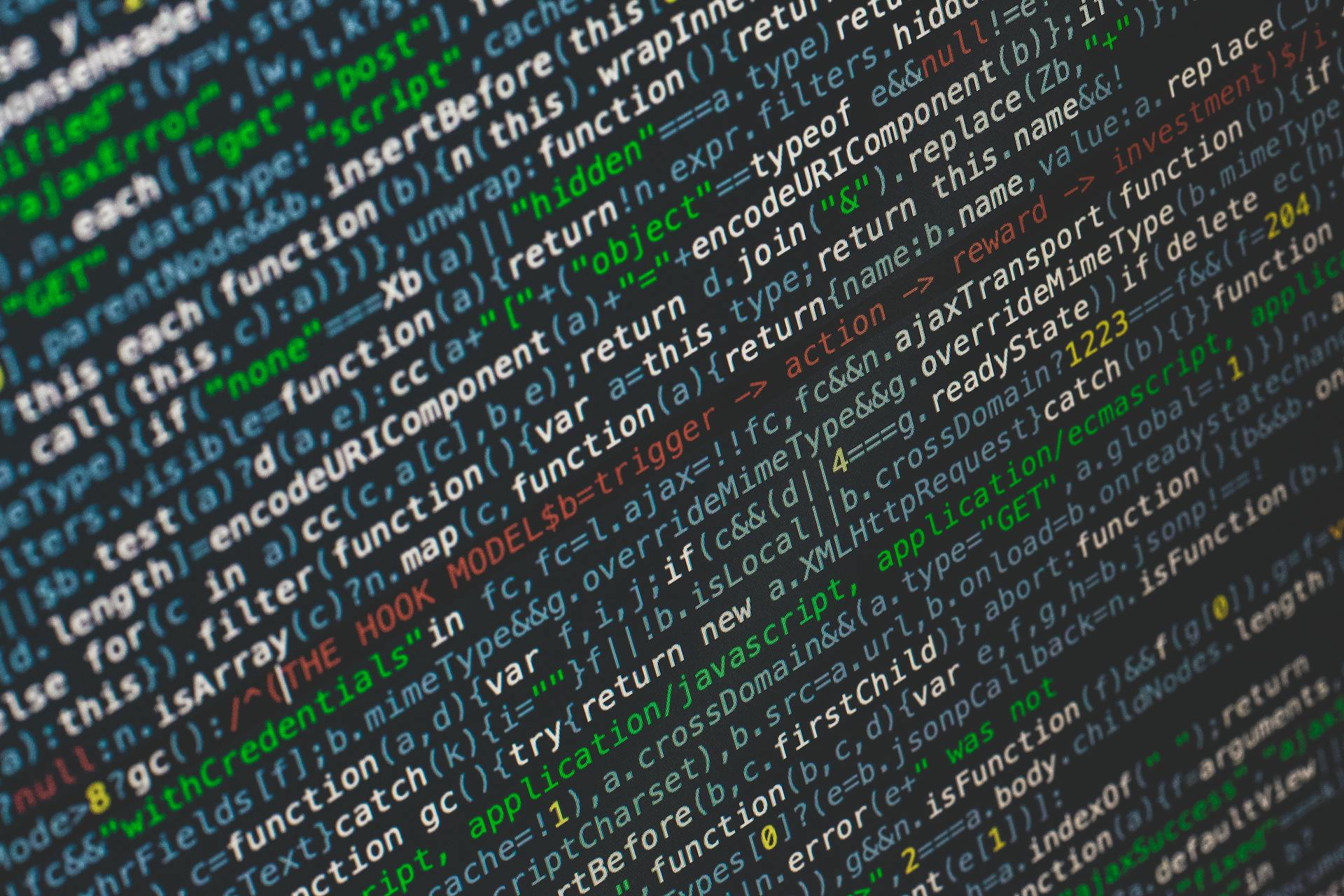
Proxy API routes in Next.js are a powerful way to handle external API requests, allowing you to abstract away the underlying implementation and focus on building your application. This approach also helps with security and caching.
By using a proxy API route, you can easily switch between different API providers without modifying your code, as demonstrated in the example where the same code can be used with both the Vercel and Netlify APIs.
Proxy API routes in Next.js are configured using the `next.config.js` file, where you can specify the target URL and other settings. This configuration allows you to customize the behavior of your proxy API routes.
In a real-world scenario, using proxy API routes can help improve the performance and reliability of your application by reducing the number of external requests.
A fresh viewpoint: Intercepting Routes Next Js
Setting Up
To set up Next.js for API testing, you'll need to use Jest as your testing framework, since you're only testing API calls and not components being rendered in the DOM.
You'll still need to do some extra configuration to get started.
