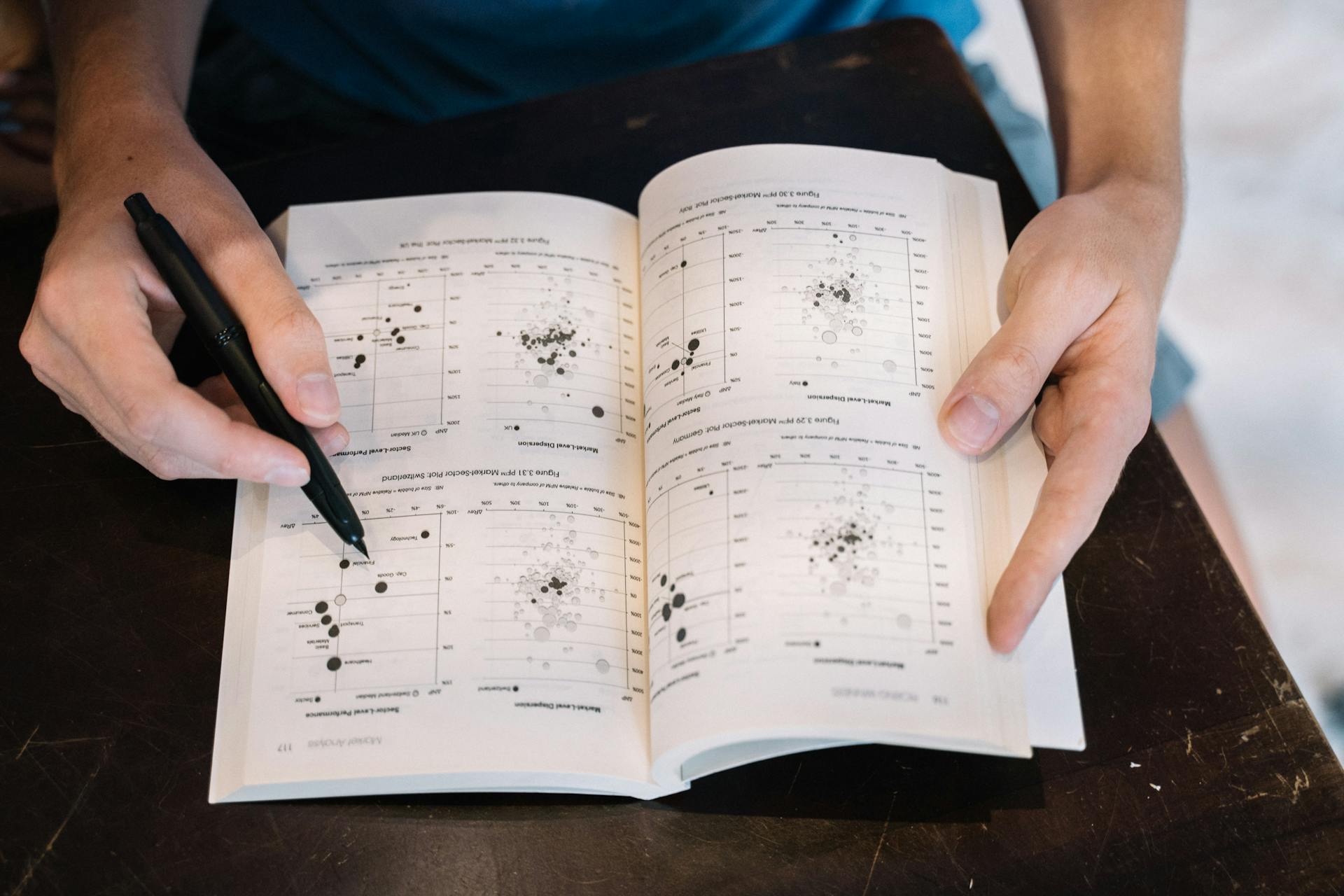
Django Rest Framework Pagination is a must-have for any large-scale API.
The Django Rest Framework (DRF) provides a built-in pagination system that allows you to limit the number of items returned in a response.
