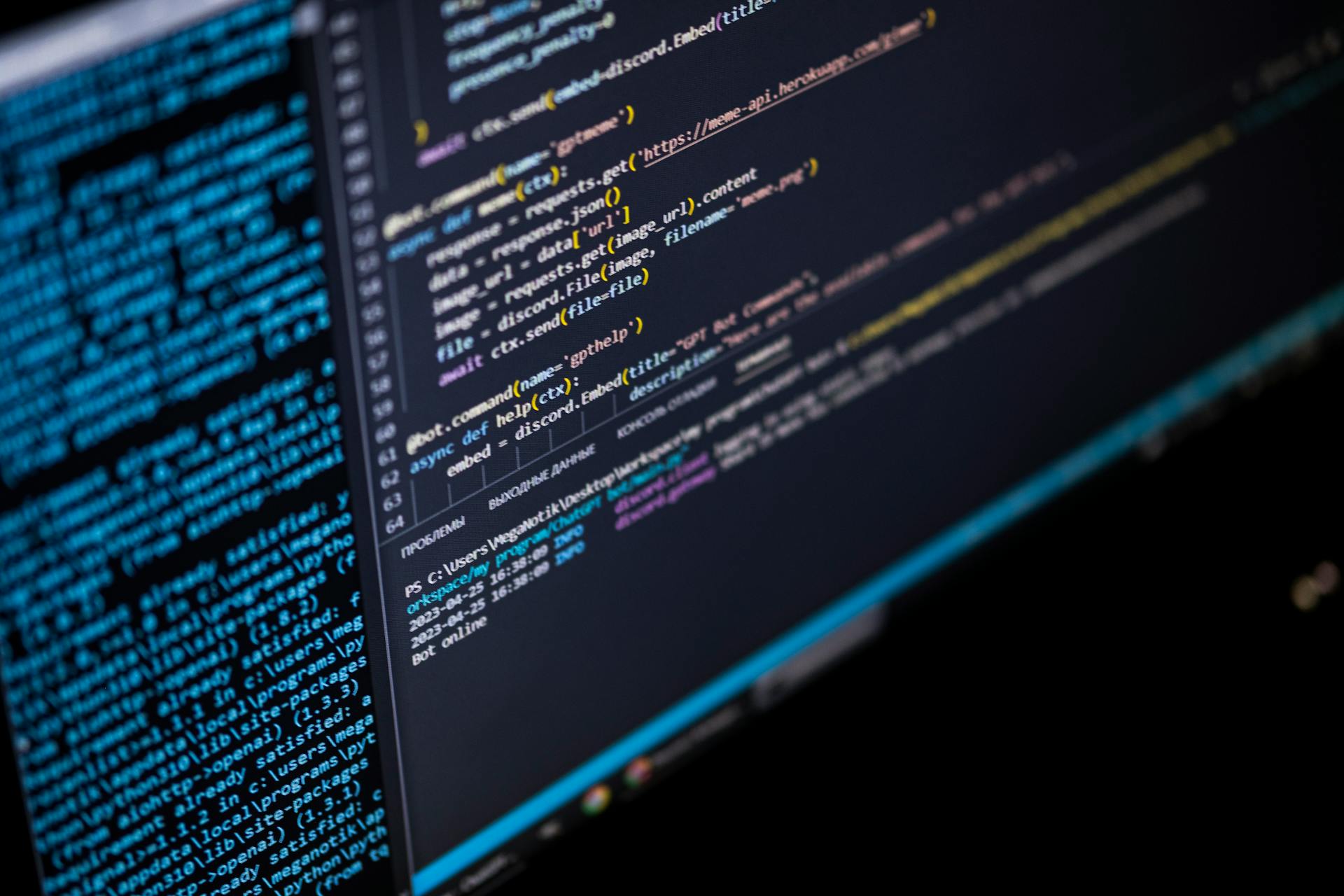
Building Next.js Applications with TanStack Query is a powerful combination that can take your web development to the next level. TanStack Query is a powerful data fetching and caching library that can be used with Next.js to build fast and efficient applications.
By using TanStack Query with Next.js, you can create a seamless user experience with instant page loads and fast data retrieval. This is achieved through TanStack Query's ability to cache data in the browser and server, reducing the number of requests made to the server.
