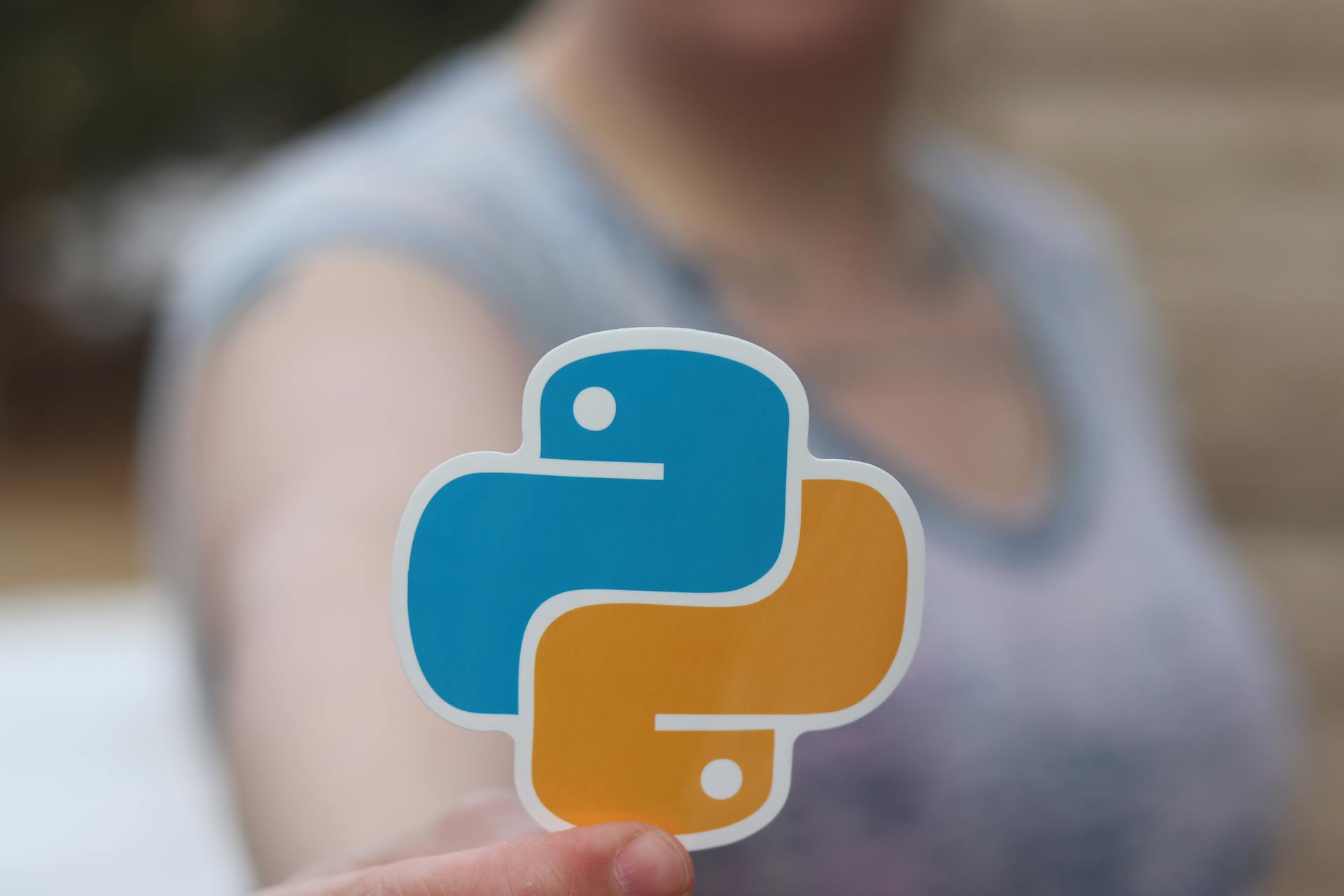
Building a web application with Django is a breeze, thanks to its high-level abstractions and modular design. Django's batteries-included approach means you get a lot of functionality out of the box.
Django's ORM (Object-Relational Mapping) system allows you to interact with databases using Python code, making it easy to perform complex database operations. This is particularly useful for tasks like data modeling, where you can define relationships between models and perform database operations using Python code.
With Django, you can quickly set up a project and start building your web application, thanks to its robust and flexible project structure. This structure includes a project directory, an app directory, and a settings file that lets you configure your project's settings.