By default, DRF uses a cursor-based pagination system.
This means that the API will return a URL that points to the next page of results, which can be used to fetch the next set of items.
What is Django REST Framework Pagination?
Django REST Framework pagination allows you to split a large result set into individual pages with some simple settings.
Pagination refers to the process of splitting a large set of data into smaller, manageable chunks or pages.
Imagine loading an entire social media feed at once — it would take ages!
The pagination feature allows you to utilize the server resources more efficiently, improve the loading times, and enhance user experiences.
For APIs, especially those handling vast amounts of data, pagination ensures that clients don’t receive overwhelming amounts of information all at once, which can lead to sluggish performance or even crashes.
Django REST Framework pagination is used in the Todo API project to test out the pagination with some todos added to the project.
Implementing Pagination
Implementing pagination in Django REST Framework is a straightforward process. You can use the built-in pagination classes, such as PageNumberPagination, to paginate your data.
To get started, you can define the pagination method in your settings and apply it to your views or viewsets. The PageNumberPagination class is a simple setup that paginates the user data and provides links to the next and previous pages.
The LimitOffsetPagination style is another option, where the client includes both a "limit" and an "offset" query parameter. The limit indicates the maximum number of items to return, while the offset indicates the starting position of the query. You can configure this style globally by setting the DEFAULT_PAGINATION_CLASS in your settings.
Here's a summary of the LimitOffsetPagination class attributes:
- default_limit: The default number of items to be returned on a single page. Defaults to None.
- max_limit: The maximum number of items to be returned in a single page. Defaults to None.
- limit_query_param: The name of the query parameter used to specify the limit. Defaults to 'limit'.
- offset_query_param: The name of the query parameter used to specify the offset. Defaults to 'offset'.
You can use this class in your views or viewsets by setting the pagination_class attribute to LimitOffsetPagination.
How Django Handles
Django REST Framework comes with built-in support for pagination, which is a game-changer for handling large datasets.
This built-in support splits the data into pages, returning only a subset at a time, which minimizes the load on your server and prevents overwhelming the user.
