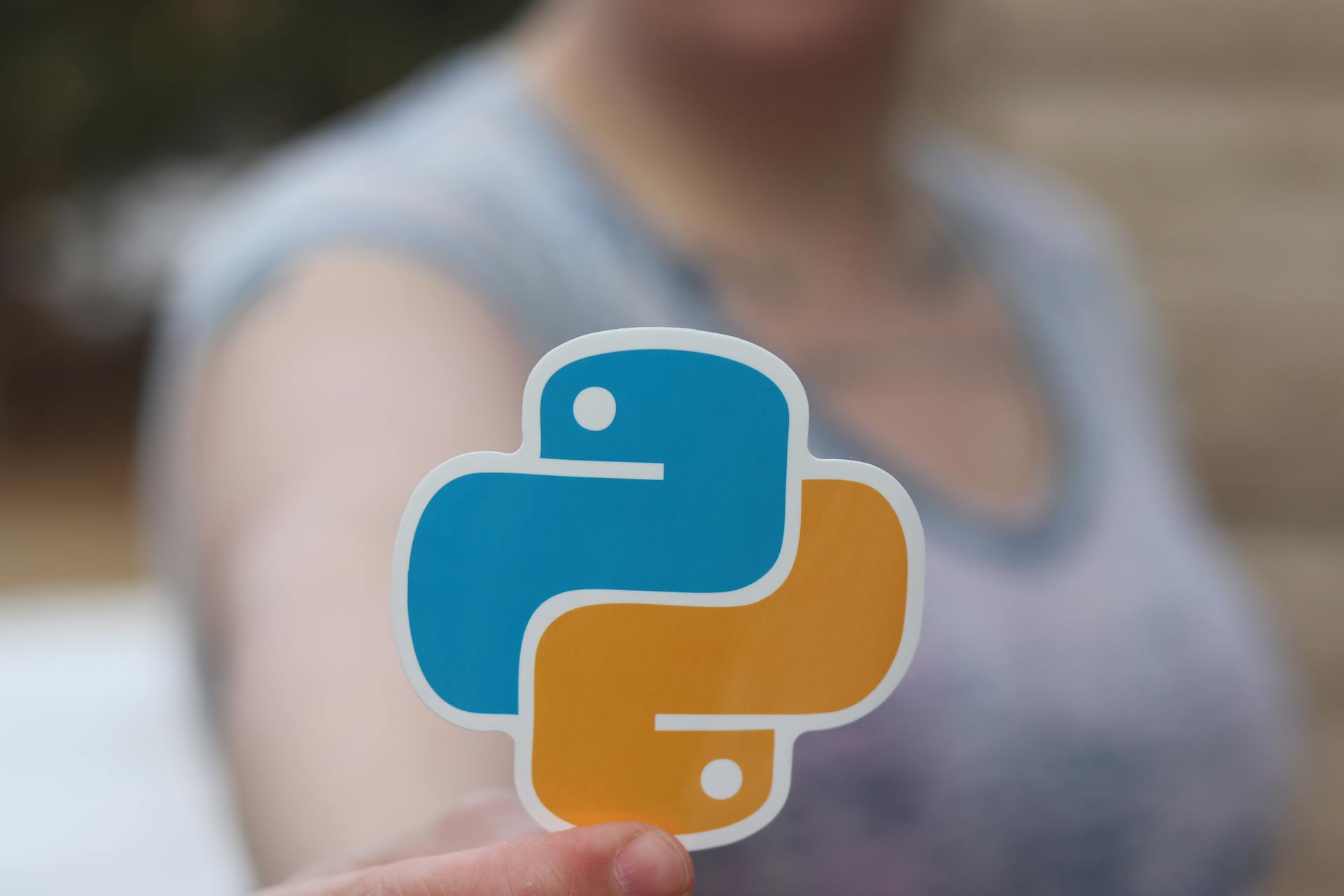
Creating a Django project from scratch is a straightforward process. You start by installing the Django framework using pip.
The next step is to create a new Django project by running the command `django-admin startproject projectname`. This will create a new directory with the basic structure for a Django project.
You'll notice that the project directory contains several files and folders, including `manage.py`, `projectname`, and `settings.py`. These files are essential for setting up and configuring your project.
To create a new Django app within your project, navigate to the project directory and run the command `python manage.py startapp appname`.
Setting Up the Project
To set up your Django project, start by creating a new directory for it. Create a virtual environment to manage dependencies by running the command `python -m venv venv` on Windows or `python3 -m venv venv` on Linux and macOS.
You'll find several files in the `venv` directory, including a copy of the Python standard library. To activate the virtual environment, run the command `venv\Scripts\activate` on Windows or `source venv/bin/activate` on Linux and macOS.
