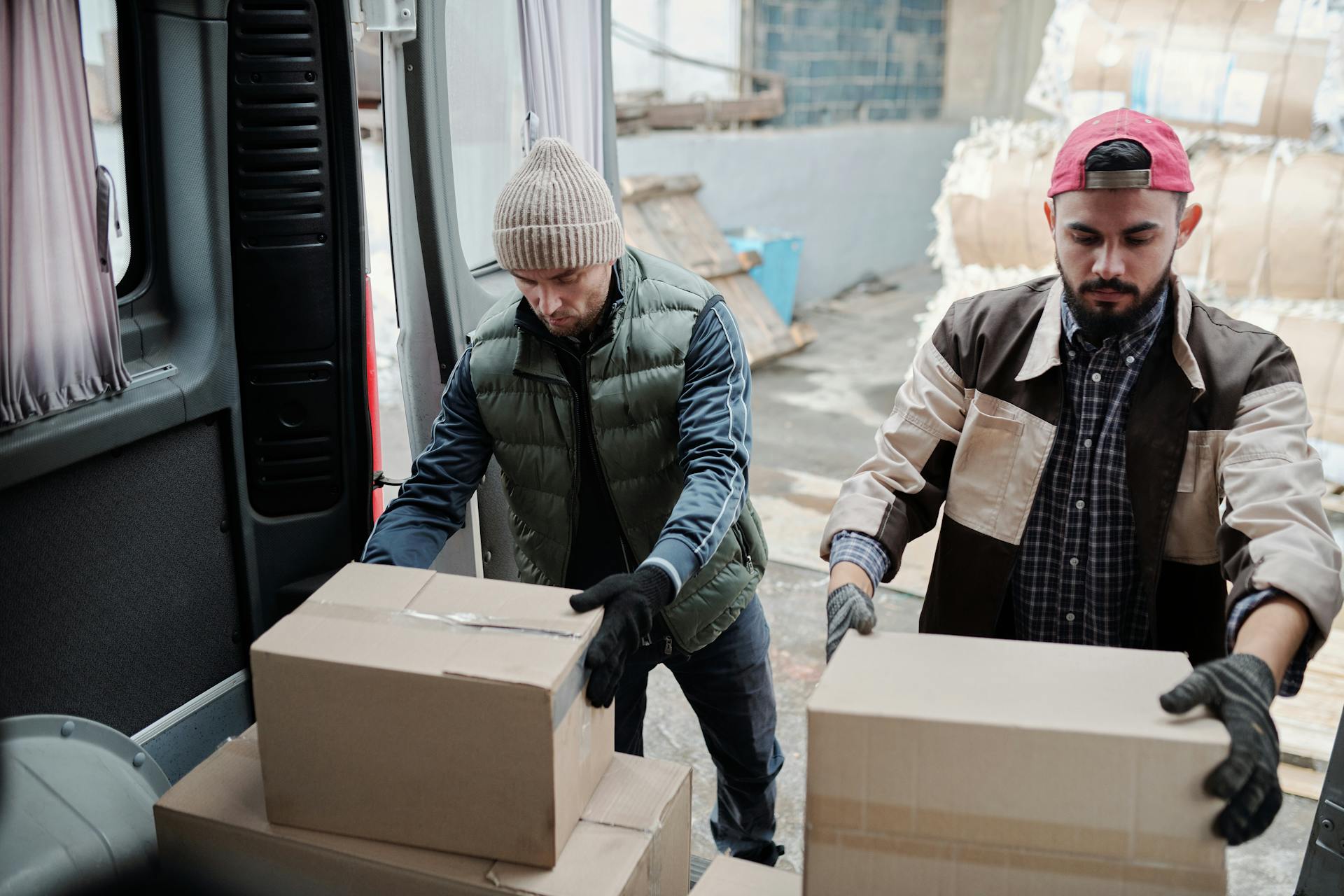
Loading indicators are a crucial aspect of creating a seamless user experience in Next.js apps, especially during route changes. They provide visual cues to users that the app is still functioning and loading the new page.