You can implement pagination by defining the pagination method in your settings and applying it to your views or viewsets.
For example, using PageNumberPagination is a simple setup that paginates the user data and provides links to the next and previous pages.
This approach ensures a smooth user experience and is a must-have for any API endpoint that returns a large dataset.
Implementing Basic
Implementing Basic Pagination in Django REST Framework is straightforward. You define the pagination method in your settings and apply it to your views or viewsets.
To get started, you can use PageNumberPagination. This simple setup paginates the user data and provides links to the next and previous pages.
With PageNumberPagination, you can easily break down large result sets into individual pages, improving user experiences and loading times.
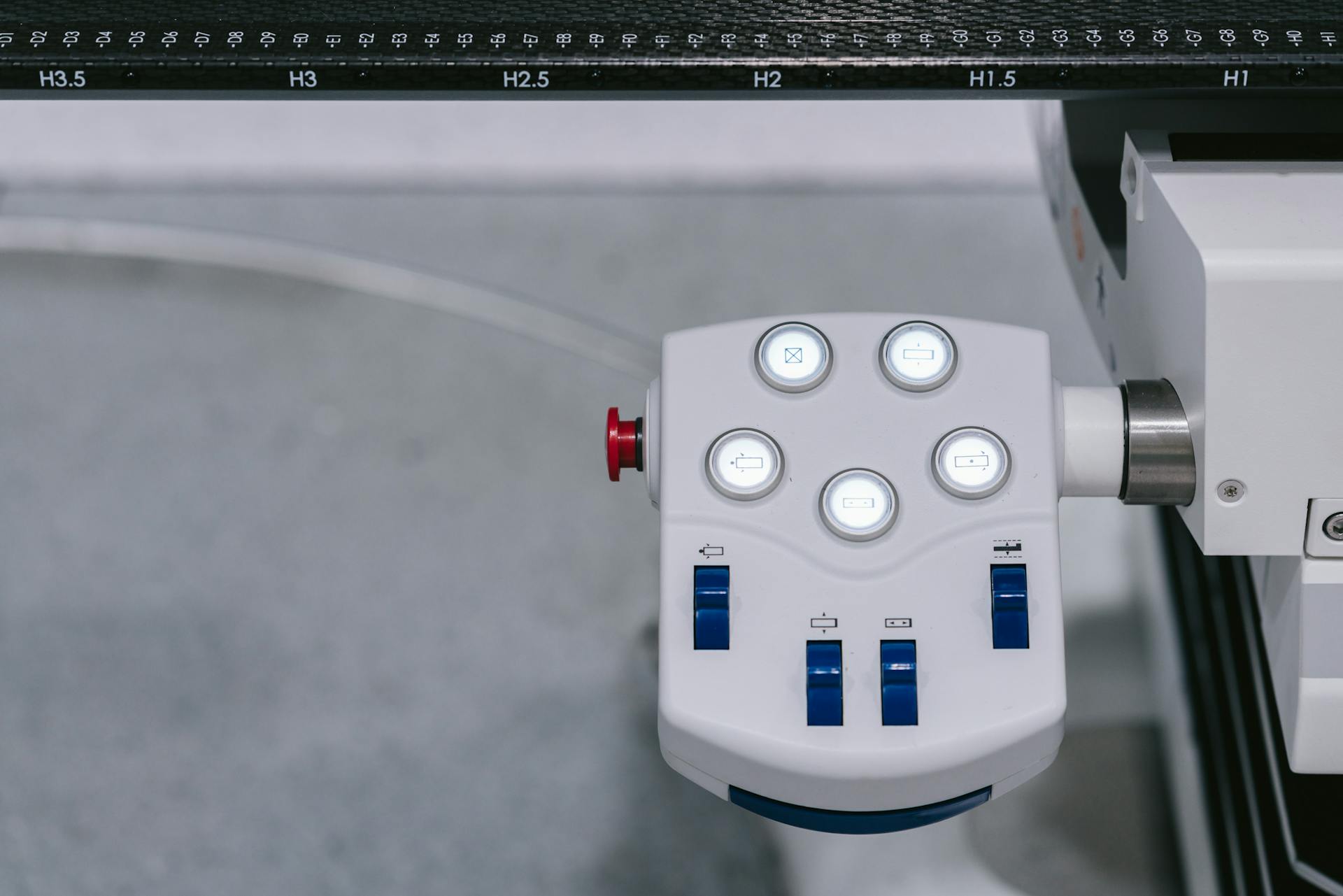
Here are the basic settings for PageNumberPagination:
- PAGE_SIZE: This setting determines the number of items to return per page. By default, it's set to 10, but you can adjust it to suit your needs.
- DEFAULT_PAGINATION_CLASS: This setting specifies the pagination class to use. In this case, it's set to PageNumberPagination.
By using PageNumberPagination, you can efficiently manage large datasets and provide a better experience for your users.
Here's an example of how to configure PageNumberPagination:
REST_FRAMEWORK = {
'DEFAULT_PAGINATION_CLASS': 'rest_framework.pagination.PageNumberPagination',
'PAGE_SIZE': 10,
}
Limit Offset
Limit Offset is a pagination style that allows clients to specify the maximum number of items to return and where to start. It's a flexible approach that's useful when you need more control over the data retrieval process.
The LimitOffsetPagination class in Django Rest Framework (DRF) is a built-in pagination class that enables this style. You can configure it globally by setting rest_framework.pagination.LimitOffsetPagination as the DEFAULT_PAGINATION_CLASS in your settings.py file.
If you want to customize the pagination style, you can override the attributes of the LimitOffsetPagination class. Some of the key attributes include default_limit, limit_query_param, offset_query_param, max_limit, and template.
Here's a summary of the key attributes and their default values:
To make a GET request using the LimitOffsetPagination style, you can use the following HTTPie command: http GET ":8000/robot/?limit=2&offset=2". You can adjust the limit and offset values to suit your needs.
