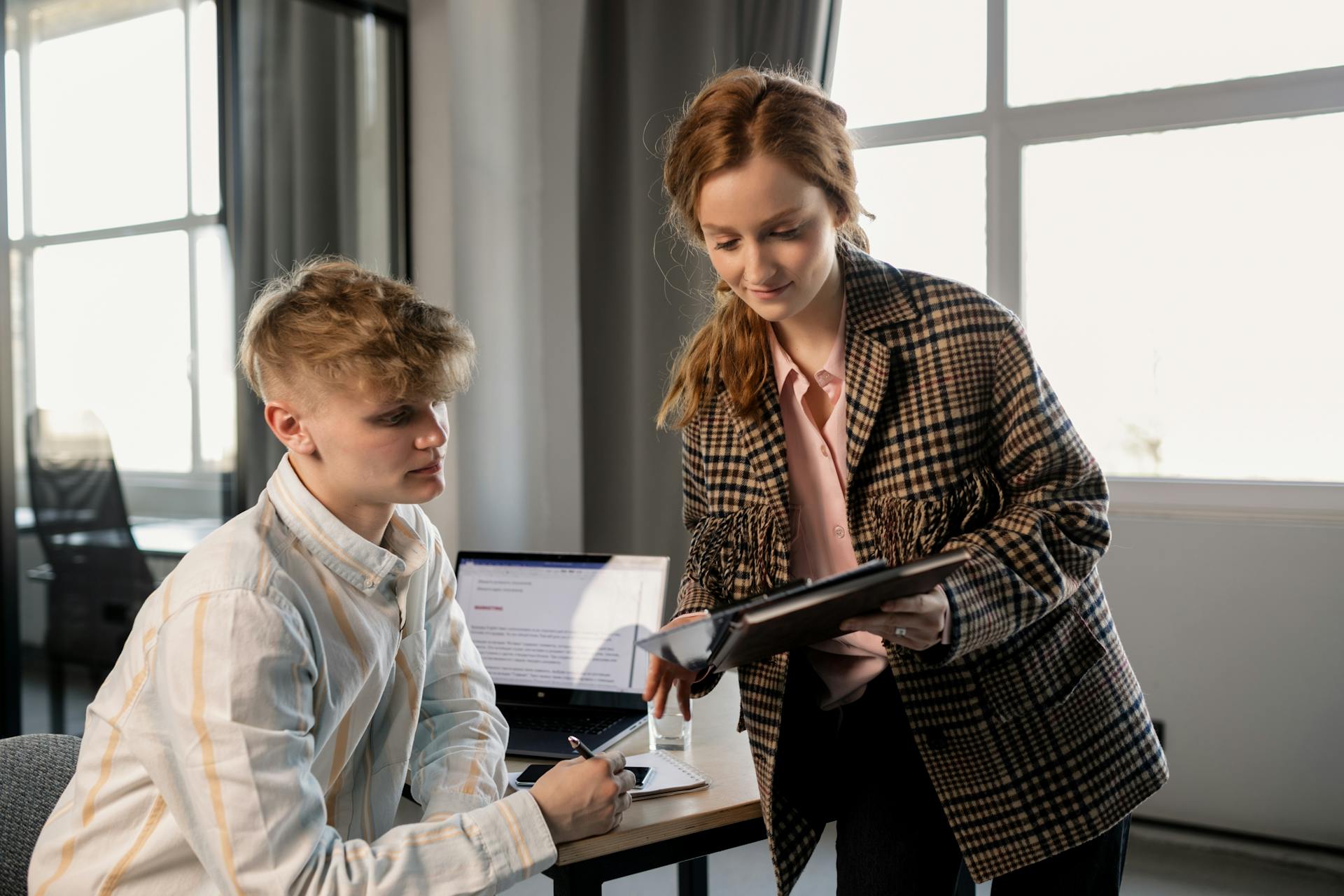
Django provides a simple way to upload and store files to Dropbox using the Django Dropbox library.
You can install the library using pip, which is a package installer for Python.
This library allows you to store files in Dropbox by creating a new file object and specifying the file path and content.
The library also provides a way to upload files by sending a POST request to the Dropbox API endpoint.
The Dropbox API endpoint requires authentication, which can be done using the OAuth 2.0 flow.
You can use the library to store any type of file, including images, videos, and documents.
The library also provides a way to store files in a specific folder in Dropbox.
You can specify the folder path when creating a new file object.
The library takes care of the underlying Dropbox API calls, making it easy to use and integrate into your Django application.
This makes it a great solution for storing and serving files in a Django application.
Installation
To get started with django dropbox, you'll need to install the Dropbox storage library in your project. This can be done by running the command `pip3 install django-storages[dropbox]` in your terminal.
