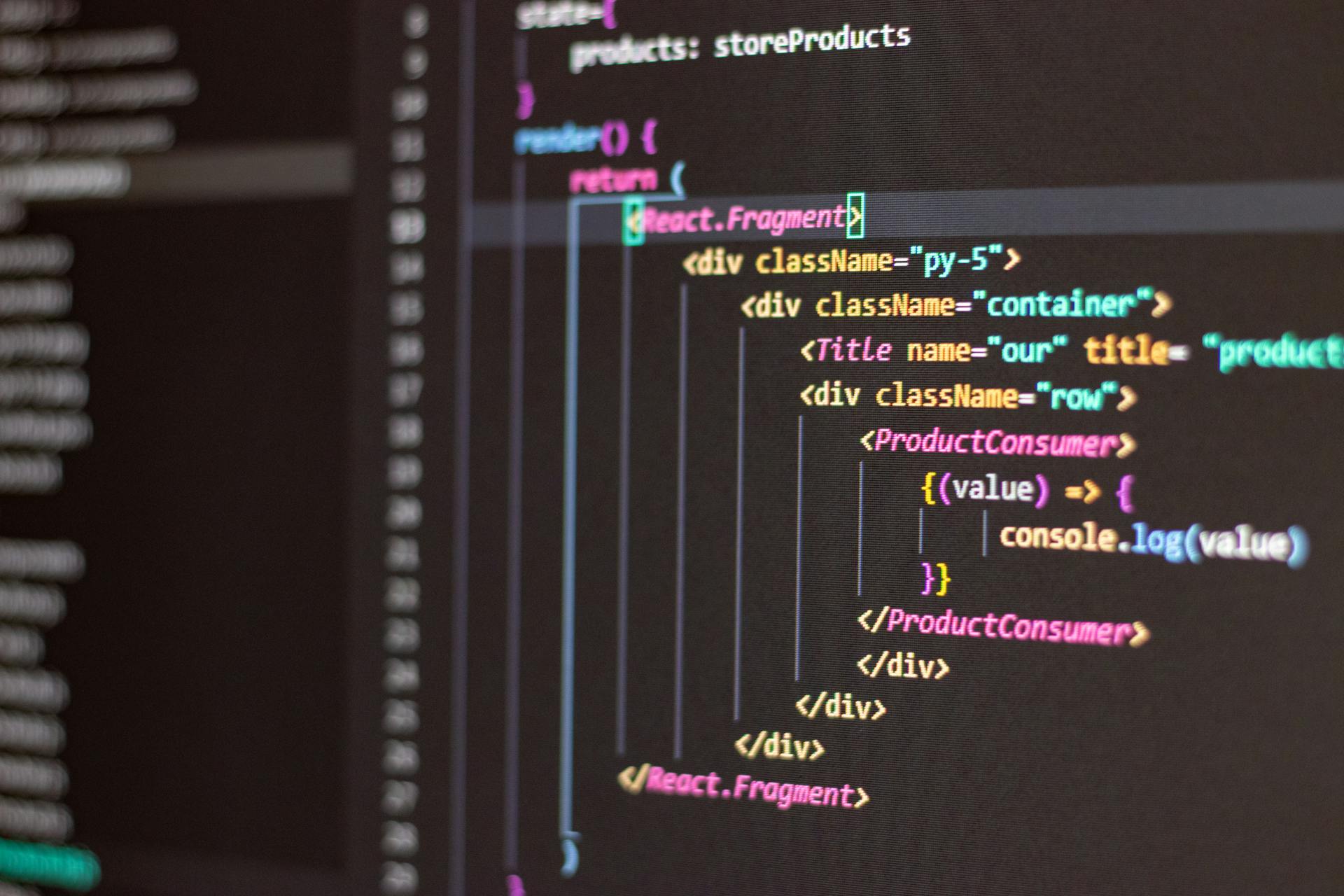
The Next Js App Router is a powerful tool for building fast and scalable applications. It's a built-in feature of Next Js that allows you to create a custom router for your app.
To get started with the Next Js App Router, you'll need to create a new file called `[routes].js` in your project's root directory. This file will contain your router configuration.