Customizing Pagination
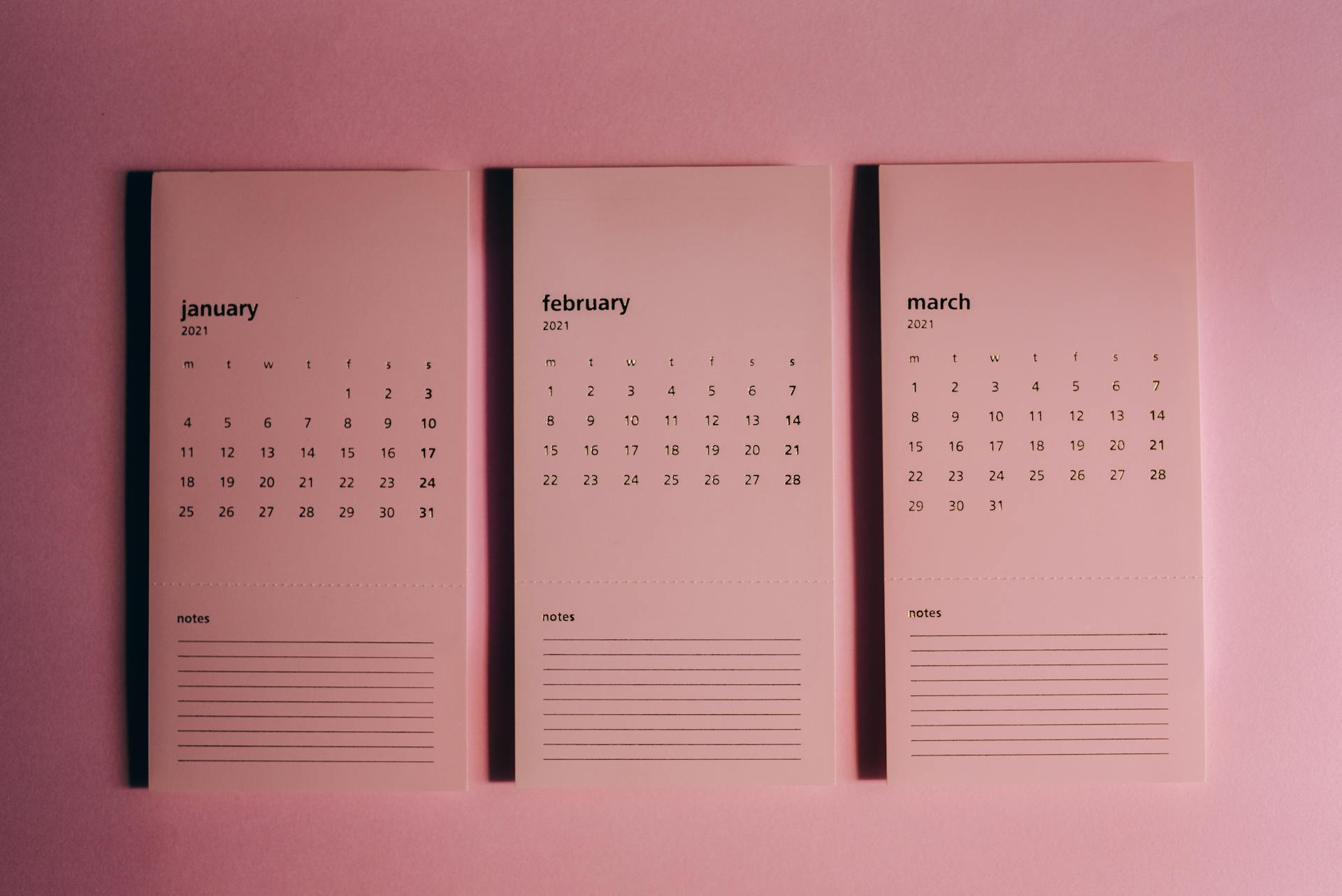
Customizing pagination in Django Rest Framework (DRF) can be done in various ways to suit your application's needs. You can adjust the page size dynamically based on user needs, or implement conditional pagination for specific endpoints.
