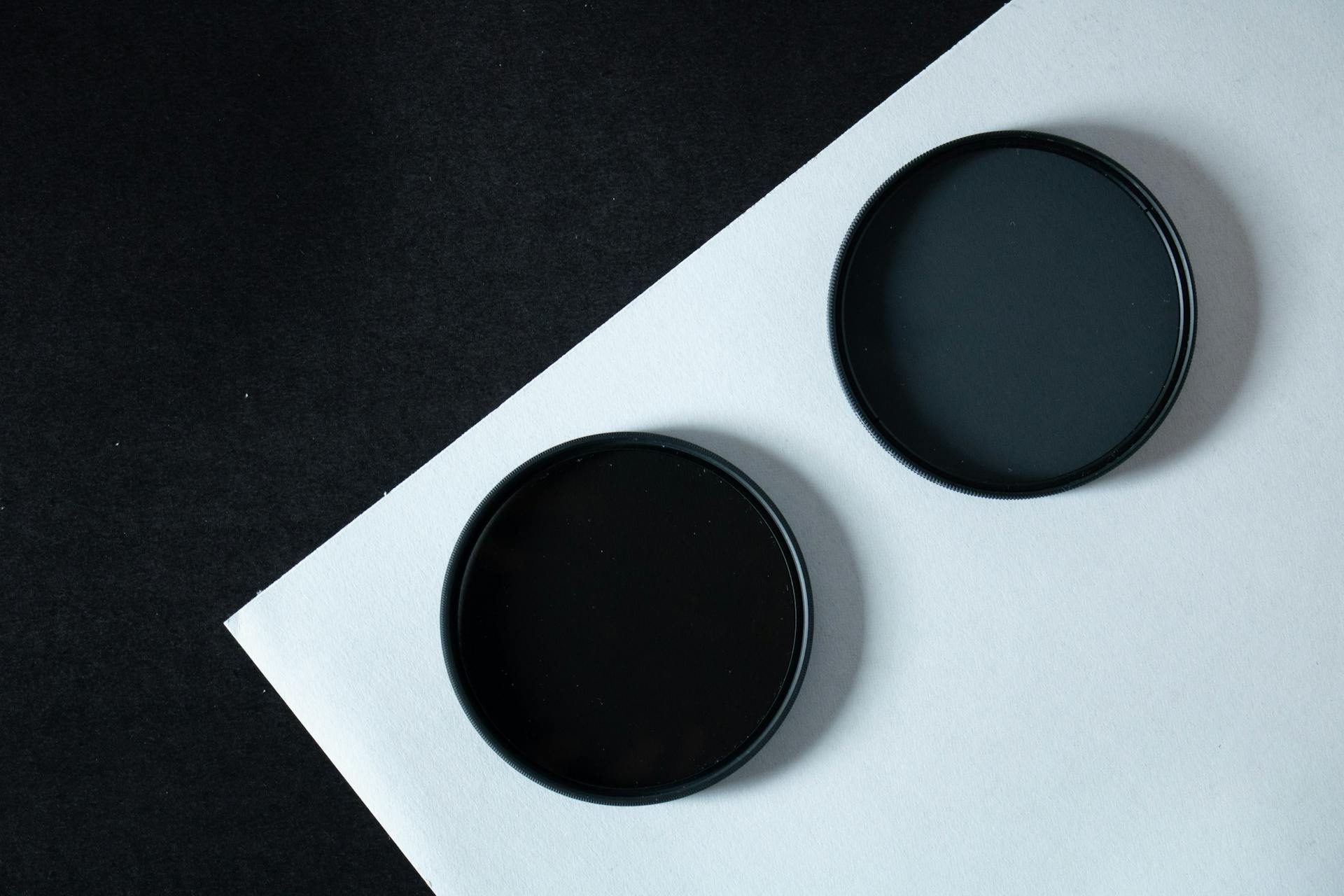
Next.js function handlers provide a powerful way to filter data in your application. They allow you to create custom APIs that can handle different types of requests and return filtered data.