If this caught your attention, see: How to Run Django Project
Getting Started
To get started with Django, make sure you're in the correct directory and have activated your virtual environment. Then, run the command `django-admin startproject .` to create your project, and `python manage.py runserver` to start the server.
Django makes it easy to organize your project into different apps, each handling specific functionality. You can start by creating a project, which will automatically set up everything you need, from a folder structure to basic settings.
To initiate a project, open Terminal and enter the command `django-admin startproject projectName`. A new folder with the project name will be created.
You can then enter the project using the terminal by entering the command `cd projectName`. Now, let's run the server and see everything is working fine or not by typing the command `python manage.py runserver`.
Here's a step-by-step guide to getting started with Django:
- Create a new project using `django-admin startproject projectName`
- Enter the project using `cd projectName`
- Run the server using `python manage.py runserver`
Once you've mastered the basics from this tutorial, you can move on to more advanced topics, such as creating apps and views.
Environment Setup
To set up your environment for Django, start by creating a project folder, such as "hello_django".
Create a virtual environment in the project folder by running the following command:
Recommended read: Package Django Project
# Linux: `sudo apt-get install python3-venv` followed by `python3 -m venv .venv` and `source .venv/bin/activate`
# macOS: `python3 -m venv .venv` and `source .venv/bin/activate`
# Windows: `py -3 -m venv .venv` and `.venv\scripts\activate`
Open your project folder in VS Code using the `code .` command or by navigating to File > Open Folder.
To select the virtual environment in VS Code, open the Command Palette and select the "Python: Select Interpreter" command.
A list of available interpreters will appear, including the virtual environment in your project folder that starts with `.venv`.
Select the virtual environment from the list, and then create a new terminal in VS Code by running Terminal: Create New Terminal.
The selected environment will appear on the right side of the VS Code status bar, with a notice that tells you that you're using a virtual environment.
To update pip in the virtual environment, run the command `python -m pip install --upgrade pip` in the VS Code Terminal.
Finally, install Django in the virtual environment by running the command `python -m pip install django` in the VS Code Terminal.
Curious to learn more? Check out: Css Selector vs Xpath
Project Structure
A Django project starts off with some basic files that set the foundation for your web application. These files include manage.py, which is used to interact with your project via the command line.
When you initialize a Django project, you'll see the following files inside the project folder: manage.py, _init_.py, settings.py, urls.py, and wsgi.py.
These files are essential to the structure of your project and help you manage settings, URLs, and deployment. For example, settings.py contains all the website settings, such as registering applications, static file locations, and database configuration details.
Here are the major files you'll find in a Django project:
- manage.py: used to interact with the project via the command line
- _init_.py: a Python package that's invoked when the package or module is imported
- settings.py: contains website settings, such as registered applications, static file locations, and database configuration details
- urls.py: stores all links of the project and functions to call
- wsgi.py: used for deploying the project in WSGI and helps the Django application communicate with the web server
Understanding Website Structure
A Django website is made up of a single project that's split into separate apps, each handling a self-contained task.
Each app has its own database, functions to control how it displays data to the user, and its own URLs, HTML templates, and static files like JavaScript and CSS.
A Django project holds configurations that apply to the project as a whole, such as project settings, URLs, shared templates, and static files.
Django apps follow the model-view-template (MVT) pattern, which is similar to the model-view-controller (MVC) pattern used in other web frameworks.
The MVT pattern separates logic into three main pieces: model defines the data structure, view displays data to the user with HTML and CSS, and template is used to render the view.
Here's a breakdown of the major files you'll find in a Django project:
A Django site starts off as a project, and you build it up with a number of applications that each handle separate functionality.
Ready for Files
To make your project ready for static files, you'll need to add a few lines of code.
In the project's web_project/urls.py file, add the following import statement: from django.contrib.staticfiles.urls import staticfiles_urlpatterns.
This will allow you to serve static files in your project.
Here are the specific steps to follow:
- In the web_project/urls.py file, add the import statement: from django.contrib.staticfiles.urls import staticfiles_urlpatterns.
- At the end of the same file, add the line: urlpatterns += staticfiles_urlpatterns()
By following these steps, you'll be able to serve static files in your project.
Project Creation
To create a Django project, you'll need to create a Django app first. This app will serve as the foundation for your project. In Django, an app is a completely independent module that can be used in multiple projects.