The Next Js App Router uses a file-system based routing system, which means you can create routes using files and directories. For example, if you create a file called `hello.js` in the `pages` directory, it will automatically be registered as a route.
You might like: Next Js vs Create React App
How to Set Up
To set up a Next.js app router, you'll need to follow the same installation process as mentioned in the Pages Directory section, but opt for "Yes" when prompted to use the App Router during setup. This creates an app directory.
The App Router installation and setup is a crucial step in getting started with routing in Next.js. You can create a route by creating a folder in the app directory and calling it whatever you want the route to be.
To create a route, nest a page.js file within the appropriate folder to define your route. This is similar to creating a route in the Pages Directory, but with the App Router, you'll be working within the app directory.
Creating a route with the App Router involves using folders within the app directory, which is a bit different from the Pages Directory approach. However, the core idea remains the same: create a folder and nest a page.js file to define your route.
By following these steps, you'll be able to set up a Next.js app router and start creating routes for your application. It's a straightforward process that will get you up and running quickly.
Explore further: Pages Router Next Js
Routing Fundamentals
Routing is a critical aspect of web apps that enables users to move between various pages.
To create a route, you can use either the Pages Directory or the App Router. The Pages Directory involves creating a folder in the pages folder and nesting an index.js file within it.
Suggestion: Next Js Pages
The App Router also uses folders, but within the app directory, and requires a page.js file to define the route.
Dynamic routes are created when you don't know the route segment name and want to use dynamic data, such as an ID or a slug.
A slug is a unique part of a URL that provides information about a web page, and should be simple and human-readable for SEO purposes.
To create a dynamic route segment, you can wrap the directory name in square brackets, like [name] or [slug].
A different take: Routing Next Js
Route Configuration
Route Configuration is a crucial aspect of Next.js App Router, and it's surprisingly easy to set up. You can create routes using the Pages Directory or the App Router, both of which involve creating folders and files in the right places.
To create a route with the Pages Directory, simply create a folder in the pages folder and call it whatever you want the route to be, like "about". Then, nest an index.js file in the about folder and fill it in with whatever content you want.
Related reading: Next Js App
For the App Router, you create folders within the app directory and nest a page.js file within the appropriate folder to define your route. This approach still uses the folder directory for routing, but with a slightly different convention.
Here's a summary of the two approaches:
This table highlights the main differences between the two approaches. Remember, the key to successful route configuration is to keep your folder structure organized and your file names clear.
Migrate Routing Hooks
Migrating routing hooks is a crucial step in the process of configuring routes in your Next.js application. You need to replace your useRouter hook with the equivalent hooks provided by next/navigation.
To do this, you'll want to follow the official guide on Migrating Routing Hooks. This process involves some legwork, but it allows your code to function with both routers. You'll remain in the /pages directory, but you'll be gradually moving closer to compatibility with the new router.
A fresh viewpoint: Next Js Hooks
One key thing to note is that you won't need to make your pages compatible with the App Router if you haven't used getStaticProps or getServerSideProps in your app.
You'll need to address a few missing pieces before you can test your app in the App Router end-to-end. This includes updating all links to point to the App Router and ensuring that page pathname logic works correctly under the /new/* slugs.
To solve this, you can add a rule in next.config.js.
More Routes
To create more routes in a Next.js application, you can use the Pages Directory or the App Router. With the Pages Directory, you can create a new route by creating a folder in the pages folder and naming it after the route you want to create. For example, to create a /blog route, you would create a blog/ directory under the pages folder.
You can also use the App Router to create routes. To do this, create a directory under the app/ directory and name it after the route you want to create. Then, create a page.js file inside the directory and add your route content.
Suggestion: Next Js Blog
To make a route accessible, you need to create a page.js file under each of the directories. Here's an example of how to create a nested route like /about/form:
- Create an about/ directory under the app/ directory.
- Create a form/ directory under the about/ directory.
- Create a page.js file under the form/ directory.
By following these steps, you can create more routes in your Next.js application and organize them in a way that makes sense for your project.
Here's a summary of how to create more routes:
Remember to create a page.js file under each directory to make the route accessible.
