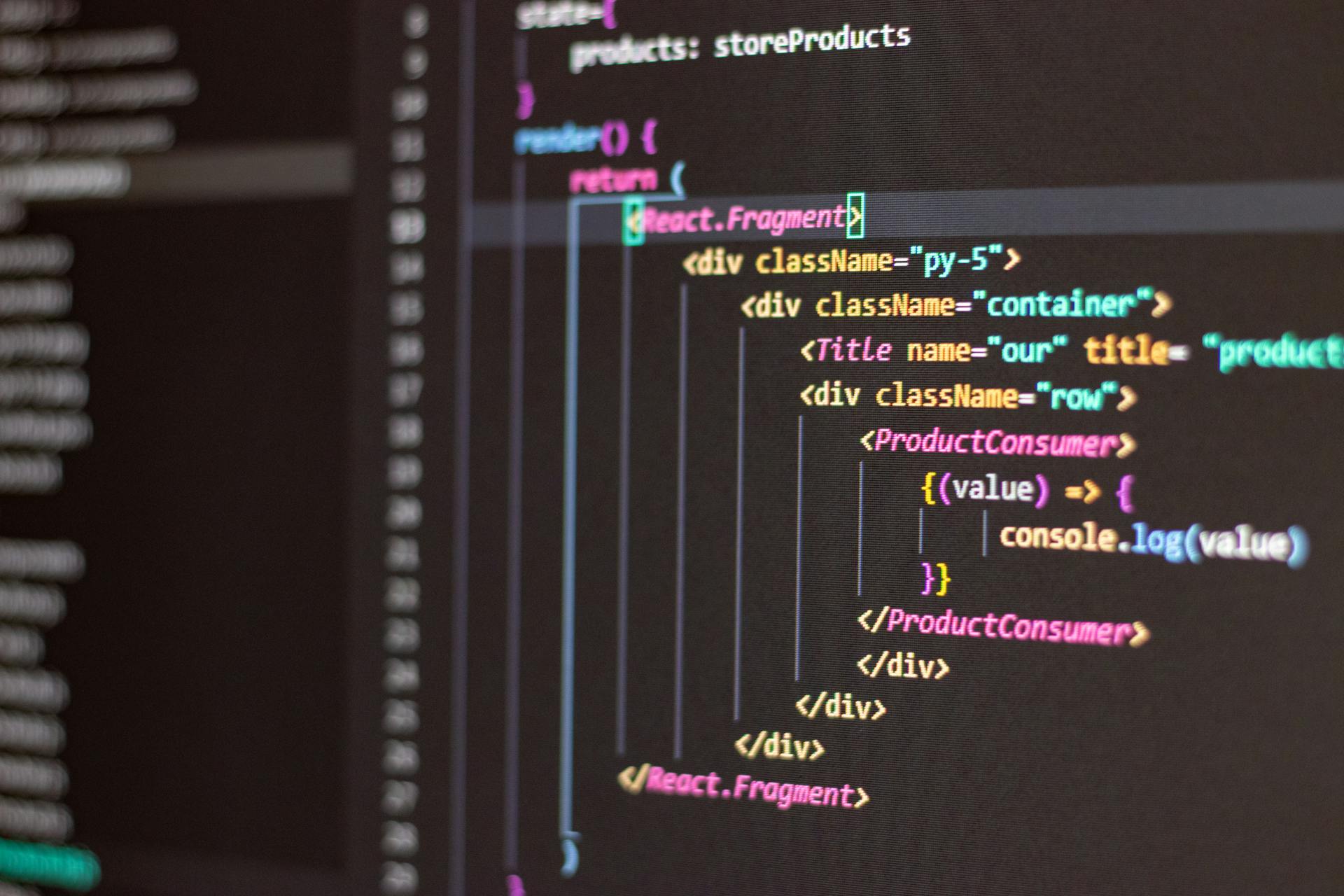
Setting up a Next.js blog is a straightforward process that requires a few key tools. You'll need to install Node.js and npm, which can be done in just a few clicks.
Next, you'll need to create a new project using the `create-next-app` command, which will set up a basic Next.js project for you. This command will also install all the necessary dependencies.
To get started with Next.js, you'll need to have a basic understanding of JavaScript and React. Don't worry if you're new to these technologies, as Next.js provides a lot of built-in functionality to help you get started quickly.
Next.js has a built-in API route system that allows you to create server-side API routes using the `pages/api` directory. This is a great way to create API endpoints that can be used by your frontend code.
Curious to learn more? Check out: New Nextjs Project Typescript
Project Setup
To set up a Next.js project, start by launching the setup wizard with `npx create-next-app`. You'll be prompted to hit `y` a few times to complete the wizard.
First, create a new Next.js project by running the setup wizard. This will get your project up and running quickly.
In our case, we'll have a home page, as well as pages for projects and about. To create these pages, you'll need to add them to your project manually.