For a TypeScript file, import the NextApiRequest and NextApiResponse types from next, just like you would for a real API route file.
Jest is the only testing framework you'll need for this task.
Check this out: Django Rest Framework Pagination
Testing Routes
Testing routes is a crucial part of ensuring our Next.js application is reliable and maintainable. We can use Jest to write unit tests for our API routes, but we'll also need to import the NextApiRequest and NextApiResponse types from next.
To write unit tests for our API routes, we can use a testing framework like Jest. This will allow us to verify that individual parts of our application work as expected.
Additional reading: Web Application Programming Interface
Testing Routes
Testing routes is a crucial step in ensuring the reliability and maintainability of our application. Unit testing is essential for verifying that individual parts of our application work as expected.
For API routes, unit testing means testing the route handlers to ensure they return the correct responses for given inputs. We can use testing frameworks like Jest to write these unit tests. Jest is the only testing framework we'll need for API calls.
To get started, we'll need to import the NextApiRequest and NextApiResponse types from next. This is because we're working with a TypeScript file. Now we can add unit tests to all other API routes as needed.
Unit testing API routes involves creating mock request and response objects to test the route handlers. We can use node-mocks-http to create these mock objects. With these mock objects, we can assert that a correct response status and body have been sent.
Here's an interesting read: Next Js Spa
What Are Routes?
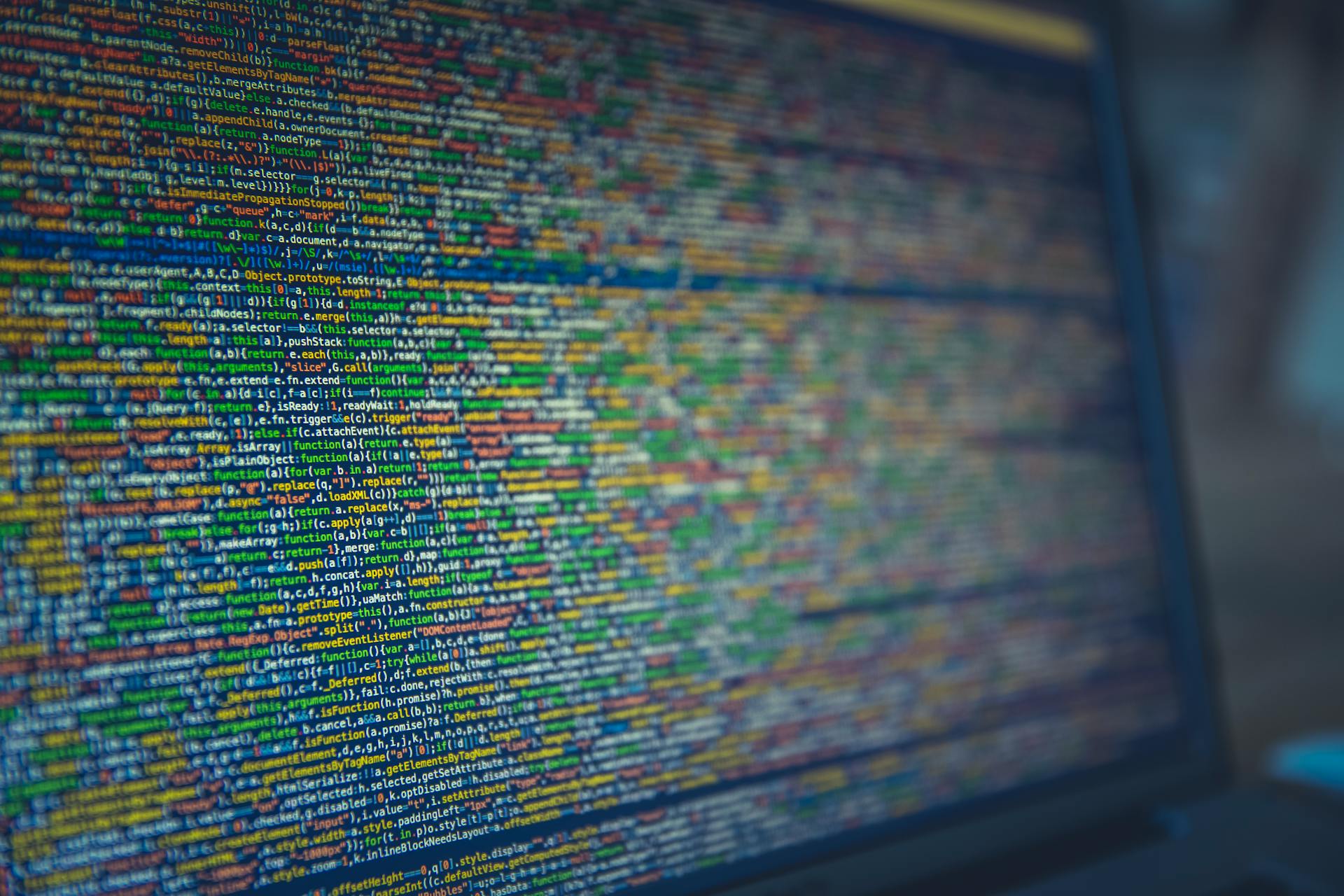
Routes are essentially a way for different applications to communicate with each other using HTTP requests and responses. This communication involves exchanging data between applications.