To create a function handler, you can use the `next-api` middleware in Next.js. This middleware provides a simple way to create API routes that can handle GET, POST, PUT, and DELETE requests.
A function handler can be as simple as a single function that takes in a request and returns a response. For example, a handler might look like this: `export default async function handler(req, res) { return res.json({ message: 'Hello World!' }); }`.
Function handlers can also be used to filter data based on parameters passed in the URL. For example, if you have a list of users and you want to filter them by age, you can create a function handler that takes in the age parameter and returns the filtered list.
Explore further: Why Is It Important to Filter Data
Creating Function Handlers
Creating function handlers is a crucial step in filtering data with Next.js.
You can create a function handler by using the `getServerSideProps` method in your page component. This method allows you to fetch data on the server and pass it to the page component as props.
In the example shown in the article, the `getServerSideProps` method is used to fetch data from a JSON file and pass it to the page component as props.
The `getServerSideProps` method is called by Next.js on the server before rendering the page, allowing you to filter data before it's sent to the client.
By using `getServerSideProps`, you can create a robust and efficient data filtering system for your Next.js application.
A different take: Nextjs Pathname Server Component
Defining Function Handlers
Function handlers are a crucial part of creating a robust and efficient function handler system. They are essentially a way to handle different functions in a program.
A function handler can be thought of as a switch statement on steroids, allowing you to handle different functions based on a specific condition. This is evident in the example where a function handler is used to handle different HTTP requests.
The function handler can be defined using a simple syntax, such as `function handler(request) { ... }`. This syntax is used in the example where a function handler is created to handle different routes in a web application.
Function handlers can also be used to handle errors, making your code more robust and less prone to errors. This is demonstrated in the example where a function handler is used to catch and handle errors in a program.
The function handler can be defined to handle specific errors, such as HTTP errors, using a specific syntax. This syntax is used in the example where a function handler is used to handle HTTP errors in a web application.
Function handlers can be used to handle a wide range of functions, from HTTP requests to database queries. They provide a flexible and efficient way to handle different functions in a program.
Explore further: Speed up a Next Js Application Fetching Data
Using getServerSideProps
Using getServerSideProps is a game-changer for handling server-side rendering in Next.js. It allows us to pre-render pages on the server, which is especially useful for SEO and performance.
Take a look at this: Next Js Server Side Api Call
getServerSideProps takes an object as an argument, which we can use to fetch data from an API or database. This data is then passed to the page component as props, making it easily accessible.
We can also use getServerSideProps to handle authentication and authorization, by checking if the user is logged in or not. This is useful for protecting routes that require a login.
By using getServerSideProps, we can keep our page components simple and focused on rendering the UI, while handling the complex logic of data fetching and authentication on the server. This separation of concerns makes our code more maintainable and scalable.
getServerSideProps can also be used to handle caching, by storing the fetched data in memory or a database. This can improve performance by reducing the number of requests made to the API or database.
By pre-rendering pages on the server, we can provide a better user experience, especially for users with slow internet connections. This is because the page is already rendered when it's sent to the client, rather than waiting for the client to render it themselves.
Suggestion: Next Js Client Portal
Error Handling
Error handling is crucial in function handlers, as seen in the example of the `divide` function. It should be able to handle cases where the divisor is zero, which can cause a runtime error.
A try-catch block can be used to catch and handle such errors. In the `divide` function, a try block is used to enclose the division operation, and a catch block is used to catch any errors that occur.
The `catch` block in the `divide` function is used to return a specific error message when the divisor is zero. This is a good practice to follow when writing function handlers.
A function handler should always validate its input to prevent errors. In the `divide` function, input validation is performed to ensure that the divisor is not zero.
The `catch` block in the `divide` function also includes a statement to rethrow the error, which allows the error to propagate up the call stack and be handled by a higher-level error handler.