If this caught your attention, see: Create Next Js Project
Creating the App
To create a Next.js app, you can either clone a copy from a GitHub repository or start from scratch by following the steps below.
First, open a terminal and point it to the directory where you want to create a new app. Run the command to create the app, which will bootstrap the application and install necessary dependencies.
You'll be prompted to answer some questions during the process. This will create a new directory with the name of your app as mdx-app and install all the required dependencies.
The development server will automatically start and open the app in your default browser. If not, you can access the application using the URL https://localhost:3000/.
The development server will reload the app whenever you make changes to the code, allowing you to see your changes in real-time on the browser via hotreloading.
Consider reading: Next Js Single Page Application
Project Setup
To set up your project, start by launching the Next.js setup wizard with npx create-next-app and follow the prompts to complete the wizard.
You'll need to create a home page, projects page, and about page. This will give you a solid foundation for your project.
Create a new directory called app/subpages/components and add a simple header component to it. This will help you organize your code and keep your layout consistent.
The new Metadata API has great documentation, so you can refer to it for more information on how to set up your project.
Worth a look: Next Js Cookie
Updating Our Home
Updating our home page is a crucial step in customizing our blog's design. We need to create a Hero slice that will display at the top of the homepage.
To do this, we'll click Add slices > Create new and create a slice called "Hero." This will give us a blank slate to add the necessary fields.
We'll add the following fields to the Non-Repeatable Zone of our Hero slice: Title, Description, and Image. The Title field will be a Rich Text field with an h1 tag only, the Description field will be a Rich Text field with only p, b, i, and link tags, and the Image field will be an Image.
Curious to learn more? Check out: Is Nextjs Backend
Once we've created our Hero slice, we can navigate to the /src/app/page.tsx file to update our home page. This file contains the necessary functions to render the content to the page.
We'll delete the existing RichText slice, as we won't need it for our Homepage. This will give us a clean start to work with.
Check this out: Nextjs Folder Structure
Creating Content
To create content for your Next.js blog, you'll need to start by defining the structure of your pages. This involves creating a "Blog Post" page type in Slice Machine, which will serve as the template for all your blog post pages.
You can add slices to your page type, including a RichText slice, which allows you to add content to your blog posts. The starter kit comes with a pre-existing RichText slice that you can add to your page type.
To add content to your blog post, you'll want to fill in the details for the UID, title, description, featured image, and publication date on the newly created page.
Discover more: Next Js App vs Pages
Here are the details you'll need to fill in:
- UID (pre-existing)
- Title: Rich Text field with only h1 tag
- Description: Rich Text field with only p, b, i, and link tags
- Featured Image: Image field
- Publication Date: Date field
Once you've filled in the details, you can add a RichText slice to your blog post by clicking "Add slice" from the table of slices on the left and selecting RichText. This will allow you to add content to your blog post.
You might like: How to Add Img to Nextjs
Writing and Rendering
You can write server components in Next.js to use static rendering, which means all HTML pages are pre-rendered at build time.
This approach allows you to keep your front-facing site separate from your backend, and query the content API only when you run the next build command.
To render markdown content, you can use next-mdx-remote, which supports experimental React Server Components. This package allows you to keep your markdown separate from your Next.js project.
Here are some common components you may want to use in your markdown files:
- next/link for client-side routing
- next/image for image optimization
- remark and rehype plugins for customizing your markdown
These components can be passed as props to your MDX pages, allowing you to customize the rendering of your content.
Rendering Markdown
Rendering Markdown can be a challenge, especially when working with Next.js. Official Next.js documentation provides a great guide for using MDX with all your pages.
Sometimes you need to render content from a remote source, like a CMS. This is where next-mdx-remote comes in, offering experimental React Server Components support.
To keep markdown separate from the Next.js project, you can use next-mdx-remote. This approach can be beneficial for niche-specific reasons, as it did for the author of the guide.
Fetching and rendering markdown requires the right tools, and next-mdx-remote can help you achieve this.
Broaden your view: Next Js Client Side Rendering
Rendering Your Posts
Rendering your posts can be a bit tricky, but it's a crucial part of making your content shine. You can use static rendering with Next.js to pre-render all your HTML pages at build time, which means the front-facing site won't send any requests to the backend.
To fetch and render markdown, you can use next-mdx-remote, which is an experimental React Server Components support. This allows you to keep your markdown separate from the Next.js project.
Take a look at this: Nextjs Server Rendering Tailwind
To render your posts, you'll need to setup MDX with remark and rehype plugins. This will give you the flexibility to use custom components in your markdown files. You can also use the official next/link and next/image components for client-side routing and image optimization.
Here are some key components you'll need to render a post:
You can optionally choose to build all of your posts at build time by adding `generateStaticParams` to the page. This will pre-render all your posts and make them available on your site.