A client-side web application can send a request to a server application, asking for resources or data stored in a database. For example, a GET request can be sent to retrieve a list of users.
To build an application that requires data and resources from an external source, you'll need an API to request those resources. This is typically done by creating two separate applications: a client-side application that runs in the browser and a server-side application that runs on the server.
There are different methods of creating an API, including REST, SOAP, and GraphQL. In the context of Next.js, API Routes eliminate the need for creating an additional backend server in your full-stack web applications.
Here's an interesting read: Next Js Debug
Middleware in Routes
Middleware in routes is a crucial aspect of API development. It allows us to perform tasks such as authentication, logging, and request validation before the request reaches our route handlers.
Middleware functions can be used to pre-process requests, making our routes more secure and maintainable. This is especially important when working with sensitive data.
In Next.js, middleware functions can be applied to API routes using the following steps: making a middleware function and applying it to an API route. For example, the authenticate middleware verifies whether a valid token is included in the request headers.
Here's a simple way to think about it: middleware functions act as a filter, allowing or denying access to our route handlers based on certain conditions. In the case of the authenticate middleware, it checks for a valid token and returns a 401 Unauthorized response if it's not present.
Here's a breakdown of the steps to apply middleware to an API route:
- Make a middleware function:
- Apply middleware to an API route
Server Actions
Server Actions are asynchronous JavaScript functions that run on the server in response to user interactions on the client. They're made possible by Next.js and can be triggered by a user action or business logic condition, just like calling an async function.
Next.js will serialize the request parameters and send them to the server, where the server will deserialize the request parameters and execute a function that represents the Server Action. This process is similar to making a request to an API endpoint.
Server Actions can be decoupled from components and actions, making the code easier to understand, maintain, reuse, and test. This is an important best practice to keep in mind when working with Server Actions.
Some other best practices for using Server Actions include caching the results of Server Actions to improve performance, handling errors gracefully, and protecting your Server Actions from unauthorized access.
Here are some key points to keep in mind when working with Server Actions:
- Decouple Server Actions from components and actions
- Cache the results of Server Actions
- Handle errors gracefully
- Protect your Server Actions
Server Actions can be used to send data to an external API, such as a third-party API. To do this, you'll create a Server Action that will trigger from a form submission, and then serialize the request parameters and send them to the server.
Fetch Gateway
The gateway device info is crucial for the app to function properly, and it's obtained by making a GET request to the Notehub project.
The required parameters for the request include an authorization token, a Notehub project's ID, and a gateway device's ID, which can be passed as query parameters in the URL.
The URL request to the Notehub project is constructed by combining the endpoint with the required headers, and Axios is used to make the call.
The JSON payload returned from Notehub is then read to check for any errors, such as a missing gateway ID.
See what others are reading: Next Js Url Params
Best Practices
To create a secure Next.js proxy API route, follow these best practices:
Decouple Server Actions from your components to keep code easier to understand, maintain, reuse, and test. This separation of concerns is crucial for clean code.
Cache the results of Server Actions to improve application performance by avoiding unnecessary data fetching. If the data doesn't frequently change, you can store the results so they don't need to be fetched every time.
Readers also liked: Nextjs Server Actions File Upload
Use HTTPS to encrypt data sent from the client to the server, protecting against eavesdropping and tampering. Ensure your production environment runs using HTTPS.
Implement rate limiting to protect your API from abuse by restricting the number of requests within a certain period. You can use middleware like express-rate-limit to achieve this.
Validate input data to prevent injection attacks by ensuring it arrives at the server in the expected format and type. Use libraries like joi or yup to validate request data.
Here's a summary of security measures to implement:
Sources
- https://www.paigeniedringhaus.com/blog/how-to-unit-test-next-js-api-routes-with-typescript
- https://nextjs.org/docs/pages/api-reference/next-config-js/rewrites
- https://authjs.dev/getting-started/migrating-to-v5
- https://auth0.com/blog/using-nextjs-server-actions-to-call-external-apis/
- https://refine.dev/blog/next-js-api-routes/
Featured Images: pexels.com