Take a look at this: Can Nextjs Be Used on Traditional Web Application
Filtering Data with Function Handlers
Filtering data with function handlers is a powerful technique in Next.js, allowing you to easily manage complex data flows.
By using function handlers, you can filter data based on specific conditions, as demonstrated in the example where the `getServerSideProps` function handler filters data based on the `req.query` object. This enables you to dynamically control what data is displayed to users.
Function handlers can also be used to filter data based on user input, such as in the example where the `getStaticProps` function handler filters data based on the `req.query.search` parameter. This allows you to create dynamic and interactive data displays.
Intriguing read: Tanstack Query Nextjs
Basic Filtering
Basic Filtering is a crucial step in data processing. It helps to extract the relevant information from a large dataset.
In the previous section, we saw an example of using a function handler to filter data based on a specific condition. The function handler returned a new array with only the items that met the condition.
You can use the same approach to filter data based on multiple conditions. For example, you can use a function handler to filter data based on both a minimum age and a maximum age.
The function handler will return a new array with only the items that meet both conditions. This is a powerful way to refine your data and get exactly what you need.
By using function handlers for filtering, you can create complex and customized filters that meet your specific needs. This can be especially useful when working with large datasets.
Discover more: Next Js Use Effect
Advanced Filtering Techniques
In advanced filtering techniques, we can use the `filter()` method to narrow down data based on a specific condition. This method returns a new array with all elements that pass the test implemented by the provided function.
For example, if we have an array of numbers and we want to get only the even numbers, we can use the `filter()` method with a function that checks if a number is even.
A unique perspective: How to Use Reducer Api in Next Js 14
The `filter()` method can be used in conjunction with other methods, such as `map()` and `reduce()`, to perform more complex data transformations.
In the example of filtering a list of students based on their grades, we can use the `filter()` method to get only the students with a grade above 85.
By using advanced filtering techniques, we can make our data processing tasks more efficient and easier to manage.
For instance, if we have a list of objects and we want to get only the objects with a specific property, we can use the `filter()` method with a function that checks if the property exists.
The `filter()` method is a powerful tool for data filtering, and by mastering it, we can write more efficient and effective code.
Best Practices for Function Handlers
When writing function handlers in Next.js, it's essential to keep them lightweight and efficient. This means avoiding unnecessary code and focusing on a single task.
Function handlers should be short and sweet, ideally no more than 10-15 lines of code. This is because Next.js is designed to handle server-side rendering and static site generation, so longer handlers can slow down performance.
Use a clear and descriptive name for your function handler, making it easy to identify its purpose. For example, `getPosts` is a straightforward name for a function handler that retrieves a list of posts.
Function handlers should always return a response, whether it's a JSON object, a redirect, or an error message. This ensures that the client receives a valid response, even if the function handler encounters an issue.
In Next.js, you can use the `useEffect` hook to fetch data and update the component state. However, in function handlers, this hook is not available, and you should use the `fetch` API or a library like Axios instead.
Use the `next/head` component to set metadata, such as the title and description, for your pages. This helps with search engine optimization (SEO) and improves the user experience.
Function handlers can also be used to handle errors and exceptions. By using a try-catch block, you can catch and handle errors, providing a better experience for your users.