TanStack Query also provides a simple and intuitive API for managing data fetching and caching, making it easy to integrate into your Next.js application. With TanStack Query, you can focus on building your application's logic without worrying about the intricacies of data fetching and caching.
By leveraging TanStack Query's features, you can build Next.js applications that are not only fast and efficient but also scalable and maintainable.
Explore further: Nextjs Pathname Server Component
Getting Started with Next.js
To get started with Next.js, you'll need to create a new project using the Next.js CLI. This will ask you to choose between a pages-based or an app-based directory, so opt for a pages-based directory for client-side rendering by default.
You can quickly spin up a new Next.js project using the CLI, which will give you a basic boilerplate app.
The Next.js CLI will ask you to choose between a pages-based or an app-based directory. For this section, it's recommended to choose a pages-based directory.
Once your app finishes installing, you will have a pages-based Next.js boilerplate app.
For your interest: Nextjs 14 New Features
Building with TanStack Query
TanStack Query is not just a data-fetching library, it's a powerful tool that handles caching, mutating requests, automatic background data refresh, and more.
To use TanStack Query with Next.js 13, you'll need to install the ReactQueryStreamedHydration API, which allows you to fetch data on the server itself during the initial request.
This API is a game-changer for Next.js 13, making it easy to integrate TanStack Query and take advantage of its features.
The ReactQueryStreamedHydration API allows you to fetch data on the server and then hydrate your UI with the received data.
To get started, create a new Next.js 13 project and install the necessary packages, including ReactQueryStreamedHydration.
Once you have the API set up, you can use it to fetch data on the server and hydrate your UI, making it a seamless experience for your users.
TanStack Query also supports prefetching data on the server and sending it along with your server components, which can improve performance and reduce the time it takes for your app to load.
However, if you're using Next.js 12 or earlier, you'll need to use a different approach to integrate TanStack Query, which involves setting up a demo project and understanding how data handling works with TanStack Query in Next.js.
In Next.js 12 or earlier, you can install TanStack Query and use the useQuery hook to fetch data, but you'll need to use a different approach to hydrate your queries on the server.
Discover more: Next.js
TanStack Query also supports mutations, which are used for modifying data, and you can use the useMutation hook to perform mutations on your data.
Overall, TanStack Query is a powerful tool that can help you build fast and scalable applications with Next.js, but it does require some setup and configuration to get it working correctly.
By following the examples and guidelines provided, you can easily integrate TanStack Query into your Next.js project and take advantage of its features to build a fast and scalable application.
With TanStack Query, you can prefetch data on the server and send it along with your server components, which can improve performance and reduce the time it takes for your app to load.
However, if you're using the experimental streaming without prefetching approach, you'll need to use the @tanstack/react-query-next-experimental package and wrap your app in the ReactQueryStreamedHydration component.
This approach can provide a good tradeoff between DX/iteration/shipping speed and performance, but it's still an experimental feature and may have some limitations.
Overall, TanStack Query is a powerful tool that can help you build fast and scalable applications with Next.js, but it does require some setup and configuration to get it working correctly.
See what others are reading: Next Js Call Api on the Server Example
Components and Data Management
You can build purely client components using the useState Hook, and TanStack Query will ensure a smooth experience.
To prefetch data and dehydrate/hydrate it, you can create a Server Component to do the prefetching part. This works similarly in any framework that supports Server Components, with the only Next.js-specific part being the file names.
A good rule of thumb is to avoid using queryClient.fetchQuery unless you need to catch errors, and treat Server Components as a place to prefetch data, nothing more.
See what others are reading: Next Js Components Folder
User Display Page
The user display page is a crucial part of our application, where we'll show the list of robots.
To fetch the list of robots, we can use the useQuery Hook in the Robots.tsx file, which takes in a function as a parameter.
This function is the getUsers function, which is defined above the JSX.
We'll set the staleTime option to 5 * 1000, meaning the fetched data will go stale after 5 seconds.
The useQuery Hook also accepts a unique key, suspense property, and the getUsers function, allowing us to customize the fetching behavior.
