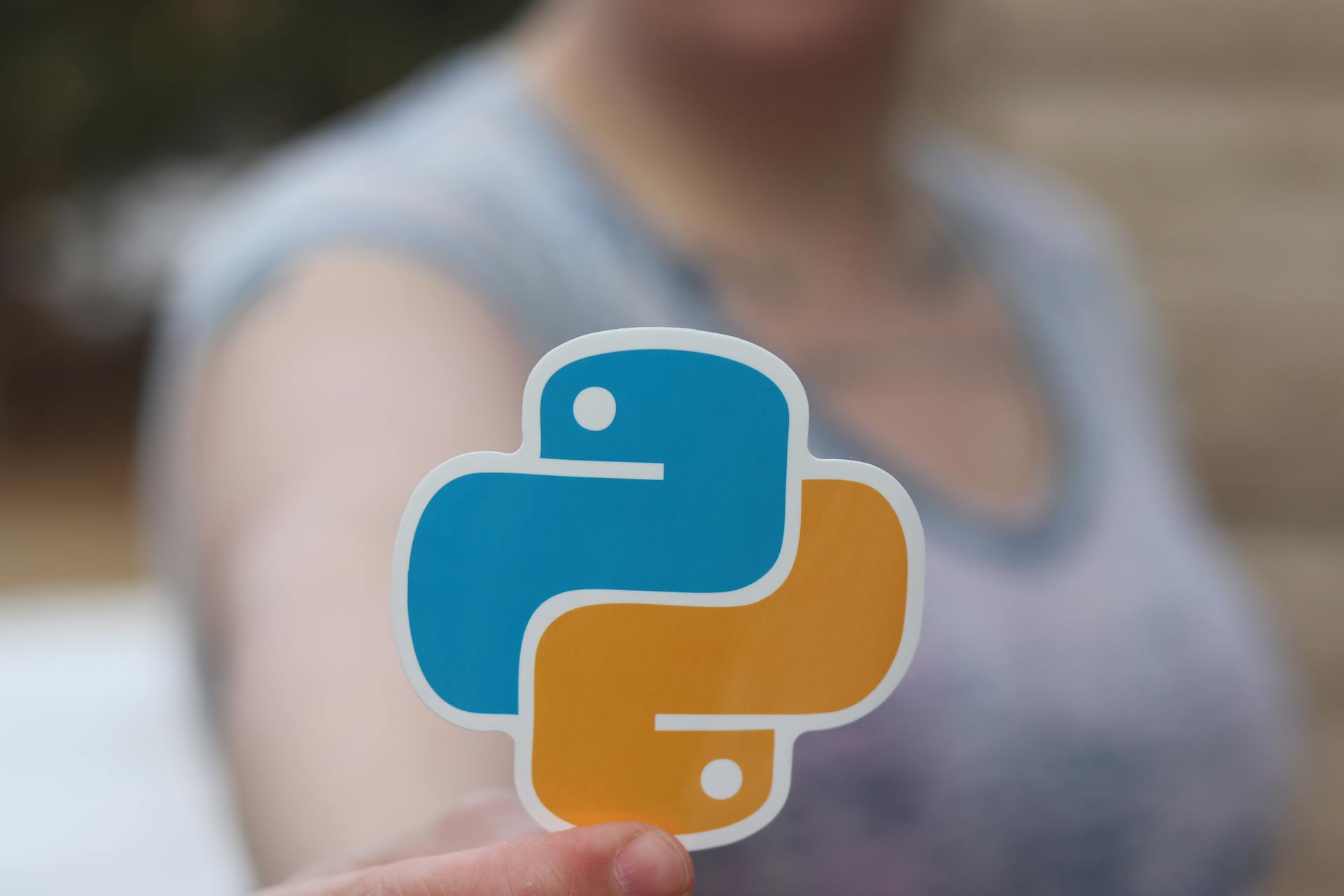
Running a Django project from scratch requires setting up a new Django project and creating a new Django app. This can be done using the command `django-admin startproject projectname` in your terminal.
