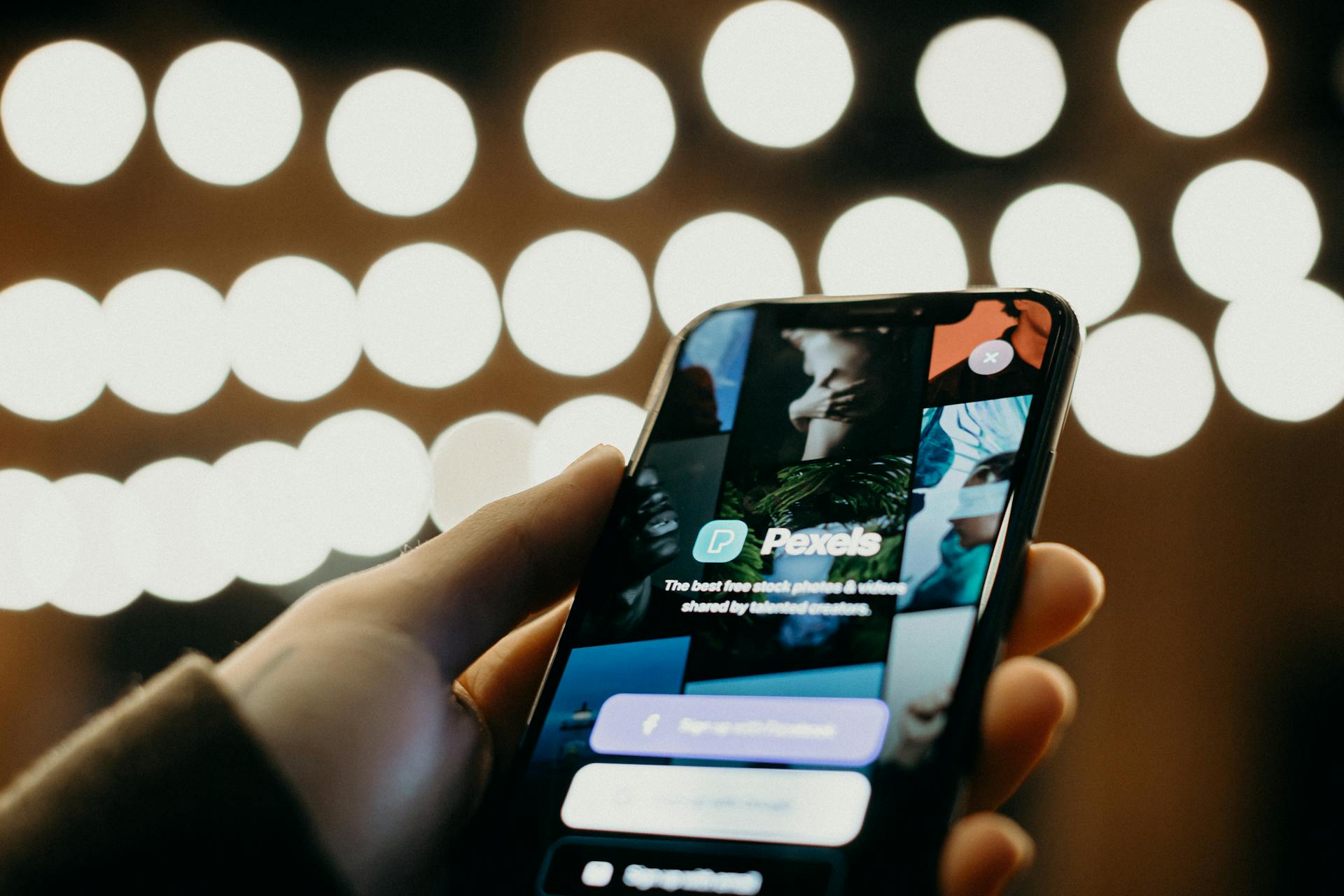
Next.js Force Dynamic Performance Optimization and Dynamic Routing Strategies are crucial for building fast and scalable applications. This is because Next.js Force Dynamic allows for server-side rendering and static site generation, which can significantly improve page load times.
By optimizing performance, developers can create a better user experience and improve search engine rankings. Next.js Force Dynamic's built-in performance optimization features, such as code splitting and dynamic imports, can help achieve this goal.
Next.js Force Dynamic's dynamic routing strategy, which uses a page-based routing system, allows for efficient routing and easy maintenance of routes. This approach also enables developers to create complex routes with ease.
By combining performance optimization and dynamic routing strategies, developers can build Next.js Force Dynamic applications that are both fast and scalable.
A different take: Nextjs Loading Page Ssr
Routing and API Rendering
In Next.js, dynamic routing allows you to load the same component with different data based on the route.
By using dynamic routing, you can avoid redundant code and make your components reusable. This is especially useful for scenarios like a blogging website where users can choose from available blogs.
Discover more: Dynamic Route Nextjs 13
You can implement dynamic routing in multiple ways, but one simple approach is to create a component named Dynamic routing with a file as [id].jsx, where the id is a dynamic field passed in the route.