A Django app can be created using the command `python manage.py startapp app_name` in the directory containing `manage.py`. This will create a basic app structure for you.
You can also create your own custom apps in Django. For example, you can create an app called `gfg_site_app` using the command `python manage.py startapp gfg_site_app`. This will create a new app with the specified name.
Here are some benefits of using Django apps:
- Django apps are reusable, meaning they can be used in multiple projects.
- They are loosely coupled, making it easy to work on different components independently.
- Multiple developers can work on different components of an app.
- Debugging and code organization are easy due to Django's excellent debugger tool.
- Django apps come with in-built features like admin pages, reducing the effort of building them from scratch.
Django provides some pre-installed apps for users, which can be found in the `INSTALLED_APPS` list in the `settings.py` file. To consider an app in your project, you need to specify your project name in the `INSTALLED_APPS` list.
A fresh viewpoint: Example Django Project
The Example Portfolio
Creating a portfolio project with Django is a great way to get started with web development. You'll build a Django project with two apps: Pages and Projects.
The Pages app will give you a first impression on how to display content to your website's visitors. It's a great starting point that you can enhance later to meet your own needs.
You can showcase previous web development projects in the Projects app, building a gallery-style page with clickable links to projects that you've completed.
One of the great things about Django is that you don't need to consider external Python packages because Django has so many features included. However, you'll include Bootstrap to help you style the templates.
By building these two apps, you'll learn the basics of Django models, view functions, templates, and the Django admin site.
Here's a rundown of the apps you'll be building:
- Pages: A Django app for displaying content to visitors.
- Projects: A gallery-style page for showcasing web development projects.
Make sure you're in the rp-portfolio/ directory and run the following command in your console:
Admin Site
The admin site is a powerful tool that comes packed with Django, allowing you to manage your portfolio projects from the comfort of your browser window. You can access it by running the development server and visiting http://localhost:8000/admin.
To access the admin site, you need to create an admin account for yourself first. You can do this by running the createsuperuser management command, which will prompt you to choose a username, provide an email address, and set a password. Make sure to remember these credentials, as you'll need them to log in.
Once you've created your admin account, you can start managing your projects in the admin site. To access your Project model, you need to register the model first by adding the code to your projects app's admin.py file.
Here's what you need to do to register the model:
- Open the admin.py file of your projects app
- Add the code below to register the model:
```
from django.contrib import admin
from .models import Project
admin.site.register(Project)
```
* Save the changes and run the development server again.
After registering the model, you can visit http://localhost:8000/admin again and spot that your projects are displayed on the admin site. You can also see the new Image field and the option to choose a file when you click on a project.
By leveraging the Django admin site, you can manage your portfolio projects from the comfort of your browser window instead of the Django shell. This is a great way to streamline your project management process and make it more efficient.
Readers also liked: Hosting Django Website
Database Management
Database Management is a breeze with Django. You work with your database almost exclusively through models you define in code, which Django then handles automatically.
Django's "migrations" system takes care of updating the database schema as you evolve your models over time. The general workflow is to make changes to your models, run `python manage.py makemigrations` to generate scripts, and then `python manage.py migrate` to apply those scripts to the actual database.
For development work, the default db.sqlite3 file is suitable, but for production, consider using a production-level data store like PostgreSQL, MySQL, or SQL Server. This is because SQLite is limited to a single computer and isn't recommended for high traffic sites.
Here are some database types you can use with Django:
Types of Databases
When choosing a database for your Django app, you have several options. SQLite is a good choice for development work, but it's not recommended for high-traffic sites with more than 100 K hits/day.
SQLite is limited to a single computer, which means it's not suitable for multi-server scenarios like load-balancing and geo-replication.
PostgreSQL, MySQL, and SQL Server are better options for production-level data storage.
These databases can handle higher volumes of traffic and are designed for use in multi-server environments.
Worth a look: Web Server Programming
Database Connection
Django comes with SQLite as its default database, which is fine for development work but not recommended for high-traffic sites.
The DATABASES dictionary in your settings.py file shows this default configuration. To change it to another database, you simply need to modify this dictionary.
For example, if you want to switch to PostgreSQL, you'll need to install the required dependencies and set up PostgreSQL first. Then, your DATABASES dictionary will look like this:
Django also supports other databases like MySQL and SQL Server, and you can find more information on how to connect to them in the Database setup section.