First, make sure you have Python and pip installed on your computer. You can check this by opening a terminal and typing `python --version` and `pip --version` to see their respective versions.
To create a new Django project, navigate to the directory where you want to create the project and run the command. The command will create a new directory with the project name and a basic directory structure.
Next, navigate into the newly created project directory and run the command `python manage.py runserver` to start the development server.
Take a look at this: New Nextjs Project Typescript
Getting Started
To get started with running a Django project, you'll need to have Python 3.6 or higher installed on your Windows machine. You can download it from the official Python website.
You'll also need to install Pipenv, which you can do by running a command in your Command Prompt (CMD) after Python is installed. If you're not sure how to open CMD, you can search for it using Windows search.
Make sure you have Python 3.6 or higher installed, and then you can proceed to install and launch Django.
Check this out: Make Sure Onedrive - Personal Is Running on Your Pc
Before We Begin
Before we dive into the world of Django, make sure you have the necessary tools installed on your Windows machine.
To get started, you'll need to have Python 3.6 or higher installed, which you can download from the official Python website.
You'll also need to have Pipenv installed, which is a package manager for Python that makes it easy to manage dependencies.
If you don't have Pipenv installed, you can easily install it by running a command in your Command Prompt (CMD) after you've installed Python.
To open the Command Prompt, simply search for "CMD" in Windows search.
Once you have these prerequisites installed, you'll be ready to move on to the next step and install Django.
Consider reading: Install Django Project
Python Tutorial
Python is a versatile programming language that's perfect for beginners. You can start creating projects right away, like building a web application with the Django framework.
To begin with Python, you'll need to set up the necessary tools and environment. Now you are ready to create your first Python Django project.
One of the best resources to learn Python is the Django framework, which offers a comprehensive tutorial to get you started.
Setting Up Django
To set up Django, start by installing Python from the official website and verifying the version installed. You can do this by opening the terminal and typing `python --version`.
To install the necessary tools, you'll need to install virtual environment wrapper using pip. Simply type `pip install virtualenvwrapper-win` in the terminal.
Next, create a virtual environment by typing `mkvirtualenv environment_name`. This will create a new environment for your project.
After that, install Django by typing `pip install Django` in the terminal. This will install the Django framework and its dependencies.
Here are the steps to set up Django in a concise format:
Now that you have Django installed, you can proceed to import your application into the project settings. This is done by manually adding your app to the `Djangoproject/settings.py` file.
Project Configuration
To configure your Django project for production, you'll want to ensure that your application is production-ready. This involves taking further steps to secure and optimize your deployment.
The structure of your project is visible in the Project tool window (Alt+1), where you'll see the following directories and files:
- myDjangoProject directory is a container for your project.
- myDjangoProject/settings.py: This file contains configuration for your Django project.
- myDjangoProject/urls.py: This file contains the URL declarations for your Django project.
- myDjangoProject/wsgi.py: This file defines an entry-point for WSGI-compatible web servers to serve your project.
The manage.py utility lets you interact with your Django project, allowing you to run commands and manage your application.
Installing a Distribution-Specific Package
Installing a distribution-specific package is a viable option for getting started with Django, especially if you're using a specific distribution or platform. This method allows for automatic installation of dependencies and supported upgrade paths.
Distribution-provided packages will rarely contain the latest release of Django. This is because they are typically maintained by the distribution's package maintainers, who may not be able to keep up with the latest developments in the Django project.
Intriguing read: Package Django Project into Exe
To install a distribution-specific package, you should check the distribution-specific notes for your platform or distribution. This will give you information on whether your distribution provides an official Django package or installer.
You can then follow the instructions provided by your distribution to install the package. This may involve running a package manager command, such as apt-get or yum, to install the Django package.
Here are some examples of distribution-specific package managers and their corresponding commands:
Keep in mind that distribution-provided packages may not always be up-to-date, so it's a good idea to check the Django documentation for any specific installation instructions or requirements for your distribution.