Once you've activated the virtual environment, install Django using pip: `pip install django`. This will set you up to create the application.
Database and Storage
The settings.py file contains the configuration for your SQL database, using the env.db() helper from django-environ to load the connection string set in DATABASE_URL into the DATABASES setting.
To set up a Cloud SQL for PostgreSQL instance, you'll need to create a PostgreSQL instance, database, and database user for your app. This is a good choice because Django officially supports multiple relational databases, but offers the most support for PostgreSQL.
Creating a PostgreSQL instance takes a few minutes, and it's ready for use once it's created. You can replace the instance in the tutorial with your own instance.
Within the created instance, you'll need to create a database, which is a self-contained collection of data.
A database user is also required, which gets additional database rights by default.
Here are the steps to create a PostgreSQL instance, database, and database user:
- Create the PostgreSQL instance.
- Within the created instance, create a database.
- Create a database user.
Project Structure and Organization
A Django project is structured around a single project that's split into separate apps, each handling a self-contained task. This is like Instagram, where user management, image feed, and private messaging are all separate pieces of functionality.
Each app has its own database, functions to control data display, and its own URLs, HTML templates, and static files like JavaScript and CSS. This separation of logic is key to a well-organized Django project.
Here's a breakdown of the key files and directories in a Django project:
- myDjangoProject directory: The container for your project.
- myDjangoProject/__init__.py: An empty file telling Python this directory is a Python package.
- myDjangoProject/settings.py: Configuration for your Django project.
- myDjangoProject/urls.py: URL declarations for your Django project.
- myDjangoProject/wsgi.py: The entry-point for WSGI-compatible web servers.
- templates directory: Holds Django templates.
- todo directory: Contains files for developing a Django application.
- migrations directory: Used to propagate changes to your models into your database schema.
- manage.py: A command-line utility for interacting with your Django project.
Understanding Website Structure
A Django website is made up of a single project that's split into separate apps, each handling a self-contained task.
Each app has its own database, and it'll have its own functions to control how it displays data to the user in HTML templates.
You can think of an application like Instagram, where there are separate pieces of functionality like user management, the image feed, and private messaging.
These are each separate apps inside a single Django project.
A Django project holds some configurations that apply to the project as a whole, such as project settings, URLs, shared templates, and static files.
Here's a breakdown of the typical components of a Django app:
- Model defines the data structure, usually the database description and often the base layer to an application.
- View displays some or all of the data to the user with HTML and CSS.
- Controller handles how the database and the view interact.
In Django, the architecture is slightly different, using the model-view-template (MVT) pattern, where the view and template make up the view in the MVC pattern of other web frameworks.
A Django site starts off as a project, and you build it up with a number of applications that each handle separate functionality.
Exploring Structure
A Django project is made up of a single project that's split into separate apps, each handling a self-contained task. Each app follows the model-view-template pattern.
The project directory contains a number of important files and directories, including the project's configuration settings, URL declarations, and an entry-point for WSGI-compatible web servers.
The project directory is denoted with a bold font and contains the actual Python package for your project. This directory is the root of your project.
The project directory includes an __init__.py file, which tells Python that this directory should be considered a Python package. This file is empty.
The project directory also includes a settings.py file, which contains configuration for your Django project. This file is crucial for setting up your project.
The project directory has a urls.py file, which contains the URL declarations for your Django project. This file is used to map URLs to views.
The project directory includes a wsgi.py file, which defines an entry-point for WSGI-compatible web servers to serve your project. This file is used for deployment.
The project directory contains a templates directory, which will hold your Django templates. This directory is currently empty.
The project directory also includes a todo directory, which contains all the files required for developing a Django application. This directory is where you'll write your code.
Here's a breakdown of the project directory structure:
- myDjangoProject directory (project container)
- myDjangoProject (actual Python package)
- __init__.py (empty file)
- settings.py (project configuration)
- urls.py (URL declarations)
- wsgi.py (entry-point for WSGI-compatible web servers)
- templates (Django templates)
- todo (Django application files)
- migrations (database schema changes)
- manage.py (command-line utility)
Django Configuration and Settings
To get your Django project production-ready, you need to configure it properly.
Django has built-in protection against Cross Site Request Forgery (CSRF), but to offer the best protections, you need to tell Django what its hosted URL is.
You can do this by supplying the app's URL as an environment variable in the settings.py file.
The settings module for your project is stored in tutorial/settings.py, so open that in a text editor.
To add rest_framework to the project, add it to the end of the INSTALLED_APPS list in the settings.py file.
Admin and User Management
You need to create an admin account for yourself to access the Django admin site. This is done by running the createsuperuser management command.