The dynamic id can be fetched in the component using the router hook, as seen in the example where the id is passed from the browser.
You can pass the id of the article and your logic will remain intact and reusable. This means you can load the same component with different data based on the route.
To access the add and edit form, you can simply load the route http://localhost:3000//internal/addEdit/add for the add page and http://localhost:3000//internal/addEdit/edit for the edit page.
Here are some examples of dynamic routes:
- http://localhost:3000//internal/addEdit/add
- http://localhost:3000//internal/addEdit/edit
These routes can be used to load the same component with different data based on the route.
Performance Optimization
In Next.js, optimizing performance is crucial for a smooth user experience. Caching is a key aspect of this optimization.
The App Router has four separate caches, but the route cache is the most likely to cause issues. If your application accepts login and renders different data based on the user, you'll need to disable the route cache by using the dynamic export from the route handler.
After a mutation, it's essential to invalidate the cache by calling revalidatePath or revalidateTag as appropriate. This ensures that the cache is updated correctly and doesn't cause any issues.
Max Duration
Max Duration is a crucial setting for optimizing performance in Next.js applications.
You can use the maxDuration property to limit the execution time of server-side logic, such as page rendering or API processing.
Some deployment platforms, like Vercel, allow you to set a specific execution limit using the maxDuration value from the Next.js build output.
Note that this setting requires Next.js 13.4.10 or later.
To set a default timeout for all Server Actions on a page, you can set the maxDuration property at the page level.
Curious to learn more? Check out: Nextjs Loading Indicator Server
Caching
Caching can be a real performance booster, but it can also cause issues if not managed correctly. The App Router has four separate caches, including fetch request and route caches.
The route cache is the most likely to cause problems, especially if your application accepts login and renders different data based on the user. To avoid issues, you may need to disable the route cache by using the dynamic export from the route handler.
After a mutation, it's essential to invalidate the cache by calling revalidatePath or revalidateTag as appropriate. This ensures that the cache is updated with the latest changes.
Function Parameters and Return
In Next.js, dynamic routes are enabled by default, but you can disable them by setting `enabled: false` in your `next.config.js` file. This is useful for specific use cases.
You can define dynamic routes using the `getStaticPaths` function, which returns an array of possible paths for a page. For example, `getStaticPaths: () => ({ paths: [{ params: { id: '1' } }] })`.
Dynamic routes in Next.js are useful for creating pages that can handle multiple URLs, such as a blog post page that can handle multiple post IDs.
Consider reading: Next.js
Params
Params play a crucial role in function calls. They allow you to pass data to a function, which can then use that data to perform different actions.
The `dynamicParams` option controls the behavior when a dynamic segment is visited that was not generated by `generateStaticParams`. It defaults to `true`.
You can think of `dynamicParams` as a way to decide what happens when a dynamic segment is visited that wasn't accounted for in `generateStaticParams`. If `dynamicParams` is `true`, the segment will be created dynamically if needed.
Here are the possible values for `dynamicParams`:
- `true` (default): Dynamic segments are created dynamically if needed.
- `false`: Dynamic segments that weren't included in `generateStaticParams` return a 404.
This option replaces the `fallback` option in `getStaticPaths` in the `pages` directory.
Return
When working with function parameters and return, it's essential to understand how dynamic rendering works in app directories. The new model in app directories prefers fine-grained caching control at the fetch request level over the binary all-or-nothing model of getServerSideProps and getStaticProps at the page level.
The dynamic option provides a convenient way to revert to the previous model, offering a simpler migration path. This option is particularly useful when working with complex layouts or pages that require dynamic rendering.
You might enjoy: Loading Indicator in Next Js App Route Change
The dynamic option can take several values, including 'auto', which caches as much as possible and prevents any components from being dynamic. Another value is 'force-dynamic', which forces dynamic rendering and re-renders the route for each user request.
Here are the possible values for the dynamic option:
- 'auto' (default): Caches as much as possible and prevents any components from being dynamic.
- 'force-dynamic': Forces dynamic rendering and re-renders the route for each user request.
- 'error': Causes an error if there are dynamic functions or uncached data in components, rendering the layout or page statically and caching the data.
- 'force-static': Forces static rendering of the layout or page, caching the data, by returning empty values from cookies(), headers(), and useSearchParams().
Frequently Asked Questions
Is Next.js static or dynamic?
Next.js offers a unique approach that combines static and dynamic rendering, allowing for cached and uncached data in dynamically rendered routes. This hybrid approach enables dynamic rendering without the performance impact of fetching all data at request time.
Sources
- https://nextjs-ko.org/docs/app/api-reference/file-conventions/route-segment-config
- https://redux.js.org/usage/nextjs
- https://javascript.plainenglish.io/different-ways-of-handling-dynamic-routing-in-next-js-312006f5ba1b
- https://mattermost.com/blog/dynamic-routing-in-next-js-using-rest-and-graphql-apis/
- https://nextjs.org/docs/app/building-your-application/rendering/server-components
Featured Images: pexels.com