A common approach to implementing loading indicators in Next.js apps is to use the `useEffect` hook in combination with the `useRouter` hook from the `next/router` package. This allows developers to detect route changes and display a loading indicator accordingly.
To get started, you can use the `useRouter` hook to get a reference to the current route. Then, you can use the `useEffect` hook to detect when the route changes and display a loading indicator. For example, you can use a simple `div` element with a loading animation to indicate that the app is still loading.
See what others are reading: Nextjs Loading Component
Next.js Router Events
Next.js Router Events are a powerful tool for tracking route changes in your application. They allow you to perform certain actions based on different events happening in the Next.js Router.
Worth a look: Next Js App Router Example
The Router object provides properties that we can use to listen to different events, such as routeChangeStart, routeChangeComplete, and routeChangeError. These events can be used to trigger actions like logging to the console or updating a loading state variable.
There are four main Router events: routeChangeStart, routeChangeComplete, routeChangeError, and beforeHistoryChange. Each event has a specific purpose and can be used to perform different actions.
Here are the four Router events and their purposes:
- routeChangeStart: fires when a route starts to change
- routeChangeComplete: fires when a route change is completed
- routeChangeError: fires when an error occurs while changing routes, or when a route load is canceled
- beforeHistoryChange: fires before changing the Router’s route history
To use Router events in your Next.js application, you'll need to import the useRouter hook and use the useEffect hook to listen to the events. You can then use the events to update a loading state variable or perform other actions.
For example, you can use the routeChangeStart event to set the isLoading state variable to true, and the routeChangeComplete event to set it to false. This will allow you to display a loading indicator while the route is changing.
By using Router events, you can create a seamless and user-friendly experience in your Next.js application.
Consider reading: Next Js App Router Project Structure
Adding Animation and Progress
Adding animation and progress to your Next.js app's route change can enhance the user experience.
You can use custom CSS, but for a more engaging experience, consider using libraries like NProgress or React Spinners.
NProgress is a lightweight library that displays a realistic trickle animation at the top of the viewport to indicate loading progress.
To use NProgress, import the NProgress function inside the _app.js component and call its start() and done() methods inside the routeChangeStart and routeChangeComplete event callbacks, respectively.
The NProgress function has a set of methods to display and configure the progress bar animation. Here are some of the available methods:
- start: shows the progress bar
- set: sets a percentage
- inc: increments by a little
- done: completes the progress
- configure: configures preference
To add the NProgress-associated CSS to your project, import the Head component from Next.js and nest the CDN link within the declaration.
By using NProgress, you can display a progress bar and a spinning icon at the top of the viewport.
Integrate with Router
You can integrate your loading indicator with the Next.js router using its built-in events. The router events are event listeners that allow you to track route changes in Next.js via the Router object.
Expand your knowledge: Nextjs Page Transition App Router Tailwind
To bind the events, you can use the useRouter hook from next/router. This hook provides access to the router object, which you can use to listen to different events happening in the Next.js Router.
The router events you can listen to are routeChangeStart, routeChangeComplete, and routeChangeError. These events fire when a route starts to change, has changed, and when an error occurs while changing routes or when a route load is canceled.
Here are the events and their corresponding actions:
- routeChangeStart: fires when a route starts to change
- routeChangeComplete: fires when a route change is completed
- routeChangeError: fires when an error occurs while changing routes, or when a route load is canceled
By binding these events, you can automatically start and stop the loading indicator when the user navigates between routes. This provides a seamless user experience and keeps the user informed about the loading process.
To ensure the event handler binds outside of components, you can create a unique component to keep the code tidy. This also helps to avoid binding multiple handlers.
Sources
- https://www.skillthrive.com/posts/next-js-route-progress-bar
- https://meje.dev/blog/building-a-nextjs-preloader-the-right-way
- https://blog.logrocket.com/how-to-build-a-progress-bar-indicator-in-next-js/
- https://medium.com/@shrsthprios/adding-route-events-in-next-13-for-loading-indicator-during-page-switch-6e4b479886c6
- https://blog.openreplay.com/how-to-build-a-progress-bar-for-nextjs/
Featured Images: pexels.com