Store Secret Values
Storing secret values is a crucial step in project configuration. You can use Secret Manager to store this information securely.
To store secret values, create a file called .env, defining the database connection string, media bucket name, and a new SECRET_KEY value. This file will store sensitive information that should be kept secure.
Here's an interesting read: How to Run an Html File
The sample app uses the Secret Manager API to retrieve the secret value, and the django-environ package to load the values into the Django environment. The secret is configured to be accessible by App Engine standard.
Here are the steps to store the secret in Secret Manager:
- Create a file called .env, defining the database connection string, the media bucket name, and a new SECRET_KEY value.
- Store the secret in Secret Manager by following the instructions in the Secret Manager UI.
- Configure access to the secret by clicking the Permissions tab, then Grant access, and finally select Secret Manager Secret Accessor for the [email protected] account.
The secret is now securely stored in Secret Manager, and can be accessed by the project. This ensures that sensitive information is kept safe and secure.
Exploring Project Structure
Your Django project is now set up, and you're eager to dive in. The project structure is visible in the Project tool window (Alt+1).
The myDjangoProject directory is the container for your project, denoted with bold font.
It contains the actual Python package for your project, which is also named myDjangoProject.
This package has an empty file called __init__.py, which tells Python that this directory should be considered a Python package.
The settings.py file contains configuration for your Django project.
Broaden your view: How to Run an Html File in vs Code
The urls.py file contains the URL declarations for your Django project.
The wsgi.py file defines an entry-point for WSGI-compatible web servers to serve your project.
The templates directory is currently empty and will contain Django templates.
The todo directory contains all the files required for developing a Django application.
The migrations directory contains the package file _init_.py, and will be used in the future to propagate changes to your models into your database schema.
The manage.py file is a command-line utility that lets you interact with your Django project.
Running the App
To run the app, you'll need to set up the Cloud SQL Auth Proxy. This involves starting the proxy in a separate terminal, establishing a connection to your Cloud SQL instance for local testing purposes. Keep the proxy running the entire time you test your app locally.
You'll also need to set environment variables on your local machine. Specifically, you'll need to set the Project ID locally, used by the Secret Manager API, and set an environment variable to indicate you're using Cloud SQL Auth Proxy.
Once you've done this, you can run the Django migrations to set up your models and assets. This involves running the following commands: `python manage.py makemigrations`, `python manage.py migrate`, and `python manage.py collectstatic`. After that, you can start the Django web server with `python manage.py runserver 8080`.
Check this out: How to Run Next Js App
Launch Pipenv Shell
To launch the pipenv shell, run the command that activates the virtual environment created in the previous step, and you'll see the name of the environment displayed in your CMD.
This command will activate the virtual environment, allowing you to work within the isolated environment where Django and its dependencies are installed.
To exit the pipenv virtual environment, simply type "exit" to terminate the environment, and its name will no longer be displayed in the CMD.
Run the App Locally
To run the app locally, start by configuring the backing services. This setup allows for local development, creating a superuser, and applying database migrations. You'll need to run the Cloud SQL Auth Proxy in a separate terminal to establish a connection from your local computer to your Cloud SQL instance for local testing purposes.
To do this, open a new terminal and type: `./cloud-sql-proxy PROJECT_ID:REGION:INSTANCE_NAME`. Keep this process running the entire time you test your app locally. Running it in a separate terminal allows you to keep working while this process runs.
Broaden your view: Nextjs App Folder
Next, set the Project ID locally by typing: `export GOOGLE_CLOUD_PROJECT=PROJECT_ID` and `set GOOGLE_CLOUD_PROJECT=PROJECT_ID`. This is used by the Secret Manager API. Then, set an environment variable to indicate you are using Cloud SQL Auth Proxy by typing: `export USE_CLOUD_SQL_AUTH_PROXY=true` and `set USE_CLOUD_SQL_AUTH_PROXY=true`.