To customize the page size, you can use a query parameter, such as /api/users?page_size=50. This allows clients to request different page sizes according to their requirements.
You can also create custom pagination classes by subclassing the built-in paginators. For example, you can create a paginator that only returns even-numbered pages, which can be useful for business-specific rules.
Here are some ways to customize pagination in DRF:
- Dynamic page size: Adjust the page size based on user needs using a query parameter.
- Conditional pagination: Implement pagination for specific endpoints.
- Custom pagination classes: Create custom pagination classes by subclassing the built-in paginators.
- Custom pagination styles: Define your logic for paginating querysets and formatting the paginated response.
By using these customization options, you can optimize pagination in your DRF application to improve performance and user experience.
Customizing for Performance
Customizing pagination can be done to optimize performance. You can adjust the page size dynamically based on user needs.
This allows clients to request different page sizes using a query parameter, such as /api/users?page_size=50.
Customizing the Controls
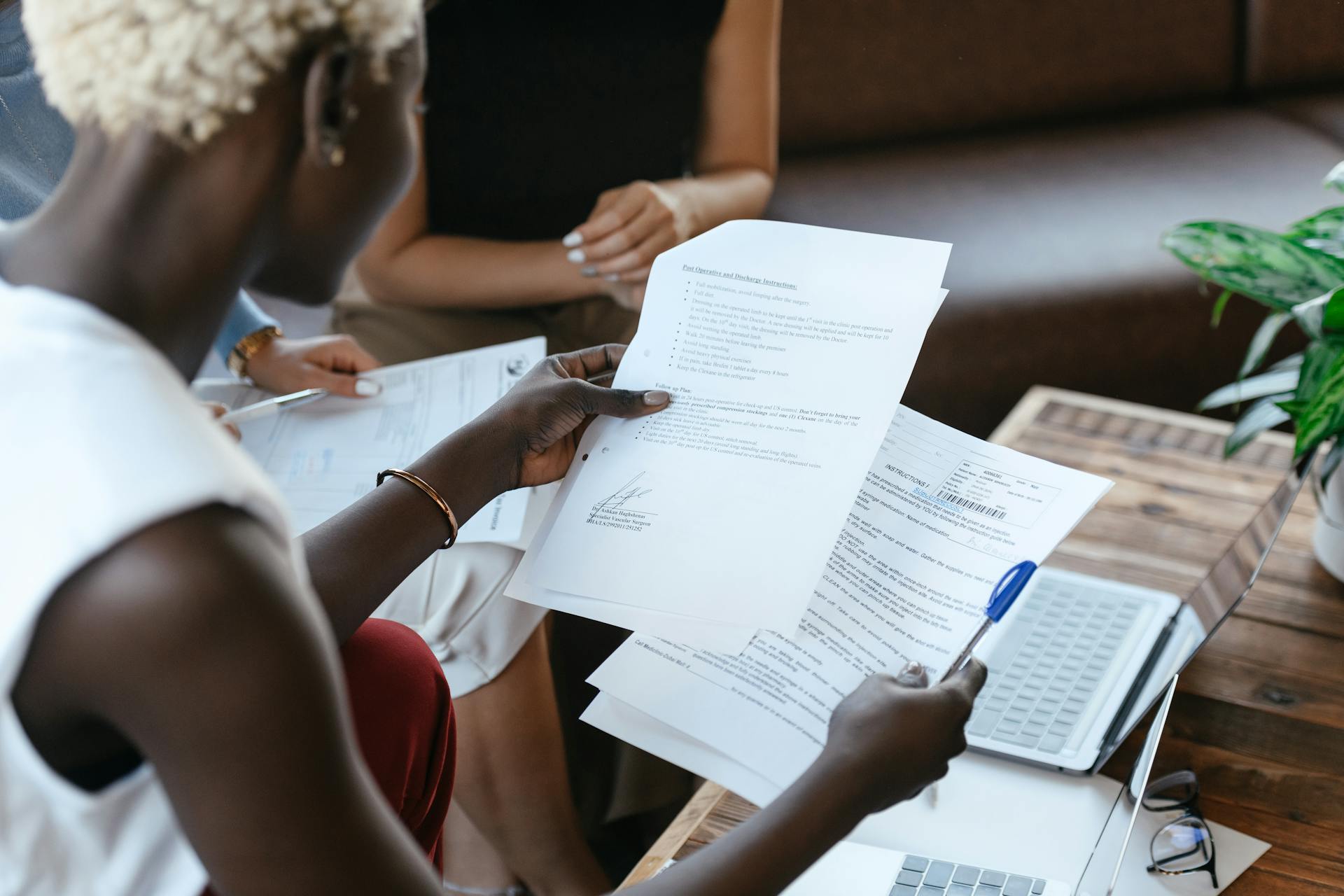
You can customize the HTML pagination controls in Django Rest Framework by creating a custom template for the pagination controls. This is done by using the template_name attribute on the PageNumberPagination class to specify the path to the template. For example, if you have a template called my_pagination.html in your templates directory.