Consider reading: Next Js Function Handlers to Filter Data
Adding a Client-Side Counter Component
Adding a client-side Counter component to your project is a great way to manage data locally. You can use the useState Hook to create a basic counter state that can be incremented, decremented, or reset from the client side.
TanStack Query will ensure that everything runs smoothly, even when working with client-side components. This means you can focus on building your application without worrying about data management.
The Counter component is a simple example of how to use the useState Hook. By creating a basic counter state, you can easily manage the data locally and respond to user interactions.
With the useState Hook, you can update the counter state in real-time. This makes it easy to build interactive components that respond to user input.
You might enjoy: Next Js Client Portal
Nesting Server Components
Nesting Server Components can be a powerful tool for managing data in your application. This is because Server Components can be nested and exist on many levels in the React tree.
Related reading: Nextjs Server Only Code
By nesting Server Components, you can prefetch data closer to where it's actually used, rather than only at the top of the application. This can be as simple as a Server Component rendering another Server Component.
If you're not careful, this can lead to a server-side waterfall, where each component waits for the previous one to finish before rendering. However, if the server latency to the data is low, this might not be a huge issue.
In Next.js, you can avoid this issue by prefetching data in parallel routes. This is because Next.js knows how to fetch all the data in parallel when you use parallel routes.
On a similar theme: Nextjs Component Library
Data Ownership and Validation
Server Components can own some data, and Client Components can own other data, but it's crucial to keep these two realities in sync.
If you're rendering data from a query in both a Server Component and a Client Component, React Query has no idea how to revalidate the Server Component when the query revalidates on the client.
Using React Query with Server Components makes most sense in specific scenarios: you're migrating to Server Components without rewriting data fetching, you want a familiar programming paradigm with Server Components benefits, or you need a use case that React Query covers but your framework doesn't.
A good rule of thumb is to avoid using queryClient.fetchQuery unless you need to catch errors.
Here are some scenarios where React Query and Server Components might not play nicely together:
- You have an app using React Query and want to migrate to Server Components without rewriting all the data fetching.
- You want a familiar programming paradigm, but want to still sprinkle in the benefits of Server Components where it makes sense.
- You have some use case that React Query covers, but that your framework of choice does not cover.
If you do use React Query with Server Components, don't render its result on the server or pass the result to another component, even a Client Component one.
Data Validation and Streaming
Data ownership and revalidation is a crucial aspect to consider when using Server Components with React Query. If you're rendering data from a query in both a Server Component and a Client Component, you'll need to think about how to keep the data in sync.
To avoid issues, it's best to treat Server Components as a place to prefetch data, nothing more. This means avoiding rendering query results on the server or passing them to other components, even Client Components.
If you do use queryClient.fetchQuery, a good rule of thumb is to avoid it unless you need to catch errors. This will help prevent data inconsistencies.
Here are some scenarios where pairing React Query with Server Components makes sense:
- You have an existing app using React Query and want to migrate to Server Components without rewriting data fetching.
- You want a familiar programming paradigm but want to still sprinkle in the benefits of Server Components where it makes sense.
- You have a use case that React Query covers, but your framework of choice doesn't.
To make streaming with Server Components work, you need to instruct the queryClient to dehydrate pending Queries. This can be done globally or by passing the option directly to dehydrate.
Here are the steps to follow:
1. Move the getQueryClient() function out of your app/providers.tsx file.
2. Use the queryClient to dehydrate pending Queries.
3. Provide a HydrationBoundary on the client.
4. Use useSuspenseQuery or useQuery to "use" the Promise created on the server.
Note that you can specify the dehydrate.serializeData and hydrate.deserializeData options to serialize and deserialize non-JSON data types on each side of the boundary.
Sources
- https://blog.logrocket.com/using-tanstack-query-next-js/
- https://brockherion.dev/blog/posts/setting-up-and-using-react-query-in-nextjs/
- https://tanstack.com/query/latest/docs/framework/react/guides/advanced-ssr
- https://prateeksurana.me/blog/mastering-data-fetching-with-react-query-and-next-js/
- https://nextjs.org/docs/14/app/building-your-application/data-fetching/fetching-caching-and-revalidating
Featured Images: pexels.com