After that, run the Django migrations to set up your models and assets. Type: `python manage.py makemigrations`, `python manage.py makemigrations polls`, `python manage.py migrate`, and `python manage.py collectstatic`. These commands will create the necessary tables in your database and collect static files.
Finally, start the Django web server by typing: `python manage.py runserver 8080`. You can then access your app by going to `http://localhost:8080` in your browser. If you're in Cloud Shell, click the Web Preview button and select Preview on port 8080.
Suggestion: Django Framework Architecture
Admin and Content
To access the admin console, you need to create a superuser. This can be done by running the command `python manage.py createsuperuser` in your terminal.
The admin console can be accessed by going to `http://localhost:8000/admin` in your browser. To log in, use the username and password you specified when creating the superuser.
To add content to your Django project, you need to register your models in the admin interface. This involves creating a superuser and accessing the admin console.
Here's a step-by-step guide to registering your models:
- Create a superuser by running `python manage.py createsuperuser`.
- Access the admin console by going to `http://localhost:8000/admin` in your browser.
- Register your models by adding the following code to your `admin.py` file: `from django.contrib import admin from .models import ToDoItem admin.site.register(ToDoItem)`
- Refresh the page in your browser to see the updated admin interface.
Limit User Privileges
Limiting user privileges is crucial to prevent unauthorized access and maintain database security.
Any users created by Cloud SQL have associated privileges, including CREATEROLE, CREATEDB, and LOGIN, which are part of the cloudsqlsuperuser role.
To prevent the Django database user from having these permissions, you'll need to manually create the user in PostgreSQL.
You can do this using the psql interactive terminal or Cloud Shell, which has this tool pre-installed.
Intriguing read: Google Cloud Platform Project Id
Admin Site Setup
To set up the admin site, create a superuser by running the command `python manage.py createsuperuser` in the terminal. You'll be prompted to enter a username, email, and password.
To access the admin site, start a local web server using `python manage.py runserver` and navigate to `http://localhost:8000/admin` in your browser. Log in using the username and password you created earlier.
To register a model in the admin interface, open the `todo/admin.py` file and add the following code: `from django.contrib import admin from .models import ToDoItem admin.site.register(ToDoItem)`. Refresh the page in your browser to see the updated admin interface.
The admin interface has an Authentication and Authorization section, but you'll need to register your models to add content. For example, to add to-do items, you'll need to register the `ToDoItem` model.
Here's a step-by-step guide to setting up the admin site:
- Create a superuser using `python manage.py createsuperuser`.
- Start a local web server using `python manage.py runserver`.
- Navigate to `http://localhost:8000/admin` in your browser and log in using your superuser credentials.
- Register your models in the `todo/admin.py` file.
By following these steps, you'll be able to access and manage your content in the admin site.
Adding Content
To add content to your project, you need to start by fixing the ToDoItem model. This involves adding a __str__() method to it. Open todo/models.py and add the following code.
We'll create a new template to display the content. To avoid duplicating code, we'll use Django template inheritance. This means we'll create a new template called today.html that will inherit from the index.html template.
For more insights, see: A Basic Html Project
Frequently Asked Questions
How to run command in Django?
To run a command in Django, use the command `django-admin help` followed by the command name or the `--commands` flag to view available options. This will provide detailed information on how to execute the command successfully.
How to run Django project without manage py?
To run a Django project without using `manage.py`, you can use the `runserver` command directly in the project folder. This approach bypasses the need for `manage.py` but requires a basic understanding of Django's project structure and configuration.
Sources
- https://cloud.google.com/python/django/appengine
- https://www.jetbrains.com/help/pycharm/creating-and-running-your-first-django-project.html
- https://www.simplilearn.com/tutorials/python-tutorial/python-django
- https://docs.djangoproject.com/en/5.1/topics/install/
- https://medium.com/powered-by-django/how-to-install-launch-django-windows-bad876b9422
Featured Images: pexels.com