The Django admin site comes packed with features, including user authorization and the ability to manage your portfolio projects from a browser window. To access your Project model, you need to register the model first in the admin.py file of your projects app.
The admin site allows you to add, edit, and manage content, but by default, it's complicated to read descriptions of tasks because they're named as ToDoItem object (1) in the list. To make content management easier, you can register your models in the admin interface.
To set up an admin site, you need to create a superuser by specifying your email address and password in the manage.py console. Then, you can log in to the administration page and register your models to make them appear in the admin site.
Here are the steps to create a superuser and set up the admin site:
- Create a superuser by running the createsuperuser management command.
- Specify your email address and password in the manage.py console.
- Log in to the administration page at http://127.0.0.1:8000/admin/.
- Register your models in the admin.py file to make them appear in the admin site.
By following these steps, you'll be able to manage your users and content from the comfort of your browser window.
Views and Templates
Views in Django are functions or classes that handle the logic for each URL visited by a user. They're defined in the views.py file in an app's directory.
A view function takes at least one argument, request, which contains metadata about the page request. This is created whenever a page loads.
To tell Django when to serve a view, you need to create a new route to the project. This is done by mapping a URL to a view function.
Django views can return various types of responses, including HTML, JSON, or XML. In the case of views that return HTML, they typically render an HTML template.
The render() function in a view looks for HTML templates inside a directory called templates/ in the app directory. It's a good practice to add a subdirectory with the app's name inside the templates/ directory.
Configuring URLs
The next step is to configure the main urls.py file in your project directory to include the paths from todo/urls.py. You'll need to import django.urls.include and add the following code: from django.contrib import admin from django.urls import path, include urlpatterns = [ path("todo/", include("todo.urls")), path("admin/", admin.site.urls), ]
Now, you can access your todo list by opening the page http://127.0.0.1:8000/todo/ in your browser. You should see the following text:
The Views
The views in Django are functions or classes that specify how web requests are processed and which web responses are returned. They are defined in the views.py file in an app's directory.
A view function takes at least one argument, request, which is created whenever a page loads and contains metadata about the page request. You can learn more about request objects in the Django documentation.
To create a view, you need to define a function or class that handles the logic for a specific URL. For example, you can create a view function named home() that renders an HTML file named home.html.
Views can also be classes that inherit from Django's ListView basic view class, as shown in Example 3. This allows you to display a list of items, such as to-do's, in a template.
In a view, you can use the render() function to render an HTML template. The render() function looks for HTML templates inside a directory called templates/ in your app directory. You can also use the context dictionary to pass data from the view to the template.
For example, in Example 2, the project_index.html template uses a for loop to iterate over a list of projects and display each project's details. The for loop syntax in the Django template engine is as follows: {% for project in projects %}.
In a view, you can also use the request object to access information about the current request. For example, you can use the request.GET or request.POST attributes to access query string parameters or form data.
Models and Serializers
In Django, models define the fields and behaviors of your data, represented by Python classes that are subclasses of django.db.models.Model.
A model, like the ToDoItem model, has class variables represented by instances of field classes. For example, the ToDoItem model has two class variables: text and due_date.
These field classes, such as CharField and DateField, are used to store specific types of data. In the case of the ToDoItem model, text is used to store the description of what should be done, and due_date is used to store the deadline for the to-do.
To translate this information into valid response data, you'll need to define serializers, which are used to represent data in a specific format. In Django, serializers are used to convert complex data types, such as instances of models, into native Python data types that can be easily rendered into JSON or other formats.
Creating a Model
In Django, models define the fields and behaviors of your data, represented by Python classes that are subclasses of the django.db.models.Model class.
A model is created by defining class variables that are instances of field classes. These field classes determine the type of data that will be stored in the database.
Let's take a look at the ToDoItem model, which has two class variables: text and due_date. The text field is an instance of the CharField class, used to store the description of what should be done.
The due_date field is an instance of the DateField class, used to store the deadline for the to-do.
Here's a brief overview of the field classes used in the ToDoItem model:
- CharField: used to store text data, such as descriptions or notes.
- DateField: used to store date data, such as deadlines or due dates.
Implement Serializers and Views
In Django, you'll need to define serializers to translate your information objects into valid response data. These serializers are created in a module named serializers.py, which you'll use for data representations.
To create a view, you'll need to open the views.py file and append the necessary code. This file already exists in your project and has some boilerplate text, so keep that and add the new text.
Views in Django are functions or classes that specify how web requests are processed and which web responses are returned. By convention, views are defined in the views.py file, located in your Django application directory.
You can define a view like the AllToDos class, which inherits from the Django ListView basic view class. This view will be used to display all available to-do's.
Install Django REST Framework
To install Django REST Framework, you'll need to install Django and the Django REST framework first.