You can use the PAGE_SIZE attribute to set the number of items per page, which allows clients to request different page sizes using a query parameter, e.g., /api/users?page_size=50. This customization allows for better performance and is achieved by adjusting the page size dynamically based on user needs.
To use a custom template for the pagination controls, you can set the template_name attribute on the PageNumberPagination class in your views.py file. This is done by specifying the path to the custom template, such as my_pagination.html. By doing this, you can create a custom look and feel for your pagination controls.
You can customize the template my_pagination.html to use the context variables provided by the pagination class to create the HTML controls. This is achieved by using the variables provided by the pagination class, such as the current page number and the total number of pages.
Drf Proxy
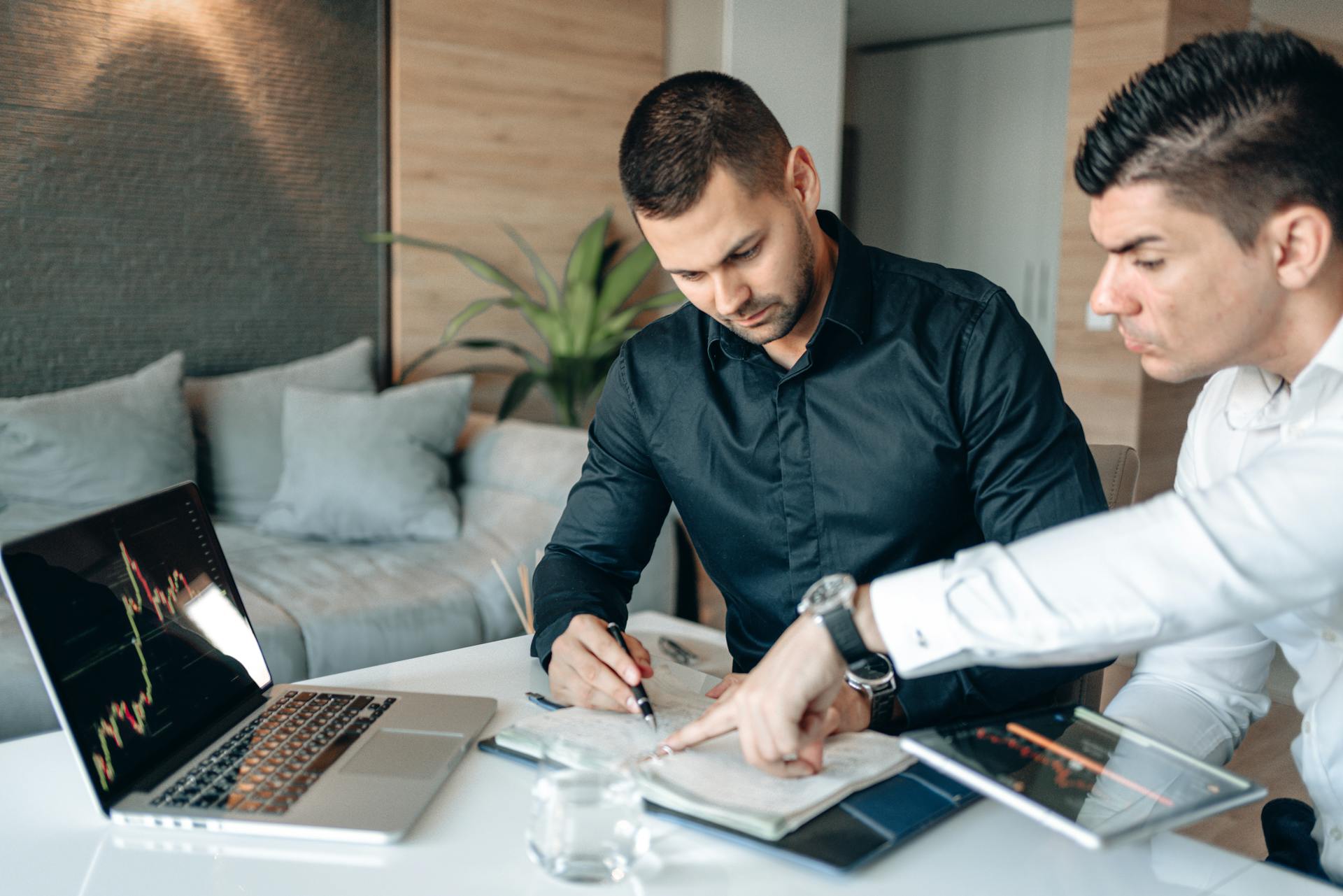
Drf-proxy-pagination is a package that provides a way to paginate a proxy view in Django Rest Framework (DRF). This package allows you to paginate the response from the proxied server so that you can display the data in a paginated format in your client-side application.
You can install DRF-proxy-pagination by running the following command: pip install drf-proxy-pagination.
To use this package, you will need to add it to your Django project's installed apps by adding 'drf_proxy_pagination' to your INSTALLED_APPS in your settings.py file.
You will also need to configure the proxy view and the pagination settings in your views.py.
Here are the basic steps to get started with DRF-proxy-pagination:
- Installation: You can install DRF-proxy-pagination by running the following command: pip install drf-proxy-pagination.
- Add to installed apps: Add 'drf_proxy_pagination' to your INSTALLED_APPS in your settings.py file.
- Configuration: To use this package, you will need to configure the proxy view and the pagination settings in your views.py.
Advanced Pagination Topics
Advanced Pagination Topics can be a bit tricky, but don't worry, I've got you covered. One way to implement business-specific rules into your pagination logic is to create custom pagination classes by subclassing the built-in paginators.
You can create a paginator that only returns even-numbered pages by subclassing the built-in paginators, as demonstrated in the example of Advanced Pagination Customization. This is a powerful feature that allows you to tailor your pagination to meet the unique needs of your application.