Migrate the Database
Migrating your database is a crucial step in database management. It's how you update the database to match the changes you've made to your data models.
To migrate your database, you need to run a few commands in your terminal. First, navigate to your project folder and activate your virtual environment. Then, run the following commands: `python manage.py makemigrations` and `python manage.py migrate`. These commands will update the database schema to match your new data models.
Here's an interesting read: Data Text Html Contenteditable
The `makemigrations` command generates scripts in the migrations folder that record the changes you've made to your data models. These scripts are like a history of all the changes you've made over time.
If you see errors when running the commands, make sure you're not using a debugging terminal that's left over from previous steps, as they may not have the virtual environment activated.
Here are the steps to migrate your database in a nutshell:
- Run `python manage.py makemigrations` to generate migration scripts.
- Run `python manage.py migrate` to apply the scripts to the database.
Remember, you can also use tools like the SQLite browser to work directly with your database, but be careful not to make changes to the database schema, as this will put it out of sync with your app's models.
Database Models
In Django, you define a model as a Python class derived from django.db.models.Model, which you place in an app's models.py file. This class represents a specific database object, typically a table.
Each model has a unique ID field named id, and other fields are defined using types from django.db.models, such as CharField, TextField, EmailField, and more. These field types determine the data type and characteristics of each field, like max_length or choices.
A model class can include methods that return values computed from other class properties, and typically includes a __str__ method for a string representation of the instance.
You can add fields to a model using attributes like max_length, blank=True, and null=True, which determine the field's characteristics. For example, a CharField might have a max_length attribute to limit the text length.
Here are some common field types in Django models:
Django models also support foreign keys and many-to-many relationships, which allow you to link models together.
To create a model, you define a class in models.py and add fields using the available field types. You can then run the makemigrations and migrate commands to create the corresponding database table.
By using Django models, you can easily manage your database and perform CRUD (create, read, update, delete) operations without writing SQL code.
Intriguing read: How to Make a Website Hosting Server
Views and Routes
A view in Django is a collection of functions or classes inside the views.py file in an app's directory, each handling the logic for a specific URL. Each view function takes at least one argument, request, which contains metadata about the page request.
To create a view, you define a function or class in the views.py file, such as the home() function, which renders an HTML file named home.html. You can use the render() function to specify the template to be used, and Django will look for the template in the templates/ directory of your app.
A view function returns an HttpResponse object that contains the generated response, which can be used to display the rendered template to the user. This is done by using the HttpResponse object to return the rendered template, such as the home.html template.
Django URLs are used to map incoming requests to the appropriate views in your web application. You can use regular expressions to define patterns that match specific URL patterns and route them to corresponding views. Each view needs to be mapped to a corresponding URL pattern, which is done via a Python module called URLConf (URL configuration).
Here are some key points to keep in mind when working with views and routes in Django:
- A view is a collection of functions or classes inside the views.py file in an app's directory.
- Each view function takes at least one argument, request, which contains metadata about the page request.
- A view function returns an HttpResponse object that contains the generated response.
- Django URLs are used to map incoming requests to the appropriate views in your web application.
- Each view needs to be mapped to a corresponding URL pattern via a Python module called URLConf (URL configuration).
A View
A view in Django is a collection of functions or classes inside the views.py file in an app's directory. Each function or class handles the logic that the app processes each time the user visits a different URL.
To create a view, you define a function or class that takes at least one argument, request. The request object is created whenever a page loads, and it contains metadata about the page request.
A view function returns an HttpResponse object that contains the generated response. Each view function is responsible for returning an HttpResponse object.
You can create a view function to render an HTML file. For example, you can create a view function named home() that renders an HTML file named home.html. The render() function looks for HTML templates inside a directory called templates/ in your app directory.
Here are some key points to remember when creating a view:
- A view function takes at least one argument, request.
- A view function returns an HttpResponse object.
- You can create a view function to render an HTML file.
- The render() function looks for HTML templates inside a directory called templates/ in your app directory.
To tell Django when to serve the view, you need to create a new route to the project. You can do this by adding a URL configuration for the pages app in the urls.py file.
CRUD Operations
CRUD Operations are the backbone of any web application, and Django makes it incredibly easy to perform these operations. You can interact with your database models using the ORM (Object Relational Mapper) API.
