast and responsive.
Middleware should be scoped properly to avoid unnecessary complications. Only apply middleware where necessary to keep things simple.
Use middleware for generic logic that applies across multiple routes. This will help keep your code organized and maintainable.
Here are some key takeaways to keep in mind:
- Minimize middleware complexity
- Scope properly
- Use middleware for generic logic
- Combine multiple pieces of middleware if necessary
By following these best practices, you can optimize your Next.js 13 middleware for better performance and security.
Best Practices
As you optimize your application, it's essential to keep middleware complexity in check. Minimize middleware functions by keeping them lightweight to reduce latency introduced by each request.
Overusing or mis-scoping middleware can lead to unnecessary complications. Only apply middleware where necessary to avoid this issue.
Middleware is best used for shared logic that applies across multiple routes. This helps keep your code organized and efficient.
If your middleware becomes too complex, consider splitting it up and chaining multiple middleware functions. This can make your code more manageable and easier to maintain.
Explore further: Nextjs Multiple Middlewares
Optimizing Content
Optimizing Content can greatly improve the user experience and reduce server load. By using middleware to optimize static and dynamic content, you can ensure that images are served efficiently.
Cache Control Headers are set by middleware to improve load times and reduce server load. This is done by setting the right cache headers for image files.
Middleware can also be used to redirect users to a new page, enhancing navigation and user experience. For example, users accessing /old-page are redirected to /new-page.
Here's a summary of the benefits of optimizing content:
- Improved load times
- Reduced server load
- Enhanced user experience
Sources
- https://nextjs.org/docs/app/building-your-application/routing/middleware
- https://www.geeksforgeeks.org/middlewares-in-next-js/
- https://codeparrot.ai/blogs/nextjs-middleware-simple-guide-to-control-requests
- https://blog.tericcabrel.com/protect-your-api-routes-in-next-js-with-middleware/
- https://www.dhiwise.com/post/how-to-secure-your-api-routes-with-nextjs-middleware
Featured Images: pexels.com