You can install Django and Django REST using pip, a package installer for Python.
Install Django and the Django REST framework by running the command pip install django djangorestframework.
Next, install the Python modules for Django and Django REST.
This will give you the necessary tools to start building your API.
Make sure you're using the latest version of Django and Django REST framework for the best results.
You can check the versions by running the command pip show django and pip show djangorestframework.
Deployment and Testing
You can deploy your Django project to the App Engine standard environment once you've set up your backing services and tested the app locally.
To deploy the app, run the command `gcloud app deploy`, which will deploy the app as described in `app.yaml` and set the newly deployed version as the default version, causing it to serve all new traffic. Confirm the settings by typing "yes" when prompted.
The deployment process will take some time, and you'll need to wait for the message that notifies you that the update has completed.
After the update is complete, open `app.yaml` and update the value of `APPENGINE_URL` with your deployed URL.
Deploy to Standard
Deploying your app to the App Engine standard environment is a straightforward process. First, make sure your backing services are set up and your application is tested locally.
To deploy, run the command `gcloud app deploy` to upload your app as described in `app.yaml` and set the newly deployed version as the default version. This will cause it to serve all new traffic.
Confirm the settings by typing "yes" when prompted. This is a crucial step to ensure everything is set up correctly.
Wait for the message that notifies you that the update has completed. This may take a few minutes, depending on the size of your app.
Once deployed, open `app.yaml` and update the value of `APPENGINE_URL` with your deployed URL. This will allow you to access your app from the correct location.
To finalize the deployment, upload your configuration changes by running the command `gcloud app deploy` again.
Run Locally
To run your app locally, you'll need to start the Cloud SQL Auth Proxy in a separate terminal. This establishes a connection from your local computer to your Cloud SQL instance for local testing purposes.
The Cloud SQL Auth Proxy is started by running the command `./cloud-sql-proxy PROJECT_ID:REGION:INSTANCE_NAME cloud-sql-proxy.exe PROJECT_ID:REGION:INSTANCE_NAME` in a separate terminal. Keep this process running the entire time you test your app locally.
Next, set the Project ID locally by running the command `export GOOGLE_CLOUD_PROJECT=PROJECT_ID` and then `set GOOGLE_CLOUD_PROJECT=PROJECT_ID`. This allows the Secret Manager API to use the Project ID.
You'll also need to set an environment variable to indicate you're using Cloud SQL Auth Proxy. This is done by running the command `export USE_CLOUD_SQL_AUTH_PROXY=true` and then `set USE_CLOUD_SQL_AUTH_PROXY=true`.
To set up your models and assets, run the Django migrations by using the following commands: `python manage.py makemigrations`, `python manage.py makemigrations polls`, `python manage.py migrate`, and `python manage.py collectstatic`.
Finally, start the Django web server by running the command `python manage.py runserver 8080`. You can then access your app by going to `http://localhost:8080` in your browser. If you're in Cloud Shell, click the Web Preview button and select Preview on port 8080.
Testing the
Testing the application is crucial to catch oversights like displaying past due tasks on the "All tasks" page. You can avoid such mistakes by introducing tests while developing applications.
A great place to start is by checking the tests.py file in the myDjangoProject/todo directory, which is intended for Django tests. This file contains the AllToDosViewTest class with methods like test_today, test_last_week, and test_next_week.
Each method creates a task with a specific due date and checks if it's rendered on the application's home page. The task with a due date 7 days before the current date shouldn't be displayed, but in the example, it was.
To run this test, you can right-click the background of the file tests.py in the editor, choose the option Run, or just press Ctrl+Shift+F10. The test results will be shown in the Test Runner tab of the Run tool window.
The test results showed that test_last_week had failed because the created to-do was added to the list of items that will be displayed on the home page despite being past due. This is where you need to fix the code.
To fix it, you can add the get_queryset method to the AllToDos class. This method will filter the objects, so that the view returns only those tasks whose due dates are greater than or equal to today's date.
Frequently Asked Questions
What are Django projects?
Django projects are Python-based applications that allow you to build and manage your data model without needing SQL, using an object-relational mapper to simplify database interactions. This enables developers to work within a fully Python environment, streamlining the development process.
Is Django for backend or frontend?
Django is primarily used for backend web development, with a focus on building efficient and scalable server-side applications. While it offers some front-end support, its main strength lies in handling backend tasks.
Sources
- https://cloud.google.com/python/django/appengine
- https://realpython.com/get-started-with-django-1/
- https://www.jetbrains.com/help/pycharm/creating-and-running-your-first-django-project.html
- https://opensource.com/article/19/11/python-web-api-django
- https://www.geeksforgeeks.org/how-to-create-a-django-project/
Featured Images: pexels.com