To add a new object to your database, you can use the ORM's database-abstraction API. For example, to create an object of model Album and save it into the database, you can write the following command: `Album.objects.create(name='My Album', release_date='2020-01-01')`.
You can modify an existing object by simply changing its attributes and saving it back to the database. This can be done using the `save()` method. For instance, to modify an existing object of model Album, you can write: `album = Album.objects.get(id=1); album.name = 'My New Album'; album.save()`.
Deleting objects is also straightforward in Django. To delete a single object, you can use the `delete()` method. For example, to delete an object of model Album with id 1, you can write: `Album.objects.get(id=1).delete()`.
Here's a quick rundown of CRUD Operations in Django:
Debugger
The debugger is a powerful tool for Django developers. It allows you to step through your code, examine variables, and identify bugs.
To use the debugger with page templates, you need to have "django": true in the debugging configuration. This is already set up for you.
You can set breakpoints in your templates, just like you would in your Python code. For example, in the `hello/home.html` template, you can set breakpoints on the `{% if message_list %}` and `{% for message in message_list %}` lines.
Here are the steps to follow:
- Run the app in the debugger and open a browser to the home page.
- Observe that VS Code breaks into the debugger in the template on the {% if %} statement and shows all the context variables in the Variables pane.
- Use the Step Over (F10) command to step through the template code.
- Use the Debug Console panel to work with variables.
- When you're ready, select Continue (F5) to finish running the app and view the rendered page in the browser.
Best Practices and Careers
Django offers a wide range of career opportunities for developers.
A Django developer can expect to earn between ₹4,00,000 and ₹10,00,000 per year in India, which translates to $50,000 to $90,000 in the United States.
Here are some common Django career roles along with their approximate salary ranges in both INR and USD:
With salaries like these, it's no wonder that Django is a popular choice among developers.
Careers
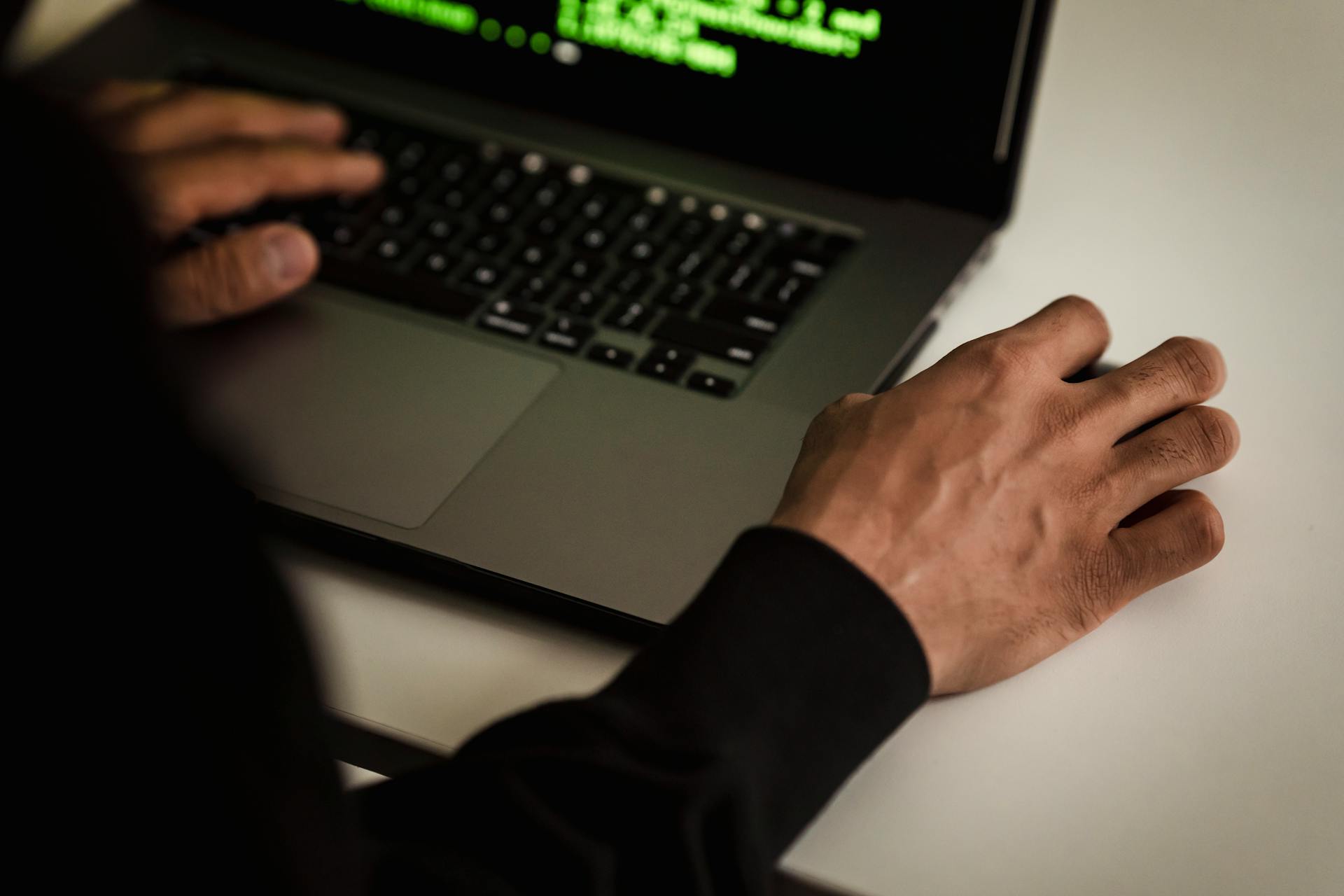
Careers with Django can be very rewarding, with a wide range of roles available. Django Developer is a common role, with salaries ranging from ₹4,00,000 to ₹10,00,000 per year, or $50,000 to $90,000 in the US.
If you're interested in working on the front-end and back-end of a project, a Full-Stack Developer role might be the way to go. These developers can earn between ₹6,00,000 and ₹15,00,000 per year, or $60,000 to $120,000 in the US.
Software Engineers are also in demand, with salaries ranging from ₹5,00,000 to ₹12,00,000 per year, or $70,000 to $110,000 in the US. This role involves designing, developing, and testing software applications.