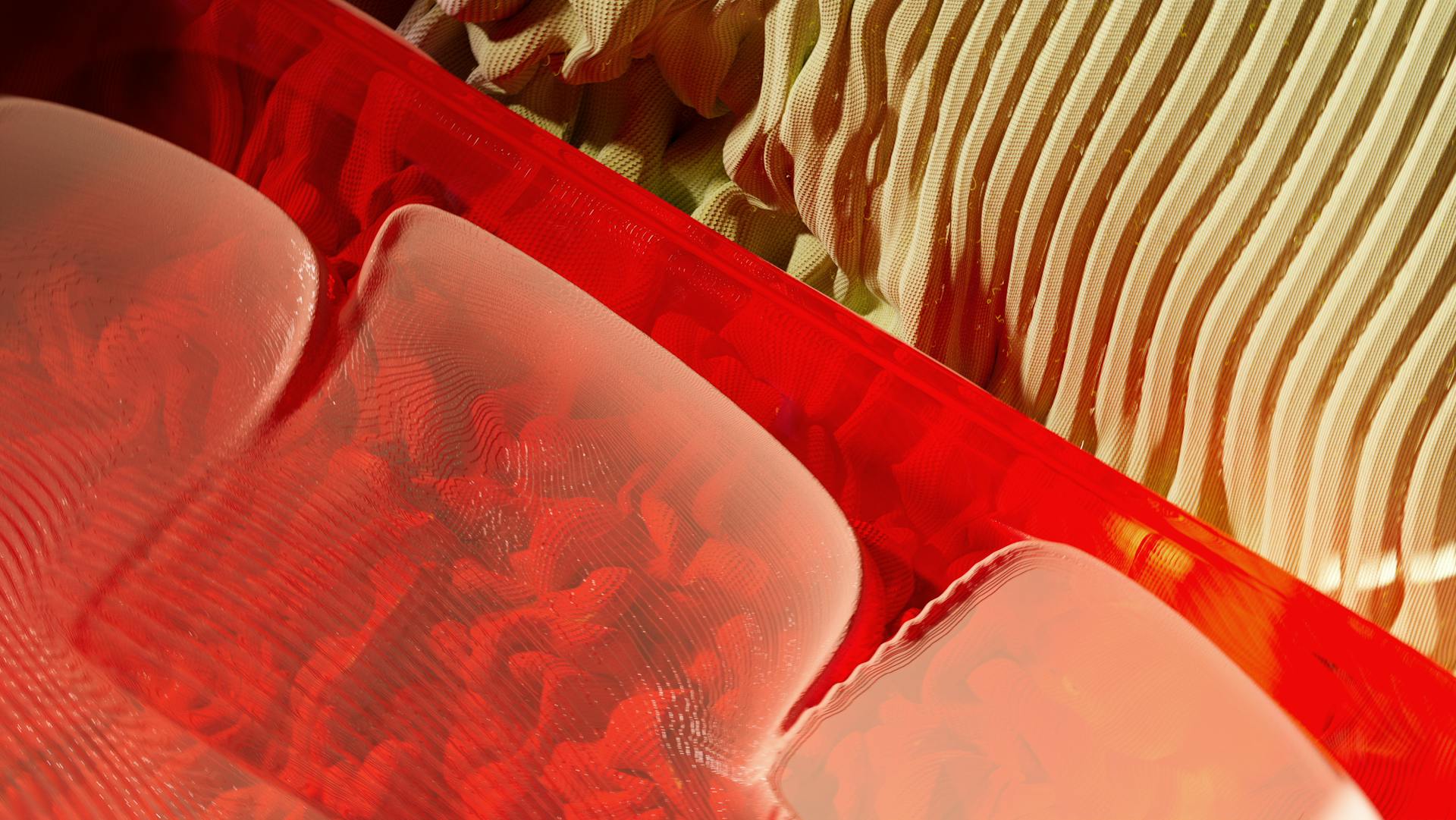
Using CursorPagination is a good idea when working with large data that has frequent updates, as it helps to improve performance and reduce the load on your database.
Here are some best practices to keep in mind when working with large datasets:
- Use CursorPagination for large data with frequent updates.
- Optimize your database queries with indexes and avoid unnecessary joins.
- Cache responses when possible to improve performance.
- Set appropriate timeouts to prevent long-running requests.
By following these best practices, you can ensure that your application is scalable and efficient, even when dealing with large datasets.
Sources
- https://medium.com/@KaziMushfiq1234/pagination-in-django-rest-framework-limitoffsetpagination-118dad998975
- https://www.geeksforgeeks.org/adding-pagination-in-apis-django-rest-framework/
- https://www.pythontutorial.net/django-tutorial/django-rest-framework-pagination/
- https://python.plainenglish.io/pagination-in-django-rest-framework-how-to-optimize-api-responses-03ce7f1da45b
- https://www.scaler.com/topics/django/searching-sorting-and-pagination-in-django/
Featured Images: pexels.com