Dynamic Routes
Dynamic routes are a powerful feature in Next.js that allow you to create routes with dynamic data. You can create dynamic routes by wrapping the directory name in square brackets, like [name] or [slug].
To create a dynamic route segment, you can use the following format: /blog/[slug]/page.js. Here, [slug] is the dynamic route segment that will be passed as the params prop to the page.
You can also create a catch-all route segment by adding an ellipsis inside the brackets, like [...slug]. This will match routes like /store/book, /store/design, and /store/book/novels.
On a similar theme: Nextjs Dynamic
A catch-all route segment can be made optional by wrapping the parameter in double square brackets, like [[...name]]. This will serve the page from the app/store/[[...name]]/page.js, but the value of name will be undefined.
Dynamic routes can be used to create routes with dynamic data, like individual blog post routes. To do this, you need to create a directory named [slug] under the blog/ directory, and a page.js file under the blog/[slug]/ directory.
The page has received a params prop, and you can extract the slug value from it. You can use it to make a network call and get details to render on the page, or you can just render it on the page.
Dynamic routes can also be used to create optional catch-all segments. For example, you can create a page.js file inside the app/store/ directory to serve the page from the app/store/ directory.
However, if you don't want to create a page.js file inside the app/store/ directory, you can make the catch-all segment as an optional catch-all segment by wrapping the parameter in double square brackets, like [[...name]]. This will serve the page from the app/store/[[...name]]/page.js, but the value of name will be undefined.
Take a look at this: Next Js Dynamic Routing
Advanced Features
The App Router in Next.js has some amazing advanced features that take it to the next level. One of these features is server actions, which provide further utility.
Server actions allow you to handle server-side logic, making it easier to manage complex operations. This can be a game-changer for large-scale applications.
Data revalidation is another feature that's worth mentioning. It ensures that your application's data remains up-to-date and accurate.
Parallel routes enable you to handle multiple routes simultaneously, improving the overall performance of your application. This can be especially useful for applications with a high volume of traffic.
Route interception is a feature that allows you to intercept and modify routes as needed. This can be useful for tasks like authentication and authorization.
Expand your knowledge: Nested Routes in Next Js