DevOps Engineers play a crucial role in ensuring the smooth operation of software systems. Their salaries can range from ₹8,00,000 to ₹18,00,000 per year, or $80,000 to $140,000 in the US.
Technical Leads and Architects are often responsible for overseeing the technical direction of a project. Their salaries can range from ₹12,00,000 to ₹25,00,000 per year, or $100,000 to $180,000 in the US.
Here's a summary of the career roles and their salary ranges:
Python Interview Question
Interviews are a crucial aspect of job recruitment, and preparing for them can significantly increase your chances of getting hired sooner.
Interviews are most important for job recruitment and you need to prepare for interviews if you want to get job sooner.
To prepare for interviews, it's essential to familiarize yourself with common interview questions, such as the ones for Django Developers found on the Top 50 Django Interview Questions and Answers page.
Interview questions can vary depending on the job role, but being prepared can give you an edge over other candidates.
Django Developers, for instance, can expect to be asked questions like those found on the Top 50 Django Interview Questions and Answers page.
Recommended read: Best Css Frameworks
Frequently Asked Questions
Is Django a web framework?
Yes, Django is a high-level Python web framework. It enables rapid web development with a focus on clean design and efficient coding.
Is Django a frontend or backend?
Django is a backend framework, handling server-side logic such as database management and user authentication. It focuses on the behind-the-scenes work, leaving frontend development to other tools and frameworks.
Is Django for frontend or backend?
Django is primarily used for backend web development, with some support for front-end development. It's ideal for building web apps efficiently, especially for the backend.
Is Django an API framework?
Django REST framework is a toolkit for building Web APIs, but Django itself is a high-level Python web framework that can be used to build APIs or traditional web applications. It's a powerful foundation for building robust and scalable web applications, including APIs.
Is Django easy to learn?
Django is a complex web framework that requires existing knowledge of Python fundamentals and another programming language to learn efficiently. Its complexity makes it a challenging but rewarding skill to acquire.
Sources
- https://code.visualstudio.com/docs/python/tutorial-django
- https://realpython.com/get-started-with-django-1/
- https://www.geeksforgeeks.org/django-tutorial/
- https://learn.microsoft.com/en-us/visualstudio/python/learn-django-in-visual-studio-step-01-project-and-solution
- https://www.geeksforgeeks.org/python-web-development-django/
Featured Images: pexels.com