If you're using Ubuntu, you'll want to use `pip3` instead of `pip` to install the library. The command will install the necessary packages for django-storages with Dropbox support.
Once installed, you'll be ready to set up your django app to handle file uploads to Dropbox.
Install Storage Library
To install a storage library, you'll need to take a few steps. Open your terminal in the project directory and get ready to write some commands.
For Ubuntu users, the command to install the Django Storages library for Dropbox is pip3 install django-storages[dropbox]. This will get you set up with the necessary storage library.
You can also use Docker to install Django Storages for Dropbox. The command for this is $ docker-compose exec web pipenv install django-storages[dropbox]. This will install the library using pipenv within the Docker container.
Django File Upload
To set up file uploads in Django, you'll need to install the Dropbox Python SDK. This will allow you to upload files to Dropbox from your Django app.
First, you'll need to add the Dropbox app key and secret key to your settings.py file. You can find these in the Dropbox app you created.
Next, add the access token and access token secret to the DROPBOX_APP_ACCESS_TOKEN and DROPBOX_APP_ACCESS_TOKEN_SECRET attributes in settings.py. You can follow the Getting Started Guide to get these.
To handle file uploads, add the DropboxFileUploadHandler to an app in your Django project, such as testapp, and reference it in the FILE_UPLOAD_HANDLERS in settings.py.
The DropboxFile returned by the upload handler is an instance of httplib.HTTPResponse, so you can use basic methods like read, but not file-like methods.
Integration
To integrate Dropbox with your Django project, you can use the DropBoxStorage backend. Simply open your project's settings.py file and add the following code.
The easiest way to integrate Dropbox with Django is to use the DropBoxStorage backend. This method requires you to paste your own Dropbox access token into your settings.py file. Here's how you do it:
- Open settings.py in your Django project
- Write the following code in your settings.py file: `DEFAULT_FILE_STORAGE = 'storages.backends.dropbox.DropBoxStorage'` and `DROPBOX_ACCESS_TOKEN = 'your access token'`
Alternatively, you can use a custom file upload handler to upload files from your application to Dropbox. This method requires you to install the Dropbox Python SDK and add the DropboxFileUploadHandler to your Django app.
Integration with Django
Integrating with Django is a breeze. To get started, open settings.py in your Django project.
You'll need to add two lines of code to your settings.py file. The first line defines the default file storage using Dropbox. The second line requires you to paste your own Dropbox access token.
Here's a step-by-step guide to add the necessary code:
- Open settings.py in your Django project
- Write `DEFAULT_FILE_STORAGE = 'storages.backends.dropbox.DropBoxStorage'`
- Write `DROPBOX_ACCESS_TOKEN = 'your access token'`
That's it! You're done with the integration process.
Authentication
Authentication is a crucial step in integrating with Dropbox. Two methods of authentication are supported: using an access token or using a refresh token with an app key and secret.
Dropbox has recently introduced short-lived access tokens, which can be identified by their prefix (short-lived access tokens start with 'sl.').
You can obtain the refresh token by following the instructions below. This involves using the commandline-oauth.py example from the Dropbox SDK for Python.
Here are the variables you'll need to obtain the refresh token:
- oauth2_access_token or DROPBOX_OAUTH2_TOKEN
- oauth2_refresh_token or DROPBOX_OAUTH2_REFRESH_TOKEN
- app_secret or DROPBOX_APP_SECRET
- app_key or DROPBOX_APP_KEY
One Answer
If you're looking for a simpler way to integrate Dropbox with Django, there's a great alternative to writing a custom file upload handler. Just use django-storages, which has a Dropbox implementation ready to go.
To get started, install django-storages using pip. Then, in your settings file, add 'storages.backends.dropbox.DropBoxBackend' to the DEFAULT_FILE_STORAGE setting.
This will save you the hassle of setting up a custom file upload handler, and it's a great way to get started with integrating Dropbox into your Django app.
Frequently Asked Questions
Does Dropbox use Django?
Dropbox is used as a backend, not as the main framework, in a Django file storage solution. This setup leverages the official Dropbox SDK for Python to integrate Dropbox with Django.
Sources
- https://django-storages.readthedocs.io/en/latest/backends/dropbox.html
- https://harshkanani014.medium.com/how-to-store-your-django-media-files-in-dropbox-7463292afa18
- https://skydata.hashnode.dev/django-with-dropbox-storage
- https://agiliq.com/blog/2012/07/dropbox-file-upload-handler-for-django/
- https://stackoverflow.com/questions/17386741/how-to-use-dropbox-as-django-media-files-storage
Featured Images: pexels.com