Features and Tools
The App Router in Next.js is a powerful tool that offers a range of features beyond routing.
One notable feature is server actions, which provide further utility in the App Router.
App Router also supports data revalidation, ensuring that your application stays up-to-date with the latest data.
Check this out: Next Js Fetch Data save in Context and Next Route
Built-in SEO
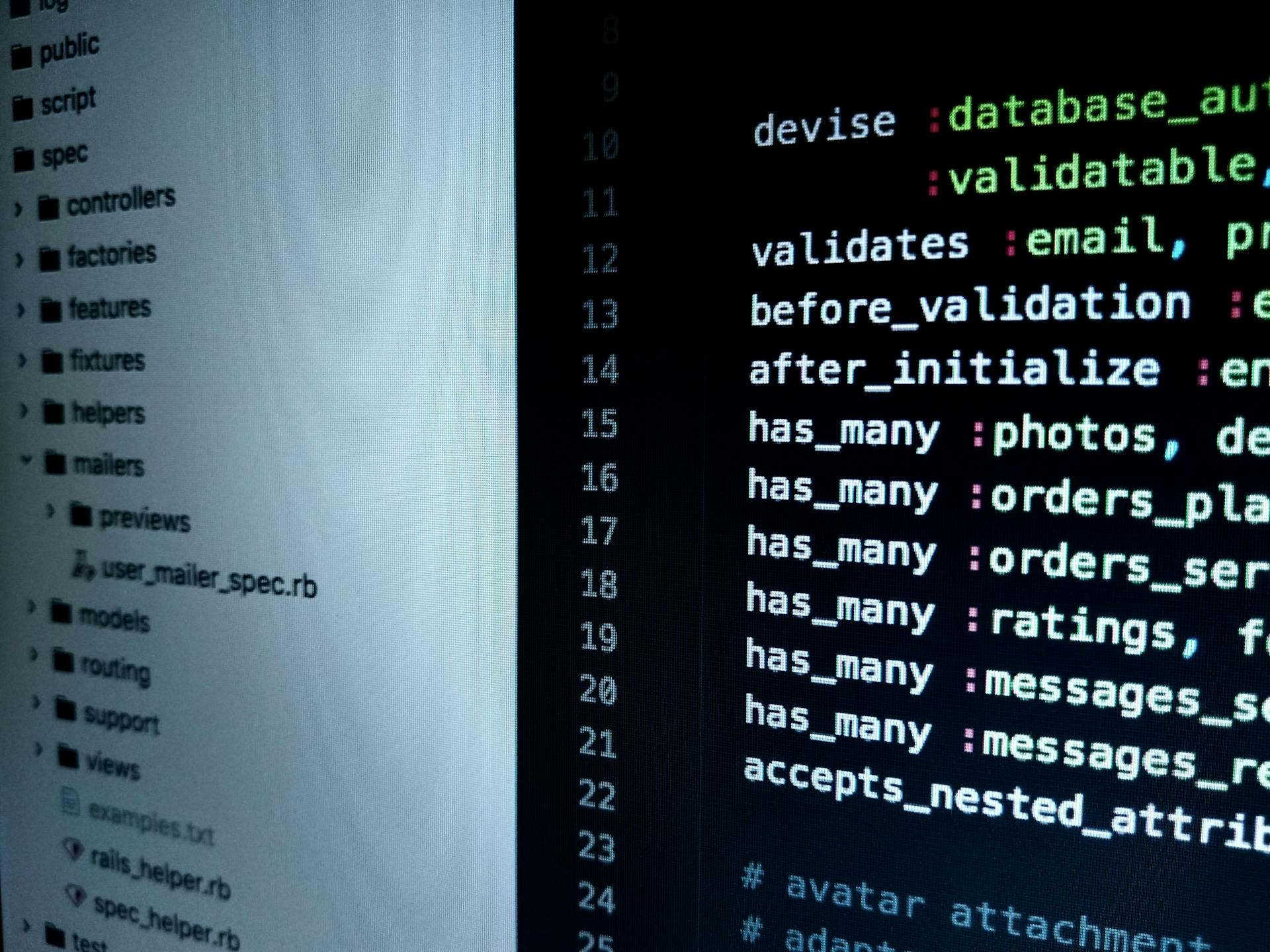
The Next.js App router comes with built-in SEO capabilities, making it a great choice for developers who want to optimize their websites for search engines.
This is achieved through the built-in Metadata API, which provides a range of SEO settings to enhance website visibility.
The Open Graph protocol is one such setting, allowing developers to customize the metadata that search engines display when sharing their website's content on social media.
By leveraging these built-in SEO features, developers can improve their website's search engine rankings and drive more traffic to their site.
Here's an interesting read: Next Js Seo
Data Fetching
Data Fetching is a powerful feature that allows async components to fetch data within them, reducing reliance on APIs like getServerSideProps.
To fetch data, mark a component as async and use the fetch function within. This is a game-changer for building data-driven applications.
The App Router caches the fetched data on the server, eliminating the need to re-fetch that data on every request, unless a revalidation parameter is passed in in the fetch function.
You can control how often the data is re-fetched by passing in a revalidation parameter, like in this example where the code causes a re-fetch of new data every 5 seconds.
Features
App Router in Next.js offers a range of features beyond routing.
One notable feature is server actions, which provide further utility to the App Router.
The App Router also boasts parallel routes, allowing for efficient handling of multiple routes simultaneously.
Data revalidation is another advanced feature, ensuring that data remains up-to-date and accurate.
Route interception is a useful feature, enabling developers to intercept and manipulate routes as needed.
These features demonstrate the App Router's capabilities and flexibility in the Next.js ecosystem.
On a similar theme: Intercepting Routes Next Js Example
Sources
- https://newrelic.com/blog/how-to-relic/how-to-monitor-app-based-router-nextjs-application
- https://workos.com/blog/migrating-to-next-js-app-router-with-zero-downtime
- https://www.freecodecamp.org/news/routing-in-nextjs/
- https://nextjs.org/docs/app/building-your-application/routing
- https://blog.greenroots.info/dynamic-routes-nextjs-app-router
Featured Images: pexels.com