By using static rendering, fetching and rendering markdown, and setting up MDX with custom components, you'll be well on your way to rendering your posts like a pro.
Tailwind Styling and Layout
Tailwind CSS is a powerful tool for styling your Next.js blog, and understanding its classes can make a big difference in how your blog looks. The flex direction of an element can be changed using the flex-col class.
Explore further: Nextjs Blog Template
To center content horizontally and vertically on a page, use the items-center and justify-between classes, which are equivalent to align-items: center and justify-content: space-between in plain CSS.
You can use the Tailwind CSS IntelliSense extension in Visual Studio Code to get autocompletion and syntax highlighting. This can save you a lot of time and effort when working with Tailwind.
To add space between items in a flex container, use the row-gap property, which can be achieved with the gap-y-8 class from Tailwind. This is more efficient than adding a margin to the wrapper, as it will not create unnecessary space on the first or last item.
The font-extrabold and text-xl classes are equivalent to font-weight: 800 and font-size: 1.25rem; line-height: 1.75rem; in plain CSS, respectively.
To change the color of an element on hover, you can use the group class on the parent element and the group-hover:text-blue-500 class on the child element. This is a great way to add interactivity to your blog without using child selectors in plain CSS.
Here are some common Tailwind classes and their equivalent CSS properties:
- items-center: align-items: center
- justify-between: justify-content: space-between
- flex-col: flex-direction: column
- gap-y-8: row-gap: 2rem
- font-extrabold: font-weight: 800
- text-xl: font-size: 1.25rem; line-height: 1.75rem;
- group-hover:text-blue-500: hover { color: blue; }
Dynamic Routing and RSS
Dynamic segments, like the [slug] in the URL /blog/[slug], can be used to create unique pages for your blog posts.
For example, the footer can live inside the /blog/[slug] layout to link to other posts. This is because dynamic segments can be used to create their own layouts, just like the /blog/[slug] page.
This approach can be applied to your own project by following the code snippets provided.
Consider reading: Next Js Dynamic
Dynamic Routing
Dynamic Routing is a powerful feature in Next.js that allows us to create pages with dynamic segments, like /blog/[slug]. This means we can create a page that displays a single blog post, and it will have its own footer to link to other posts.
To create a dynamic route, we need to create a new file at a specific location, like src/app/articles/[slug]/page.tsx. The square brackets on the folder name create a dynamic route, and our component will receive a prop called slug, which contains the dynamic part of the route.
For your interest: Next Js Slug
In development mode, every route will work, but at build time, Next.js will not know which routes should be created, and therefore none of these pages will be generated. To fix this, we need to create a function for fetching the content of a single blog post, based on the slug.
We can use a feature of Next.js called Dynamic Routes to create pages with dynamic segments. For example, we can create a page at /blog/[uid] that displays a single blog post and its content.
To generate a new page in Next.js for each blog post in Prismic, we need to use the generateStaticParams function. This function queries Prismic for all of the pages that use our custom page type blog_post and returns an array of all of the pages UID's.
With the generateStaticParams function, we can automatically generate new pages in Next.js whenever we create new pages in Prismic. This is useful for creating a dynamic blog that can display multiple posts.
On a similar theme: Use Client Nextjs
Creating an RSS Feed
Creating an RSS Feed is a custom solution that works well for the author, who uses the marked library to parse markdown files and the rss library to generate the feed.
The JSX components for MDX are passed through to the RSS feed, making it easy to ensure the components are legible even when not rendered.
To generate an RSS feed, the author uses a combination of the marked and rss libraries, which provides a reliable and efficient solution.
This approach allows the author to create a custom RSS feed that meets their specific needs, without relying on an official solution.
Explore further: Next Js Custom Server
Frequently Asked Questions
Is Next.js good for a blog?
Yes, Next.js is a great choice for blogs due to its support for static site generation and server-side rendering, making it easy for search engines to crawl and index articles. This ensures better visibility and accessibility for your blog.
Is Next.js gaining popularity?
Yes, Next.js has seen a significant surge in popularity, jumping from the 11th to the 6th most popular framework among web developers in just a few years, according to the Stack Overflow survey of 2023. Its rapid growth indicates increasing acceptance and effectiveness in the developer community.
Will Next.js replace React?
Next.js is designed to complement React, not replace it, by adding features like server-side rendering and routing. It enhances React's capabilities for specific project types, but React remains a core component.
Sources
- https://www.contentful.com/blog/build-blog-next-js-tailwind-css-contentful/
- https://mattermost.com/blog/create-a-next-js-blog-on-vercel-using-mdx-and-tailwindcss/
- https://maxleiter.com/blog/build-a-blog-with-nextjs-13
- https://leonardqmarcq.com/posts/how-to-build-your-own-blog-with-nextjs
- https://prismic.io/blog/nextjs-blog-tutorial
Featured Images: pexels.com