On a similar theme: Next Js Fetch Data save in Context and Next Route
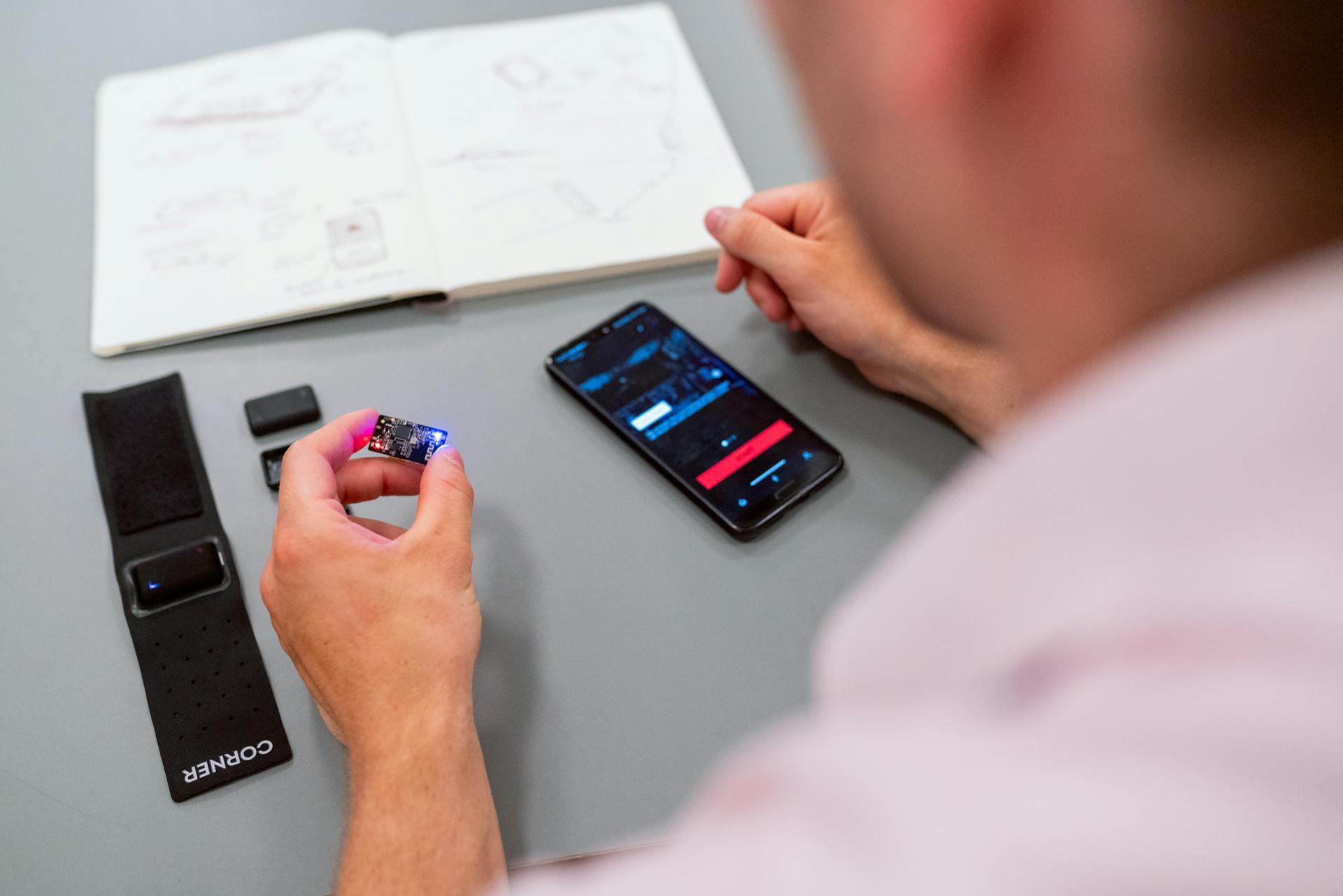
In Next.js, you can use the `getStaticProps` function to pre-render pages at build time. However, in function handlers, this function is not available, and you should use the `getServerSideProps` function instead.
Use the `useRouter` hook to access the router object and navigate to different pages. However, in function handlers, this hook is not available, and you should use the `res.redirect` method instead.
A fresh viewpoint: Next Js Pages
Sources
- https://marmelab.com/react-admin/DataProviders.html
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://nextjs.org/docs/app/building-your-application/routing/route-handlers
- https://www.robinwieruch.de/next-server-actions-fetch-data/
- https://docs.sentry.io/platforms/javascript/guides/nextjs/configuration/filtering/
Featured Images: pexels.com